힙 | Javascript를 이용한 우선순위 대기열 구현
소개
힙은 힙 속성을 만족하는 특별한 트리 기반 데이터 구조입니다.
완전 이진 트리입니다. 즉, 왼쪽에서 오른쪽으로 채워지는 마지막 수준을 제외하고 트리의 모든 수준이 완전히 채워집니다.
힙에는 두 가지 유형이 있습니다.
- 최대 힙
- 최소 힙.
최대 힙:
최대 힙에서 특정 노드 I에 대해 I의 값은 해당 하위 노드의 값보다 크거나 같습니다.
이 속성은 이진 트리의 모든 노드에 대해 true여야 합니다. 가장 높은 값(최대값)은 트리의 루트에 있습니다.
최소 힙:
최소 힙에서 특정 노드 I에 대해 I의 값은 해당 하위 노드의 값보다 작거나 같습니다.
이 속성은 이진 트리의 모든 노드에 대해 true여야 합니다. 가장 낮은 값(최소값)은 트리 루트에 있습니다.
삽입 및 삭제 작업을 수행할 때마다 각각 bubbleUp 및 bubbleDown을 수행하므로 우선순위 대기열이라고 부를 수 있습니다.
배열에서도 동일하게 구현할 수 있지만 leftChildindex, rightChildIndex 및 parentIndex를 어떻게 계산합니까?
수식
아이를 얻으려면
2i+1(왼쪽)
2i+2(오른쪽)
부모님을 모시려면
i-1/2
아래에 minHeap 구현을 추가했습니다. 확인하세요.
class MinHeap { constructor() { this.data = []; this.length = 0; } insert(value) { this.data.push(value); this.length++; this.bubbleUp(this.length-1); } bubbleUp(index) { if (index == 0) { return ; } const parentIndex = this.getParentIndex(index); const parentValue = this.data[parentIndex]; const value = this.data[index]; if (parentValue > value) { this.data[parentIndex] = this.data[index]; this.data[index] = parentValue; this.bubbleUp(parentIndex); } } getParentIndex(index) { return Math.floor((index-1)/2); } getLeftChildIndex(index) { return 2*index + 1; } getRightChildIndex(index) { return 2*index + 2; } bubbleDown(index) { if (index >= this.length) { return; } const lIndex = this.getLeftChildIndex(index); const rIndex = this.getRightChildIndex(index); const lValue = this.data[lIndex]; const rValue = this.data[rIndex]; const value = this.data[index]; if (lValue < rValue && lValue < value) { // swap this.data[index] = this.data[lIndex]; this.data[lIndex] = value; this.bubbleDown(lIndex); } else if (rValue < lValue && rValue < value) { this.data[index] = this.data[rIndex]; this.data[rIndex] = value; this.bubbleDown(rIndex) } } delete() { if (this.length === 0) { return -1; } const out = this.data[0]; if (this.length == 1) { this.data = []; this.length = 0; return out; } this.data[0] = this.data[this.length-1]; this.length--; this.bubbleDown(0); } } const test = new MinHeap(); test.insert(50); test.insert(100); test.insert(101); test.insert(20); test.insert(110); console.log(test) test.delete(); console.log('---------After Delete -------') console.log(test) /* MinHeap { data: [ 20, 50, 101, 100, 110 ], length: 5 } ---------After Delete ------- MinHeap { data: [ 50, 100, 101, 110, 110 ], length: 4 } */
질문이 있으시면 언제든지 연락주세요.
위 내용은 힙 | Javascript를 이용한 우선순위 대기열 구현의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
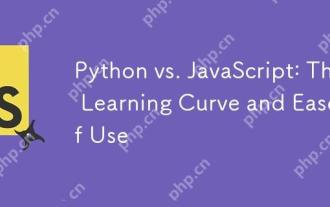
Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
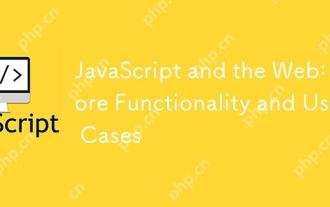
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.
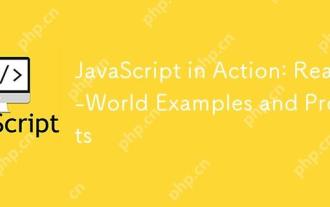
실제 세계에서 JavaScript의 응용 프로그램에는 프론트 엔드 및 백엔드 개발이 포함됩니다. 1) DOM 운영 및 이벤트 처리와 관련된 TODO 목록 응용 프로그램을 구축하여 프론트 엔드 애플리케이션을 표시합니다. 2) Node.js를 통해 RESTFULAPI를 구축하고 Express를 통해 백엔드 응용 프로그램을 시연하십시오.
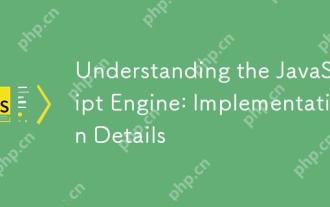
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.
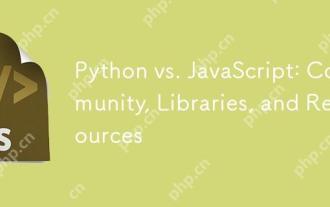
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
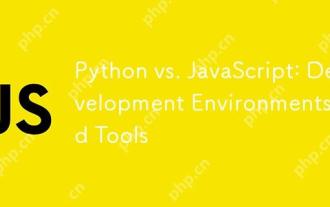
개발 환경에서 Python과 JavaScript의 선택이 모두 중요합니다. 1) Python의 개발 환경에는 Pycharm, Jupyternotebook 및 Anaconda가 포함되어 있으며 데이터 과학 및 빠른 프로토 타이핑에 적합합니다. 2) JavaScript의 개발 환경에는 Node.js, VScode 및 Webpack이 포함되어 있으며 프론트 엔드 및 백엔드 개발에 적합합니다. 프로젝트 요구에 따라 올바른 도구를 선택하면 개발 효율성과 프로젝트 성공률이 향상 될 수 있습니다.
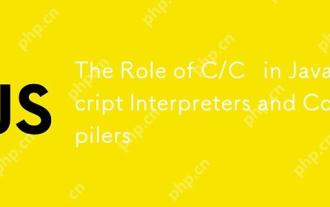
C와 C는 주로 통역사와 JIT 컴파일러를 구현하는 데 사용되는 JavaScript 엔진에서 중요한 역할을합니다. 1) C는 JavaScript 소스 코드를 구문 분석하고 추상 구문 트리를 생성하는 데 사용됩니다. 2) C는 바이트 코드 생성 및 실행을 담당합니다. 3) C는 JIT 컴파일러를 구현하고 런타임에 핫스팟 코드를 최적화하고 컴파일하며 JavaScript의 실행 효율을 크게 향상시킵니다.

Python은 데이터 과학 및 자동화에 더 적합한 반면 JavaScript는 프론트 엔드 및 풀 스택 개발에 더 적합합니다. 1. Python은 데이터 처리 및 모델링을 위해 Numpy 및 Pandas와 같은 라이브러리를 사용하여 데이터 과학 및 기계 학습에서 잘 수행됩니다. 2. 파이썬은 간결하고 자동화 및 스크립팅이 효율적입니다. 3. JavaScript는 프론트 엔드 개발에 없어서는 안될 것이며 동적 웹 페이지 및 단일 페이지 응용 프로그램을 구축하는 데 사용됩니다. 4. JavaScript는 Node.js를 통해 백엔드 개발에 역할을하며 전체 스택 개발을 지원합니다.
