What are the inheritance methods in js
The officially released ES6 has encapsulated and implemented the inheritance## in other OO languages. #Form, Class Extends##, here mainly records the prototype inheritance and borrowing of jsConstructorInheritance
一, Prototype chain inheritancefunction Super(){
this.name="小明";
}
Super.prototype.sayName = function(){
alert(this.name)
};function Sub(){}
Sub.prototype = new Super();var instance = new Sub();
instance.sayName();//小明1234567891011
//When the super class contains reference type attribute values, multiple instances of one of the subclasses , as long as one instance reference attribute is modified only once, the reference type attribute value of other instances will also change immediately //The reason is that the super class attribute becomes the prototype attribute of the sub class function Super(){this.name=" Xiao Ming"; this.friends = ['Xiaohong','Xiaoqiang'];
}Super.prototype.sayName = function(){ alert(this.name) };function Sub(){} Sub.prototype = new Super();var instance1 = new Sub();var instance2 = new Sub(); instance1.friends.push('张三'); console.log(instance1.friends);//["小红", "小强", "张三"]console.log(instance2.friends);//["小红", "小强", "张三"]1234567891011121314151617
2. Borrowing constructor inheritance function Super(){this.name="小明"; this.friends = ['小红','小强'];
}
Super.prototype.sayName = function(){
alert(this.name)
};function Sub(){
Super.call(this);
}var instance1 = new Sub();var instance2 = new Sub();
instance1.friends.push('张三');
console.log(instance1.friends);//["小红", "小强", "张三"]console.log(instance2.friends);//["小红", "小强"]12345678910111213141516
If you use the borrowed constructor alone, you cannot inherit the prototype in the super class
Properties and methods3. Combined inheritance (prototypal inheritance borrows constructor inheritance)Combined inheritance is also the most commonly used inheritance method in actual development
function Super(){this.name="小明"; this.friends = ['小红','小强']; } Super.prototype.sayName = function(){ alert(this.name) };function Sub(){ Super.call(this); } Sub.prototype = new Super();var instance1 = new Sub();var instance2 = new Sub(); instance1.friends.push('张三'); console.log(instance1.friends);//["小红", "小强", "张三"]console.log(instance2.friends);//["小红", "小强"]instance1.sayName();//小明instance2.sayName();//小明12345678910111213141516171819
Problems with combined inheritance
//组合式继承中,超类的构造函数将被调用两次function Super(){this.name="小明"; this.friends = ['小红','小强']; } Super.prototype.sayName = function(){ alert(this.name) };function Sub(){ Super.call(this);//第二次调用} Sub.prototype = new Super();//第一次调用var instance = new Sub();1234567891011121314
4. Parasitic inheritance//When the main consideration is the object instead of defining the type and constructor yourself, parasitic inheritance It is a useful pattern, but it cannot reuse functions. function
createAnother(original){ var clone = Object(original);//创建一个新对象,original的副本 clone.sayName = function(){ //对象的增强,添加额外的方法 alert('hi'); } return clone; }var person = { name:'小明', friends:['小红','小强'] }var person1 = createAnother(person);var person2 = createAnother(person); person1.friends.push('张三'); console.log(person.friends);//["小红", "小强", "张三"]console.log(person1.friends);//["小红", "小强", "张三"]console.log(person2.friends);//["小红", "小强", "张三"]123456789101112131415161718
Parasitic combined inheritance
//寄生组合式继承解决了组合式继承调用两次超类构造函数的问题function inheritPrototype(sub,superr){var prototype = Object.create(superr.prototype);//ES5中 创建对象 副本的方法 prototype.constructor = superr; //弥补重写原型失去的默认constructor属性 sub.prototype = prototype; }function Super(name){ this.name = name; this.friends = ['小红','小强']; } Super.prototype.sayName = function(){ alert(this.name); }function Sub(name){ Super.call(this,name); } inheritPrototype(Sub,Super);var person1 = new Sub('小明');var person2 = new Sub('小华'); person1.friends.push('张三'); console.log(person1.friends);//["小红", "小强", "张三"]console.log(person2.friends);//["小红", "小强"]console.log(person1.sayName());//小明console.log(person2.sayName());//小华
The above are several inheritance methods in js that I have compiled for you. I hope it will be helpful to everyone in the future.
Related articles:
Specific steps on how to pass two parameters to the JS method in JS onclickjs basic syntax Detailed answer#Explanation of methods of defining classes in JSThe above is the detailed content of What are the inheritance methods in js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










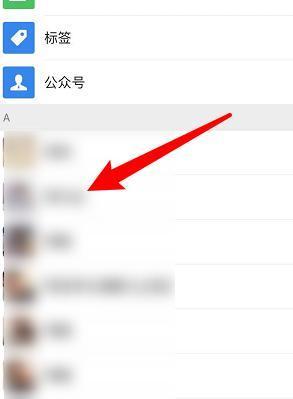
Unfortunately, people often delete certain contacts accidentally for some reasons. WeChat is a widely used social software. To help users solve this problem, this article will introduce how to retrieve deleted contacts in a simple way. 1. Understand the WeChat contact deletion mechanism. This provides us with the possibility to retrieve deleted contacts. The contact deletion mechanism in WeChat removes them from the address book, but does not delete them completely. 2. Use WeChat’s built-in “Contact Book Recovery” function. WeChat provides “Contact Book Recovery” to save time and energy. Users can quickly retrieve previously deleted contacts through this function. 3. Enter the WeChat settings page and click the lower right corner, open the WeChat application "Me" and click the settings icon in the upper right corner to enter the settings page.
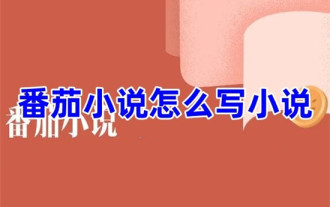
Tomato Novel is a very popular novel reading software. We often have new novels and comics to read in Tomato Novel. Every novel and comic is very interesting. Many friends also want to write novels. Earn pocket money and edit the content of the novel you want to write into text. So how do we write the novel in it? My friends don’t know, so let’s go to this site together. Let’s take some time to look at an introduction to how to write a novel. Share the Tomato novel tutorial on how to write a novel. 1. First open the Tomato free novel app on your mobile phone and click on Personal Center - Writer Center. 2. Jump to the Tomato Writer Assistant page - click on Create a new book at the end of the novel.
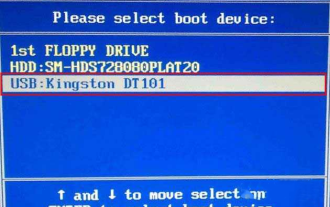
Colorful motherboards enjoy high popularity and market share in the Chinese domestic market, but some users of Colorful motherboards still don’t know how to enter the bios for settings? In response to this situation, the editor has specially brought you two methods to enter the colorful motherboard bios. Come and try it! Method 1: Use the U disk startup shortcut key to directly enter the U disk installation system. The shortcut key for the Colorful motherboard to start the U disk with one click is ESC or F11. First, use Black Shark Installation Master to create a Black Shark U disk boot disk, and then turn on the computer. When you see the startup screen, continuously press the ESC or F11 key on the keyboard to enter a window for sequential selection of startup items. Move the cursor to the place where "USB" is displayed, and then
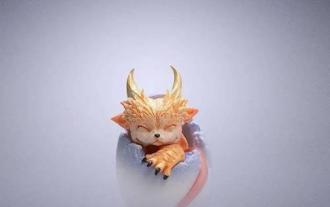
Mobile games have become an integral part of people's lives with the development of technology. It has attracted the attention of many players with its cute dragon egg image and interesting hatching process, and one of the games that has attracted much attention is the mobile version of Dragon Egg. To help players better cultivate and grow their own dragons in the game, this article will introduce to you how to hatch dragon eggs in the mobile version. 1. Choose the appropriate type of dragon egg. Players need to carefully choose the type of dragon egg that they like and suit themselves, based on the different types of dragon egg attributes and abilities provided in the game. 2. Upgrade the level of the incubation machine. Players need to improve the level of the incubation machine by completing tasks and collecting props. The level of the incubation machine determines the hatching speed and hatching success rate. 3. Collect the resources required for hatching. Players need to be in the game
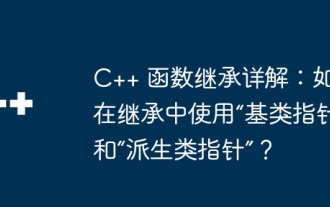
In function inheritance, use "base class pointer" and "derived class pointer" to understand the inheritance mechanism: when the base class pointer points to the derived class object, upward transformation is performed and only the base class members are accessed. When a derived class pointer points to a base class object, a downward cast is performed (unsafe) and must be used with caution.
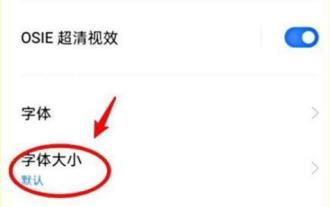
Setting font size has become an important personalization requirement as mobile phones become an important tool in people's daily lives. In order to meet the needs of different users, this article will introduce how to improve the mobile phone use experience and adjust the font size of the mobile phone through simple operations. Why do you need to adjust the font size of your mobile phone - Adjusting the font size can make the text clearer and easier to read - Suitable for the reading needs of users of different ages - Convenient for users with poor vision to use the font size setting function of the mobile phone system - How to enter the system settings interface - In Find and enter the "Display" option in the settings interface - find the "Font Size" option and adjust it. Adjust the font size with a third-party application - download and install an application that supports font size adjustment - open the application and enter the relevant settings interface - according to the individual
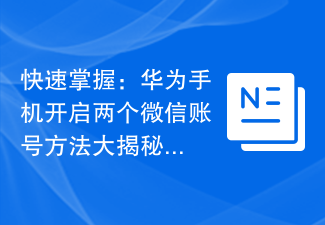
In today's society, mobile phones have become an indispensable part of our lives. As an important tool for our daily communication, work, and life, WeChat is often used. However, it may be necessary to separate two WeChat accounts when handling different transactions, which requires the mobile phone to support logging in to two WeChat accounts at the same time. As a well-known domestic brand, Huawei mobile phones are used by many people. So what is the method to open two WeChat accounts on Huawei mobile phones? Let’s reveal the secret of this method. First of all, you need to use two WeChat accounts at the same time on your Huawei mobile phone. The easiest way is to
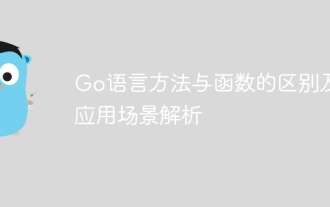
The difference between Go language methods and functions lies in their association with structures: methods are associated with structures and are used to operate structure data or methods; functions are independent of types and are used to perform general operations.
