LeetCode 명상: 회문 부분 문자열
회문 하위 문자열에 대한 설명은 다음과 같습니다.
문자열 s가 주어지면 그 안에 있는 회문 하위 문자열의 개수를 반환합니다.
문자열은 뒤에서 읽어도 앞으로 읽어도 같은 내용을 읽을 때 회문입니다.
하위 문자열은 문자열 내의 연속된 문자 시퀀스입니다.
예:
Input: s = "abc" Output: 3 Explanation: Three palindromic strings: "a", "b", "c".
또는:
Input: s = "aaa" Output: 6 Explanation: Six palindromic strings: "a", "a", "a", "aa", "aa", "aaa".
또한 제약 조건에 따르면 s는 영문 소문자로 구성됩니다.
이전 문제에서는 주어진 문자열에서 가장 긴 회문 부분 문자열을 찾는 솔루션을 찾았습니다. 회문을 찾기 위해 우리는 각 문자를 부분 문자열의 중간 문자로 가정하는 "가운데 위로 확장" 접근 방식을 사용했습니다. 이에 따라 왼쪽 포인터와 오른쪽 포인터를 이동시켰습니다.
Note |
---|
Checking a palindrome is easy with two pointers approach, which we've seen before with Valid Palindrome. |
하나의 하위 문자열에서 회문을 세는 것은 다음과 같습니다.
function countPalindromesInSubstr(s: string, left: number, right: number): number { let result = 0; while (left >= 0 && right < s.length && s[left] === s[right]) { result++; left--; right++; } return result; }
범위 내에 있는 한(왼쪽 >= 0 && 오른쪽 < s.length) 왼쪽과 오른쪽의 두 문자가 동일한지 확인합니다. 그렇다면 결과를 업데이트하고 문자를 이동합니다. 포인터.
그러나 일단 생각해 보면 포인터가 어느 인덱스에서 초기화되는지가 중요합니다. 예를 들어 문자열 "abc"를 countPalindromesInSubstr에 전달하고 왼쪽 포인터가 0이고 오른쪽 포인터가 마지막 인덱스(2)에 있는 경우 결과는 단순히 0입니다.
각 문자를 하위 문자열의 중간 문자로 가정하고 각 단일 문자도 하위 문자열이므로 문자 자체를 가리키도록 왼쪽 및 오른쪽 포인터를 초기화합니다.
Note |
---|
A character by itself is considered palindromic, i.e., "abc" has three palindromic substrings: 'a', 'b' and 'c'. |
Let's see how this process might look like.
If we have the string "abc", we'll first assume that 'a' is the middle of a substring:
"abc" left = 0 right = 0 currentSubstr = 'a' totalPalindromes = 1 // A single character is a palindrome
Then, we'll try to expand the substring to see if 'a' can be the middle character of another substring:
"abc" left = -1 right = 1 currentSubstr = undefined totalPalindromes = 1
Now that our left pointer is out of bounds, we can jump to the next character:
"abc" left = 1 right = 1 currentSubstr = 'b' totalPalindromes = 2
Now, we'll update our pointers, and indeed, 'b' can be the middle character of another substring:
s = "abc" left = 0 right = 2 currentSubstr = 'abc' totalPalindromes = 2
Well, currentSubstr is not a palindrome. Now we update our pointers again:
s = "abc" left = -1 right = 3 currentSubstr = undefined totalPalindromes = 2
And, we're out of bounds again. Time to move on to the next character:
s = "abc" left = 2 right = 2 currentSubstr = 'c' totalPalindromes = 3
Shifting our pointers, we'll be out of bounds again:
s = "abc" left = 1 right = 3 currentSubstr = undefined totalPalindromes = 3
Now that we've gone through each character, our final result of totalPalindromes is, in this case, 3. Meaning that there are 3 palindromic substrings in "abc".
However, there is an important caveat: each time we assume a character as the middle and initialize two pointers to the left and right of it, we're trying to find only odd-length palindromes. In order to mitigate that, instead of considering a single character as the middle, we can consider two characters as the middle and expand as we did before.
In this case, the process of finding the even-length substring palindromes will look like this — initially, our right pointer is left + 1:
s = "abc" left = 0 right = 1 currentSubstr = 'ab' totalPalindromes = 0
Then, we'll update our pointers:
s = "abc" left = -1 right = 2 currentSubstr = undefined totalPalindromes = 0
Out of bounds. On to the next character:
s = "abc" left = 1 right = 2 currentSubstr = 'bc' totalPalindromes = 0
Updating our pointers:
s = "abc" left = 0 right = 3 currentSubstr = undefined totalPalindromes = 0
The right pointer is out of bounds, so we go on to the next character:
s = "abc" left = 2 right = 3 currentSubstr = undefined totalPalindromes = 0
Once again we're out of bounds, and we're done going through each character. There are no palindromes for even-length substrings in this example.
We can write a function that does the work of counting the palindromes in each substring:
function countPalindromes(s: string, isOddLength: boolean): number { let result = 0; for (let i = 0; i < s.length; i++) { let left = i; let right = isOddLength ? i : i + 1; result += countPalindromesInSubstr(s, left, right); } return result; }
In our main function, we can call countPalindromes twice for both odd and even length substrings, and return the result:
function countSubstrings(s: string): number { let result = 0; result += countPalindromes(s, true); // Odd-length palindromes result += countPalindromes(s, false); // Even-length palindromes return result; }
Overall, our solution looks like this:
function countSubstrings(s: string): number { let result = 0; result += countPalindromes(s, true); // Odd-length palindromes result += countPalindromes(s, false); // Even-length palindromes return result; } function countPalindromes(s: string, isOddLength: boolean): number { let result = 0; for (let i = 0; i < s.length; i++) { let left = i; let right = isOddLength ? i : i + 1; result += countPalindromesInSubstr(s, left, right); } return result; } function countPalindromesInSubstr(s: string, left: number, right: number): number { let result = 0; while (left >= 0 && right < s.length && s[left] === s[right]) { result++; left--; right++; } return result; }
Time and space complexity
The time complexity is
O(n2)
as we go through each substring for each character (countPalindromes is doing an
O(n2)
operation, we call it twice separately.)
The space complexity is
O(1)
as we don't have an additional data structure whose size will grow with the input size.
Next up is the problem called Decode Ways. Until then, happy coding.
위 내용은 LeetCode 명상: 회문 부분 문자열의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
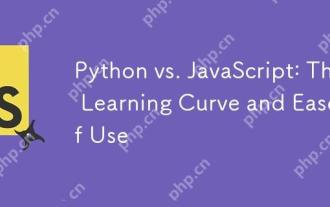
Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
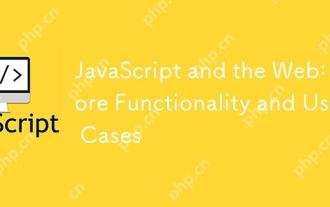
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.
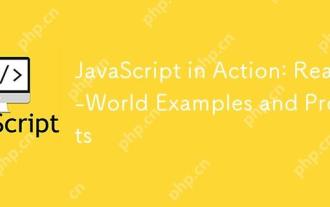
실제 세계에서 JavaScript의 응용 프로그램에는 프론트 엔드 및 백엔드 개발이 포함됩니다. 1) DOM 운영 및 이벤트 처리와 관련된 TODO 목록 응용 프로그램을 구축하여 프론트 엔드 애플리케이션을 표시합니다. 2) Node.js를 통해 RESTFULAPI를 구축하고 Express를 통해 백엔드 응용 프로그램을 시연하십시오.
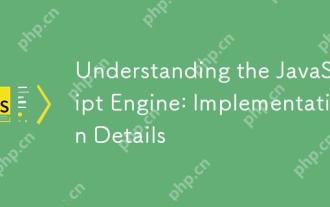
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.
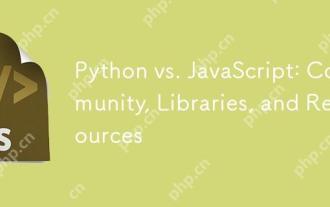
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
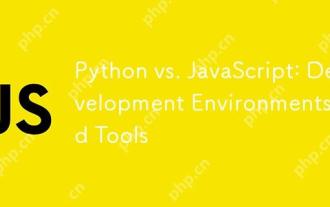
개발 환경에서 Python과 JavaScript의 선택이 모두 중요합니다. 1) Python의 개발 환경에는 Pycharm, Jupyternotebook 및 Anaconda가 포함되어 있으며 데이터 과학 및 빠른 프로토 타이핑에 적합합니다. 2) JavaScript의 개발 환경에는 Node.js, VScode 및 Webpack이 포함되어 있으며 프론트 엔드 및 백엔드 개발에 적합합니다. 프로젝트 요구에 따라 올바른 도구를 선택하면 개발 효율성과 프로젝트 성공률이 향상 될 수 있습니다.
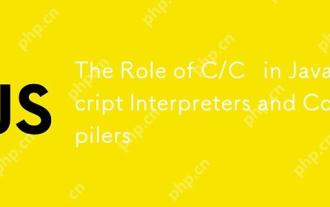
C와 C는 주로 통역사와 JIT 컴파일러를 구현하는 데 사용되는 JavaScript 엔진에서 중요한 역할을합니다. 1) C는 JavaScript 소스 코드를 구문 분석하고 추상 구문 트리를 생성하는 데 사용됩니다. 2) C는 바이트 코드 생성 및 실행을 담당합니다. 3) C는 JIT 컴파일러를 구현하고 런타임에 핫스팟 코드를 최적화하고 컴파일하며 JavaScript의 실행 효율을 크게 향상시킵니다.
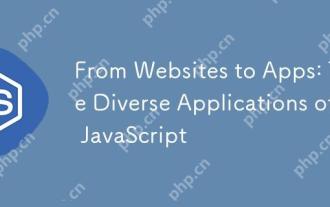
JavaScript는 웹 사이트, 모바일 응용 프로그램, 데스크탑 응용 프로그램 및 서버 측 프로그래밍에서 널리 사용됩니다. 1) 웹 사이트 개발에서 JavaScript는 HTML 및 CSS와 함께 DOM을 운영하여 동적 효과를 달성하고 jQuery 및 React와 같은 프레임 워크를 지원합니다. 2) 반응 및 이온 성을 통해 JavaScript는 크로스 플랫폼 모바일 애플리케이션을 개발하는 데 사용됩니다. 3) 전자 프레임 워크를 사용하면 JavaScript가 데스크탑 애플리케이션을 구축 할 수 있습니다. 4) node.js는 JavaScript가 서버 측에서 실행되도록하고 동시 요청이 높은 높은 요청을 지원합니다.
