How to delete an element in JS array
Now I will share with you a JS method to delete an element in an array. It has a good reference value and I hope it will be helpful to everyone.
Delete an element specified in the array
First, you can define a function for the JS array object to find the position of the specified element in the array, that is, the index , the code is:
Array.prototype.indexOf = function(val) { for (var i = 0; i < this.length; i++) { if (this[i] == val) return i; } return -1; };
Then use to get the index of this element, and use the js array's own inherent function to delete this element:
The code is:
Array.prototype.remove = function(val) { var index = this.indexOf(val); if (index > -1) { this.splice(index, 1); } };
This way Constructed such a function, for example, I have an array:
var emp = ['abs','dsf','sdf','fd']
If we want to delete the 'fd' in it, we can use:
emp.remove('fd');
Deleted array An item of
splice(index,len,[item]) Note: This method will change the original array.
splice has 3 parameters, it can also be used to replace/delete/add one or several values in the array
index: array starting subscript len: replacement/delete length item :Replacement value, if the delete operation is performed, the item will be empty
For example: arr = ['a','b','c','d']
Delete
//删除起始下标为1,长度为1的一个值(len设置1,如果为0,则数组不变) var arr = ['a','b','c','d']; arr.splice(1,1); console.log(arr); //['a','c','d']; //删除起始下标为1,长度为2的一个值(len设置2) var arr2 = ['a','b','c','d'] arr2.splice(1,2); console.log(arr2); //['a','d']
Replace
//替换起始下标为1,长度为1的一个值为‘ttt',len设置的1 var arr = ['a','b','c','d']; arr.splice(1,1,'ttt'); console.log(arr); //['a','ttt','c','d'] var arr2 = ['a','b','c','d']; arr2.splice(1,2,'ttt'); console.log(arr2); //['a','ttt','d'] 替换起始下标为1,长度为2的两个值为‘ttt',len设置的1
Add----len is set to 0, item is the added value
var arr = ['a','b','c','d']; arr.splice(1,0,'ttt'); console.log(arr); //['a','ttt','b','c','d'] 表示在下标为1处添加一项'ttt'<span style="font-size:14px;font-family:Arial, Helvetica, sans-serif;background-color:rgb(255,255,255);"> </span>
After the delete method deletes the elements in the array, it will set the subscripted value to undefined, and the length of the array will not change.
var arr = ['a','b','c','d']; delete arr[1]; arr; //["a", undefined × 1, "c", "d"] 中间出现两个逗号,数组长度不变,有一项为undefined
The above is what I compiled for everyone. I hope it will be helpful to everyone in the future. .
Related articles:
About how to use datepicker in vue2.0
JavaScript mediator mode (detailed tutorial)
How to use Dom elements in jQuery?
The above is the detailed content of How to delete an element in JS array. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










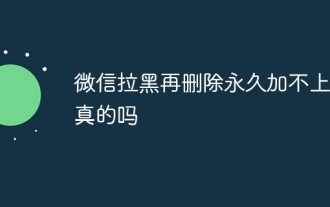
1. First of all, it is false to block and delete someone permanently and not add them permanently. If you want to add the other party after you have blocked them and deleted them, you only need the other party's consent. 2. If a user blocks someone, the other party will not be able to send messages to the user, view the user's circle of friends, or make calls with the user. 3. Blocking does not mean deleting the other party from the user's WeChat contact list. 4. If the user deletes the other party from the user's WeChat contact list after blocking them, there is no way to recover after deletion. 5. If the user wants to add the other party as a friend again, the other party needs to agree and add the user again.
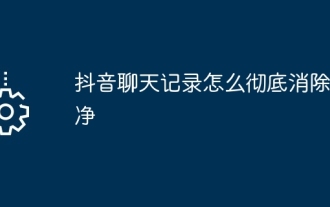
1. Open the Douyin app, click [Message] at the bottom of the interface, and click the chat conversation entry that needs to be deleted. 2. Long press any chat record, click [Multiple Select], and check the chat records you want to delete. 3. Click the [Delete] button in the lower right corner and select [Confirm deletion] in the pop-up window to permanently delete these records.
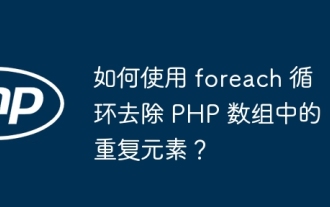
The method of using a foreach loop to remove duplicate elements from a PHP array is as follows: traverse the array, and if the element already exists and the current position is not the first occurrence, delete it. For example, if there are duplicate records in the database query results, you can use this method to remove them and obtain results without duplicate records.
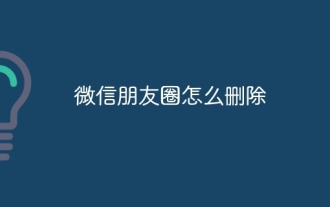
1. Open the WeChat app, click [Me] in the lower right corner, find and click the [Moments] option. 2. Click [My Moments] in the upper right corner and find the content in the Moments you want to delete on the My Moments interface. 3. Click to enter the details page of this circle of friends, and click the [small trash can] icon to the right of the content release time. 4. Select [OK] in the pop-up window, thus completing the operation of deleting the content in the circle of friends.

The performance comparison of PHP array key value flipping methods shows that the array_flip() function performs better than the for loop in large arrays (more than 1 million elements) and takes less time. The for loop method of manually flipping key values takes a relatively long time.
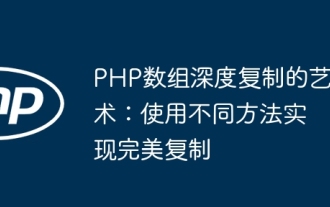
Methods for deep copying arrays in PHP include: JSON encoding and decoding using json_decode and json_encode. Use array_map and clone to make deep copies of keys and values. Use serialize and unserialize for serialization and deserialization.
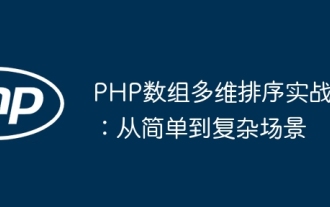
Multidimensional array sorting can be divided into single column sorting and nested sorting. Single column sorting can use the array_multisort() function to sort by columns; nested sorting requires a recursive function to traverse the array and sort it. Practical cases include sorting by product name and compound sorting by sales volume and price.
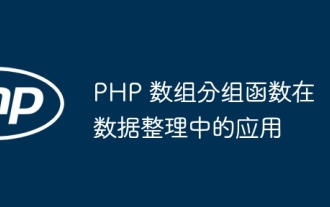
PHP's array_group_by function can group elements in an array based on keys or closure functions, returning an associative array where the key is the group name and the value is an array of elements belonging to the group.
