Java의 Memento 디자인 패턴 이해
문제
Memento 패턴은 캡슐화를 위반하지 않고 객체의 내부 상태를 캡처하고 복원해야 하는 필요성을 해결합니다. 이는 실행 취소/다시 실행 기능을 구현하여 객체를 이전 상태로 되돌리려는 시나리오에서 유용합니다.
해결책
Memento 패턴에는 세 가지 주요 구성 요소가 포함됩니다.
- Originator: 내부 상태를 저장하고 복원해야 하는 객체입니다.
- 메멘토: 원본의 내부 상태를 저장하는 개체입니다. 기념품은 불변입니다.
- 관리자: 작성자에게 기념품의 상태를 저장하거나 복원하도록 요청하는 역할을 담당합니다.
발신자는 현재 상태의 스냅샷이 포함된 기념품을 만듭니다. 이 기념품은 관리자가 저장하고 필요할 때 작성자의 상태를 복원하는 데 사용할 수 있습니다.
장점과 단점
장점
- 캡슐화 유지: 구현 세부 정보를 노출하지 않고 개체의 내부 상태를 저장하고 복원할 수 있습니다.
- 간단한 실행 취소/다시 실행: 실행 취소/다시 실행 기능 구현을 촉진하여 시스템을 더욱 강력하고 사용자 친화적으로 만듭니다.
- 상태 기록: 객체의 이전 상태 기록을 유지하여 여러 상태 간 탐색을 가능하게 합니다.
단점
- 메모리 소비: 여러 개의 기념품을 저장하면 특히 개체의 상태가 큰 경우 상당한 메모리를 소비할 수 있습니다.
- 추가 복잡성: 기념품 생성 및 복원을 관리해야 하므로 코드가 더욱 복잡해집니다.
- 관리인의 책임: 관리인은 기념품을 효율적으로 관리해야 하며, 이로 인해 시스템에 책임과 복잡성이 가중될 수 있습니다.
실제 적용 사례
Memento 패턴의 실제 예는 실행 취소/다시 실행 기능을 제공하는 텍스트 편집기에 있습니다. 문서의 각 변경 사항은 기념품으로 저장될 수 있으므로 사용자는 필요에 따라 문서의 이전 상태로 되돌릴 수 있습니다.
Java의 예제 코드
코드의 메모 패턴:
// Originator public class Editor { private String content; public void setContent(String content) { this.content = content; } public String getContent() { return content; } public Memento save() { return new Memento(content); } public void restore(Memento memento) { content = memento.getContent(); } // Memento public static class Memento { private final String content; public Memento(String content) { this.content = content; } private String getContent() { return content; } } } // Caretaker public class History { private final Stack<Editor.Memento> history = new Stack<>(); public void save(Editor editor) { history.push(editor.save()); } public void undo(Editor editor) { if (!history.isEmpty()) { editor.restore(history.pop()); } } } // Client code public class Client { public static void main(String[] args) { Editor editor = new Editor(); History history = new History(); editor.setContent("Version 1"); history.save(editor); System.out.println(editor.getContent()); editor.setContent("Version 2"); history.save(editor); System.out.println(editor.getContent()); editor.setContent("Version 3"); System.out.println(editor.getContent()); history.undo(editor); System.out.println(editor.getContent()); history.undo(editor); System.out.println(editor.getContent()); } }
위 내용은 Java의 Memento 디자인 패턴 이해의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
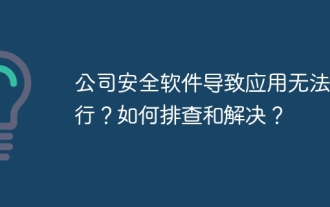
일부 애플리케이션이 제대로 작동하지 않는 회사의 보안 소프트웨어에 대한 문제 해결 및 솔루션. 많은 회사들이 내부 네트워크 보안을 보장하기 위해 보안 소프트웨어를 배포 할 것입니다. ...
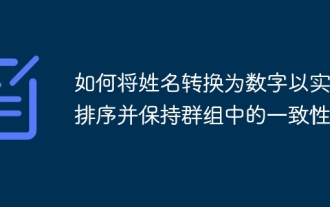
많은 응용 프로그램 시나리오에서 정렬을 구현하기 위해 이름으로 이름을 변환하는 솔루션, 사용자는 그룹으로, 특히 하나로 분류해야 할 수도 있습니다.
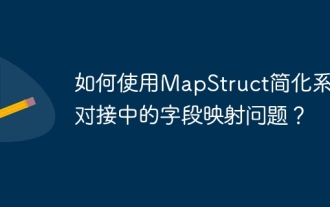
시스템 도킹의 필드 매핑 처리 시스템 도킹을 수행 할 때 어려운 문제가 발생합니다. 시스템의 인터페이스 필드를 효과적으로 매핑하는 방법 ...
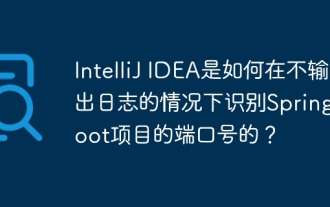
IntellijideAultimate 버전을 사용하여 봄을 시작하십시오 ...
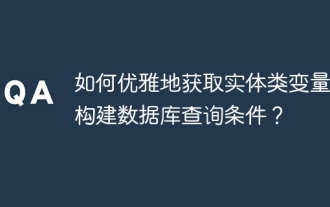
데이터베이스 작업에 MyBatis-Plus 또는 기타 ORM 프레임 워크를 사용하는 경우 엔티티 클래스의 속성 이름을 기반으로 쿼리 조건을 구성해야합니다. 매번 수동으로 ...
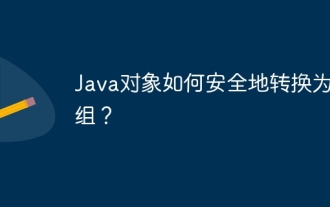
Java 객체 및 배열의 변환 : 캐스트 유형 변환의 위험과 올바른 방법에 대한 심층적 인 논의 많은 Java 초보자가 객체를 배열로 변환 할 것입니다 ...
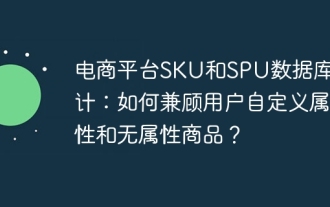
전자 상거래 플랫폼에서 SKU 및 SPU 테이블의 디자인에 대한 자세한 설명이 기사는 전자 상거래 플랫폼에서 SKU 및 SPU의 데이터베이스 설계 문제, 특히 사용자 정의 판매를 처리하는 방법에 대해 논의 할 것입니다 ...
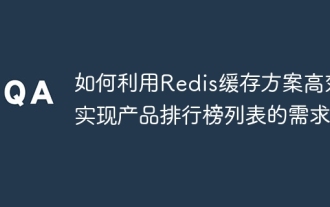
Redis 캐싱 솔루션은 제품 순위 목록의 요구 사항을 어떻게 인식합니까? 개발 과정에서 우리는 종종 a ... 표시와 같은 순위의 요구 사항을 처리해야합니다.
