JavaScript event delegation and jQuery event binding on, off and one
1. Event delegation
What is event delegation? A realistic understanding is: 100 students receive express delivery at noon on a certain day at the same time, but it is impossible for these 100 students to stand at the school gate waiting at the same time, so they will entrust the doorman to collect it, and then hand it over to the students one by one. .
In jQuery, we use the event bubbling feature to allow events bound to child elements to bubble up to the parent element (or ancestor element)
, and then perform related processing.
If an enterprise-level application does report processing, the table has 2000 rows, and each row has a button for processing. If you use
previous .bind() processing, then you need to bind 2000 events, just like 2000 students standing at the school gate waiting for
express delivery, which will constantly block the intersection and cause various accidents. The same situation applies to the page, which may cause the page
to be extremely slow or directly abnormal. Moreover, if 2000 buttons are paginated using ajax, the .bind() method cannot dynamically bind elements that
do not exist yet. For example, if the courier cannot verify the identity of a newly transferred student, he may not receive the courier.
//HTML part
<div style="background:red;width:200px;height:200px;" id="box"> <input type="button" value="按钮" class="button" /> </div>
$('.button').bind('click', function () { $(this).clone().appendTo('#box'); });
$('.button').live('click', function () { $(this).clone().appendTo('#box'); });
to $(document) once, instead of 2000 times. Then you can handle the click event of the subsequent dynamically loaded button. When accepting any
event, the $(document) object will check the event type (event.type) and event target (event.target). If the click
event is .button, then the processing delegated to it will be performed. program. The .live() method has been deleted and cannot be used. If you need
to test and use it, you need to introduce a backward compatible plug-in.
//.live()无法使用链接连缀调用,因为参数的特性导致 $('#box').children(0).live('click', function () { $(this).clone().appendTo('#box'); });
One is copy event behavior, and the other is event delegation. Under non-cloning operations, such functions can only use event delegation.
$('.button').live('click', function () { $('<input type="button" value="复制的" class="button" />').appendTo('#box'); });
When we need to stop event delegation, we can use .die() to cancel it.
$('.button').die('click'); 由于.live()和.die()在jQuery1.4.3 版本中废弃了,之后推出语义清晰、减少冒泡传播层次、 又支持链接连缀调用方式的方法:.delegate()和.undelegate()。但这个方法在jQuery1.7 版本中 被.on()方法整合替代了。 $('#box').delegate('.button', 'click', function () { $(this).clone().appendTo('#box'); }); $('#box').undelegate('.button','click'); //支持连缀调用方式 $('div').first().delegate('.button', 'click', function () { $(this).clone().appendTo('div:first'); });
Note: .delegate() needs to specify the parent element, then the first parameter is the current element, the second parameter is the event method, and the third parameter is the execution function. Like the .bind() method, additional parameters can be passed. The .undelegate() and .unbind() methods can directly delete all events, such as: .undelegate('click'). You can also delete namespace events,
For example: .undelegate('click.abc').
Note: .live(), .delegate() and .bind() methods are both event bindings, so the difference is obvious. In terms of usage,
follows two rules: 1. Many elements in the DOM When binding the same event; 2. When there is no upcoming
element binding event in the DOM; we recommend using the event delegate binding method, otherwise we recommend using the ordinary binding of .bind().
two. on, off and one
For this reason, jQuery 1.7 and later introduced the .on() and .off() methods to completely abandon the first three groups.
/
/替代.bind()方式 $('.button').on('click', function () { alert('替代.bind()'); }); //替代.bind()方式,并使用额外数据和事件对象 $('.button').on('click', {user : 'Lee'}, function (e) { alert('替代.bind()' + e.data.user); }); //替代.bind()方式,并绑定多个事件 $('.button').on('mouseover mouseout', function () { alert('替代.bind()移入移出!'); }); //替代.bind()方式,以对象模式绑定多个事件 $('.button').on({ mouseover : function () { alert('替代.bind()移入!'); }, mouseout : function () { alert('替代.bind()移出!'); } }); //替代.bind()方式,阻止默认行为并取消冒泡 $('form').on('submit', function () { return false; });
or
$('form').on('submit', false); //替代.bind()方式,阻止默认行为 $('form').on('submit', function (e) { e.preventDefault(); }); //替代.bind()方式,取消冒泡 $('form').on('submit', function (e) { e.stopPropagation(); }); //替代.unbind()方式,移除事件 $('.button').off('click'); $('.button').off('click', fn); $('.button').off('click.abc'); //替代.live()和.delegate(),事件委托 $('#box').on('click', '.button', function () { $(this).clone().appendTo('#box'); }); //替代.die()和.undelegate(),取消事件委托 $('#box').off('click', '.button'); 注意:和之前方式一样,事件委托和取消事件委托也有各种搭配方式,比如额外数据、 命名空间等等,这里不在赘述。 不管是.bind()还是.on(),绑定事件后都不是自动移除事件的,需要通过.unbind()和.off() 来手工移除。jQuery 提供了.one()方法,绑定元素执行完毕后自动移除事件,可以方法仅触 发一次的事件。 //类似于.bind()只触发一次 $('.button').one('click', function () { alert('one 仅触发一次!'); }); //类似于.delegate()只触发一次 $('#box).one('click', 'click', function () { alert('one 仅触发一次!'); });
<div> <input id="a" type="button" value="按钮1"> <input id="a" type="button" value="按钮2"> </div>
$('div').click(function(e){ alert('事件类型:'+ e.type + ',Value:' + $(e.target).val()); })
The above is the content of javascript event delegation and jQuery event binding on, off and one. For more related content, please pay attention to the PHP Chinese website (www.php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
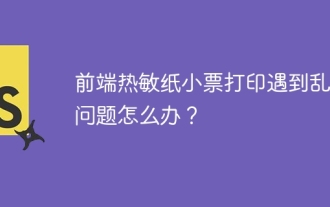
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
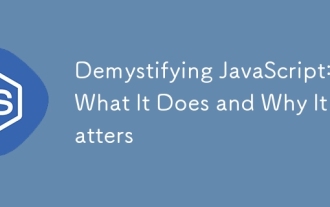
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
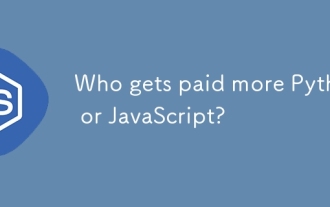
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
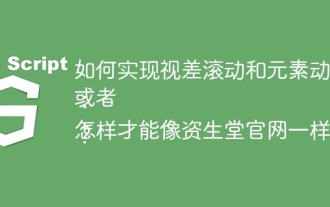
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
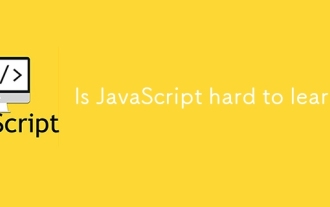
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
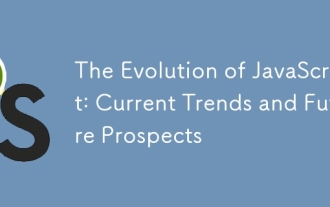
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
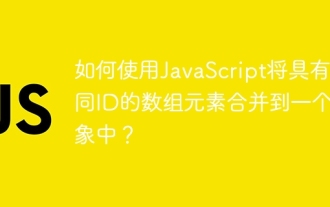
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
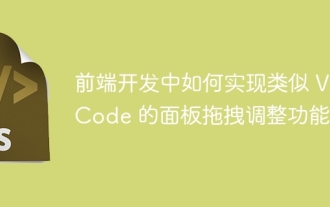
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
