Java 사용자 입력
Java 프로그램에는 명령줄 환경에서 사용자 입력을 읽어서 사용자 입력을 얻을 수 있는 방법이 3가지 있는데, Java BufferedReader 클래스, Java Scanner 클래스, Console 클래스입니다. 수업에 대해 자세히 알아보겠습니다. 사용자 입력을 얻기 위해 Scanner 클래스를 사용합니다. 이 프로그램은 사용자에게 정수, 문자열, 부동소수점을 입력하도록 요청하고 이를 디스플레이에 인쇄합니다. java.util의 스캐너 클래스가 존재하므로 이 패키지를 소프트웨어에 추가할 수 있습니다. 먼저 Scanner Class 객체를 생성하고 Scanner Class 메소드를 사용합니다.
Java 사용자 입력의 3가지 방법
사용자 입력을 읽는 세 가지 방법이 있습니다.
광고 이 카테고리에서 인기 있는 강좌 JAVA MASTERY - 전문 분야 | 78 코스 시리즈 | 15가지 모의고사무료 소프트웨어 개발 과정 시작
웹 개발, 프로그래밍 언어, 소프트웨어 테스팅 등
- Java BufferedReader 클래스
- 자바 스캐너 수업
- 콘솔 클래스 사용
이 세 클래스는 아래에 언급되어 있습니다. 자세히 논의해 보겠습니다.
1. Java BufferedReader 클래스
독자 클래스를 확장합니다. BufferedReader는 문자 입력 스트림에서 입력을 읽고 모든 입력을 효율적으로 읽을 수 있도록 문자를 버퍼링합니다. 버퍼링을 위한 기본 크기는 큽니다. 사용자가 읽기를 요청하면 해당 요청이 리더기로 이동하고 문자 또는 바이트 스트림에 대한 읽기 요청이 이루어집니다. 따라서 BufferedReader 클래스는 FileReader 또는 InputStreamReaders와 같은 다른 입력 스트림 주위에 래핑됩니다.
예:
BufferedReader reader = new BufferedReader(new FileReader("foo.in"));
BufferedReader는 readLine() 메서드를 사용하여 한 줄씩 데이터를 읽을 수 있습니다.
BufferedReader를 사용하면 코드 성능이 더 빨라질 수 있습니다.
건축자
BufferedReader에는 다음과 같은 두 개의 생성자가 있습니다.
1. BufferedReader(리더 리더): 입력 버퍼의 기본 크기를 사용하는 버퍼링된 입력 문자 스트림을 생성하는 데 사용됩니다.
2. BufferedReader(리더 리더, 입력 크기): 입력 버퍼에 제공된 크기를 사용하는 버퍼링된 입력 문자 스트림을 생성하는 데 사용됩니다.
기능
- int read: 단일 문자를 읽는 데 사용됩니다.
- int read(char[] cbuffer, int offset, int length): 배열의 지정된 부분에 있는 문자를 읽는 데 사용됩니다.
- String readLine (): 입력을 한 줄씩 읽는 데 사용됩니다.
- boolean Ready(): 입력 버퍼를 읽을 준비가 되었는지 테스트하는 데 사용됩니다.
- 긴 건너뛰기: 문자를 건너뛰는 데 사용됩니다.
- void close(): 입력 스트림 버퍼와 스트림과 관련된 시스템 리소스를 닫습니다.
사용자가 키보드에서 문자를 입력하면 장치 버퍼에서 읽은 다음 System.in에서 버퍼 리더 또는 입력 스트림 리더로 전달되어 입력 버퍼에 저장됩니다.
코드:
import java.util.*; import java.lang.*; import java.io.*; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; /*package whatever //do not write package name here */ class BufferedReaderDemo { public static void main (String[] args) throws NumberFormatException, IOException { System.out.println("Enter your number"); BufferedReader br = new BufferedReader(new InputStreamReader(System.in)); int t = Integer.parseInt(br.readLine()); System.out.println("Number you entered is: " + t); System.out.println("Enter your string"); String s = br.readLine(); System.out.println("String you entered is: " + s); } }
출력:
InputStreamReader 및 BufferedReader에서 읽는 프로그램:
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; public class BufferedReaderDemo { public static void main(String args[]) throws IOException{ InputStreamReader reader = new InputStreamReader(System.in); BufferedReader br = new BufferedReader(reader); System.out.println("What is your name?"); String name=br.readLine(); System.out.println("Welcome "+name); } }
출력:
2. 자바 스캐너 클래스
java.util. scanner 클래스는 키보드에서 사용자 입력을 읽는 데 사용되는 클래스 중 하나입니다. util 패키지에서 사용할 수 있습니다. 스캐너 클래스는 기본적으로 대부분 공백인 구분 기호를 사용하여 사용자 입력을 구분합니다. 스캐너에는 double, int, float, long, Boolean, short, byte 등과 같은 다양한 기본 유형의 콘솔 입력을 읽는 여러 가지 방법이 있습니다. 이는 Java에서 입력을 얻는 가장 간단한 방법입니다. Scanner 클래스는 Iterator 및 Closeable 인터페이스를 구현합니다. 스캐너는 기본 유형의 입력을 읽기 위한 nextInt() 및 다양한 기본 유형 메소드를 제공합니다. 문자열 입력에는 next() 메소드가 사용됩니다.
Constructors
- Scanner(File source): It constructs a scanner to read from a specified file.
- Scanner(File source, String charsetName): It constructs a scanner to read from a specified file.
- Scanner(InputStream source), Scanner(InputStream source, String charsetName): It constructs a scanner to read from a specified input stream.
- Scanner(0Readable source): It constructs a scanner to read from a specified readable source.
- Scanner(String source): It constructs a scanner to read from a specified string source.
- Scanner(ReadableByteChannel source): It constructs a scanner to read from a specified channel source.
- Scanner(ReadableByteChannel source, String charsetName): It constructs a scanner to read from a specified channel source.
Functions
Below are mentioned the method to scan the primitive types from console input through Scanner class.
- nextInt(),
- nextFloat(),
- nectDouble(),
- nextLong(),
- nextShort(),
- nextBoolean(),
- nextDouble(),
- nextByte(),
Program to read from Scanner Class:
Using scanner class. import java.util.Scanner; /*package whatever //do not write package name here */ class ScannerDemo { public static void main (String[] args) { Scanner sc = new Scanner(System.in); System.out.println("Enter your number"); int t = sc.nextInt(); System.out.println("Number you entered is: " + t); System.out.println("Enter your string"); String s = sc.next(); System.out.println("String you entered is: " + s); } }
Output:
3. Using console Class
Using the console class to read the input from the command-line interface. It does not work on IDE.
Code:
public class Main { public static void main(String[] args) { // Using Console to input data from user System.out.println("Enter your data"); String name = System.console().readLine(); System.out.println("You entered: "+name); } }
Output:
위 내용은 Java 사용자 입력의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
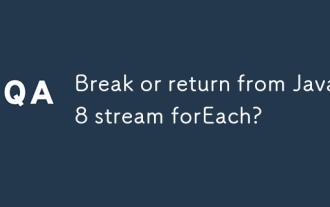
Java 8은 스트림 API를 소개하여 데이터 컬렉션을 처리하는 강력하고 표현적인 방법을 제공합니다. 그러나 스트림을 사용할 때 일반적인 질문은 다음과 같은 것입니다. 기존 루프는 조기 중단 또는 반환을 허용하지만 스트림의 Foreach 메소드는이 방법을 직접 지원하지 않습니다. 이 기사는 이유를 설명하고 스트림 처리 시스템에서 조기 종료를 구현하기위한 대체 방법을 탐색합니다. 추가 읽기 : Java Stream API 개선 스트림 foreach를 이해하십시오 Foreach 메소드는 스트림의 각 요소에서 하나의 작업을 수행하는 터미널 작동입니다. 디자인 의도입니다
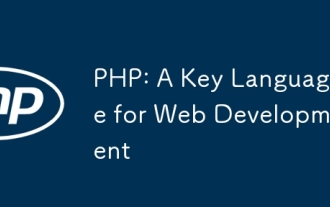
PHP는 서버 측에서 널리 사용되는 스크립팅 언어이며 특히 웹 개발에 적합합니다. 1.PHP는 HTML을 포함하고 HTTP 요청 및 응답을 처리 할 수 있으며 다양한 데이터베이스를 지원할 수 있습니다. 2.PHP는 강력한 커뮤니티 지원 및 오픈 소스 리소스를 통해 동적 웹 컨텐츠, 프로세스 양식 데이터, 액세스 데이터베이스 등을 생성하는 데 사용됩니다. 3. PHP는 해석 된 언어이며, 실행 프로세스에는 어휘 분석, 문법 분석, 편집 및 실행이 포함됩니다. 4. PHP는 사용자 등록 시스템과 같은 고급 응용 프로그램을 위해 MySQL과 결합 할 수 있습니다. 5. PHP를 디버깅 할 때 error_reporting () 및 var_dump ()와 같은 함수를 사용할 수 있습니다. 6. 캐싱 메커니즘을 사용하여 PHP 코드를 최적화하고 데이터베이스 쿼리를 최적화하며 내장 기능을 사용하십시오. 7
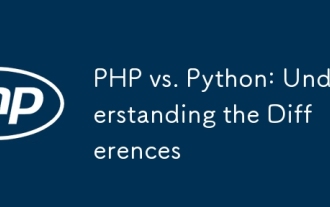
PHP와 Python은 각각 고유 한 장점이 있으며 선택은 프로젝트 요구 사항을 기반으로해야합니다. 1.PHP는 간단한 구문과 높은 실행 효율로 웹 개발에 적합합니다. 2. Python은 간결한 구문 및 풍부한 라이브러리를 갖춘 데이터 과학 및 기계 학습에 적합합니다.
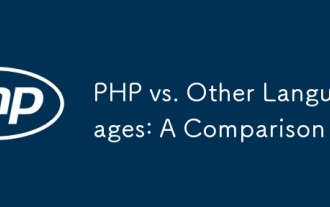
PHP는 특히 빠른 개발 및 동적 컨텐츠를 처리하는 데 웹 개발에 적합하지만 데이터 과학 및 엔터프라이즈 수준의 애플리케이션에는 적합하지 않습니다. Python과 비교할 때 PHP는 웹 개발에 더 많은 장점이 있지만 데이터 과학 분야에서는 Python만큼 좋지 않습니다. Java와 비교할 때 PHP는 엔터프라이즈 레벨 애플리케이션에서 더 나빠지지만 웹 개발에서는 더 유연합니다. JavaScript와 비교할 때 PHP는 백엔드 개발에서 더 간결하지만 프론트 엔드 개발에서는 JavaScript만큼 좋지 않습니다.
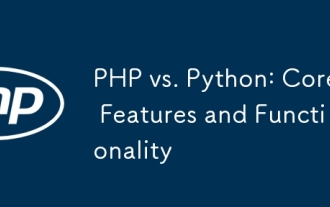
PHP와 Python은 각각 고유 한 장점이 있으며 다양한 시나리오에 적합합니다. 1.PHP는 웹 개발에 적합하며 내장 웹 서버 및 풍부한 기능 라이브러리를 제공합니다. 2. Python은 간결한 구문과 강력한 표준 라이브러리가있는 데이터 과학 및 기계 학습에 적합합니다. 선택할 때 프로젝트 요구 사항에 따라 결정해야합니다.
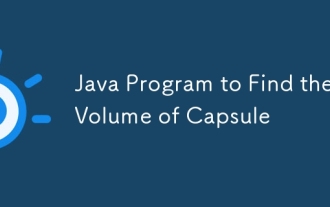
캡슐은 3 차원 기하학적 그림이며, 양쪽 끝에 실린더와 반구로 구성됩니다. 캡슐의 부피는 실린더의 부피와 양쪽 끝에 반구의 부피를 첨가하여 계산할 수 있습니다. 이 튜토리얼은 다른 방법을 사용하여 Java에서 주어진 캡슐의 부피를 계산하는 방법에 대해 논의합니다. 캡슐 볼륨 공식 캡슐 볼륨에 대한 공식은 다음과 같습니다. 캡슐 부피 = 원통형 볼륨 2 반구 볼륨 안에, R : 반구의 반경. H : 실린더의 높이 (반구 제외). 예 1 입력하다 반경 = 5 단위 높이 = 10 단위 산출 볼륨 = 1570.8 입방 단위 설명하다 공식을 사용하여 볼륨 계산 : 부피 = π × r2 × h (4
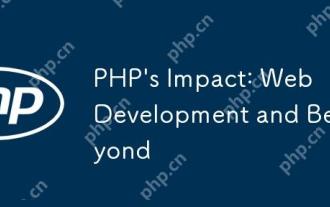
phphassignificallyimpactedwebdevelopmentandextendsbeyondit
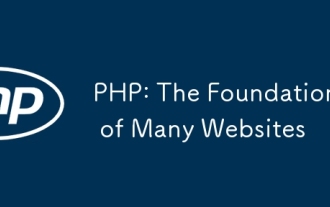
PHP가 많은 웹 사이트에서 선호되는 기술 스택 인 이유에는 사용 편의성, 강력한 커뮤니티 지원 및 광범위한 사용이 포함됩니다. 1) 배우고 사용하기 쉽고 초보자에게 적합합니다. 2) 거대한 개발자 커뮤니티와 풍부한 자원이 있습니다. 3) WordPress, Drupal 및 기타 플랫폼에서 널리 사용됩니다. 4) 웹 서버와 밀접하게 통합하여 개발 배포를 단순화합니다.
