MERN 스택 애플리케이션 구축 - 모범 사례 및 팁
1. MERN 스택 이해
MERN 스택은 MongoDB, Express.js, React.js 및 Node.js를 결합하여 최신 웹 애플리케이션을 구축하는 데 가장 인기 있는 선택 중 하나가 되었습니다. 전체 스택 JavaScript 프레임워크인 MERN 스택은 프런트엔드 및 백엔드 개발자 모두에게 원활한 개발 환경을 제공합니다. 그러나 MERN 스택 애플리케이션을 구축하려면 최적의 성능과 확장성을 보장하기 위해 신중한 계획과 구현이 필요합니다. 이 블로그에서는 API 통합 및 데이터베이스 관리부터 인증 및 배포에 이르기까지 모든 것을 다루는 MERN 스택 애플리케이션 구축을 위한 모범 사례와 팁을 살펴보겠습니다. 풀 스택 개발을 처음 접하는 사람이든 기존 MERN 스택 프로젝트를 개선하려는 사람이든 이 블로그는 강력하고 효율적인 웹 애플리케이션을 구축하는 데 도움이 되는 귀중한 통찰력을 제공할 것입니다.
2. MERN 스택 애플리케이션 구축을 위한 모범 사례
MERN 스택 애플리케이션의 성공을 보장하려면 효율성, 확장성 및 유지 관리성을 촉진하는 모범 사례를 따르는 것이 중요합니다. 명심해야 할 몇 가지 주요 모범 사례는 다음과 같습니다.
- 코드 모듈화:
코드 구성과 재사용성을 향상하려면 코드를 재사용 가능한 구성 요소로 분할하세요.
// Example: Modularizing a React Component function Header() { return <h1>My MERN Stack App</h1>; } function App() { return ( <div> <Header /> {/* Other components */} </div> ); }
- 적절한 오류 처리 구현:
오류를 적절하게 처리하여 더 나은 사용자 경험을 제공하고 디버깅을 용이하게 합니다.
// Example: Express.js Error Handling Middleware app.use((err, req, res, next) => { console.error(err.stack); res.status(500).send('Something broke!'); });
- 성능 최적화:
향상된 성능을 위해 효율적인 코드를 작성하고, 캐싱 메커니즘을 활용하고, 데이터베이스 쿼리를 최적화하세요.
// Example: Using Redis for Caching const redis = require('redis'); const client = redis.createClient(); app.get('/data', (req, res) => { client.get('data', (err, data) => { if (data) { res.send(JSON.parse(data)); } else { // Fetch data from database and cache it } }); });
- 철저한 테스트 실시:
개발 프로세스 초기에 문제를 식별하고 수정하려면 애플리케이션을 엄격하게 테스트하세요.
// Example: Jest Unit Test for a Function test('adds 1 + 2 to equal 3', () => { expect(1 + 2).toBe(3); });
- 보안 모범 사례를 따릅니다.
보안 인증 방법을 구현하고, 사용자 입력을 삭제하고, 일반적인 보안 취약성으로부터 보호합니다.
// Example: Sanitizing User Input const sanitizeHtml = require('sanitize-html'); const cleanInput = sanitizeHtml(userInput);
이러한 모범 사례를 준수하면 최고 수준의 품질을 충족하는 안정적이고 확장 가능한 MERN 스택 애플리케이션을 구축할 수 있습니다.
아. 올바른 도구 및 기술 선택
MERN 스택 애플리케이션을 구축할 때는 올바른 도구와 기술을 선택하는 것이 중요합니다. 적절한 라이브러리, 프레임워크 및 개발 도구를 선택하면 프로젝트의 품질과 효율성에 큰 영향을 미칠 수 있습니다. 이러한 결정을 내릴 때 커뮤니티 지원, 애플리케이션 요구 사항과의 호환성 및 통합 용이성과 같은 요소를 고려하십시오. 예를 들어, 안정적인 성능을 위해 Express.js 및 React.js와 같이 잘 확립된 라이브러리를 선택하십시오. 또한 API 테스트용 Postman, 데이터베이스 관리용 MongoDB Compass와 같은 도구를 활용하여 개발 프로세스를 간소화하세요. 프로젝트 요구 사항에 맞는 도구와 기술을 신중하게 선택하면 생산성을 높이고 최고의 MERN 스택 애플리케이션을 제공할 수 있습니다.
ㄴ. 깔끔하고 확장 가능한 코드 유지
MERN 스택 애플리케이션의 지속 가능성과 확장성을 보장하려면 개발 프로세스 전반에 걸쳐 깨끗하고 효율적인 코드를 유지하는 것이 가장 중요합니다. 일관된 코딩 스타일 준수, 모듈화 활용, 디자인 패턴 구현과 같은 모범 사례를 준수하면 코드 가독성과 관리 용이성을 향상시킬 수 있습니다. 코드베이스를 정기적으로 리팩터링하여 중복성을 제거하고 성능을 향상시키세요. 코드 베이스의 이해와 유지 관리에 도움이 되는 의미 있는 주석과 문서 작성의 중요성을 강조합니다. 깔끔하고 확장 가능한 코드를 우선시함으로써 MERN 스택 애플리케이션의 장기적인 성공을 위한 견고한 기반을 마련할 수 있습니다.
// Example: Using ES6 Modules for Clean Code import express from 'express'; import { connectToDB } from './db'; import routes from './routes'; const app = express(); connectToDB(); app.use('/api', routes);
ㄷ. 보안 조치 구현
MERN 스택 애플리케이션을 잠재적인 사이버 위협으로부터 보호하려면 강력한 보안 조치를 구현하는 것이 중요합니다. 사용자 권한 부여 및 인증을 위해 JWT(JSON 웹 토큰)와 같은 보안 인증 방법을 활용하세요. SQL 주입 및 크로스 사이트 스크립팅 공격과 같은 일반적인 보안 취약점을 방지하기 위해 입력 유효성 검사를 구현합니다. 애플리케이션에 사용된 패키지에서 보고된 보안 취약성을 해결하려면 종속성을 정기적으로 업데이트하세요. 잠재적인 보안 허점을 식별하고 수정하기 위해 보안 감사 및 침투 테스트를 수행합니다. MERN 스택 애플리케이션의 보안을 보장하는 것은 사용자 신뢰를 유지하고 민감한 데이터를 보호하는 데 매우 중요합니다.
// Example: Implementing JWT Authentication import jwt from 'jsonwebtoken'; function authenticateToken(req, res, next) { const token = req.header('Authorization'); if (!token) return res.status(401).send('Access Denied'); try { const verified = jwt.verify(token, process.env.JWT_SECRET); req.user = verified; next(); } catch (err) { res.status(400).send('Invalid Token'); } }
3. 성능 최적화를 위한 팁
To optimize performance in your MERN stack application, consider the following tips:
- Implement server-side rendering to enhance page load times and improve SEO rankings.
// Example: Implementing SSR with React and Node.js import ReactDOMServer from 'react-dom/server'; import App from './App'; app.get('*', (req, res) => { const html = ReactDOMServer.renderToString(<App />); res.send(`<!DOCTYPE html><html><body>${html}</body></html>`); });
Utilize caching techniques like Redis to store frequently accessed data and reduce latency.
Minify and compress assets to reduce the size of files sent to the client, improving loading speed.
// Example: Using Gzip Compression in Express import compression from 'compression'; app.use(compression());
- Optimize database queries to efficiently retrieve and manipulate data.
// Example: MongoDB Query Optimization db.collection('users').find({ age: { $gte: 21 } }).sort({ age: 1 }).limit(10);
Use performance monitoring tools like New Relic or Datadog to identify bottlenecks and optimize performance.
Employ lazy loading for images and components to decrease initial load times.
// Example: Implementing Lazy Loading in React const LazyComponent = React.lazy(() => import('./LazyComponent')); function App() { return ( <Suspense fallback={<div>Loading...</div>}> <LazyComponent /> </Suspense> ); }
By implementing these performance optimization tips, you can ensure your MERN stack application runs smoothly and efficiently for users.
4. Testing and debugging your MERN stack application
Ensuring the stability and functionality of your MERN stack application is crucial for delivering an exceptional user experience. Prioritize testing by incorporating unit tests, integration tests, and end-to-end testing using tools like Jest, Enzyme, and Mocha. Implement continuous integration and deployment (CI/CD) pipelines to automate testing processes and catch errors early on. Utilize debugging tools like Chrome DevTools to troubleshoot issues and optimize code performance. By dedicating time to testing and debugging, you can identify and resolve potential issues before they impact your users, leading to a more robust and reliable application.
5. Continuous integration and deployment
Implementing a robust continuous integration and deployment (CI/CD) pipeline is essential for streamlining development processes in your MERN stack application. By automating testing, builds, and deployments with tools such as Jenkins or GitLab CI/CD, teams can ensure quicker delivery of features and updates while maintaining code integrity. Integrate version control systems like Git to manage code changes effectively and facilitate collaboration among team members. A well-structured CI/CD pipeline not only enhances productivity but also helps in maintaining the overall quality and reliability of your application. Stay tuned for more insights on optimizing the CI/CD process in our upcoming blogs.
# Example: GitLab CI/CD Pipeline Configuration stages: - build - test - deploy build: script: - npm install - npm run build test: script: - npm run test deploy: script: - npm run deploy
6. Conclusion: Embracing best practices for a successful MERN stack application
In conclusion, implementing a well-structured continuous integration and deployment (CI/CD) pipeline, along with leveraging version control systems like Git, is crucial for ensuring the efficiency and quality of your MERN stack application development. By following best practices and incorporating automation tools such as Jenkins or GitLab CI/CD, teams can accelerate delivery timelines and enhance collaboration among team members. Stay committed to optimizing your CI/CD process and integrating the latest industry standards to achieve a successful MERN stack application that meets user expectations and maintains code integrity. Keep exploring new techniques and stay updated with upcoming blogs for more insightful tips on building robust MERN stack applications.
Congratulations if you've made it to this point of the article.
If you found this article useful, let me know in the comments.?
위 내용은 MERN 스택 애플리케이션 구축 - 모범 사례 및 팁의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
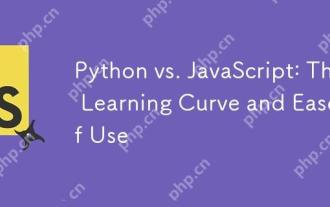
Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
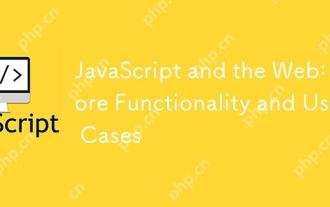
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.
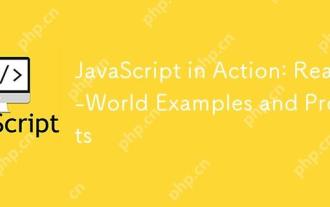
실제 세계에서 JavaScript의 응용 프로그램에는 프론트 엔드 및 백엔드 개발이 포함됩니다. 1) DOM 운영 및 이벤트 처리와 관련된 TODO 목록 응용 프로그램을 구축하여 프론트 엔드 애플리케이션을 표시합니다. 2) Node.js를 통해 RESTFULAPI를 구축하고 Express를 통해 백엔드 응용 프로그램을 시연하십시오.
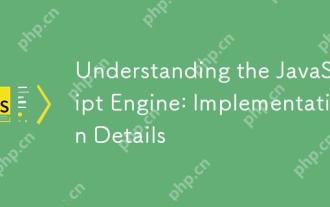
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.
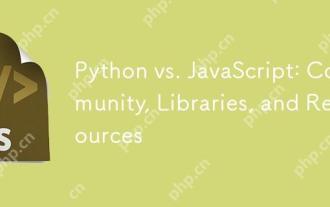
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
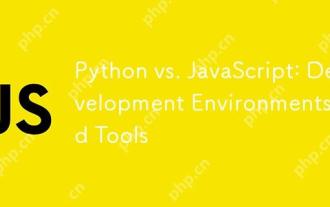
개발 환경에서 Python과 JavaScript의 선택이 모두 중요합니다. 1) Python의 개발 환경에는 Pycharm, Jupyternotebook 및 Anaconda가 포함되어 있으며 데이터 과학 및 빠른 프로토 타이핑에 적합합니다. 2) JavaScript의 개발 환경에는 Node.js, VScode 및 Webpack이 포함되어 있으며 프론트 엔드 및 백엔드 개발에 적합합니다. 프로젝트 요구에 따라 올바른 도구를 선택하면 개발 효율성과 프로젝트 성공률이 향상 될 수 있습니다.
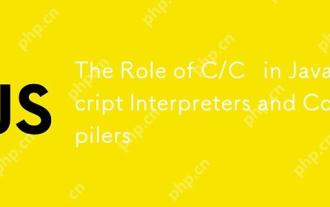
C와 C는 주로 통역사와 JIT 컴파일러를 구현하는 데 사용되는 JavaScript 엔진에서 중요한 역할을합니다. 1) C는 JavaScript 소스 코드를 구문 분석하고 추상 구문 트리를 생성하는 데 사용됩니다. 2) C는 바이트 코드 생성 및 실행을 담당합니다. 3) C는 JIT 컴파일러를 구현하고 런타임에 핫스팟 코드를 최적화하고 컴파일하며 JavaScript의 실행 효율을 크게 향상시킵니다.

Python은 데이터 과학 및 자동화에 더 적합한 반면 JavaScript는 프론트 엔드 및 풀 스택 개발에 더 적합합니다. 1. Python은 데이터 처리 및 모델링을 위해 Numpy 및 Pandas와 같은 라이브러리를 사용하여 데이터 과학 및 기계 학습에서 잘 수행됩니다. 2. 파이썬은 간결하고 자동화 및 스크립팅이 효율적입니다. 3. JavaScript는 프론트 엔드 개발에 없어서는 안될 것이며 동적 웹 페이지 및 단일 페이지 응용 프로그램을 구축하는 데 사용됩니다. 4. JavaScript는 Node.js를 통해 백엔드 개발에 역할을하며 전체 스택 개발을 지원합니다.
