AWS Rekognition 및 Node.js를 사용하여 이미지에서 텍스트 감지
안녕하세요 여러분! 이 기사에서는 Node.js와 함께 AWS Rekognition을 사용하여 이미지 텍스트 감지를 수행하는 간단한 애플리케이션을 생성하겠습니다.
AWS Rekognition이란 무엇입니까?
Amazon Rekognition은 애플리케이션에 이미지 및 비디오 분석을 쉽게 추가할 수 있게 해주는 서비스입니다. 텍스트 감지, 얼굴 인식, 유명 인사 감지 등의 기능을 제공합니다.
Rekognition은 S3에 저장된 이미지나 비디오를 분석할 수 있지만, 이 튜토리얼에서는 작업을 단순하게 유지하기 위해 S3 없이 작업하겠습니다.
우리는 백엔드에 Express를 사용하고 프론트엔드에 React를 사용할 것입니다.
첫걸음
시작하기 전에 AWS 계정을 생성하고 IAM 사용자를 설정해야 합니다. 이미 갖고 계시다면 이 섹션을 건너뛰셔도 됩니다.
IAM 사용자 생성
- AWS에 로그인: 먼저 AWS 루트 계정에 로그인하세요.
- IAM 검색: AWS 콘솔에서 IAM을 검색하여 선택합니다.
- 사용자 섹션으로 이동하여 사용자 만들기를 클릭합니다.
- 사용자 이름을 설정하고 권한 설정에서 정책 직접 연결을 선택합니다.
- 인식 정책을 검색하여 선택한 후 다음을 클릭하고 사용자를 생성하세요.
- 액세스 키 생성: 사용자를 생성한 후 사용자를 선택하고 보안 자격 증명 탭에서 액세스 키를 생성합니다. 액세스 키와 비밀 액세스 키가 포함된 .csv 파일을 다운로드하세요.
- 자세한 지침은 공식 AWS 설명서(https://docs.aws.amazon.com/IAM/latest/UserGuide/id_users_create.html)를 참조하세요.
aws-sdk 구성
- AWS CLI 설치: 시스템에 AWS CLI를 설치합니다.
- 설치 확인: 터미널이나 명령 프롬프트를 열고 aws --version을 입력하여 CLI가 올바르게 설치되었는지 확인하세요.
- AWS CLI 구성: awsconfigure를 실행하고 다운로드한 .csv 파일에서 액세스 키, 보안 액세스 키 및 리전을 제공합니다.
프로젝트 디렉토리
my-directory/ │ ├── client/ │ └── src/ │ └── App.jsx │ └── public/ │ └── package.json │ └── ... (other React project files) │ └── server/ ├── index.js └── rekognition/ └── aws.rek.js
프런트엔드 설정
npm은 vite @latest를 만듭니다. -- --템플릿 반응
클라이언트 폴더에 반응 프로젝트가 생성됩니다.
App.jsx에서
import { useState } from "react"; function App() { const [img, setImg] = useState(null); const handleImg = (e) => { setImg(e.target.files[0]); // Store the selected image in state }; const handleSubmit = (e) => { e.preventDefault(); if (!img) return; const formData = new FormData(); formData.append("img", img); console.log(formData); // Log the form data to the console }; return ( <div> <form onSubmit={handleSubmit}> <input type="file" name="img" accept="image/*" onChange={handleImg} /> <br /> <button type="submit">Submit</button> </form> </div> ); } export default App;
제출 후 이미지가 콘솔에 기록되는지 확인하여 이를 테스트해 보겠습니다.
이제 백엔드로 이동하여 이 프로젝트의 영혼을 만들어 보겠습니다.
백엔드 초기화
서버 폴더
npm init -y
npm install express cors nodemon multer @aws-sdk/client-rekognition
로직 분석을 처리하고 해당 폴더 안에 파일을 생성하기 위해 인식을 위한 별도의 폴더를 만들었습니다.
//aws.rek.js import { RekognitionClient, DetectTextCommand, } from "@aws-sdk/client-rekognition"; const client = new RekognitionClient({}); export const Reko = async (params) => { try { const command = new DetectTextCommand( { Image: { Bytes:params //we are using Bytes directly instead of S3 } } ); const response = await client.send(command); return response } catch (error) { console.log(error.message); } };
설명
- RekognitionClient 객체를 초기화합니다. 이미 SDK를 구성했으므로 중괄호를 비워 둘 수 있습니다.
- 이미지를 처리하기 위해 비동기 함수 Reko를 만듭니다. 이 함수에서는 바이트 단위로 이미지를 가져오는 DetectTextCommand 개체를 초기화합니다.
- 이 DectedTextCommand는 텍스트 감지에 특별히 사용됩니다.
- 함수는 응답을 기다렸다가 반환합니다.
API 생성
서버 폴더에서 index.js 파일이나 원하는 이름을 만드세요.
//index.js import express from "express" import multer from "multer" import cors from "cors" import { Reko } from "./rekognition/aws.rek.js"; const app = express() app.use(cors()) const storage = multer.memoryStorage() const upload = multer() const texts = [] let data = [] app.post("/img", upload.single("img"), async(req,res) => { const file = req.file data = await Reko(file.buffer) data.TextDetections.map((item) => { texts.push(item.DetectedText) }) res.status(200).send(texts) }) app.listen(3000, () => { console.log("server started"); })
설명
- Express를 초기화하고 서버를 시작합니다.
- 멀터를 사용하여 다중 부분 양식 데이터를 처리하고 이를 임시로 버퍼에 저장합니다.
- 사용자로부터 이미지를 가져오기 위해 게시물 요청을 생성합니다. 이것은 비동기 기능입니다.
- 사용자가 이미지를 업로드하면 해당 이미지는 req.file에서 사용할 수 있습니다.
- 이 req.file에는 이미지 데이터를 8비트 버퍼로 보유하는 Buffer 속성이 있다는 점에서 몇 가지 속성이 포함되어 있습니다.
- 이것이 필요하므로 해당 req.file.buffer를 인식 기능에 전달합니다. 이를 분석한 후 함수는 객체 배열을 반환합니다.
- 해당 개체의 텍스트를 사용자에게 보냅니다.
프런트엔드로 돌아옴
import axios from "axios"; import { useState } from "react"; import "./App.css"; function App() { const [img, setImg] = useState(null); const [pending, setPending] = useState(false); const [texts, setTexts] = useState([]); const handleImg = (e) => { setImg(e.target.files[0]); }; const handleSubmit = async (e) => { e.preventDefault(); if (!img) return; const formData = new FormData(); formData.append("img", img); try { setPending(true); const response = await axios.post("http://localhost:3000/img", formData); setTexts(response.data); } catch (error) { console.log("Error uploading image:", error); } finally { setPending(false); } }; return ( <div className="app-container"> <div className="form-container"> <form onSubmit={handleSubmit}> <input type="file" name="img" accept="image/*" onChange={handleImg} /> <br /> <button type="submit" disabled={pending}> {pending ? "Uploading..." : "Upload Image"} </button> </form> </div> <div className="result-container"> {pending && <h1>Loading...</h1>} {texts.length > 0 && ( <ul> {texts.map((text, index) => ( <li key={index}>{text}</li> ))} </ul> )} </div> </div> ); } export default App;
- Axios를 사용하여 이미지를 게시합니다. 응답을 텍스트 상태로 저장합니다.
- 텍스트를 표시하면서 지금은 인덱스를 키로 사용하고 있지만 인덱스를 키로 사용하는 것은 권장되지 않습니다.
- 로드 상태 및 일부 스타일과 같은 몇 가지 추가 사항도 추가했습니다.
최종 출력
'이미지 업로드' 버튼을 클릭하면 백엔드가 이미지를 처리하고 감지된 텍스트를 반환한 다음 사용자에게 표시합니다.
전체 코드를 보려면 내 GitHub Repo를 확인하세요
감사합니다!!!
팔로우: Medium, GitHub, LinkedIn, X, Instagram
위 내용은 AWS Rekognition 및 Node.js를 사용하여 이미지에서 텍스트 감지의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
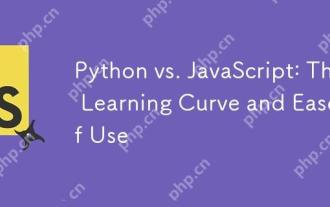
Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
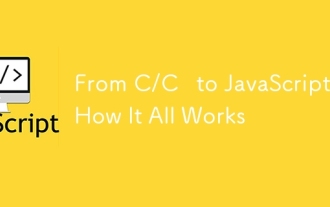
C/C에서 JavaScript로 전환하려면 동적 타이핑, 쓰레기 수집 및 비동기 프로그래밍으로 적응해야합니다. 1) C/C는 수동 메모리 관리가 필요한 정적으로 입력 한 언어이며 JavaScript는 동적으로 입력하고 쓰레기 수집이 자동으로 처리됩니다. 2) C/C를 기계 코드로 컴파일 해야하는 반면 JavaScript는 해석 된 언어입니다. 3) JavaScript는 폐쇄, 프로토 타입 체인 및 약속과 같은 개념을 소개하여 유연성과 비동기 프로그래밍 기능을 향상시킵니다.
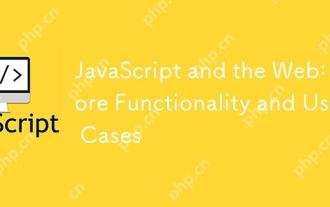
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.
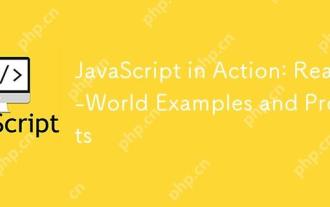
실제 세계에서 JavaScript의 응용 프로그램에는 프론트 엔드 및 백엔드 개발이 포함됩니다. 1) DOM 운영 및 이벤트 처리와 관련된 TODO 목록 응용 프로그램을 구축하여 프론트 엔드 애플리케이션을 표시합니다. 2) Node.js를 통해 RESTFULAPI를 구축하고 Express를 통해 백엔드 응용 프로그램을 시연하십시오.
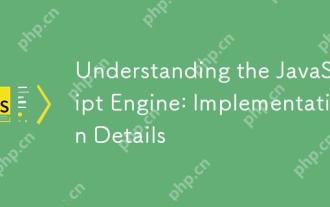
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.
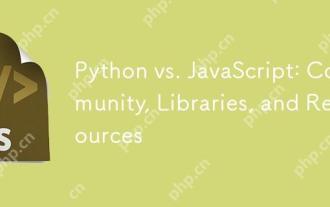
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
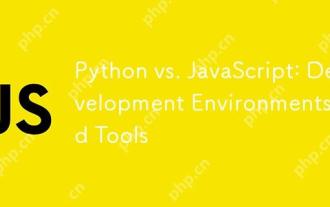
개발 환경에서 Python과 JavaScript의 선택이 모두 중요합니다. 1) Python의 개발 환경에는 Pycharm, Jupyternotebook 및 Anaconda가 포함되어 있으며 데이터 과학 및 빠른 프로토 타이핑에 적합합니다. 2) JavaScript의 개발 환경에는 Node.js, VScode 및 Webpack이 포함되어 있으며 프론트 엔드 및 백엔드 개발에 적합합니다. 프로젝트 요구에 따라 올바른 도구를 선택하면 개발 효율성과 프로젝트 성공률이 향상 될 수 있습니다.
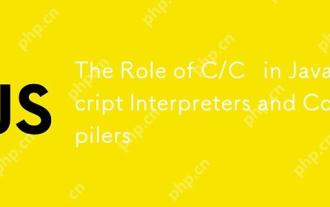
C와 C는 주로 통역사와 JIT 컴파일러를 구현하는 데 사용되는 JavaScript 엔진에서 중요한 역할을합니다. 1) C는 JavaScript 소스 코드를 구문 분석하고 추상 구문 트리를 생성하는 데 사용됩니다. 2) C는 바이트 코드 생성 및 실행을 담당합니다. 3) C는 JIT 컴파일러를 구현하고 런타임에 핫스팟 코드를 최적화하고 컴파일하며 JavaScript의 실행 효율을 크게 향상시킵니다.
