About making a simple frame chart using JavaScript
This article mainly introduces JavaScript to create a simple frame chart in detail, which has certain reference value. Interested friends can refer to it
Story background: I encountered this in the past few days A customer is taking meeting minutes. During each meeting, a specific device will record the speaker's position and display it as an angle value. He gave me the angle values and asked me to make him a diagram showing an approximate position of each person.
The customer thought of using Echarts charts. The first thing I thought of was using Echarts. However, after thinking about his request, I realized that using Echarts to create a simple frame selection chart would be overkill, and There is also so much useless code to import.
So I thought of using canvas to imitate it, but after thinking about it again, canvas was not easy to operate. Can I use ordinary css combined with javascript to make it? This reflection proves that you must use your brain more when doing anything in order to find a simpler way to solve the problem.
Considering that maybe everyone will need it one day, I released it. Note: It has portability and can be moved to any position on the page, and the effect will not change
Let’s take a look at the final effect first:
Figure 1:
Picture 2:
<!doctype html> <html> <head> <meta charset="utf-8" /> <title>仿Echarts图表</title> <style> * { padding:0; margin:0; } #getcharts { position:relative; width:510px; height:510px; } #wrapcharts { list-style:none; height:500px; width:500px; border:2px solid #aaa; border-radius:50%; position:relative; margin:20px auto; } #wrapcharts li { height:10px; width:10px; diaplay:block; position:absolute; cursor:pointer; left:247px; top:2px; height:10px; width:10px; transition:0.2s; background:red; border-radius:50%; } #boxshadow { position:absolute; background:blue; opacity:0.2; height:0; width:0; left:0; top:0; } </style> </head> <body> <ul id="wrapcharts"></ul> <p id="boxshadow"></p> <script> /* **模拟从后端取值过来的【角度】和相对应的【人名】数组 **/ var degArr = [25,88,252,323,33,28,30,90,290,100,300,50,180,205,220,331,195,97,102,77,62,38,32,79]; var nameArr = ['内衣天使','小恶魔','金正恩','奥巴马','duolaA梦','午夜激情','梁静茹','刘亦菲','琪琪','大熊','小静','小屁孩','张三','李四','王五','麻六','小明','小张','丽丽','多多','瑾瑾','biubiu','Mr.boluo','Hanson']; /* **声明 getPos(param)函数: 利用三角函数定理根据传入的角度值获取对边和临边的x,y值 **/ function getPos(deg) { var X = Math.sin(deg*Math.PI/180)*250 + 245; var Y = -Math.cos(deg*Math.PI/180)*250 + 245; return {x:X,y:Y}; } /* **这里不用说吧,获取页面中的ul,和ul中的li对象,以及框选时的那个任意变动大小的小方块对象 **/ var oWrap = document.getElementById('wrapcharts'); var aLi = oWrap.getElementsByTagName('li'); var oBox =document.getElementById('boxshadow'); var allLi = ''; var posArr = []; /* **for循环中调用getPos(param)来获取degArr数组中的所有角度对应的x,y值(就是每个角度对应的x,y坐标),并传入到一个数组中保存,方便取用 **/ for(var i=0;i<degArr.length; i++) { posArr.push(getPos(degArr[i])); } /* **for循环根据度数数组degArr的长度插入li小圆点到ul中,并将之前获取的每个点对应的x,y左边插入到行内样式 **/ for(var i=0; i<degArr.length; i++) { allLi += '<li style="left:'+posArr[i].x+'px;top:'+posArr[i].y+'px;" title="'+degArr[i]+'°;姓名:'+nameArr[i]+'"></li>'; } oWrap.innerHTML = allLi; /* **遍历最终得到的ul中的li **/ for(var i=0; i<aLi.length; i++) { aLi[i].index = i; /* **封装鼠标移入每个小圆点时的放大事件,这里用到了matrix矩阵,为的事想兼容ie9以下浏览器,但是好像出了点问题 */ function focusOn(_this,color, size) { _this.style.background = color; _this.style.WebkitTransform = 'matrix('+size+', 0, 0, '+size+', 0, 0)'; _this.style.MozTransform = 'matrix('+size+', 0, 0, '+size+', 0, 0)'; _this.style.transform = 'matrix('+size+', 0, 0, '+size+', 0, 0)'; _this.style.filter="progid:DXImageTransform.Microsoft.Matrix( M11= "+size+", M12= 0, M21= 0 , M22="+size+",SizingMethod='auto expend')"; } aLi[i].onmouseover = function() { //alert(this.offsetLeft); _this = this; focusOn(_this,'blue', 2); } aLi[i].onmouseout = function() { //alert(this.offsetLeft); _this = this; focusOn(_this,'red', 1); } } /***框选***/ /* **拖拽框选代码区域,这个我就不解释了,明白人都一眼知道什么意思,这就像是公式, */ var allSelect = {}; document.onmousedown = function(ev) { var ev = ev || window.event; var disX = ev.clientX; var disY = ev.clientY; var H = W = clientleft = clienttop = clientright = clientbottom = 0; oBox.style.cssText = 'left:'+disX+'px;top:'+disY+'px;'; //console.log(disX+';'+disY); function again(f) { for(var i=0; i<posArr.length; i++) { if(posArr[i].x > clientleft && posArr[i].y > clienttop && (posArr[i].x + 10) < clientright && (posArr[i].y +10) < clientbottom) { //console.log(clientleft+';'+ clienttop +';'+ clientright +';' + clientbottom); if(f){allSelect[i] = i;}else{ aLi[i].style.background = 'blue'; } } else { aLi[i].style.background = 'red'; } } } document.onmousemove = function(ev) { var ev = ev || window.event; /* **当鼠标向四个方向拖拉的时候进行方向判断,并相应的改变小方块的left,top以及width,height **其实我这里有个问题,那就是,代码重复了一些,本想着合并一下,但是作者有点懒,嘿嘿,你们也可以尝试一下 **修改后你们拿去当做你们的发布,作者不会介意的 */ if(ev.clientX > disX && ev.clientY > disY) { W = ev.clientX - disX; H = ev.clientY - disY; oBox.style.width = W + 'px'; oBox.style.height = H + 'px'; clienttop = disY-oWrap.offsetTop; clientleft = disX-oWrap.offsetLeft; }else if(ev.clientX < disX && ev.clientY < disY) { W = disX - ev.clientX; H = disY - ev.clientY; oBox.style.top = ev.clientY + 'px'; oBox.style.left = ev.clientX + 'px'; oBox.style.width = W + 'px'; oBox.style.height = H + 'px'; clienttop = ev.clientY - oWrap.offsetTop; clientleft = ev.clientX - oWrap.offsetLeft; }else if(ev.clientX > disX && ev.clientY < disY) { W = ev.clientX - disX; H = disY - ev.clientY; oBox.style.top = ev.clientY + 'px'; oBox.style.width = W + 'px'; oBox.style.height = H + 'px'; clienttop = ev.clientY - oWrap.offsetTop; clientleft = disX - oWrap.offsetLeft; }else if(ev.clientX < disX && ev.clientY > disY) { W = disX - ev.clientX; H = ev.clientY - disY; oBox.style.left = ev.clientX + 'px'; oBox.style.width = W + 'px'; oBox.style.height = H + 'px'; clienttop = disY-oWrap.offsetTop; clientleft = ev.clientX - oWrap.offsetLeft; } clientright = clientleft+ W; clientbottom = clienttop + H; W = ''; H = ''; again(); } document.onmouseup = function() { again(1); document.onmouseup = document.onmousemove = null; oBox.style.cssText = 'height:0;width:0;'; if(JSON.stringify(allSelect) == '{}'){return;} console.log(allSelect); var lastSelect = []; for(var attr in allSelect){ lastSelect.push(nameArr[attr]); } allSelect = {}; console.log(lastSelect); alert('你选中的人是:\n\n'+lastSelect+'\n\n'); for(var i=0; i<aLi.length; i++) { aLi[i].style.background = 'red'; } } return false; } </script> </body> </html>
b = Math.sin(deg/180*Math.PI);c = -Math.sin(deg/180*Math.PI);d = Math. cos(deg/180*Math.PI);7. As for trigonometric functions, I won’t introduce them, Baidu has a lot of them. The above is the entire content of this article. I hope it will be helpful to everyone's study. For more related content, please pay attention to the PHP Chinese website! Related recommendations:
How to use JS and CSS3 to create cool pop-up window effects
javascript to achieve waterfall flow dynamics Loading images
jQuery implements the function of fixing the top display of the sliding page
##
The above is the detailed content of About making a simple frame chart using JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
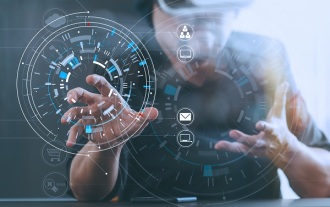
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
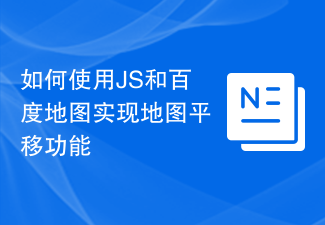
How to use JS and Baidu Map to implement map pan function Baidu Map is a widely used map service platform, which is often used in web development to display geographical information, positioning and other functions. This article will introduce how to use JS and Baidu Map API to implement the map pan function, and provide specific code examples. 1. Preparation Before using Baidu Map API, you first need to apply for a developer account on Baidu Map Open Platform (http://lbsyun.baidu.com/) and create an application. Creation completed
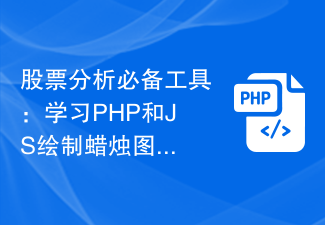
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
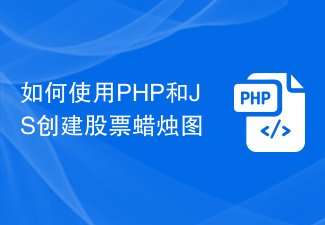
How to use PHP and JS to create a stock candle chart. A stock candle chart is a common technical analysis graphic in the stock market. It helps investors understand stocks more intuitively by drawing data such as the opening price, closing price, highest price and lowest price of the stock. price fluctuations. This article will teach you how to create stock candle charts using PHP and JS, with specific code examples. 1. Preparation Before starting, we need to prepare the following environment: 1. A server running PHP 2. A browser that supports HTML5 and Canvas 3
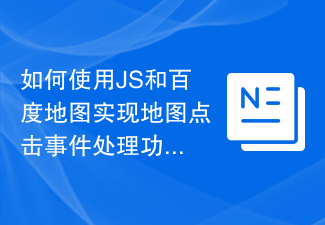
Overview of how to use JS and Baidu Maps to implement map click event processing: In web development, it is often necessary to use map functions to display geographical location and geographical information. Click event processing on the map is a commonly used and important part of the map function. This article will introduce how to use JS and Baidu Map API to implement the click event processing function of the map, and give specific code examples. Steps: Import the API file of Baidu Map. First, import the file of Baidu Map API in the HTML file. This can be achieved through the following code:
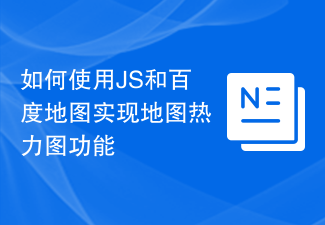
How to use JS and Baidu Maps to implement the map heat map function Introduction: With the rapid development of the Internet and mobile devices, maps have become a common application scenario. As a visual display method, heat maps can help us understand the distribution of data more intuitively. This article will introduce how to use JS and Baidu Map API to implement the map heat map function, and provide specific code examples. Preparation work: Before starting, you need to prepare the following items: a Baidu developer account, create an application, and obtain the corresponding AP
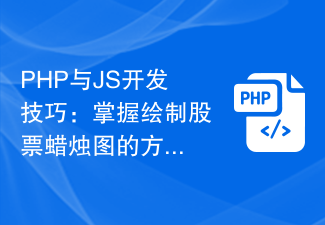
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
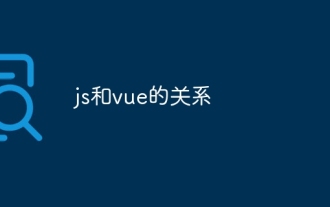
The relationship between js and vue: 1. JS as the cornerstone of Web development; 2. The rise of Vue.js as a front-end framework; 3. The complementary relationship between JS and Vue; 4. The practical application of JS and Vue.
