Liskov 대체 원칙
객체는 코드의 정확성에 영향을 주지 않고 해당 하위 유형으로 대체 가능해야 합니다
상속으로 이해해보자(is-a 관계)
예: 타조는 새, 꼽추는 자동차 등
예: Racing-car는 자동차입니다
public class Car{ public double getCabinWidth(){ //return cabin width } }
public class RacingCar extends Car{ @Override public double getCabinWidth(){ //UNIMPLEMENTED } public double getCockpitWidth(){ //return the cockpit width of the racing car } }
RacingCar는 자동차 클래스의 getCabinWidth()를 재정의하지만 구현되지 않은 상태로 둡니다. 왜냐하면 경주용 자동차에는 캐빈 너비가 없기 때문입니다(F1 경주용 자동차를 보면 내부 공간이 없습니다. 운전자가 앉는 조종석만 있을 뿐입니다)
그래서 경주용 자동차의 내부 공간을 콕핏(Cockpit)이라고 부릅니다.
참고: 레이싱카에는 일반 자동차와 일치하지 않을 수 있는 일부 사양이 있습니다
public class CarUtil{ Car car1 = new Car(); Car car2 = new Car(); Car car3 = new RacingCar(); List<Car> myCars = new ArrayList<>(); myCars.add(car1); myCars.add(car2); myCars.add(car3); // this will not work in 3rd iteration, because the getCabinWidth() in RacingCar is not implemented for(Car car : myCars){ System.out.println(car.getCabinWidth()); } }
3번째 반복에서는 for 루프가 실패하므로 노출된 디자인입니다.
이 문제를 해결하려면 상속 자체인 뿌리를 공격해야 합니다.
해결책 1 : (계층 구조 깨기)
상속을 깨야 합니다. 대신 Car와 RacingCar 모두에 대한 공통 부모를 찾아내겠습니다
Vehicle이라는 매우 일반적인 상위 클래스를 생성합니다
public class Vehicle{ public double getInteriorWidth(){ //return the interior width } }
public class Car extends Vehicle{ @Override public double getInteriorWidth(){ return this.getCabinWidth(); } public double getCabinWidth(){ //return cabin width } }
public class RacingCar extends Vehicle{ @Override public double getInteriorWidth(){ return this.getCockpitWidth(); } public double getCockpitWidth(){ //return the cockpit width of the racing car } }
public class VehicleUtils{ Vehicle vehicle1 = new Car(); Vehicle vehicle2 = new Car(); Vehicle vehicle2 = new RacingCar(); List<Vehicle> vehicles = new ArrayList<>(); vehicles.add(vehicle1); vehicles.add(vehicle2); vehicles.add(vehicle3); for(Vehicle vehicle : vehicles){ System.out.println(vehicle.getInteriorWidth()); } }
**계층 구조 깨기: 대체가 실패하면 계층 구조 깨기
해결책 2: 묻지 말고 말하세요
아마존의 또 다른 예를 들어보겠습니다
Amazon은 모든 타사 제품에 대해 x 금액의 할인을 제공합니다.
그리고 모든 사내 제품에 대해 1.5배 x 혜택을 제공합니다(Amazon Basics 제품은 Amazon 사내 제품입니다)
public class Product{ public double discount = 20;//x amount of discount on all the third-party products on Amazon public double getDiscount(){ return discount; } }
public class InHouseProduct extends Product{ public void applyDiscount(){ discount = discount*1.5;// 1.5 times more discount on InHouseProducts } }
public class PricingUtils{ Product p1 = new Product(); Product p2 = new Product(); Product p2 = new InHouseProduct(); List<Product> products = new ArrayList<>(); products.add(p1); products.add(p2); products.add(p2); for(Product product : products){ if(product instanceOf InHouseProduct){ ((InHouseProduct)product).applyDiscount(); } System.out.println(product.getDiscount()); } }
if 문은 Liskov 대체 원칙에 위배되는 InHouseProduct의 할인 금액을 업데이트하는 데 관련되어 있습니다(대상 Product를 해당 하위 유형 InHouseProduct로 대체할 수 있어야 했기 때문에). if 문에서는 수행해서는 안 되는 할인 금액을 수동으로 업데이트하고 있습니다.
InHouseProduct 클래스를 약간 수정하면 이 문제가 해결됩니다
public class InHouseProduct extends Product{ @Override public double getDiscount(){ applyDiscount(); return discount; } public void applyDiscount(){ discount = discount*1.5; } }
마지막으로 PricingUtils 클래스에서 if 문을 제거했습니다
public class PricingUtils{ Product p1 = new Product(); Product p2 = new Product(); Product p2 = new InHouseProduct(); List<Product> products = new ArrayList<>(); products.add(p1); products.add(p2); products.add(p2); for(Product product : products){ System.out.println(product.getDiscount()); } }
묻지마 말하세요: 여기서는 utils 클래스에 모든 할인을 인쇄하라고 지시하고 있으며 utils 클래스는 아무것도 묻지 않아도 됩니다. 이전 성명)
위 내용은 Liskov 대체 원칙의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










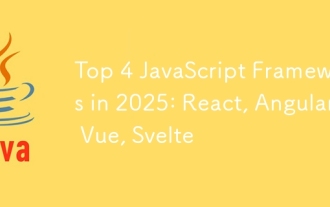
2025 년 상위 4 개의 JavaScript 프레임 워크 : React, Angular, Vue, Svelte
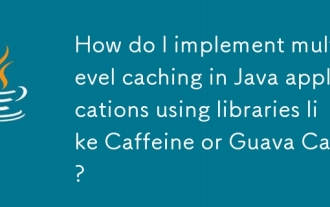
카페인 또는 구아바 캐시와 같은 라이브러리를 사용하여 자바 애플리케이션에서 다단계 캐싱을 구현하려면 어떻게해야합니까?
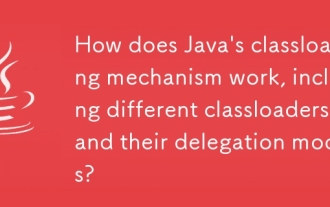
Java의 클래스로드 메커니즘은 다른 클래스 로더 및 대표 모델을 포함하여 어떻게 작동합니까?
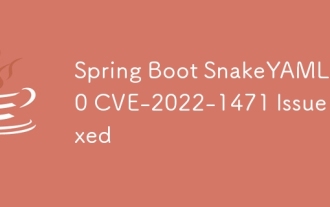
Spring Boot Snakeyaml 2.0 CVE-2022-1471 문제 고정
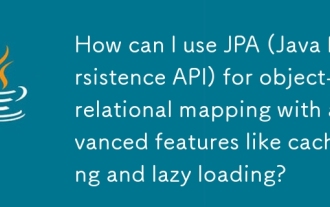
캐싱 및 게으른 하중과 같은 고급 기능을 사용하여 객체 관계 매핑에 JPA (Java Persistence API)를 어떻게 사용하려면 어떻게해야합니까?
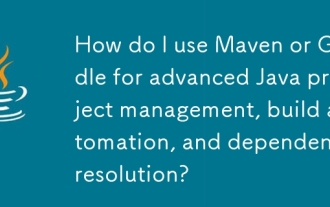
고급 Java 프로젝트 관리, 구축 자동화 및 종속성 해상도에 Maven 또는 Gradle을 어떻게 사용합니까?
