JVM의 가상 스레드 메커니즘에서 고정 탐색
Java's virtual threads offer a lightweight alternative to traditional OS threads, enabling efficient concurrency management. But understanding their behavior is crucial for optimal performance. This blog post dives into pinning, a scenario that can impact virtual thread execution, and explores techniques to monitor and address it.
Virtual Threads: A Lightweight Concurrency Approach
Java's virtual threads are managed entities that run on top of the underlying operating system threads (carrier threads). They provide a more efficient way to handle concurrency compared to creating numerous OS threads, as they incur lower overhead. The JVM maps virtual threads to carrier threads dynamically, allowing for better resource utilization.
Managed by the JVM: Unlike OS threads that are directly managed by the operating system, virtual threads are created and scheduled by the Java Virtual Machine (JVM). This allows for finer-grained control and optimization within the JVM environment.
Reduced Overhead: Creating and managing virtual threads incurs significantly lower overhead compared to OS threads. This is because the JVM can manage a larger pool of virtual threads efficiently, utilizing a smaller number of underlying OS threads.
Compatibility with Existing Code: Virtual threads are designed to be seamlessly integrated with existing Java code. They can be used alongside traditional OS threads and work within the familiar constructs like Executor and ExecutorService for managing concurrent.
The figure below shows the relationship between virtual threads and platform threads:
Pinning: When a Virtual Thread Gets Stuck
Pinning occurs when a virtual thread becomes tied to its carrier thread. This essentially means the virtual thread cannot be preempted (switched to another carrier thread) while it's in a pinned state. Here are common scenarios that trigger pinning:
- Synchronized Blocks and Methods: Executing code within a synchronized block or method leads to pinning. This ensures exclusive access to shared resources, preventing data corruption issues.
Code Example:
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class Main { public static void main(String[] args) throws InterruptedException { final Counter counter = new Counter(); Runnable task = () -> { for (int i = 0; i < 1000; i++) { counter.increment(); } }; Thread thread1 = new Thread(task); Thread thread2 = new Thread(task); thread1.start(); thread2.start(); thread1.join(); thread2.join(); System.out.println("Final counter value: " + counter.getCount()); } } class Counter { private int count = 0; public synchronized void increment() { count++; } public synchronized int getCount() { return count; } }
In this example, when a virtual thread enters the synchronized block, it becomes pinned to its carrier thread, but this is not always true. Java's synchronized keyword alone is not enough to cause thread pinning in virtual threads. For thread pinning to occur, there must be a blocking point within a synchronized block that causes a virtual thread to trigger park, and ultimately disallows unmounting from its carrier thread. Thread pinning could cause a decrease in performance as it would negate the benefits of using lightweight/virtual threads.
Whenever a virtual thread encounters a blocking point, its state is transitioned to PARKING. This state transition is indicated by invoking the VirtualThread.park() method:
// JDK core code void park() { assert Thread.currentThread() == this; // complete immediately if parking permit available or interrupted if (getAndSetParkPermit(false) || interrupted) return; // park the thread setState(PARKING); try { if (!yieldContinuation()) { // park on the carrier thread when pinned parkOnCarrierThread(false, 0); } } finally { assert (Thread.currentThread() == this) && (state() == RUNNING); } }
Let's take a look at a code sample to illustrate this concept:
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class Main { public static void main(String[] args) { Counter counter = new Counter(); Runnable task = () -> { for (int i = 0; i < 100; i++) { counter.increment(); } }; Thread thread1 = Thread.ofVirtual().start(task); Thread thread2 = Thread.ofVirtual().start(task); try { thread1.join(); thread2.join(); } catch (InterruptedException e) { throw new RuntimeException(e); } System.out.println("Final counter value: " + counter.getCount()); } } class Counter { private int count = 0; public void increment() { synchronized (this) { try { Thread.sleep(100); // This simulates a blocking call within the synchronized block } catch (InterruptedException e) { e.printStackTrace(); } count++; } } public synchronized int getCount() { return count; } }
- Native Methods/Foreign Functions: Running native methods or foreign functions can also cause pinning. The JVM might not be able to efficiently manage the virtual thread's state during these operations.
Monitoring Pinning with -Djdk.tracePinnedThreads=full
The -Djdk.tracePinnedThreads=full flag is a JVM startup argument that provides detailed tracing information about virtual thread pinning. When enabled, it logs events like:
- Virtual thread ID involved in pinning
- Carrier thread ID to which the virtual thread is pinned
- Stack trace indicating the code section causing pinning
Use this flag judiciously during debugging sessions only, as it introduces performance overhead.
-
Compile the our demo code:
javac Main.java
로그인 후 복사 -
Start the compiled code with the -Djdk.tracePinnedThreads=full flag:
java -Djdk.tracePinnedThreads=full Main
로그인 후 복사 -
Observe the output in the console, which shows detailed information about virtual thread pinning:
Thread[#29,ForkJoinPool-1-worker-1,5,CarrierThreads] java.base/java.lang.VirtualThread$VThreadContinuation.onPinned(VirtualThread.java:183) java.base/jdk.internal.vm.Continuation.onPinned0(Continuation.java:393) java.base/java.lang.VirtualThread.parkNanos(VirtualThread.java:621) java.base/java.lang.VirtualThread.sleepNanos(VirtualThread.java:791) java.base/java.lang.Thread.sleep(Thread.java:507) Counter.increment(Main.java:38) <== monitors:1 Main.lambda$main\$0(Main.java:13) java.base/java.lang.VirtualThread.run(VirtualThread.java:309) Final counter value: 200
로그인 후 복사
Fixing Pinning with Reentrant Locks
Pinning is an undesirable scenario which impedes the performance of virtual threads. Reentrant locks serve as an effective tool to counteract pinning. Here's how you can use Reentrant locks to mitigate pinning situations:
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; import java.util.concurrent.locks.ReentrantLock; public class Main { public static void main(String[] args) { Counter counter = new Counter(); Runnable task = () -> { for (int i = 0; i < 100; i++) { counter.increment(); } }; Thread thread1 = Thread.ofVirtual().start(task); Thread thread2 = Thread.ofVirtual().start(task); try { thread1.join(); thread2.join(); } catch (InterruptedException e) { throw new RuntimeException(e); } System.out.println("Final counter value: " + counter.getCount()); } } class Counter { private int count = 0; private final ReentrantLock lock = new ReentrantLock(); public void increment() { lock.lock(); try { Thread.sleep(100); // This simulates a blocking call count++; } catch (InterruptedException e) { e.printStackTrace(); } finally { lock.unlock(); } } public int getCount() { return count; } }
In the updated example, we use a ReentrantLock instead of a synchronized block. The thread can acquire the lock and release it immediately after it completes its operation, potentially reducing the duration of pinning compared to a synchronized block which might hold the lock for a longer period.
결론적으로
Java의 가상 스레드는 언어의 진화와 기능에 대한 증거입니다. 이는 기존 OS 스레드에 대한 새롭고 가벼운 대안을 제공하여 효율적인 동시성 관리를 위한 다리를 제공합니다. 스레드 고정과 같은 핵심 개념을 깊이 파고들고 이해하는 데 시간을 투자하면 개발자는 이러한 경량 스레드의 잠재력을 최대한 활용할 수 있는 노하우를 얻을 수 있습니다. 이러한 지식은 개발자가 향후 기능을 활용할 수 있도록 준비할 뿐만 아니라 현재 프로젝트에서 복잡한 동시성 제어 문제를 보다 효과적으로 해결할 수 있도록 지원합니다.
위 내용은 JVM의 가상 스레드 메커니즘에서 고정 탐색의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
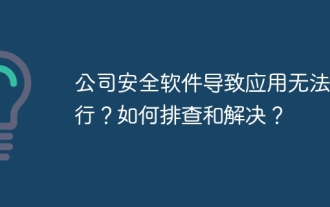
일부 애플리케이션이 제대로 작동하지 않는 회사의 보안 소프트웨어에 대한 문제 해결 및 솔루션. 많은 회사들이 내부 네트워크 보안을 보장하기 위해 보안 소프트웨어를 배포 할 것입니다. ...
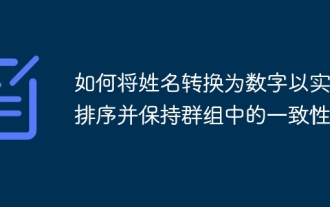
많은 응용 프로그램 시나리오에서 정렬을 구현하기 위해 이름으로 이름을 변환하는 솔루션, 사용자는 그룹으로, 특히 하나로 분류해야 할 수도 있습니다.
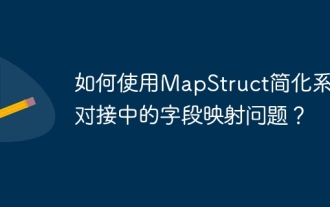
시스템 도킹의 필드 매핑 처리 시스템 도킹을 수행 할 때 어려운 문제가 발생합니다. 시스템의 인터페이스 필드를 효과적으로 매핑하는 방법 ...
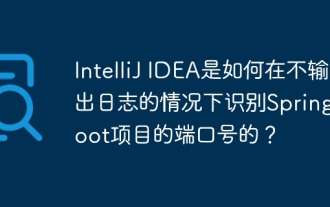
IntellijideAultimate 버전을 사용하여 봄을 시작하십시오 ...
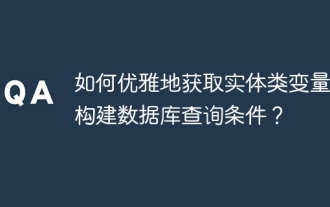
데이터베이스 작업에 MyBatis-Plus 또는 기타 ORM 프레임 워크를 사용하는 경우 엔티티 클래스의 속성 이름을 기반으로 쿼리 조건을 구성해야합니다. 매번 수동으로 ...
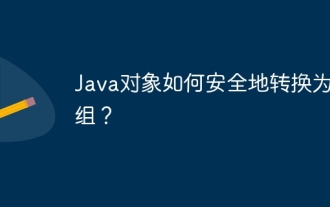
Java 객체 및 배열의 변환 : 캐스트 유형 변환의 위험과 올바른 방법에 대한 심층적 인 논의 많은 Java 초보자가 객체를 배열로 변환 할 것입니다 ...
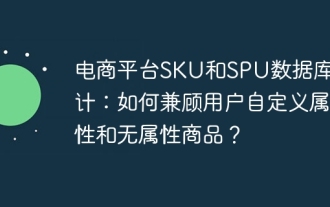
전자 상거래 플랫폼에서 SKU 및 SPU 테이블의 디자인에 대한 자세한 설명이 기사는 전자 상거래 플랫폼에서 SKU 및 SPU의 데이터베이스 설계 문제, 특히 사용자 정의 판매를 처리하는 방법에 대해 논의 할 것입니다 ...
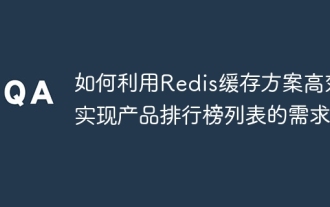
Redis 캐싱 솔루션은 제품 순위 목록의 요구 사항을 어떻게 인식합니까? 개발 과정에서 우리는 종종 a ... 표시와 같은 순위의 요구 사항을 처리해야합니다.
