Remove last item from array using JavaScript (3 ways)
In the previous article "JavaScript restricts the input box to only allow integers and decimal points (two methods)", I introduced how to use javascript to restrict the input box to only allow integers and decimal points. Interested Friends can learn about it~
What this article will explain is to teach you how to use JavaScript to delete the last item from an array.
Below I will explain how to use JavaScript to delete the last item from an array through 3 examples:
First sample code:
Note: This example uses the splice() method to remove the last item from the array.
<!DOCTYPE HTML> <html> <head> <meta charset="UTF-8"> <title></title> </head> <body style="text-align:center;" id="body"> <h1 style="color:red;"> PHP中文网 </h1> <p id="GFG_UP" style="font-size: 16px;"> </p> <button onclick="gfg_Run()"> 删除最后一项 </button> <p id="GFG_DOWN" style="color:red; font-size: 20px; font-weight: bold;"> </p> <script> var el_up = document.getElementById("GFG_UP"); var el_down = document.getElementById("GFG_DOWN"); var array = [34, 24, 31, 48]; el_up.innerHTML = "Array = [" + array + "]"; function gfg_Run() { array.splice(-1, 1); el_down.innerHTML = "删除后的数组 = [" + array + "]"; } </script> </body> </html>
The running results are as follows:
JavaScript Array splice() method adds/removes items to/from the array and returns The item that was removed; this method mutates the original array.
Second sample code:
Note: This example uses the pop() method to remove the last item from the array.
<!DOCTYPE HTML> <html> <head> <meta charset="UTF-8"> <title></title> </head> <body style="text-align:center;" id="body"> <h1 style="color:orange;"> PHP中文网 </h1> <p id="GFG_UP" style="font-size: 16px;"> </p> <button onclick="gfg_Run()"> 删除最后一项 </button> <p id="GFG_DOWN" style="color:orange; font-size: 20px; font-weight: bold;"> </p> <script> var el_up = document.getElementById("GFG_UP"); var el_down = document.getElementById("GFG_DOWN"); var array = [34, 24, 31, 48]; el_up.innerHTML = "Array = [" + array + "]"; function gfg_Run() { array.pop(); el_down.innerHTML = "删除后的数组 = [" + array + "]"; } </script> </body> </html>
The running results are as follows:
The JavaScript Array pop() method will delete the arrayObject , decrements the array length by 1 and returns the value of the element it removed. If the array is already empty, pop() does not modify the array and returns an undefined value.
Third code example:
Note: This example does not remove the last item from the array, instead using The slice() method returns a new array with the items removed.
<!DOCTYPE HTML> <html> <head> <meta charset="UTF-8"> <title></title> </head> <body style="text-align:center;" id="body"> <h1 style="color:pink;"> PHP中文网 </h1> <p id="GFG_UP" style="font-size: 16px;"> </p> <button onclick="gfg_Run()"> 删除最后一项 </button> <p id="GFG_DOWN" style="color:pink; font-size: 20px; font-weight: bold;"> </p> <script> var el_up = document.getElementById("GFG_UP"); var el_down = document.getElementById("GFG_DOWN"); var array = [34, 24, 31, 48]; el_up.innerHTML = "Array = [" + array + "]"; function gfg_Run() { el_down.innerHTML = "删除后的数组 = [" + array.slice(0, -1) + "]"; } </script> </body> </html>
The running results are as follows:
The JavaScript Array slice() method can return selected elements from an existing array , the slice() method can extract a certain part of the string and return the extracted part as a new string; the slice() method does not change the original array.
Finally, I would like to recommend "JavaScript Basics Tutorial" ~ Welcome everyone to learn ~
The above is the detailed content of Remove last item from array using JavaScript (3 ways). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
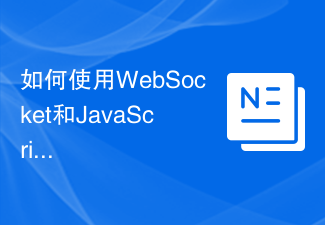
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
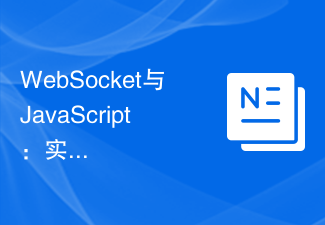
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
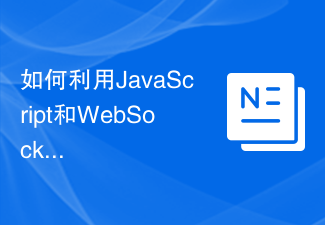
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
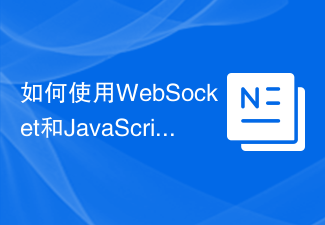
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
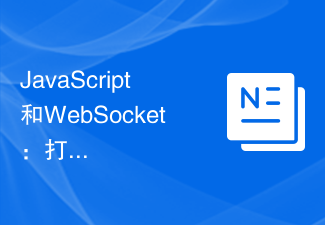
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
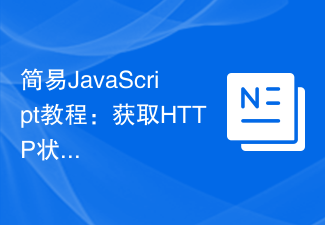
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
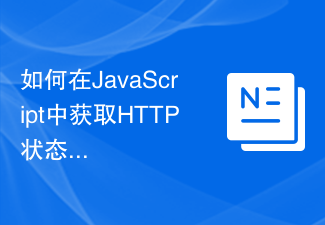
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
