Detailed explanation of Javascript heap sort algorithm
This article mainly introduces the Javascript heap sorting algorithm and its examples. It is very practical. Friends in need can refer to it.
Heap sorting is divided into two processes:
1. Build a heap.
A heap is essentially a complete binary tree, which must satisfy: the keyword of any non-leaf node in the tree is not greater than (or not less than) the keyword of its left and right child nodes (if they exist).
The heap is divided into: large root heap and small root heap. The large root heap is used for ascending sorting, and the small root heap is used for descending sorting.
If it is a large root heap, adjust the node with the largest value to the root of the heap through the adjustment function.
2. Save the heap root at the tail and call the adjustment function on the remaining sequences. After the adjustment is completed, save the maximum heap at the tail -1 (-1, -2,..., -i) , then adjust the remaining sequences, and repeat this process until the sorting is completed.
//调整函数 function headAdjust(elements, pos, len){ //将当前节点值进行保存 var swap = elements[pos]; //定位到当前节点的左边的子节点 var child = pos * 2 + 1; //递归,直至没有子节点为止 while(child < len){ //如果当前节点有右边的子节点,并且右子节点较大的场合,采用右子节点 //和当前节点进行比较 if(child + 1 < len && elements[child] < elements[child + 1]){ child += 1; } //比较当前节点和最大的子节点,小于则进行值交换,交换后将当前节点定位 //于子节点上 if(elements[pos] < elements[child]){ elements[pos] = elements[child]; pos = child; child = pos * 2 + 1; } else{ break; } elements[pos] = swap; } } //构建堆 function buildHeap(elements){ //从最后一个拥有子节点的节点开始,将该节点连同其子节点进行比较, //将最大的数交换与该节点,交换后,再依次向前节点进行相同交换处理, //直至构建出大顶堆(升序为大顶,降序为小顶) for(var i=elements.length/2; i>=0; i--){ headAdjust(elements, i, elements.length); } } function sort(elements){ //构建堆 buildHeap(elements); //从数列的尾部开始进行调整 for(var i=elements.length-1; i>0; i--){ //堆顶永远是最大元素,故,将堆顶和尾部元素交换,将 //最大元素保存于尾部,并且不参与后面的调整 var swap = elements[i]; elements[i] = elements[0]; elements[0] = swap; //进行调整,将最大)元素调整至堆顶 headAdjust(elements, 0, i); } } var elements = [3, 1, 5, 7, 2, 4, 9, 6, 10, 8]; console.log('before: ' + elements); sort(elements); console.log(' after: ' + elements);
Efficiency:
Time complexity: Best: O(nlog2n), Worst: O(nlog2n), Average: O(nlog2n).
Space complexity: O(1).
Stability: Unstable
The above is the entire content of this chapter. For more related tutorials, please visit JavaScript Video Tutorial!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
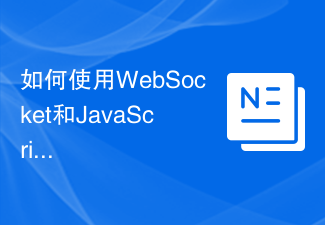
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
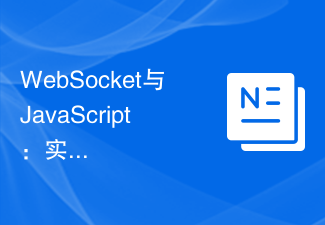
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
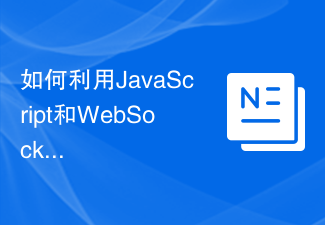
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
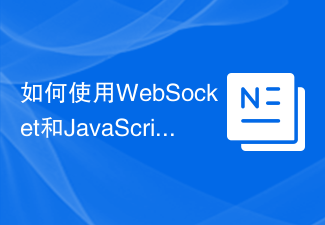
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
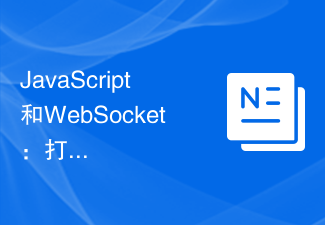
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
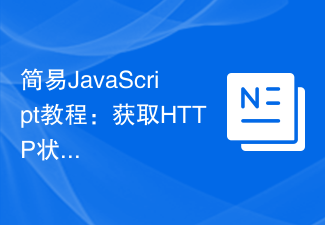
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
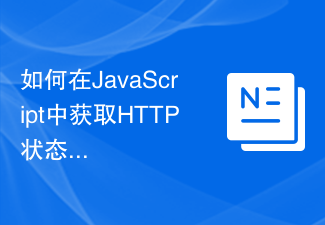
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
