소프트웨어 개발의 견고한 원칙
소프트웨어 개발 영역에서 SOLID 원칙은 강력하고 유지 관리 가능하며 확장 가능한 소프트웨어 시스템을 만드는 것을 목표로 하는 5가지 설계 원칙 집합입니다. Robert C. Martin(Bob 삼촌이라고도 함)이 만든 이러한 원칙은 개발자가 코드베이스를 깔끔하고 확장 가능하게 유지하기 위해 따라야 할 지침을 제공합니다. 여기서는 각 SOLID 원칙을 살펴보고 이를 Python의 예제를 통해 구현하는 방법을 보여드리겠습니다.
1. 단일 책임 원칙(SRP)
정의: 클래스를 변경해야 하는 이유는 단 하나여야 합니다. 즉, 클래스에는 하나의 작업이나 책임만 있어야 합니다.
예:
class Order: def __init__(self, items): self.items = items def calculate_total(self): return sum(item.price for item in self.items) class InvoicePrinter: @staticmethod def print_invoice(order): print("Invoice:") for item in order.items: print(f"{item.name}: ${item.price}") print(f"Total: ${order.calculate_total()}") # Usage class Item: def __init__(self, name, price): self.name = name self.price = price items = [Item("Apple", 1), Item("Banana", 2)] order = Order(items) InvoicePrinter.print_invoice(order)
이 예에서 Order 클래스는 주문 관리만 담당하고 InvoicePrinter 클래스는 송장 인쇄를 담당합니다. 이는 각 클래스에 단일 책임이 있음을 보장하여 SRP를 준수합니다.
2. 개방/폐쇄 원칙(OCP)
정의: 소프트웨어 엔터티는 확장을 위해 열려 있어야 하고 수정을 위해 닫혀 있어야 합니다.
예:
class Discount: def apply(self, total): return total class PercentageDiscount(Discount): def __init__(self, percentage): self.percentage = percentage def apply(self, total): return total - (total * self.percentage / 100) class FixedDiscount(Discount): def __init__(self, amount): self.amount = amount def apply(self, total): return total - self.amount def calculate_total(order, discount): total = order.calculate_total() return discount.apply(total) # Usage discount = PercentageDiscount(10) print(calculate_total(order, discount))
이 예에서는 OCP를 준수하면서 기본 클래스를 수정하지 않고 할인 클래스가 PercentageDiscount 및 FixDiscount로 확장되었습니다.
3. 리스코프 대체 원리(LSP)
정의: 하위 유형은 프로그램의 정확성을 변경하지 않고 기본 유형을 대체할 수 있어야 합니다.
예:
class Bird: def fly(self): pass class Sparrow(Bird): def fly(self): print("Sparrow is flying") class Ostrich(Bird): def fly(self): raise Exception("Ostrich can't fly") def make_bird_fly(bird): bird.fly() # Usage sparrow = Sparrow() make_bird_fly(sparrow) ostrich = Ostrich() try: make_bird_fly(ostrich) except Exception as e: print(e)
여기서 타조는 날 수 없기 때문에 LSP를 위반하므로 Bird 기반 클래스를 대체할 수 없습니다.
4. 인터페이스 분리 원칙(ISP)
정의: 클라이언트가 사용하지 않는 인터페이스에 의존하도록 강요해서는 안 됩니다.
예:
from abc import ABC, abstractmethod class Printer(ABC): @abstractmethod def print_document(self, document): pass class Scanner(ABC): @abstractmethod def scan_document(self, document): pass class MultiFunctionPrinter(Printer, Scanner): def print_document(self, document): print(f"Printing: {document}") def scan_document(self, document): print(f"Scanning: {document}") class SimplePrinter(Printer): def print_document(self, document): print(f"Printing: {document}") # Usage mfp = MultiFunctionPrinter() mfp.print_document("Report") mfp.scan_document("Report") printer = SimplePrinter() printer.print_document("Report")
이 예에서 MultiFunctionPrinter는 프린터와 스캐너 인터페이스를 모두 구현하는 반면 SimplePrinter는 ISP를 준수하는 프린터만 구현합니다.
5. 의존성 역전 원리(DIP)
정의: 상위 모듈은 하위 모듈에 종속되어서는 안 됩니다. 둘 다 추상화에 의존해야 합니다. 추상화는 세부사항에 의존해서는 안 됩니다. 세부 사항은 추상화에 따라 달라집니다.
예:
from abc import ABC, abstractmethod class Database(ABC): @abstractmethod def save(self, data): pass class MySQLDatabase(Database): def save(self, data): print("Saving data to MySQL database") class MongoDBDatabase(Database): def save(self, data): print("Saving data to MongoDB database") class UserService: def __init__(self, database: Database): self.database = database def save_user(self, user_data): self.database.save(user_data) # Usage mysql_db = MySQLDatabase() mongo_db = MongoDBDatabase() user_service = UserService(mysql_db) user_service.save_user({"name": "John Doe"}) user_service = UserService(mongo_db) user_service.save_user({"name": "Jane Doe"})
이 예에서 UserService는 데이터베이스 추상화에 의존하여 유연성을 허용하고 DIP를 준수합니다.
결론
SOLID 원칙을 준수함으로써 개발자는 더욱 모듈화되고, 유지 관리가 더 쉽고, 확장 가능한 소프트웨어를 만들 수 있습니다. 이러한 원칙은 소프트웨어 개발의 복잡성을 관리하고 코드가 깔끔하고 확장 가능하도록 보장하는 데 도움이 됩니다. Python의 실제 예제를 통해 이러한 원칙을 적용하여 강력하고 유지 관리가 가능한 시스템을 만드는 방법을 확인할 수 있습니다.
위 내용은 소프트웨어 개발의 견고한 원칙의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










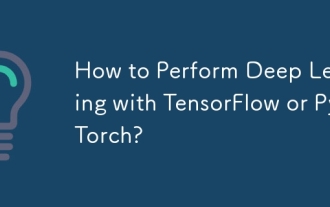
Tensorflow 또는 Pytorch로 딥 러닝을 수행하는 방법은 무엇입니까?
