Detailed introduction to UMD specification in javascript (with code)
This article brings you a detailed introduction to the UMD specification in javascript (with code). It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
1. UMD specification
Address: https://github.com/umdjs/umd
UMD specification is the ugliest one among all specifications, there is no one! ! ! It appears to make the module compatible with both AMD and CommonJs specifications. It is mostly used by some third-party libraries that need to support both browser-side and server-side references. UMD is a product of an era. When various environments finally realize the unified specifications of ES harmony, it will also withdraw from the stage of history.
The structure of the UMD specification is very complicated at first glance, mainly because you need some basic knowledge of javascript to understand this paradigm. Its basic structure is as follows:
(function (root, factory) { if (typeof define === 'function' && define.amd) { // AMD define(['jquery', 'underscore'], factory); } else if (typeof exports === 'object') { // Node, CommonJS之类的 module.exports = factory(require('jquery'), require('underscore')); } else { // 浏览器全局变量(root 即 window) root.returnExports = factory(root.jQuery, root._); } }(this, function ($, _) { // 方法 function a(){}; // 私有方法,因为它没被返回 (见下面) function b(){}; // 公共方法,因为被返回了 function c(){}; // 公共方法,因为被返回了 // 暴露公共方法 return { b: b, c: c } }));
2. Source code Paradigm deduction
2.1 Basic structure
Let’s first look at the outermost structure:
(function (){}());
It is very simple, it is a self-executing function. Since it is a modular standard, it means that this self-executing function can eventually export a module. From a code perspective, there are actually two common implementation methods:
- return returns a module;
- The actual parameters are passed in an object, and the things that are generated within the function and need to be exported are hung on the properties of this object;
(function (factory){ //假设没有使用任何模块化方案,那么将工厂函数执行后返回的内容直接挂载到全局 window.Some_Attr = factory(); }(function(){ //自定义模块主体的内容 /* var a,b,c function a1(){} function b1(){} function c1(){} return { a:a1, b:b1 } */ }))
(function(root,factory){ root.Some_Attr = factory(); }(self !== undefined ? self : this, function(){ }));
/* * AMD规范的模块定义格式是define(id?, dependencies?, factory),factory就是实际的模块内容 */ (function (factory){ //判断全局环境是否支持AMD标准 if(typeof define === 'function' && define.amd){ //定义一个AMD模块 define([/*denpendencies*/],factory); } }(function(/*formal parameters*/){ //自定义模块主体的内容 /* var a,b,c function a1(){} function b1(){} function c1(){} return { a:a1, b:b1 } */ }))
CommonJs:
/* * CommonJs规范使用require('moduleName')的格式来引用模块,使用module.exports对象输出模块,所以只要把模块的输出内容挂载到module.exports上就完成了模块定义。 */ (function (factory){ //判断全局环境是否支持CommonJs标准 if(typeof exports === 'object' && typeof define !== 'function'){ module.exports = factory(/*require(moduleA), require(moduleB)*/); } }(function(/*formal parameters*/){ //自定义模块主体的内容 /* var a,b,c function a1(){} function b1(){} function c1(){} return { a:a1, b:b1 } */ }))
CommonJs## After the adaptation of #, the return content (usually an object) in the function body is mounted on module.exports
. If you have written node.js
code, for This will certainly not be unfamiliar. Crush the above fragments together, and you will understand what
looks like. 3. A more targeted UMD paradigm
UMD provides a more targeted paradigm on its github homepage, which is suitable for different scenarios. Interested readers can check it out by themselves ( The address is given in Section 1).
Here is a jqueryPlugin development paradigm that may be useful to most developers. If you understand the above analysis, the following code should not be difficult to understand:
// Uses CommonJS, AMD or browser globals to create a jQuery plugin. (function (factory) { if (typeof define === 'function' && define.amd) { // AMD. Register as an anonymous module. define(['jquery'], factory); } else if (typeof module === 'object' && module.exports) { // Node/CommonJS module.exports = function( root, jQuery ) { if ( jQuery === undefined ) { // require('jQuery') returns a factory that requires window to // build a jQuery instance, we normalize how we use modules // that require this pattern but the window provided is a noop // if it's defined (how jquery works) if ( typeof window !== 'undefined' ) { jQuery = require('jquery'); } else { jQuery = require('jquery')(root); } } factory(jQuery); return jQuery; }; } else { // Browser globals factory(jQuery); } }(function ($) { $.fn.jqueryPlugin = function () { return true; }; }));
4. Modular development
Front-end modularization itself is a slightly confusing topic. The author himself was initially confused about require() and require.js, but modularization is very important in front-end development. If you don’t want to just write code on one page for the rest of your life, you must pass this level. Interested readers can study in chunks according to the basic categories below.
The above is the detailed content of Detailed introduction to UMD specification in javascript (with code). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










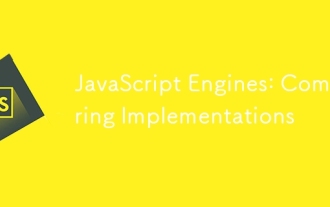
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
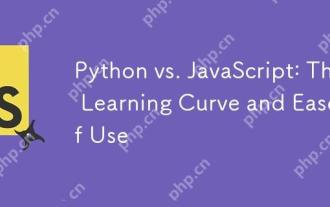
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
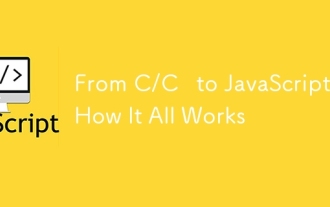
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
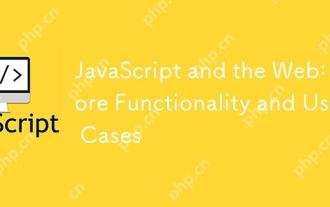
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
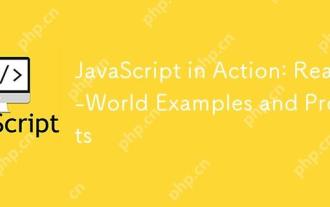
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
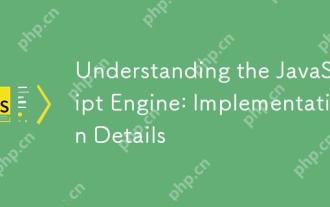
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.
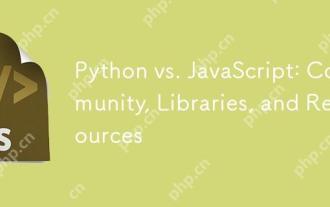
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
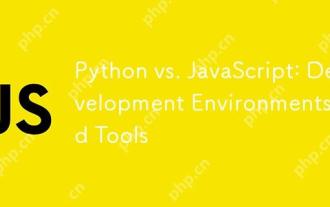
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
