얼굴 인증으로 안전한 직원 대시보드 구축: 종합적인 Next.js 튜토리얼
직장 관리를 혁신할 준비가 되셨나요? 이 포괄적인 튜토리얼에서는 얼굴 인증을 활용하는 최첨단 직원 대시보드를 만드는 방법을 자세히 살펴보겠습니다. 우리는 웹 개발에서 가장 인기 있는 도구 중 일부인 Next.js, FACEIO 및 Shadcn UI를 사용할 것입니다. 이 가이드를 마치면 직원들이 미래에 살고 있는 듯한 느낌을 줄 수 있는 세련되고 안전한 대시보드를 갖게 될 것입니다!
시작하기 전에 필요한 것
자세히 살펴보기 전에 모든 오리를 연속해서 확보했는지 확인하세요.
- 컴퓨터에 Node.js가 설치되어 있습니다
- npm 또는 Yarn(배를 띄우는 것 중 하나)
다 알아냈나요? 엄청난! 이동 중에도 이 쇼를 감상해 보세요.
프로젝트 설정: 첫 번째 단계
1단계: Next.js 프로젝트 시작하기
먼저 Next.js 프로젝트를 만들어 보겠습니다. 터미널을 열고 다음 마법의 단어를 입력하세요:
npx create-next-app@latest faceio-app cd faceio-app
몇 가지 질문을 받게 됩니다. 답변하는 방법은 다음과 같습니다.
- 타입스크립트? 그렇죠!
- ESLint? 물론이죠!
- 테일윈드 CSS? 물론이죠!
- src/디렉토리? 아냐, 우린 괜찮아.
- 앱 라우터? 네, 부탁드립니다!
- 기본 가져오기 별칭을 맞춤설정하시겠습니까? 이건 패스하겠습니다.
2단계: 도구 수집
이제 필요한 물품을 모두 챙기세요. 종속 항목을 설치하려면 다음 명령을 실행하세요.
npm install @faceio/fiojs @shadcn/ui class-variance-authority clsx tailwind-merge
3단계: 비밀 소스 설정
프로젝트 루트에 .env.local이라는 파일을 만듭니다. 여기에 비밀 FACEIO 앱 ID를 보관할 것입니다:
NEXT_PUBLIC_FACEIO_APP_ID=your-super-secret-faceio-app-id
'your-super-secret-faceio-app-id'를 실제 FACEIO 애플리케이션 ID로 바꾸는 것을 잊지 마세요. 안전하게 보관하세요!
4단계: 파일 구조
프로젝트 구조는 다음과 같아야 합니다.
faceio-app/ ├── app/ │ ├── layout.tsx │ ├── page.tsx │ └── components/ │ ├── FaceAuth.tsx │ └── EmployeeDashboard.tsx ├── public/ ├── .env.local ├── next.config.js ├── package.json ├── tsconfig.json └── tailwind.config.js
5단계: Tailwind CSS 다듬기
Tailwind를 새롭게 단장할 시간입니다. 다음과 같은 멋진 구성으로 tailwind.config.js 파일을 업데이트하세요.
/** @type {import('tailwindcss').Config} */ module.exports = { darkMode: ["class"], content: [ './app/**/*.{ts,tsx}', ], theme: { container: { center: true, padding: "2rem", screens: { "2xl": "1400px", }, }, extend: { colors: { border: "hsl(var(--border))", input: "hsl(var(--input))", ring: "hsl(var(--ring))", background: "hsl(var(--background))", foreground: "hsl(var(--foreground))", primary: { DEFAULT: "hsl(var(--primary))", foreground: "hsl(var(--primary-foreground))", }, secondary: { DEFAULT: "hsl(var(--secondary))", foreground: "hsl(var(--secondary-foreground))", }, destructive: { DEFAULT: "hsl(var(--destructive))", foreground: "hsl(var(--destructive-foreground))", }, muted: { DEFAULT: "hsl(var(--muted))", foreground: "hsl(var(--muted-foreground))", }, accent: { DEFAULT: "hsl(var(--accent))", foreground: "hsl(var(--accent-foreground))", }, popover: { DEFAULT: "hsl(var(--popover))", foreground: "hsl(var(--popover-foreground))", }, card: { DEFAULT: "hsl(var(--card))", foreground: "hsl(var(--card-foreground))", }, }, borderRadius: { lg: "var(--radius)", md: "calc(var(--radius) - 2px)", sm: "calc(var(--radius) - 4px)", }, keyframes: { "accordion-down": { from: { height: 0 }, to: { height: "var(--radix-accordion-content-height)" }, }, "accordion-up": { from: { height: "var(--radix-accordion-content-height)" }, to: { height: 0 }, }, }, animation: { "accordion-down": "accordion-down 0.2s ease-out", "accordion-up": "accordion-up 0.2s ease-out", }, }, }, plugins: [require("tailwindcss-animate")], }
대시보드의 핵심 구축
1단계: FaceAuth 구성요소 제작
우리 쇼의 스타인 FaceAuth 구성 요소를 만들어 보겠습니다. 새 파일 app/comComponents/FaceAuth.tsx를 만들고 다음 코드를 붙여넣습니다.
import { useEffect } from 'react'; import faceIO from '@faceio/fiojs'; import { Button, Card, CardHeader, CardTitle, CardContent } from '@shadcn/ui'; import { useToast } from '@shadcn/ui'; interface FaceAuthProps { onSuccessfulAuth: (data: any) => void; } const FaceAuth: React.FC<FaceAuthProps> = ({ onSuccessfulAuth }) => { const { toast } = useToast(); useEffect(() => { const faceio = new faceIO(process.env.NEXT_PUBLIC_FACEIO_APP_ID); const enrollNewUser = async () => { try { const userInfo = await faceio.enroll({ locale: 'auto', payload: { email: 'employee@example.com', pin: '12345', }, }); toast({ title: "Success!", description: "You're now enrolled in the facial recognition system!", }); console.log('User Enrolled!', userInfo); } catch (errCode) { toast({ title: "Oops!", description: "Enrollment failed. Please try again.", variant: "destructive", }); console.error('Enrollment Failed', errCode); } }; const authenticateUser = async () => { try { const userData = await faceio.authenticate(); toast({ title: "Welcome back!", description: "Authentication successful.", }); console.log('User Authenticated!', userData); onSuccessfulAuth({ name: 'John Doe', position: 'Software Developer', department: 'Engineering', photoUrl: 'https://example.com/john-doe.jpg', }); } catch (errCode) { toast({ title: "Authentication failed", description: "Please try again or enroll.", variant: "destructive", }); console.error('Authentication Failed', errCode); } }; const enrollBtn = document.getElementById('enroll-btn'); const authBtn = document.getElementById('auth-btn'); if (enrollBtn) enrollBtn.onclick = enrollNewUser; if (authBtn) authBtn.onclick = authenticateUser; return () => { if (enrollBtn) enrollBtn.onclick = null; if (authBtn) authBtn.onclick = null; }; }, [toast, onSuccessfulAuth]); return ( <Card className="w-full max-w-md mx-auto"> <CardHeader> <CardTitle>Facial Authentication</CardTitle> </CardHeader> <CardContent className="space-y-4"> <Button id="enroll-btn" variant="outline" className="w-full"> Enroll New Employee </Button> <Button id="auth-btn" variant="default" className="w-full"> Authenticate </Button> </CardContent> </Card> ); }; export default FaceAuth;
2단계: EmployeeDashboard 구성 요소 구축
이제 직원들이 보게 될 대시보드를 만들어 보겠습니다. 앱/구성 요소/EmployeeDashboard.tsx 만들기:
import { useState } from 'react'; import { Card, CardHeader, CardTitle, CardContent } from '@shadcn/ui'; import { Button, Avatar, Badge, Table, TableBody, TableCell, TableHead, TableHeader, TableRow } from '@shadcn/ui'; import FaceAuth from './FaceAuth'; interface EmployeeData { name: string; position: string; department: string; photoUrl: string; } const EmployeeDashboard: React.FC = () => { const [isAuthenticated, setIsAuthenticated] = useState(false); const [employeeData, setEmployeeData] = useState<EmployeeData | null>(null); const handleSuccessfulAuth = (data: EmployeeData) => { setIsAuthenticated(true); setEmployeeData(data); }; const mockAttendanceData = [ { date: '2024-07-14', timeIn: '09:00 AM', timeOut: '05:30 PM' }, { date: '2024-07-13', timeIn: '08:55 AM', timeOut: '05:25 PM' }, { date: '2024-07-12', timeIn: '09:05 AM', timeOut: '05:35 PM' }, ]; return ( <div className="space-y-6"> {!isAuthenticated ? ( <FaceAuth onSuccessfulAuth={handleSuccessfulAuth} /> ) : ( <> <Card> <CardHeader> <CardTitle>Employee Profile</CardTitle> </CardHeader> <CardContent className="flex items-center space-x-4"> <Avatar className="h-20 w-20" src={employeeData?.photoUrl} alt={employeeData?.name} /> <div> <h2 className="text-2xl font-bold">{employeeData?.name}</h2> <p className="text-gray-500">{employeeData?.position}</p> <Badge variant="outline">{employeeData?.department}</Badge> </div> </CardContent> </Card> <Card> <CardHeader> <CardTitle>Quick Actions</CardTitle> </CardHeader> <CardContent className="space-y-4"> <Button className="w-full">Check-in</Button> <Button className="w-full" variant="secondary">Request Leave</Button> </CardContent> </Card> <Card> <CardHeader> <CardTitle>Attendance Records</CardTitle> </CardHeader> <CardContent> <Table> <TableHeader> <TableRow> <TableHead>Date</TableHead> <TableHead>Time In</TableHead> <TableHead>Time Out</TableHead> </TableRow> </TableHeader> <TableBody> {mockAttendanceData.map((record, index) => ( <TableRow key={index}> <TableCell>{record.date}</TableCell> <TableCell>{record.timeIn}</TableCell> <TableCell>{record.timeOut}</TableCell> </TableRow> ))} </TableBody> </Table> </CardContent> </Card> </> )} </div> ); }; export default EmployeeDashboard;
3단계: 모든 것을 하나로 모으기
마지막으로 메인 페이지를 업데이트하여 우리의 노력을 보여드리겠습니다. app/page.tsx 업데이트:
import EmployeeDashboard from './components/EmployeeDashboard'; export default function Home() { return ( <main className="flex min-h-screen flex-col items-center justify-center p-4"> <EmployeeDashboard /> </main> ); }
이제 전체 앱을 감싸는 레이아웃을 설정해 보겠습니다. 다음 코드를 추가하세요: app/layout.tsx
import './globals.css' import type { Metadata } from 'next' import { Inter } from 'next/font/google' const inter = Inter({ subsets: ['latin'] }) export const metadata: Metadata = { title: 'Employee Dashboard with Facial Authentication', description: 'A cutting-edge employee dashboard featuring facial recognition for secure authentication and efficient workplace management.', } export default function RootLayout({ children, }: { children: React.ReactNode }) { return ( <html lang="en"> <body className={inter.className}> <header className="bg-primary text-white p-4"> <h1 className="text-2xl font-bold">Faceio Solutions</h1> </header> <main className="container mx-auto p-4"> {children} </main> <footer className="bg-gray-100 text-center p-4 mt-8"> <p>© 2024 Faceio . All rights reserved.</p> </footer> </body> </html> ) }
이 레이아웃은 집의 틀과 같아서 전체 앱에 구조를 제공합니다. 여기에는 회사 이름이 포함된 헤더, 대시보드가 표시될 기본 콘텐츠 영역 및 바닥글이 포함됩니다. 게다가 메타데이터로 SEO 마법을 설정합니다!
FACEIO 통합을 위한 주요 개인 정보 보호 및 보안 관행
개인정보 보호 설계
- 액세스 제어, 사용자 동의, 거부 옵션을 사용하여 개인정보를 보호하세요.
의미있는 동의
- 사용자에게 데이터 수집에 대해 알리십시오.
- 데이터에 대한 선택과 통제의 자유를 제공합니다.
- 언제든지 동의 철회 및 데이터 삭제를 허용하세요.
모범 사례
- 특히 미성년자의 경우 명확하고 적절한 동의를 얻으세요.
- 동의 요청을 쉽게 찾고 이해할 수 있도록 하세요.
- 자동 등록 및 승인되지 않은 등록을 피하세요.
- 생체인식 데이터를 수집하기 전에 사용자에게 알립니다.
- 법적 데이터 개인정보 보호 요구사항을 따르세요.
데이터 보안
- 계정 삭제 시 사용자 데이터를 삭제하세요.
- 강력한 데이터 보관 및 폐기 관행을 유지하세요.
- 보안 조치를 정기적으로 구현하고 검토하세요.
자세한 내용은 FACEIO 모범 사례를 참조하세요.
FACEIO 통합을 위한 주요 보안 고려 사항
보안 설계
- 사용자 신뢰를 유지하려면 애플리케이션 보안이 필수적입니다.
- FACEIO의 보안 모범 사례를 따라 위험을 완화하세요.
핵심 보안 기능
-
취약한 PIN 거부
- 0000이나 1234와 같은 취약한 PIN을 방지하세요.
- 기본값: 아니요
-
중복등록 방지
- 사용자가 여러 번 등록하는 것을 방지합니다.
- 기본값: 아니요
-
딥 페이크로부터 보호
- 스푸핑 시도를 탐지하고 차단합니다.
- 기본값: 아니요
-
미성년자 등록 금지
- 18세 미만 사용자의 등록을 차단합니다.
- 기본값: 아니요
-
인증을 위해 PIN 필요
- 인증할 때마다 PIN 코드가 필요합니다.
- 기본값: 예.
-
고유 PIN 시행
- 각 사용자의 PIN이 고유한지 확인합니다.
- 기본값: 아니요
-
가려진 얼굴 무시
- 어두운 조명이나 부분적으로 마스크를 쓴 얼굴은 폐기됩니다.
- 기본값: 예.
-
누락된 헤더 거부
- 적절한 HTTP 헤더 없이 인스턴스화를 차단합니다.
- 기본값: 예.
-
인스턴스화 제한
- 특정 도메인 및 국가로 제한됩니다.
- 기본값: 아니요
-
웹훅 활성화
- FACEIO 이벤트를 백엔드에 알립니다.
- 기본값: 아니요
자세한 내용은 FACEIO 보안 모범 사례를 참조하세요.
실제 응용 프로그램: 이것을 어디에 사용할 수 있습니까?
이제 멋진 대시보드를 만들었으니 "이걸 실제로 어디에 사용할 수 있지?"라고 궁금해하실 것입니다. 글쎄요, 가능성은 무한합니다! 다음은 몇 가지 아이디어입니다.
사무실 관리: 기존의 펀치 카드와 작별하세요! 이 시스템은 출석을 추적하고, 사무실의 다양한 영역에 대한 접근을 제어하고, 직원 정보를 관리하는 방법을 혁신적으로 바꿀 수 있습니다.
보안 시스템: Fort Knox에 사무실이 있으면서도 번거로움이 없는 세상을 상상해 보세요. 이 얼굴 인식 시스템은 강력한 보안 프로토콜의 초석이 될 수 있습니다.
고객 서비스 키오스크: 고객이 키오스크에 다가가면 즉시 인식하고 맞춤형 서비스를 제공합니다. 더 이상 공상과학 소설이 아닙니다!
다음은 무엇입니까? 하늘이 한계다!
축하합니다, 기술 마법사님! 얼굴 인증 기능을 갖춘 최첨단 직원 대시보드를 구축하셨습니다. 그런데 왜 여기서 멈춰야 할까요? 이 시스템의 장점은 유연성입니다. 다음 단계로 나아가기 위한 몇 가지 아이디어는 다음과 같습니다.
- 중요 업데이트에 대한 실시간 알림 구현
- HR에 대한 세부 보고 기능 추가
- 급여 또는 프로젝트 관리 도구와 같은 다른 시스템과 통합
기술 세계에서 유일한 한계는 상상력(그리고 카페인 섭취량)뿐이라는 점을 기억하세요.
그럼 어떻게 생각하시나요? 귀하의 업무 공간을 미래로 바꿀 준비가 되셨나요? 이 프로젝트를 시도해보고 어떻게 진행되는지 알려주세요. 귀하의 경험, 추가한 멋진 기능 또는 그 과정에서 직면한 어려움에 대해 듣고 싶습니다.
즐거운 코딩 되시기 바랍니다. 얼굴 인식 기능을 사무실 식물로 오해하지 않으시길 바랍니다!
위 내용은 얼굴 인증으로 안전한 직원 대시보드 구축: 종합적인 Next.js 튜토리얼의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
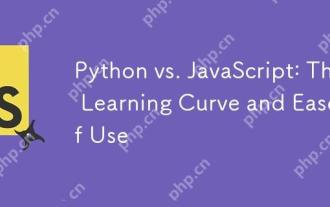
Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
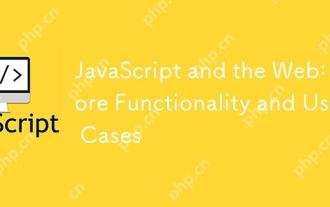
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.
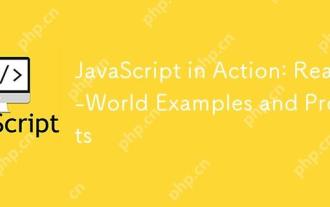
실제 세계에서 JavaScript의 응용 프로그램에는 프론트 엔드 및 백엔드 개발이 포함됩니다. 1) DOM 운영 및 이벤트 처리와 관련된 TODO 목록 응용 프로그램을 구축하여 프론트 엔드 애플리케이션을 표시합니다. 2) Node.js를 통해 RESTFULAPI를 구축하고 Express를 통해 백엔드 응용 프로그램을 시연하십시오.
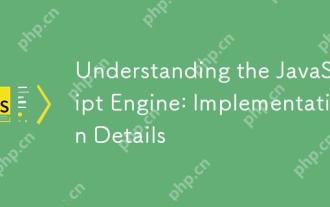
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.
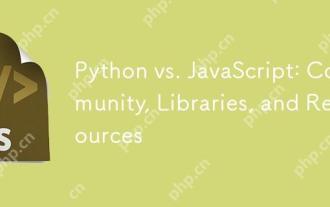
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
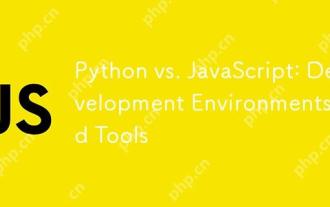
개발 환경에서 Python과 JavaScript의 선택이 모두 중요합니다. 1) Python의 개발 환경에는 Pycharm, Jupyternotebook 및 Anaconda가 포함되어 있으며 데이터 과학 및 빠른 프로토 타이핑에 적합합니다. 2) JavaScript의 개발 환경에는 Node.js, VScode 및 Webpack이 포함되어 있으며 프론트 엔드 및 백엔드 개발에 적합합니다. 프로젝트 요구에 따라 올바른 도구를 선택하면 개발 효율성과 프로젝트 성공률이 향상 될 수 있습니다.
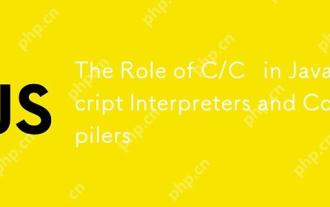
C와 C는 주로 통역사와 JIT 컴파일러를 구현하는 데 사용되는 JavaScript 엔진에서 중요한 역할을합니다. 1) C는 JavaScript 소스 코드를 구문 분석하고 추상 구문 트리를 생성하는 데 사용됩니다. 2) C는 바이트 코드 생성 및 실행을 담당합니다. 3) C는 JIT 컴파일러를 구현하고 런타임에 핫스팟 코드를 최적화하고 컴파일하며 JavaScript의 실행 효율을 크게 향상시킵니다.

Python은 데이터 과학 및 자동화에 더 적합한 반면 JavaScript는 프론트 엔드 및 풀 스택 개발에 더 적합합니다. 1. Python은 데이터 처리 및 모델링을 위해 Numpy 및 Pandas와 같은 라이브러리를 사용하여 데이터 과학 및 기계 학습에서 잘 수행됩니다. 2. 파이썬은 간결하고 자동화 및 스크립팅이 효율적입니다. 3. JavaScript는 프론트 엔드 개발에 없어서는 안될 것이며 동적 웹 페이지 및 단일 페이지 응용 프로그램을 구축하는 데 사용됩니다. 4. JavaScript는 Node.js를 통해 백엔드 개발에 역할을하며 전체 스택 개발을 지원합니다.
