How to use ajax to submit comments and automatically refresh them
This time I will show you how to use ajax to submit comments and automatically refresh them. What are the precautions for using ajax to submit comments and automatically refresh them. The following is a practical case, let's take a look.
After trying many times, I finally got it, let’s code it. (I use jQuery's ajax, not native)
js code:
<script> $(document).ready(function () { getcomment(); $('.comment-box button').click(function () { var comment_text = $('.comment-box textarea').val(); $.ajax({ type: 'POST', url: '/bbs/article/{{ article_list.id }}/comment/', data: {comment: comment_text}, success:function (callback) { var data = $.parseJSON(callback); $('.callback').html(data.result); if(data.result === 'successfully') { getcomment(); } } }) }); }); function getcomment() { $.ajax({ type: 'GET', url: '/bbs/article/{{ article_list.id }}/get_comment/', success:function (call) { var datas = $.parseJSON(call); $('.comment-list').html(datas.answer); } }) } </script>
Call the getcomment() function after the full text is loaded, get the comments from the database, and submit the comments written by yourself Then call the getcomment() function again to automatically refresh the
html template (the bootstrap template is used):
<p class="row"> <p class="comment-list" style="margin-left: 10px"> </p> </p> <p class="row"> <article class="col-xs-12"> <h4>请评论:</h4> <p class="comment-box"> <textarea class="form-control" rows="3"></textarea> <span class="callback"></span><button type="submit" class="btn btn-success pull-right" style="max-width: 5px;">评论</button> </p> </article> </p> <hr>
View function:
@csrf_exempt def comment(request,article_id): if request.method == 'POST': comments = request.POST['comment'] if len(comments) < 5: result = u'评论数需大于5' return HttpResponse(json.dumps({'result': result})) else: result = 'successfully' Comment.objects.create(content= comments, article_id=article_id) return HttpResponse(json.dumps({'result': result}))
This It is the function to submit comments. Don’t forget to add the csrf decorator
def get_comment(request, article_id): article_list = get_object_or_404(Article, id=article_id) comments = article_list.comment_set.all() html = '' for i in comments: ele = '<p class="row"><article class="col-xs-12"><p class="pull-right"><span class="label label-default">作者:' + 'i.user' + '</span></p><p>' + i.content + '<ul class="list-inline"><li><a href="#" rel="external nofollow" ></a></li></ul></article></p><hr>' html += ele return HttpResponse(json.dumps({'answer': html}))
The function to get comments in the background.
Finally clear the value of textarea:
function resettext() { $('.form-control').val(''); }
I believe you have mastered the method after reading the case in this article. For more exciting information, please pay attention to other related articles on the PHP Chinese website!
Recommended reading:
Detailed tutorial on making a shopping cart with Ajax+PHP
How to implement AJAX paging effect
The above is the detailed content of How to use ajax to submit comments and automatically refresh them. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










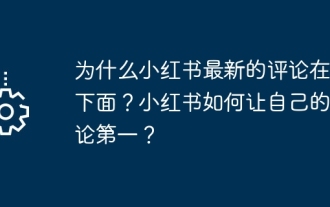
As a popular social e-commerce platform, Xiaohongshu’s user comments are an important interactive link. Many users find that on Xiaohongshu, the latest comments often appear at the bottom of the comment area. Why is this? This article will delve into the sorting mechanism of Xiaohongshu’s comment area and share some tips for ranking comments at the front. 1. Why are the latest comments on Xiaohongshu at the bottom? The default sorting method of the Xiaohongshu comment area is to display comments in chronological order, that is, the latest comments will be displayed at the bottom of the comment area. This sorting method helps users easily view the latest comments and obtain real-time information. Xiaohongshu encourages interaction between users and encourages users to actively participate in comments by placing the latest comments at the bottom of the page. This design allows users to browse other users’ comments,
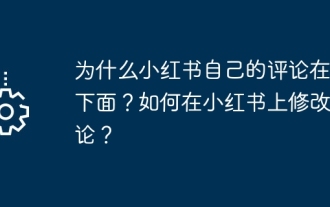
Xiaohongshu is a popular social e-commerce platform where users can share shopping tips, recommend products and exchange shopping experiences. Some users found that comments they posted on Xiaohongshu were always displayed at the bottom, which confused them. This article will explore why Xiaohongshu’s comments arrangement causes user comments to appear at the bottom, and introduce how to modify the position of comments on Xiaohongshu. 1. Why are Xiaohongshu’s own comments at the bottom? The display order of comments on Xiaohongshu is usually based on the chronological order of the comments. If your comment is posted after another user has commented, it will appear at the bottom. This is Xiaohongshu’s default comment sorting method, which is designed to maintain order and clarity in the comment area. Xiaohongshu may based on the content of the comments
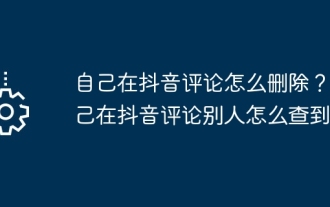
People enjoy posting videos, browsing videos, commenting and interacting on Douyin. But in the comment interaction, sometimes we may make some inappropriate remarks or make mistakes and want to delete these comments. So how to delete my own comments on Douyin? 1. How to delete my comments on Douyin? 1. Log in to Douyin App and find the video with the comment you want to delete. 2. At the bottom of the video page, find a list of all comments. 3. Find your comment, click the heart icon (like) on the right side of the comment, then click the delete icon (trash can) to confirm deletion. 4. If you @ other people in the comment, you will receive a prompt when deleting the comment: "After deleting this comment, the person @ will not receive the notification." If you want the person @ to receive the notification, you can Cancel @ first,
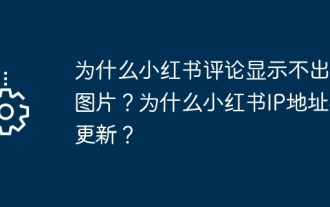
Xiaohongshu is a popular social e-commerce platform where users can share their shopping experiences and recommend products. Some users reported that they were troubled by the fact that the images they uploaded in their comments were not displayed properly. This article will help you find out why images in Xiaohongshu comments cannot be displayed and provide solutions. 1. Why can’t pictures be displayed in Xiaohongshu comments? Image formats may not be supported: Xiaohongshu’s comment function only supports limited image formats. If the image format you upload is not supported, the image may not be displayed. It is recommended that you try other formats, such as JPG, PNG, etc. The size of images uploaded in comments may be limited by Xiaohongshu. If the size of your image is too large, it may not be displayed properly. It is recommended that you try pressing

Xiaohongshu is a popular social media platform where users can share their lives and exchange experiences. Some users will find that when they comment on other people's posts, they cannot see it. So, why can’t others see my comments on Xiaohongshu? This article will explore this issue in detail to help you resolve your confusion. 1. Why can’t others see my comments on Xiaohongshu? Delayed review: Xiaohongshu review content has been reviewed and may be temporarily hidden due to containing sensitive words or illegal content. In this case, the other party may not be able to see the comment temporarily after it is posted. Generally, these comments will reappear once moderation is complete. Account anomalies may cause comments posted by commenters not to be seen by the other party, even if the comments are successfully posted. Such abnormal situations include accounts being banned or functions limited.
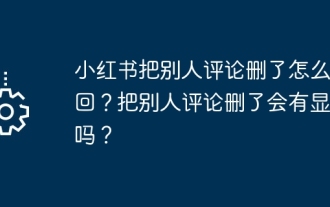
Xiaohongshu is a popular social e-commerce platform, and interactive comments between users are an indispensable method of communication on the platform. Occasionally, we may find that our comments have been deleted by others, which can be confusing. 1. How can I retrieve someone else’s deleted comments on Xiaohongshu? When you find that your comments have been deleted, you can first try to directly search for relevant posts or products on the platform to see if you can still find the comment. If the comment is still displayed after being deleted, it may have been deleted by the original post owner. At this time, you can try to contact the original post owner to ask the reason for deleting the comment and request to restore the comment. If a comment has been completely deleted and cannot be found on the original post, the chances of it being reinstated on the platform are relatively slim. You can try other ways
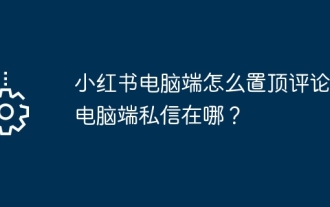
On Xiaohongshu, users can browse a variety of beauty, fashion, travel and other content, while also expressing their own opinions and comments. Some users encountered the problem of how to pin comments when using the Xiaohongshu computer version. 1. How to pin comments to the top of Xiaohongshu on the computer? 1. Open the computer version of Xiaohongshu and enter the post or update you want to comment on. 2. In the comment area, find the comment you want to pin. 3. Click on the comment and you will see a "Pin" button. 4. Click the "Pin" button, and the comment will appear at the top of the comment area and become a pinned comment. It should be noted that the Xiaohongshu computer version currently only supports pinning your own comments, and the pinning function is only valid in the comment area of posts or updates, and cannot be pinned on topic pages or global comment areas. two,
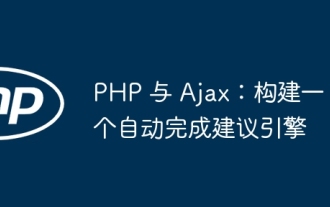
Build an autocomplete suggestion engine using PHP and Ajax: Server-side script: handles Ajax requests and returns suggestions (autocomplete.php). Client script: Send Ajax request and display suggestions (autocomplete.js). Practical case: Include script in HTML page and specify search-input element identifier.
