How to implement data transfer with vue+props
This time I will show you how to implement vue props to transfer data, and what are the precautions for vue props to transfer data. The following is a practical case, let's take a look.
In Vue, the relationship between parent and child components can be summarized as props are passed downwards and events are passed upwards. The parent component sends data to the child component through props, and the child component sends messages to the parent component through events. See how they work.
1. Basic Usage
Components are not just about reusing the content of the template. What's more important is communication between components.
In the component, use the option props to declare the data that needs to be received from the parent. The value of props can be two types, one is a string array, and the other is an object.
1.1 String array:
1 2 3 4 5 6 7 8 9 10 |
|
The result after rendering is: The data declared in
1 2 3 |
|
props and the data returned by the component data function are mainly The difference is that the props come from the parent, while the data in the data is the component's own data, and the scope is the component itself. Both types of data can be used in the template, computed properties, and methods.
The data message in the above example is passed from the parent through props. Write the name of the props directly on the custom label of the component. If you want to pass multiple data, just add items in the props array. .
Sometimes, the data passed is not directly hard-coded, but dynamic data from the parent. In this case, you can use the command v -bind to dynamically bind the value of props. When the data of the parent component changes , will also be passed to child components.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
|
A few points to note:
1. If you want to directly pass numbers, Boolean values, arrays, and objects, and do not use v-bind, only strings will be passed.
2. If you want to pass all the properties of an object as props, you can use v-bind without any parameters (that is, use v-bind instead of v -bind:prop-name). For example, a known todo object:
1.2 Object:
When prop needs to be verified, object writing is required.
Generally when your component needs to be provided to others, it is recommended to perform data verification. For example, a certain data must be of numeric type. If a string is passed in, a warning will pop up on the console.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
|
The verified type type can be:
• String
• Number
• Boolean
• Object
• Array
• Function
type can also be a custom constructor, detected using instanceof.
When prop verification fails, a warning will be thrown in the console under the development version.
2. One-way data flow
The biggest change between Vue 2.x and Vue l.x is that Vue2.x is passed through props The data is one-way, that is, when the data of the parent component changes, it will be passed to the child component, but not the other way around.
In business, we often encounter two situations where prop needs to be changed.
2.1 One is that the parent component passes in the initial value, and the subcomponent saves it as the initial value. It can be used and modified at will within the scope. (After Prop is passed in as the initial value, the subcomponent wants to use it as local data;)
In this case, you can declare another data in the component data and reference the prop of the parent component. The sample code is as follows :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
The data count is declared in the component. It will obtain the initCount from the parent component when the component is initialized. After that, it has nothing to do with it. It only maintains the count, so that you can avoid directly operating the initCount.
2.2 Prop is passed in as the original value that needs to be transformed. (Prop is passed in as raw data and is processed into other data output by the subcomponent.)
In this case, calculated properties are enough. The sample code is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
|
Note that objects and Arrays are reference types, pointing to the same memory space. If prop is an object or array, changing it inside the child component will affect the state of the parent component.
Я считаю, что вы освоили этот метод после прочтения примера, описанного в этой статье. Для получения более интересной информации обратите внимание на другие статьи по теме на китайском веб-сайте php!
Рекомендуется к прочтению:
Подробное объяснение использования древовидного меню с проверкой и каскадным выбором
Область использования комментариев в регулярных выражениях Что
The above is the detailed content of How to implement data transfer with vue+props. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
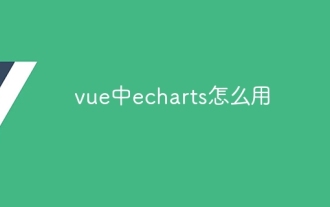
Using ECharts in Vue makes it easy to add data visualization capabilities to your application. Specific steps include: installing ECharts and Vue ECharts packages, introducing ECharts, creating chart components, configuring options, using chart components, making charts responsive to Vue data, adding interactive features, and using advanced usage.
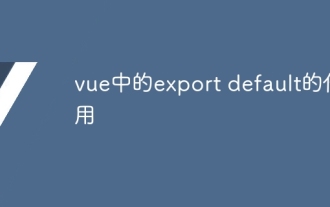
Question: What is the role of export default in Vue? Detailed description: export default defines the default export of the component. When importing, components are automatically imported. Simplify the import process, improve clarity and prevent conflicts. Commonly used for exporting individual components, using both named and default exports, and registering global components.
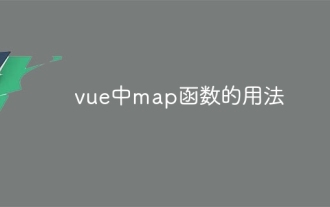
The Vue.js map function is a built-in higher-order function that creates a new array where each element is the transformed result of each element in the original array. The syntax is map(callbackFn), where callbackFn receives each element in the array as the first argument, optionally the index as the second argument, and returns a value. The map function does not change the original array.
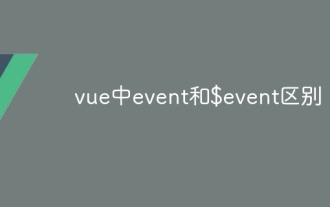
In Vue.js, event is a native JavaScript event triggered by the browser, while $event is a Vue-specific abstract event object used in Vue components. It is generally more convenient to use $event because it is formatted and enhanced to support data binding. Use event when you need to access specific functionality of the native event object.
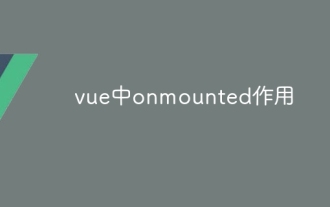
onMounted is a component mounting life cycle hook in Vue. Its function is to perform initialization operations after the component is mounted to the DOM, such as obtaining references to DOM elements, setting data, sending HTTP requests, registering event listeners, etc. It is only called once when the component is mounted. If you need to perform operations after the component is updated or before it is destroyed, you can use other lifecycle hooks.
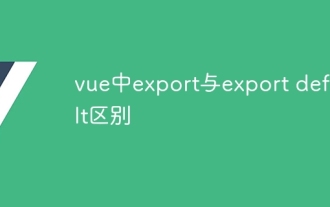
There are two ways to export modules in Vue.js: export and export default. export is used to export named entities and requires the use of curly braces; export default is used to export default entities and does not require curly braces. When importing, entities exported by export need to use their names, while entities exported by export default can be used implicitly. It is recommended to use export default for modules that need to be imported multiple times, and use export for modules that are only exported once.
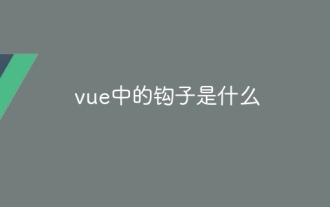
Vue hooks are callback functions that perform actions on specific events or lifecycle stages. They include life cycle hooks (such as beforeCreate, mounted, beforeDestroy), event handling hooks (such as click, input, keydown) and custom hooks. Hooks enhance component control, respond to component life cycles, handle user interactions and improve component reusability. To use hooks, just define the hook function, execute the logic and return an optional value.
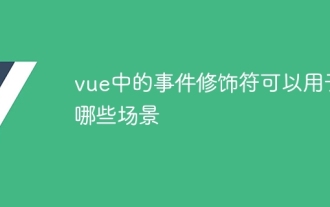
Vue.js event modifiers are used to add specific behaviors, including: preventing default behavior (.prevent) stopping event bubbling (.stop) one-time event (.once) capturing event (.capture) passive event listening (.passive) Adaptive modifier (.self)Key modifier (.key)
