자바의 타이머
Java의 타이머는 Java에서 사용할 수 있습니다. util 패키지는 객체 클래스를 확장하고 직렬화 가능 및 복제 가능 인터페이스를 구현합니다. 타이머 클래스에는 타이밍 관련 활동을 수행하는 데 사용되는 메서드가 포함되어 있습니다. Java의 타이머 클래스는 시간 관련 작업 스케줄링을 수행하는 데 사용됩니다. Java 스레드는 Timer 클래스의 메서드를 사용하여 일정 시간이 지난 후 코드 섹션을 실행하거나 미리 정의된 일정 시간이 지난 후 코드를 반복적으로 실행하는 등의 작업을 예약합니다. 각 Timer 개체는 스레드와 관련된 모든 작업을 실행하는 별도의 백그라운드 실행 스레드에 바인딩됩니다. Java의 타이머 클래스는 스레드로부터 안전합니다. 즉, 한 번에 하나의 스레드만 Timer 클래스 메서드를 실행할 수 있습니다. 또한 Timer 클래스는 작업을 저장하기 위한 기본 데이터 구조로 바이너리 힙을 사용합니다.
무료 소프트웨어 개발 과정 시작
웹 개발, 프로그래밍 언어, 소프트웨어 테스팅 등
Java의 타이머 구문
다음은 Java에서 Timer 클래스를 사용하는 방법에 대한 기본 구문입니다.
구문:
// create a class extending TimerTask class TimerHelper extends TimerTask { //define run method public void run() { // Write Code to be executed by Timer } } class MainClass{ public static void main(String[] args) { //create timer instance Timer timer = new Timer(); // create Timer class instance TimerTask task = new TimerHelper (); // call timer method timer.schedule(task, 3000,6000); //first argument is timer object // second argument is time in milliseconds after which the code will be first executed // Third argument is time in milliseconds after which the code will be executed regularly. } }
위 구문 설명: 구문은 Java에서 Timer 클래스가 사용되는 방식을 보여줍니다. 타이머 클래스를 사용하려면 TimerTask를 확장하는 클래스를 만들고 그 안에 run 메서드를 정의해야 합니다. run 메소드에는 시간에 따라 실행되어야 하는 로직이 포함되어 있습니다. 다음은 Timer 클래스 선언입니다.
public class Timer extends Object implements Serializable, Cloneable
Java의 Timer 클래스 메서드
이제 Java Timer 클래스에서 사용할 수 있는 다양한 메소드와 필드가 무엇인지 살펴보겠습니다. Timer 클래스에서 일반적으로 사용되는 메소드 목록은 다음과 같습니다.
Method Name | Description |
public void schedule(TimerTask task, Date date) | Schedules a task to be executed on the defined date. |
public void schedule (TimerTask task, Date firstTime, long timeperiod) | The first argument is TimerTask to be executed; the second argument is the time after which the task is executed for the first time, and the third argument is seconds in milliseconds after which task will be executed regularly. |
public int purge() | Used for removing all canceled tasks from the timer’s queue. |
public void cancel() | Cancel’s the timer. |
public void schedule(TimeTask task, long delay) | Schedules the task to be executed after the specified time in milliseconds. |
public void schedule(TimeTask task, long delay, long period) | The first argument is TimerTask to be executed; the second argument is the time in milliseconds after which task is executed for the first time, and the third argument is seconds in milliseconds after which task will be executed regularly. |
public void scheduleAtFixedRate(TimerTask task, Date firstTime, long timeperiod) | The first argument is TimerTask to be executed; the second argument is the time after which the task is executed for the first time, and the third argument is seconds in milliseconds after which the task will be executed regularly. |
public void scheduleAtFixedRate (TimeTask task, long delay, long period) | The first argument is TimerTask to be executed; the second argument is the time in milliseconds after which task is executed for the first time, and the third argument is seconds in milliseconds after which task will be executed regularly. |
From the above-stated methods, we have found two methods that are similar in working but different in the name; they are schedule and scheduleAtFixedRate. The difference between the two is that in the case of fixed-rate execution, each execution is scheduled in accordance with the initial execution. If there is a delay in execution, then two or more executions will occur in quick succession to overcome the delay.
Constructors in Timer Class
The timer class contains four constructors for instantiating timer object.
- Timer(): Creates a new Timer Object.
- Timer(boolean isDaemon): Creates a timer object with a corresponding thread specified to run as a daemon.
- Timer(String name): Creates a timer object with a corresponding thread name.
- Timer(String name, boolean isDaemon): This method is a combination of the above two constructors.
One of the above four listed constructors can be called depending on our requirements.
Examples of Implementing Timer in Java
Below is the example of Timer in Java:
Example #1
To start things, let us see a basic example of Timer class. In this example, we will demonstrate the use of the schedule method of the Timer class.
Code:
package com.edubca.timer; import java.util.Timer; import java.util.TimerTask; class TimerHelper extends TimerTask { public static int counter = 0; public void run() { counter++; System.out.println("Timer run Number " + counter); } } public class Main { public static void main(String[] args) { Timer timer = new Timer(); TimerTask timerhelper = new TimerHelper(); timer.schedule(timerhelper, 3000, 2000); } }
Explanation of the above code: The above code will execute the run method for the first time after 3 seconds as the first argument is 3000, and after every 2 seconds, the run method will be executed regularly. Here is the output that will be displayed:
Output:
Example #2
In this example, we will see how to terminate a timer thread after a given number of timer runs.
Code:
package com.edubca.timer; import java.util.Timer; import java.util.TimerTask; class TimerHelper extends TimerTask { public static int counter = 0; public void run() { counter++; if(counter ==3){ this.cancel(); System.out.println("Now Cancelling Thread!!!!!"); return; } System.out.println("Timer run Number " + counter); } } public class Demo { public static void main(String[] args) { Timer timer = new Timer(); TimerTask helper = new TimerHelper(); helper.schedule(task, 3000, 2000); } }
In the above example, the timer will cancel after the three times run method is called using the timer class’s cancel method.
Output:
위 내용은 자바의 타이머의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
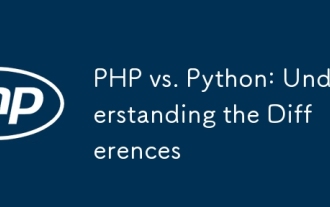
PHP와 Python은 각각 고유 한 장점이 있으며 선택은 프로젝트 요구 사항을 기반으로해야합니다. 1.PHP는 간단한 구문과 높은 실행 효율로 웹 개발에 적합합니다. 2. Python은 간결한 구문 및 풍부한 라이브러리를 갖춘 데이터 과학 및 기계 학습에 적합합니다.
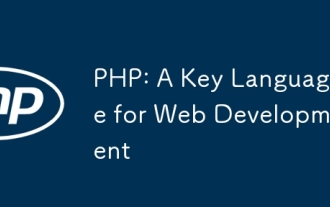
PHP는 서버 측에서 널리 사용되는 스크립팅 언어이며 특히 웹 개발에 적합합니다. 1.PHP는 HTML을 포함하고 HTTP 요청 및 응답을 처리 할 수 있으며 다양한 데이터베이스를 지원할 수 있습니다. 2.PHP는 강력한 커뮤니티 지원 및 오픈 소스 리소스를 통해 동적 웹 컨텐츠, 프로세스 양식 데이터, 액세스 데이터베이스 등을 생성하는 데 사용됩니다. 3. PHP는 해석 된 언어이며, 실행 프로세스에는 어휘 분석, 문법 분석, 편집 및 실행이 포함됩니다. 4. PHP는 사용자 등록 시스템과 같은 고급 응용 프로그램을 위해 MySQL과 결합 할 수 있습니다. 5. PHP를 디버깅 할 때 error_reporting () 및 var_dump ()와 같은 함수를 사용할 수 있습니다. 6. 캐싱 메커니즘을 사용하여 PHP 코드를 최적화하고 데이터베이스 쿼리를 최적화하며 내장 기능을 사용하십시오. 7
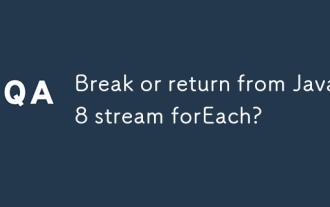
Java 8은 스트림 API를 소개하여 데이터 컬렉션을 처리하는 강력하고 표현적인 방법을 제공합니다. 그러나 스트림을 사용할 때 일반적인 질문은 다음과 같은 것입니다. 기존 루프는 조기 중단 또는 반환을 허용하지만 스트림의 Foreach 메소드는이 방법을 직접 지원하지 않습니다. 이 기사는 이유를 설명하고 스트림 처리 시스템에서 조기 종료를 구현하기위한 대체 방법을 탐색합니다. 추가 읽기 : Java Stream API 개선 스트림 foreach를 이해하십시오 Foreach 메소드는 스트림의 각 요소에서 하나의 작업을 수행하는 터미널 작동입니다. 디자인 의도입니다
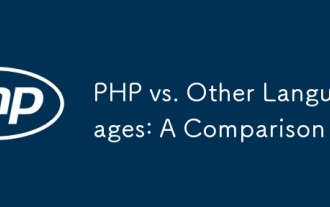
PHP는 특히 빠른 개발 및 동적 컨텐츠를 처리하는 데 웹 개발에 적합하지만 데이터 과학 및 엔터프라이즈 수준의 애플리케이션에는 적합하지 않습니다. Python과 비교할 때 PHP는 웹 개발에 더 많은 장점이 있지만 데이터 과학 분야에서는 Python만큼 좋지 않습니다. Java와 비교할 때 PHP는 엔터프라이즈 레벨 애플리케이션에서 더 나빠지지만 웹 개발에서는 더 유연합니다. JavaScript와 비교할 때 PHP는 백엔드 개발에서 더 간결하지만 프론트 엔드 개발에서는 JavaScript만큼 좋지 않습니다.
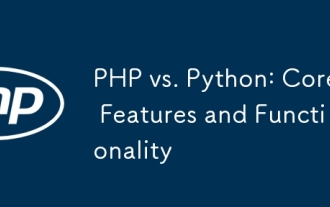
PHP와 Python은 각각 고유 한 장점이 있으며 다양한 시나리오에 적합합니다. 1.PHP는 웹 개발에 적합하며 내장 웹 서버 및 풍부한 기능 라이브러리를 제공합니다. 2. Python은 간결한 구문과 강력한 표준 라이브러리가있는 데이터 과학 및 기계 학습에 적합합니다. 선택할 때 프로젝트 요구 사항에 따라 결정해야합니다.
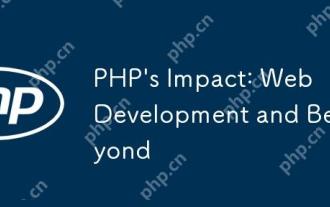
phphassignificallyimpactedwebdevelopmentandextendsbeyondit
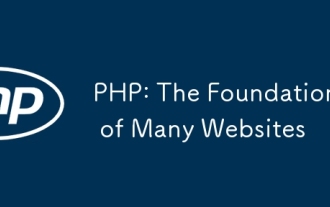
PHP가 많은 웹 사이트에서 선호되는 기술 스택 인 이유에는 사용 편의성, 강력한 커뮤니티 지원 및 광범위한 사용이 포함됩니다. 1) 배우고 사용하기 쉽고 초보자에게 적합합니다. 2) 거대한 개발자 커뮤니티와 풍부한 자원이 있습니다. 3) WordPress, Drupal 및 기타 플랫폼에서 널리 사용됩니다. 4) 웹 서버와 밀접하게 통합하여 개발 배포를 단순화합니다.
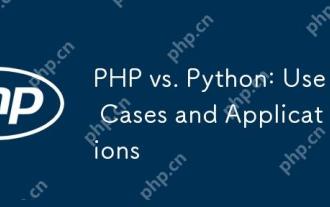
PHP는 웹 개발 및 컨텐츠 관리 시스템에 적합하며 Python은 데이터 과학, 기계 학습 및 자동화 스크립트에 적합합니다. 1.PHP는 빠르고 확장 가능한 웹 사이트 및 응용 프로그램을 구축하는 데 잘 작동하며 WordPress와 같은 CMS에서 일반적으로 사용됩니다. 2. Python은 Numpy 및 Tensorflow와 같은 풍부한 라이브러리를 통해 데이터 과학 및 기계 학습 분야에서 뛰어난 공연을했습니다.
