How to use Ajax to dynamically load data
This time I will show you how to use Ajax to dynamically load data. What are the precautions for Ajax to dynamically load data? The following is a practical case, let's take a look.
Foreword:
1. This essay implements an example of Ajax dynamic loading. 2. Implemented using the .net MVC framework. 3. This example focuses on the front-end and back-end interactions, and the others are abbreviated.Start:
1.ControllerActionResult code (used to display the page)
/// <summary> /// 电话查询页面 /// </summary> /// <returns></returns> public ActionResult PhoneSearch(string sql) { phoneList=从数据库查询数据; ViewBag.phoneList = phoneList; return View(); }
<table border="1" cellspacing="0" cellpadding="0" class="toLang" id="phoneTable"> <tr> <th>序号</th> <th>公司</th> <th>部门</th> <th>小组</th> <th>姓名</th> <th>职位</th> <th>电话</th> </tr> <tbody id="todeListTBODY"> @if (ViewBag.phoneList != null) { foreach (var item in ViewBag.phoneList) { number = number + 1; <tr> <td>@number</td> <td>@item.Conpany</td> <td>@item.Department</td> <td>@item.Team</td> <td>@item.Name</td> <td>@item.Position</td> <td>@item.PhoneNumber</td> </tr> } } </tbody> </table>
<p style="display:block;float:left; width:100%; "> 公司: <select class="InputTestStyle" id="company" onclick="initDeptSelect()"> <option>==请选择公司==</option> </select> 部门: <select class="InputTestStyle" id="department" onclick="initGroupSelect()"> <option>==请选择公司==</option> </select> 小组: <select class="InputTestStyle" id="group" onclick="QueryPhoneNum()"> <option>==请选择公司==</option> </select> </p>
JavaScript code
//打开页面的时候执行 window.onunload = initCompanySelect(); //初始化“公司”下拉框 function initCompanySelect() { $.ajax({ type: 'POST', url: '/Home/GetCompantListForPhone', dataType: 'json', data: { }, success: function (data) { //1.清空这个下拉框的数据 // $('#company option').remove();//也能成功实现 $('#company').empty(); $("#company").append($('<option>' + '==请选择公司==' + '</option>')); //2.将返回值动态加载进下拉框,动态生成标签。 for (i = 0; i < data.length;i++) { $("#company").append($('<option >' + data[i].Conpany + '</option>')); } }, error: function (XMLHttpRequest, textStatus, errorThown) { alert("操作失败!"); } }) }
/// <summary> /// 获取电话查询公司下拉数据 /// </summary> /// <returns></returns> [HttpPost] public JsonResult GetCompantListForPhone() { compantList = 从数据库获取这个下拉框数据的集合; return Json(compantList); }
//根据条件查询电话 function QueryPhoneNum() { if ($('#group').val() == '==请选择小组==') { return; } number = 0; $.ajax({ type: 'POST', url: '/Home/PhoneSearchSubmit', dataType: 'json', data: { company:$('#company').val(), dept: $('#department').val(), group: $('#group').val() }, success: function (phoneList) { //1.清空这个表格的数据 $('#todeListTBODY tr').remove(); //2.将返回值动态加载进表格。 $.each(phoneList, function (index, element) { number = number + 1; $('#todeListTBODY').prepend(function (i) { return "<tr>" + "<td>" +number + "<td>" + element.Conpany + "<td>" + element.Department + "<td>" + element.Team + "<td>" + element.Name + "<td>" + element.Position + "<td>" + element.PhoneNumber + "</tr>"; }) }) }, error: function (XMLHttpRequest, textStatus, errorThown) { alert("操作失败!"); } }) }
/// <summary> /// 电话查询 /// </summary> /// <returns></returns> [HttpPost] public JsonResult PhoneSearchSubmit(string company, string dept, string group) { phoneList = 根据条件查询数据; return Json(phoneList); }
How to handle errors when returning json data to ajax in spring mvc
How to upload files with Ajax Progress bar Codular
The above is the detailed content of How to use Ajax to dynamically load data. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










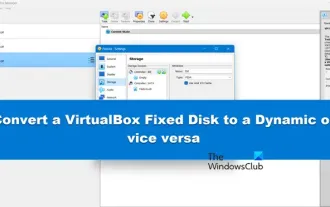
When creating a virtual machine, you will be asked to select a disk type, you can select fixed disk or dynamic disk. What if you choose fixed disks and later realize you need dynamic disks, or vice versa? Good! You can convert one to the other. In this post, we will see how to convert VirtualBox fixed disk to dynamic disk and vice versa. A dynamic disk is a virtual hard disk that initially has a small size and grows in size as you store data in the virtual machine. Dynamic disks are very efficient at saving storage space because they only take up as much host storage space as needed. However, as disk capacity expands, your computer's performance may be slightly affected. Fixed disks and dynamic disks are commonly used in virtual machines
![Error loading plugin in Illustrator [Fixed]](https://img.php.cn/upload/article/000/465/014/170831522770626.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
When launching Adobe Illustrator, does a message about an error loading the plug-in pop up? Some Illustrator users have encountered this error when opening the application. The message is followed by a list of problematic plugins. This error message indicates that there is a problem with the installed plug-in, but it may also be caused by other reasons such as a damaged Visual C++ DLL file or a damaged preference file. If you encounter this error, we will guide you in this article to fix the problem, so continue reading below. Error loading plug-in in Illustrator If you receive an "Error loading plug-in" error message when trying to launch Adobe Illustrator, you can use the following: As an administrator
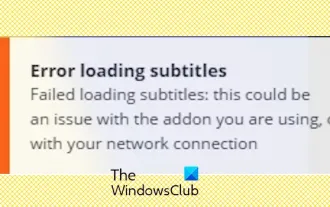
Subtitles not working on Stremio on your Windows PC? Some Stremio users reported that subtitles were not displayed in the videos. Many users reported encountering an error message that said "Error loading subtitles." Here is the full error message that appears with this error: An error occurred while loading subtitles Failed to load subtitles: This could be a problem with the plugin you are using or your network. As the error message says, it could be your internet connection that is causing the error. So please check your network connection and make sure your internet is working properly. Apart from this, there could be other reasons behind this error, including conflicting subtitles add-on, unsupported subtitles for specific video content, and outdated Stremio app. like
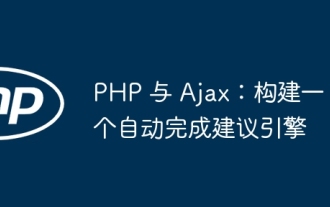
Build an autocomplete suggestion engine using PHP and Ajax: Server-side script: handles Ajax requests and returns suggestions (autocomplete.php). Client script: Send Ajax request and display suggestions (autocomplete.js). Practical case: Include script in HTML page and specify search-input element identifier.
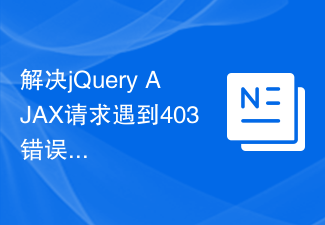
Title: Methods and code examples to resolve 403 errors in jQuery AJAX requests. The 403 error refers to a request that the server prohibits access to a resource. This error usually occurs because the request lacks permissions or is rejected by the server. When making jQueryAJAX requests, you sometimes encounter this situation. This article will introduce how to solve this problem and provide code examples. Solution: Check permissions: First ensure that the requested URL address is correct and verify that you have sufficient permissions to access the resource.
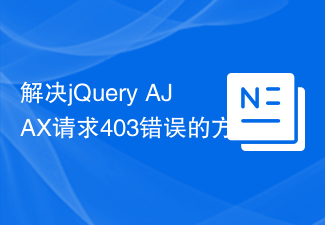
jQuery is a popular JavaScript library used to simplify client-side development. AJAX is a technology that sends asynchronous requests and interacts with the server without reloading the entire web page. However, when using jQuery to make AJAX requests, you sometimes encounter 403 errors. 403 errors are usually server-denied access errors, possibly due to security policy or permission issues. In this article, we will discuss how to resolve jQueryAJAX request encountering 403 error
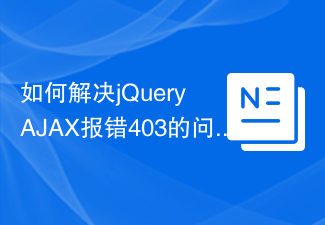
How to solve the problem of jQueryAJAX error 403? When developing web applications, jQuery is often used to send asynchronous requests. However, sometimes you may encounter error code 403 when using jQueryAJAX, indicating that access is forbidden by the server. This is usually caused by server-side security settings, but there are ways to work around it. This article will introduce how to solve the problem of jQueryAJAX error 403 and provide specific code examples. 1. to make
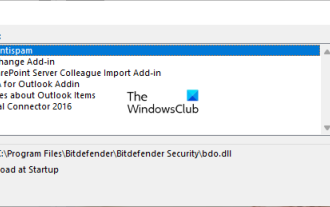
If you encounter freezing issues when inserting hyperlinks into Outlook, it may be due to unstable network connections, old Outlook versions, interference from antivirus software, or add-in conflicts. These factors may cause Outlook to fail to handle hyperlink operations properly. Fix Outlook freezes when inserting hyperlinks Use the following fixes to fix Outlook freezes when inserting hyperlinks: Check installed add-ins Update Outlook Temporarily disable your antivirus software and then try creating a new user profile Fix Office apps Program Uninstall and reinstall Office Let’s get started. 1] Check the installed add-ins. It may be that an add-in installed in Outlook is causing the problem.
