Application of call and apply in javascript
This time I will bring you the application of call and apply in javascript, what are the precautions for the application of call and apply in javascript, the following is a practical case, let’s take a look .
Find the maximum and minimum values of the array
Define an array:
var ary = [23, 34, 24, 12, 35, 36, 14, 25];
Sort and then take the value method
First, perform the array Sorting (small -> large), the first and last are the minimum and maximum values we want.
var ary = [23, 34, 24, 12, 35, 36, 14, 25]; ary.sort(function (a, b) { return a - b; });var min = ary[0];var max = ary[ary.length - 1]; console.log(min, max);1234567
Assumption method
Assume that the first value in the current array is the maximum value, and then compare this value with the following items one by one. If one of the latter values is greater than the assumed value Hit, indicating that the assumption is wrong, we replace the assumed value...
var max = ary[0], min = ary[0];for (var i = 1; i < ary.length; i++) { var cur = ary[i]; cur > max ? max = cur : null; cur < min ? min = cur : null; } console.log(min, max);1234567
The max/min method in Math is implemented (through apply)
Use Math.min
directlyvar min = Math.min(ary); console.log(min); // NaN console.log(Math.min(23, 34, 24, 12, 35, 36, 14, 25));
When using Math.min directly, you need to pass the pile of numbers to be compared one by one, so that you can get the final demerit. It is not possible to put an ary array in at once.
Try: Use eval
var max = eval(“Math.max(” + ary.toString() + “)”); console.log(max); var min = eval(“Math.min(” + ary.toString() + “)”); console.log(min); “Math.max(” + ary.toString() + “)” –> “Math.max(23,34,24,12,35,36,14,25)”
Don’t worry about anything else first, first change the last code we want to execute into String, and then put it in the array The value of each item is spliced into this string separately.
eval: Convert a string into a JavaScript expression for execution
For example: eval(“12+23+34+45”) // 114
Call through apply max/min in Math
var max = Math.max.apply(null, ary); var min = Math.min.apply(null, ary); console.log(min, max);
In non-strict mode, when the first parameter to apply is null, this in max/min will be pointed to window, and then the ary parameters will be passed one by one. Give max/min.
Find the average
Now simulate a scenario and conduct a certain competition. After the judges score, they are required to remove the highest score and the lowest score, and the average of the remaining scores is for the final score.
Maybe many students will think of writing a method to receive all the scores, and then use the built-in attribute arguments of the function to sort the arguments by calling the sort method, and then..., but it should be noted that arguments are not A real array object is just a collection of pseudo-arrays, so directly calling the sort method with arguments will report an error:
arguments.sort(); // Uncaught TypeError: arguments.sort is not a function
So at this time, can you convert the arguments into a real array first? , and then proceed with the operation. According to this idea, we implement a business method to achieve the requirements of the question:
function avgFn() { // 1、将类数组转换为数组:把arguments克隆一份一模一样的数组出来 var ary = []; for (var i = 0; i < arguments.length; i++) { ary[ary.length] = arguments[i]; } // 2、给数组排序,去掉开头和结尾,剩下的求平均数 ary.sort(function (a, b) { return a - b; }); ary.shift(); ary.pop(); return (eval(ary.join('+')) / ary.length).toFixed(2); }var res = avgFn(9.8, 9.7, 10, 9.9, 9.0, 9.8, 3.0); console.log(res);1234567891011121314151617
We found that there is a step in the avgFn method we implemented to clone the arguments and generate them. is an array. If you are familiar with the slice method of arrays, you can know that when no parameters are passed to the slice method, the current array is cloned, which can be simulated as:
function mySlice () { // this->当前要操作的这个数组ary var ary = []; for (var i = 0; i < this.length; i++) { ary[ary.length] = this[i]; } return ary; };var ary = [12, 23, 34];var newAry = mySlice(ary); console.log(newAry);1234567891011
So in the avgFn method, the arguments are converted into arrays The operation can be borrowed from the slice method in Array through the call method.
function avgFn() { // 1、将类数组转换为数组:把arguments克隆一份一模一样的数组出来 // var ary = Array.prototype.slice.call(arguments); var ary = [].slice.call(arguments); // 2、给数组排序,去掉开头和结尾,剩下的求平均数....123 }
Our current approach is to convert arguments into an array first, and then operate the converted array. Can we just use arguments directly instead of converting them into an array first? Of course it is possible, it can be achieved by borrowing the array method through call.
function avgFn() { Array.prototype.sort.call(arguments , function (a, b) { return a - b; }); [].shift.call(arguments); [].pop.call(arguments); return (eval([].join.call(arguments, '+')) / arguments.length).toFixed(2); }var res = avgFn(9.8, 9.7, 10, 9.9, 9.0, 9.8, 3.0); console.log(res);123456789101112
Convert class array to array
As mentioned before, use the slice method of the array to convert the array-like object into an array, then obtain it through getElementsByTagName and other methods Can an array-like object also use the slice method to convert it into an array object?
var oLis = document.getElementsByTagName('div');
var ary = Array.prototype.slice.call(oLis);
console.log(ary);
In standard It can indeed be used in this way under the browser, but it will be a tragedy under IE6~8, and an error will be reported:
SCRIPT5014: Array.prototype.slice: 'this' is not a JavaScript object (error report)
Then in Under IE6~8, you can only add them to the array one by one through a loop:
for (var i = 0; i < oLis.length; i++) { ary[ary.length] = oLis[i]; }
Note: There is no compatibility problem with the method of borrowing arrays for arguments.
Based on the difference between IE6~8 and standard browsers, extract the tool class for converting array-like objects into arrays:
function listToArray(likeAry) { var ary = []; try { ary = Array.prototype.slice.call(likeAry); } catch (e) { for (var i = 0; i < likeAry.length; i++) { ary[ary.length] = likeAry[i]; } } return ary; }1234567891011
This tool method uses browser exceptions Information capture, so let’s introduce it here.
console.log(num);
When we output an undefined variable, an error will be reported: Uncaught ReferenceError: num is not defined. In JavaScript, this line will report an error, and the following code will not Executed again.
But if try..catch is used to capture exception information, it will not affect the execution of the following code. If an error occurs during the execution of the code in try, the try in catch will be executed by default {
console.log(num); } catch (e) { // 形参必须要写,我们一般起名为e console.log(e.message); // –> num is not defined 可以收集当前代码报错的原因 } console.log(‘ok’);
So the usage format of try...catch is (very similar to Java):
try { // } catch (e) { // 如果代码报错执行catch中的代码 } finally { // 一般不用:不管try中的代码是否报错,都要执行finally中的代码 }
At this time, I want to capture the information, but I don’t want the following diamante to be executed. So what should be done?
try { console.log(num); } catch (e) { // console.log(e.message); // --> 可以得到错误信息,把其进行统计 // 手动抛出一条错误信息,终止代码执行 throw new Error('当前网络繁忙,请稍后再试'); // new ReferenceError --> 引用错误 // new TypeError --> 类型错误 // new RangeError --> 范围错误 }console.log('ok');
I believe you have mastered the method after reading the case in this article. For more exciting information, please pay attention to other related articles on the php Chinese website!
Recommended reading:
What are the differences between call, apply and bind in javascript
Detailed explanation of call in javascript
The above is the detailed content of Application of call and apply in javascript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










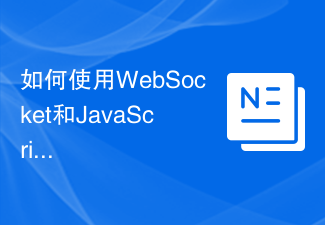
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
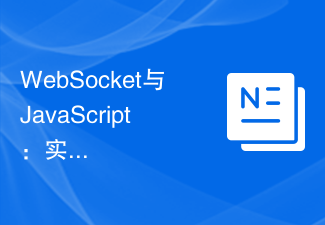
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
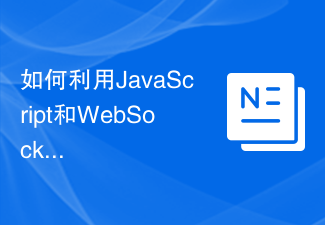
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
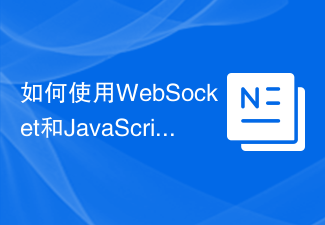
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
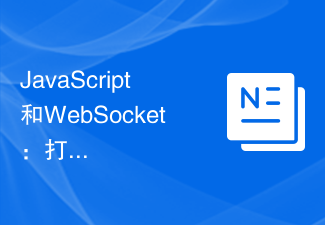
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
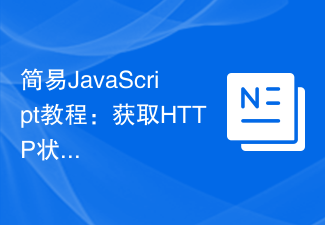
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
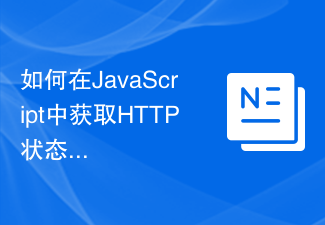
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
