13 super practical PHP functions, which ones do you know?
This article will introduce you to 13 super practical PHP functions. It has certain reference value. Friends in need can refer to it. I hope it will be helpful to everyone.
1. PHP encryption and decryption
PHP encryption and decryption functions can be used to encrypt some useful strings and store them in the database , and by reversibly decrypting the string, this function uses base64 and MD5 encryption and decryption.
function encryptDecrypt($key, $string, $decrypt){ if($decrypt){ $decrypted = rtrim(mcrypt_decrypt(MCRYPT_RIJNDAEL_256, md5($key), base64_decode($string), MCRYPT_MODE_CBC, md5(md5($key))), "12"); return $decrypted; }else{ $encrypted = base64_encode(mcrypt_encrypt(MCRYPT_RIJNDAEL_256, md5($key), $string, MCRYPT_MODE_CBC, md5(md5($key)))); return $encrypted; } }
The usage method is as follows:
//以下是将字符串“Helloweba欢迎您”分别加密和解密 //加密: echo encryptDecrypt('password', 'jb51欢迎您',0); //解密: echo encryptDecrypt('password', 'z0JAx4qMwcF+db5TNbp/xwdUM84snRsXvvpXuaCa4Bk=',1);
2. PHP generates a random string
When we need to generate a random name, temporarily The following function can be used when entering strings such as passwords:
function generateRandomString($length = 10) { $characters = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'; $randomString = ''; for ($i = 0; $i < $length; $i++) { $randomString .= $characters[rand(0, strlen($characters) - 1)]; } return $randomString; }
The usage method is as follows:
echo generateRandomString(20);
3. PHP gets the file extension (suffix)
The following function can quickly obtain the file extension or suffix.
function getExtension($filename){ $myext = substr($filename, strrpos($filename, '.')); return str_replace('.','',$myext); }
The usage method is as follows:
$filename = '我的文档.doc'; echo getExtension($filename);
4. PHP gets the file size and formats
below The function used can get the size of the file and convert it into easy-to-read KB, MB and other formats.
function formatSize($size) { $sizes = array(" Bytes", " KB", " MB", " GB", " TB", " PB", " EB", " ZB", " YB"); if ($size == 0) { return('n/a'); } else { return (round($size/pow(1024, ($i = floor(log($size, 1024)))), 2) . $sizes[$i]); } }
is used as follows:
$thefile = filesize('test_file.mp3'); echo formatSize($thefile);
5. PHP replaces tag characters
Sometimes we need to To replace strings and template tags with specified content, you can use the following function:
function stringParser($string,$replacer){ $result = str_replace(array_keys($replacer), array_values($replacer),$string); return $result; }
The usage method is as follows:
$string = 'The {b}anchor text{/b} is the {b}actual word{/b} or words used {br}to describe the link {br}itself'; $replace_array = array('{b}' => '<b>','{/b}' => '</b>','{br}' => '<br />'); echo stringParser($string,$replace_array);
6. PHP lists the directory The file name is
If you want to list all the files in the directory, use the following code:
function listDirFiles($DirPath){ if($dir = opendir($DirPath)){ while(($file = readdir($dir))!== false){ if(!is_dir($DirPath.$file)) { echo "filename: $file<br />"; } } } }
The usage is as follows:
listDirFiles('home/some_folder/');
7. PHP gets the current page URL
The following function can get the URL of the current page, whether it is http or https.
function curPageURL() { $pageURL = 'http'; if (!empty($_SERVER['HTTPS'])) {$pageURL .= "s";} $pageURL .= "://"; if ($_SERVER["SERVER_PORT"] != "80") { $pageURL .= $_SERVER["SERVER_NAME"].":".$_SERVER["SERVER_PORT"].$_SERVER["REQUEST_URI"]; } else { $pageURL .= $_SERVER["SERVER_NAME"].$_SERVER["REQUEST_URI"]; } return $pageURL; }
The usage method is as follows:
echo curPageURL();
8. PHP force downloads files
Sometimes we don’t want to If the browser directly opens a file, such as a PDF file, but wants to download the file directly, the following function can force the file to be downloaded. The application/octet-stream header type is used in the function.
function download($filename){ if ((isset($filename))&&(file_exists($filename))){ header("Content-length: ".filesize($filename)); header('Content-Type: application/octet-stream'); header('Content-Disposition: attachment; filename="' . $filename . '"'); readfile("$filename"); } else { echo "Looks like file does not exist!"; } }
The usage method is as follows:
download('/down/test_45f73e852.zip');
9. PHP intercepts the string length
We often When you encounter a situation where you need to intercept the length of a string (including Chinese characters), for example, the title cannot display more than a few characters, and the excess length is represented by..., the following function can meet your needs.
/* Utf-8、gb2312都支持的汉字截取函数 cut_str(字符串, 截取长度, 开始长度, 编码); 编码默认为 utf-8 开始长度默认为 0 */ function cutStr($string, $sublen, $start = 0, $code = 'UTF-8'){ if($code == 'UTF-8'){ $pa = "/[\x01-\x7f]|[\xc2-\xdf][\x80-\xbf]|\xe0[\xa0-\xbf][\x80-\xbf]|[\xe1-\xef][\x80-\xbf][\x80-\xbf]|\xf0[\x90-\xbf][\x80-\xbf][\x80-\xbf]|[\xf1-\xf7][\x80-\xbf][\x80-\xbf][\x80-\xbf]/"; preg_match_all($pa, $string, $t_string); if(count($t_string[0]) - $start > $sublen) return join('', array_slice($t_string[0], $start, $sublen))."..."; return join('', array_slice($t_string[0], $start, $sublen)); }else{ $start = $start*2; $sublen = $sublen*2; $strlen = strlen($string); $tmpstr = ''; for($i=0; $i<$strlen; $i++){ if($i>=$start && $i<($start+$sublen)){ if(ord(substr($string, $i, 1))>129){ $tmpstr.= substr($string, $i, 2); }else{ $tmpstr.= substr($string, $i, 1); } } if(ord(substr($string, $i, 1))>129) $i++; } if(strlen($tmpstr)<$strlen ) $tmpstr.= "..."; return $tmpstr; } }
The usage method is as follows:
$str = "jQuery插件实现的加载图片和页面效果"; echo cutStr($str,16);
10. PHP gets the real IP of the client
We often To record the user's IP in the database, the following code can obtain the real IP of the client:
//获取用户真实IP function getIp() { if (getenv("HTTP_CLIENT_IP") && strcasecmp(getenv("HTTP_CLIENT_IP"), "unknown")) $ip = getenv("HTTP_CLIENT_IP"); else if (getenv("HTTP_X_FORWARDED_FOR") && strcasecmp(getenv("HTTP_X_FORWARDED_FOR"), "unknown")) $ip = getenv("HTTP_X_FORWARDED_FOR"); else if (getenv("REMOTE_ADDR") && strcasecmp(getenv("REMOTE_ADDR"), "unknown")) $ip = getenv("REMOTE_ADDR"); else if (isset ($_SERVER['REMOTE_ADDR']) && $_SERVER['REMOTE_ADDR'] && strcasecmp($_SERVER['REMOTE_ADDR'], "unknown")) $ip = $_SERVER['REMOTE_ADDR']; else $ip = "unknown"; return ($ip); }
The usage is as follows:
echo getIp();
11 , PHP prevents SQL injection
When we query the database, for security reasons, we need to filter some illegal characters to prevent malicious SQL injection. Please take a look at the function:
function injCheck($sql_str) { $check = preg_match('/select|insert|update|delete|\'|\/\*|\*|\.\.\/|\.\/|union|into|load_file|outfile/', $sql_str); if ($check) { echo '非法字符!!'; exit; } else { return $sql_str; } }
Use The method is as follows:
echo injCheck('1 or 1=1');
12. PHP page prompts and jumps
When we perform form operations, sometimes we need prompts for the sake of friendliness The user operates the results and jumps to the relevant page. Please see the following function:
function message($msgTitle,$message,$jumpUrl){ $str = '<!DOCTYPE HTML>'; $str .= '<html>'; $str .= '<head>'; $str .= '<meta charset="utf-8">'; $str .= '<title>页面提示</title>'; $str .= '<style type="text/css">'; $str .= '*{margin:0; padding:0}a{color:#369; text-decoration:none;}a:hover{text-decoration:underline}body{height:100%; font:12px/18px Tahoma, Arial, sans-serif; color:#424242; background:#fff}.message{width:450px; height:120px; margin:16% auto; border:1px solid #99b1c4; background:#ecf7fb}.message h3{height:28px; line-height:28px; background:#2c91c6; text-align:center; color:#fff; font-size:14px}.msg_txt{padding:10px; margin-top:8px}.msg_txt h4{line-height:26px; font-size:14px}.msg_txt h4.red{color:#f30}.msg_txt p{line-height:22px}'; $str .= '</style>'; $str .= '</head>'; $str .= '<body>'; $str .= '<div>'; $str .= '<h3>'.$msgTitle.'</h3>'; $str .= '<div>'; $str .= '<h4>'.$message.'</h4>'; $str .= '<p>系统将在 <span style="color:blue;font-weight:bold">3</span> 秒后自动跳转,如果不想等待,直接点击 <a href="{$jumpUrl}">这里</a> 跳转</p>'; $str .= "<script>setTimeout('location.replace(\'".$jumpUrl."\')',2000)</script>"; $str .= '</div>'; $str .= '</div>'; $str .= '</body>'; $str .= '</html>'; echo $str; }
The usage method is as follows:
message('操作提示','操作成功!','http://www.php.cn');
13. PHP calculation time
When we process time, we need to calculate the length of time from the current time to a certain point in time. For example, calculating the running time of the client is usually represented by hh:mm:ss.
function changeTimeType($seconds) { if ($seconds > 3600) { $hours = intval($seconds / 3600); $minutes = $seconds % 3600; $time = $hours . ":" . gmstrftime('%M:%S', $minutes); } else { $time = gmstrftime('%H:%M:%S', $seconds); } return $time; }
The usage method is as follows:
$seconds = 3712; echo changeTimeType($seconds);
Recommended learning: "PHP Video Tutorial"

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
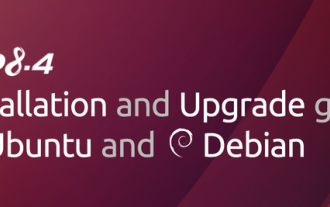
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
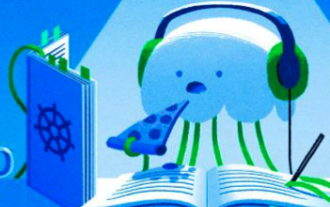
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
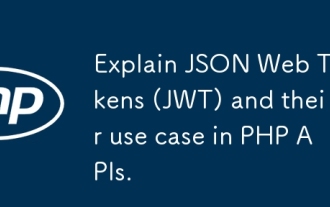
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
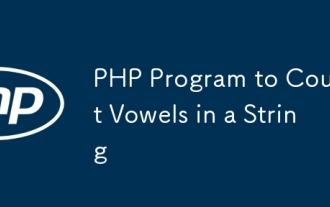
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
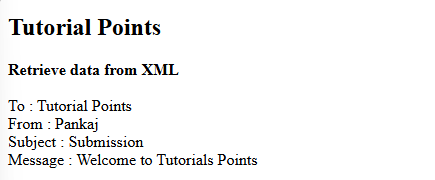
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
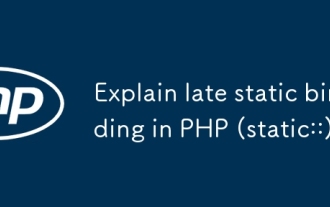
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
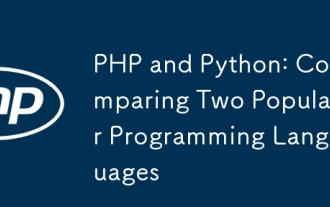
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
