Python GUI 시계에 사용자 제어 카메라 기능 추가
이전 튜토리얼에서는 Python과 Tkinter를 사용하여 사용자 정의 가능한 GUI 시계를 구축했습니다. 사용자가 필요에 따라 이미지를 캡처하고 저장할 수 있는 카메라 기능을 추가하여 한 단계 더 발전시켜 보겠습니다. 이 프로젝트에서는 Python에서 카메라 입력 작업을 소개하여 GUI 개발 및 파일 처리 기술을 향상시킵니다.
환경 설정
시작하기 전에 필요한 라이브러리가 설치되어 있는지 확인하세요. 우리는 카메라 처리를 위해 OpenCV를 사용할 것입니다. pip를 사용하여 설치하세요.
pip install opencv-python
다음으로 pip를 사용하여 Pillow를 설치하겠습니다.
pip install Pillow
이제 모든 종속성을 설치했으므로 카메라를 추가할 수 있습니다. 우리는 두 종류의 카메라, 즉 일반 카메라와 클릭 뒤에 숨겨진 카메라를 만들어 보겠습니다.
나와 함께 있어주세요.
import cv2 from PIL import Image, ImageTk import os from datetime import datetime
카메라 기능 만들기
카메라 캡처를 처리하는 기능을 추가해 보겠습니다.
def capture_image(): # Initialize the camera cap = cv2.VideoCapture(0) if not cap.isOpened(): print("Error: Could not open camera.") return # Capture a frame ret, frame = cap.read() if not ret: print("Error: Could not capture image.") cap.release() return # Create a directory to store images if it doesn't exist if not os.path.exists("captured_images"): os.makedirs("captured_images") # Generate a unique filename using timestamp timestamp = datetime.now().strftime("%Y%m%d_%H%M%S") filename = f"captured_images/image_{timestamp}.png" # Save the image cv2.imwrite(filename, frame) print(f"Image saved as {filename}") # Release the camera cap.release() # Display the captured image display_image(filename)
초보자가 이해하기 쉽도록 Capture_image() 함수를 분해해 보겠습니다. 각 부분을 단계별로 살펴보고 현재 상황과 이유를 설명하겠습니다.
`def capture_image()`
이 줄은 Capture_image()라는 새로운 함수를 생성합니다. 기능은 사진을 찍고 싶을 때마다 사용할 수 있는 일련의 지침이라고 생각하세요.
`cap = cv2.VideoCapture(0)`
여기서 카메라를 설정하고 있습니다. 디지털 카메라를 켜고 있다고 상상해 보세요.
- cv2는 Python에서 카메라와 이미지 작업을 도와주는 도구(라이브러리)입니다.
- VideoCapture(0)은 카메라의 전원 버튼을 누르는 것과 같습니다. 0은 "찾은 첫 번째 카메라 사용"(일반적으로 노트북에 내장된 웹캠)을 의미합니다.
- 이것을 카메라 설정 캡(capture의 줄임말)이라고 부르기 때문에 나중에 참고할 수 있습니다.
if not cap.isOpened(): print("Error: Could not open camera.") return
카메라가 제대로 켜졌는지 확인하는 부분입니다.
- cap.isOpened()가 아닌 경우: "카메라가 켜지지 않았나요?"라고 묻습니다.
- 실패한 경우 오류 메시지가 인쇄됩니다.
-
return은 문제가 있을 경우 "여기서 멈추고 기능을 종료한다"는 뜻입니다.
ret, 프레임 = cap.read()
이제 실제 사진을 찍고 있습니다.
cap.read()는 카메라의 셔터 버튼을 누르는 것과 같습니다.
두 가지를 제공합니다.
- ret: "사진이 잘 찍혔나요?"에 대한 예/아니요 대답
- 프레임: 실제 사진입니다(촬영된 경우).
if not ret: print("Error: Could not capture image.") cap.release() return
사진이 성공적으로 촬영되었는지 확인합니다.
- ret이 "no"인 경우(사진이 실패했음을 의미), 우리는 다음을 수행합니다.
오류 메시지를 인쇄하세요.
cap.release()는 카메라를 끕니다.
return은 기능을 종료합니다.
if not os.path.exists("captured_images"): os.makedirs("captured_images")
이 부분에서는 사진을 저장할 특수 폴더를 만듭니다.
if not os.path.exists("captured_images"):` checks if a folder named "captured_images" already exists.
- 존재하지 않는 경우 os.makedirs("captured_images")가 이 폴더를 생성합니다.
- 사진을 저장하기 위해 새 앨범을 만드는 것과 같습니다.
timestamp = datetime.now().strftime("%Y%m%d_%H%M%S") filename = f"captured_images/image_{timestamp}.png"
여기서 사진에 대한 고유한 이름을 만듭니다.
datetime.now()는 현재 날짜와 시간을 가져옵니다.
.strftime("%Y%m%d_%H%M%S")는 이 시간을 "20240628_152059"(연-월-일_HourMinuteSecond)와 같은 문자열로 형식화합니다.
- 이를 사용하여 "captured_images/image_20240628_152059.png"와 같은 파일 이름을 만듭니다.
- 이렇게 하면 각 사진에 촬영 시기를 기준으로 고유한 이름이 부여됩니다.
cv2.imwrite(filename, frame) print(f"Image saved as {filename}")
이제 사진을 저장합니다.
v2.imwrite(파일 이름, 프레임)는 우리가 만든 파일 이름으로 사진(프레임)을 저장합니다.
그런 다음 이미지가 저장된 위치를 알려주는 메시지를 인쇄합니다.
`cap.release()`
이 줄은 작업이 끝나면 전원 버튼을 다시 누르는 것처럼 카메라를 끕니다.
`display_image(filename)`
마지막으로 방금 찍은 사진을 화면에 표시하기 위해 또 다른 함수를 호출합니다.
요약하면 이 함수는 다음을 수행합니다.
- 카메라를 켭니다
- 카메라가 작동하는지 확인
- 사진을 찍습니다
- 사진이 성공적으로 촬영되었는지 확인
- 사진이 없으면 사진을 저장할 폴더를 만듭니다
- 현재 시간을 기준으로 사진에 고유한 이름을 지정합니다
- 폴더에 사진을 저장합니다
- 카메라를 끕니다
각 단계에는 제대로 작동하는지 확인하는 검사가 있으며, 어느 시점에든 문제가 있으면 기능이 중지되고 무엇이 잘못되었는지 알려줍니다.
캡처된 이미지 표시
캡처된 이미지를 표시하는 기능 추가:
def display_image(filename): # Open the image file img = Image.open(filename) # Resize the image to fit in the window img = img.resize((300, 200), Image.LANCZOS) # Convert the image for Tkinter photo = ImageTk.PhotoImage(img) # Update the image label image_label.config(image=photo) image_label.image = photo
초보자 친화적인 파일 작업 설명부터 시작해 코드를 자세히 살펴보겠습니다.
초보자를 위한 파일 작업:
-
읽기:
- 책을 펼쳐 내용을 보는 것과 같습니다.
- 프로그래밍에서 파일을 읽는다는 것은 파일을 변경하지 않고 해당 콘텐츠에 액세스하는 것을 의미합니다.
- 예: 이미지를 열어서 봅니다.
-
쓰기:
- This is like writing in a notebook.
- In programming, writing means adding new content to a file or changing existing content.
- Example: Saving a new image or modifying an existing one.
-
Execute:
- This is like following a set of instructions.
- In programming, executing usually refers to running a program or script.
- For images, we don't typically "execute" them, but we can process or display them.
Now, let's focus on the display_image(filename) function:
`def display_image(filename)`
This line defines a function named display_image that takes a filename as input. This filename is the path to the image we want to display.
`img = Image.open(filename)`
Here's where we use the "read" operation:
- Image.open() is a function from the PIL (Python Imaging Library) that opens an image file.
- It's like telling the computer, "Please look at this picture for me."
- The opened image is stored in the variable img.
-
This operation doesn't change the original file; it allows us to work with its contents.
img = img.resize((300, 200), Image.LANCZOS)
This line resizes the image:
- img.resize() changes the size of the image.
- (300, 200) sets the new width to 300 pixels and height to 200 pixels.
-
Image.LANCZOS is a high-quality resizing method that helps maintain image quality.
photo = ImageTk.PhotoImage(img)
This line converts the image for use with Tkinter (the GUI library we're using):
- ImageTk.PhotoImage() takes our resized image and converts it into a format that Tkinter can display.
- This converted image is stored in the photo variable.
image_label.config(image=photo) image_label.image = photo
These lines update the GUI to show the image:
- image_label is a Tkinter widget (like a container) that can display images.
- config(image=photo) tells this label to display our processed image.
- image_label.image = photo is a special line that prevents the image from being deleted by Python's garbage collector.
In summary, this function does the following:
- Opens an image file (read operation).
- Resize the image to fit nicely in our GUI window.
- Converts the image to a format our GUI system (Tkinter) can understand.
- Updates a label in our GUI to display this image.
This process doesn't involve writing to the file or executing it. We're simply reading the image, processing it in memory, and displaying it in our application.
Adding GUI Elements
Update your existing GUI to include a button for image capture and a label to display the image:
# Add this after your existing GUI elements capture_button = tk.Button(window, text="Capture Image", command=capture_image) capture_button.pack(anchor='center', pady=5) image_label = tk.Label(window) image_label.pack(anchor='center', pady=10)
- Adjusting the Window Size:
You may need to adjust the window size to accommodate the new elements:
window.geometry("350x400") # Increase the height
- Complete Code:
Here's the complete code incorporating the new camera feature:
import tkinter as tk from time import strftime import cv2 from PIL import Image, ImageTk import os from datetime import datetime window = tk.Tk() window.title("Python GUI Clock with Camera") window.geometry("350x400") is_24_hour = True def update_time(): global is_24_hour time_format = '%H:%M:%S' if is_24_hour else '%I:%M:%S %p' time_string = strftime(time_format) date_string = strftime('%B %d, %Y') time_label.config(text=time_string) date_label.config(text=date_string) time_label.after(1000, update_time) def change_color(): colors = ['black', 'red', 'green', 'blue', 'yellow', 'purple', 'orange'] current_bg = time_label.cget("background") next_color = colors[(colors.index(current_bg) + 1) % len(colors)] time_label.config(background=next_color) date_label.config(background=next_color) def toggle_format(): global is_24_hour is_24_hour = not is_24_hour def capture_image(): cap = cv2.VideoCapture(0) if not cap.isOpened(): print("Error: Could not open camera.") return ret, frame = cap.read() if not ret: print("Error: Could not capture image.") cap.release() return if not os.path.exists("captured_images"): os.makedirs("captured_images") timestamp = datetime.now().strftime("%Y%m%d_%H%M%S") filename = f"captured_images/image_{timestamp}.png" cv2.imwrite(filename, frame) print(f"Image saved as {filename}") cap.release() display_image(filename) def display_image(filename): img = Image.open(filename) img = img.resize((300, 200), Image.LANCZOS) photo = ImageTk.PhotoImage(img) image_label.config(image=photo) image_label.image = photo time_label = tk.Label(window, font=('calibri', 40, 'bold'), background='black', foreground='white') time_label.pack(anchor='center') date_label = tk.Label(window, font=('calibri', 24), background='black', foreground='white') date_label.pack(anchor='center') color_button = tk.Button(window, text="Change Color", command=change_color) color_button.pack(anchor='center', pady=5) format_button = tk.Button(window, text="Toggle 12/24 Hour", command=toggle_format) format_button.pack(anchor='center', pady=5) capture_button = tk.Button(window, text="Capture Image", command=capture_image) capture_button.pack(anchor='center', pady=5) image_label = tk.Label(window) image_label.pack(anchor='center', pady=10) update_time() window.mainloop()
Conclusion
You've now enhanced your GUI clock with a user-controlled camera feature. This addition demonstrates how to integrate hardware interactions into a Python GUI application, handle file operations, and dynamically update the interface.
Always respect user privacy and obtain the necessary permissions when working with camera features in your applications.
Resource
- How to Identify a Phishing Email in 2024
- Build Your First Password Cracker
- Python for Beginners
위 내용은 Python GUI 시계에 사용자 제어 카메라 기능 추가의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
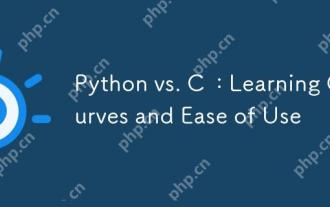
Python은 배우고 사용하기 쉽고 C는 더 강력하지만 복잡합니다. 1. Python Syntax는 간결하며 초보자에게 적합합니다. 동적 타이핑 및 자동 메모리 관리를 사용하면 사용하기 쉽지만 런타임 오류가 발생할 수 있습니다. 2.C는 고성능 응용 프로그램에 적합한 저수준 제어 및 고급 기능을 제공하지만 학습 임계 값이 높고 수동 메모리 및 유형 안전 관리가 필요합니다.
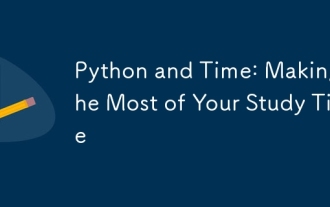
제한된 시간에 Python 학습 효율을 극대화하려면 Python의 DateTime, Time 및 Schedule 모듈을 사용할 수 있습니다. 1. DateTime 모듈은 학습 시간을 기록하고 계획하는 데 사용됩니다. 2. 시간 모듈은 학습과 휴식 시간을 설정하는 데 도움이됩니다. 3. 일정 모듈은 주간 학습 작업을 자동으로 배열합니다.
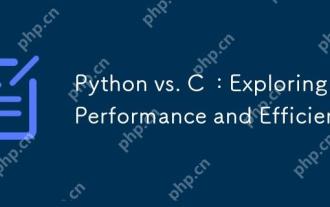
Python은 개발 효율에서 C보다 낫지 만 C는 실행 성능이 높습니다. 1. Python의 간결한 구문 및 풍부한 라이브러리는 개발 효율성을 향상시킵니다. 2.C의 컴파일 유형 특성 및 하드웨어 제어는 실행 성능을 향상시킵니다. 선택할 때는 프로젝트 요구에 따라 개발 속도 및 실행 효율성을 평가해야합니다.
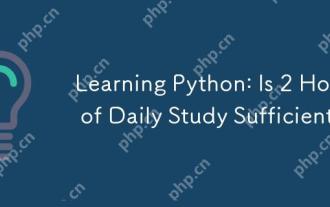
하루에 2 시간 동안 파이썬을 배우는 것으로 충분합니까? 목표와 학습 방법에 따라 다릅니다. 1) 명확한 학습 계획을 개발, 2) 적절한 학습 자원 및 방법을 선택하고 3) 실습 연습 및 검토 및 통합 연습 및 검토 및 통합,이 기간 동안 Python의 기본 지식과 고급 기능을 점차적으로 마스터 할 수 있습니다.
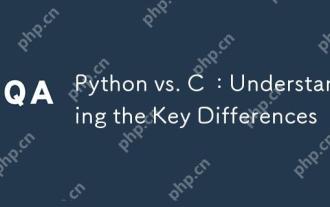
Python과 C는 각각 고유 한 장점이 있으며 선택은 프로젝트 요구 사항을 기반으로해야합니다. 1) Python은 간결한 구문 및 동적 타이핑으로 인해 빠른 개발 및 데이터 처리에 적합합니다. 2) C는 정적 타이핑 및 수동 메모리 관리로 인해 고성능 및 시스템 프로그래밍에 적합합니다.
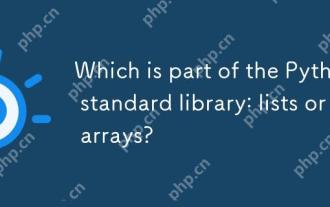
Pythonlistsarepartoftsandardlardlibrary, whileraysarenot.listsarebuilt-in, 다재다능하고, 수집 할 수있는 반면, arraysarreprovidedByTearRaymoduledlesscommonlyusedDuetolimitedFunctionality.
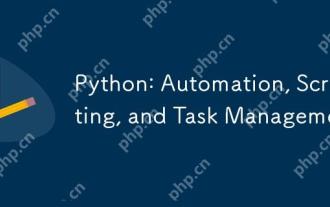
파이썬은 자동화, 스크립팅 및 작업 관리가 탁월합니다. 1) 자동화 : 파일 백업은 OS 및 Shutil과 같은 표준 라이브러리를 통해 실현됩니다. 2) 스크립트 쓰기 : PSUTIL 라이브러리를 사용하여 시스템 리소스를 모니터링합니다. 3) 작업 관리 : 일정 라이브러리를 사용하여 작업을 예약하십시오. Python의 사용 편의성과 풍부한 라이브러리 지원으로 인해 이러한 영역에서 선호하는 도구가됩니다.
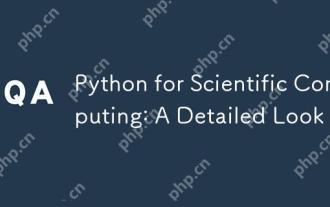
과학 컴퓨팅에서 Python의 응용 프로그램에는 데이터 분석, 머신 러닝, 수치 시뮬레이션 및 시각화가 포함됩니다. 1.numpy는 효율적인 다차원 배열 및 수학적 함수를 제공합니다. 2. Scipy는 Numpy 기능을 확장하고 최적화 및 선형 대수 도구를 제공합니다. 3. 팬더는 데이터 처리 및 분석에 사용됩니다. 4. matplotlib는 다양한 그래프와 시각적 결과를 생성하는 데 사용됩니다.
