개발 원칙
프로그래밍은 지속적인 개선의 여정입니다. 경험을 쌓으면서 우리는 기술을 개선하고 더 높은 품질의 코드를 만드는 데 도움이 되는 관행과 원칙을 접하게 됩니다. 이 기사에서는 SOLID 원칙, 디자인 패턴 및 코딩 표준을 포함하여 더 나은 프로그래머가 되는 데 도움이 될 수 있는 주요 원칙과 사례를 안내합니다. 이러한 개념이 개발 프로세스를 어떻게 개선할 수 있는지 살펴보겠습니다.
예제에 대한 참고 사항: 단순성과 가독성 때문에 코드 예제로 Go를 선택했습니다. Go는 종종 "실행 가능한 의사 코드"로 설명됩니다. Go에 익숙하지 않더라도 걱정하지 마세요! 배울 수 있는 절호의 기회입니다.
익숙한 구문이나 개념이 나타나면 잠시 시간을 내어 찾아보세요. 이러한 발견 과정은 학습 경험에 재미있는 보너스가 될 수 있습니다. 우리가 논의하는 원칙은 프로그래밍 언어 전반에 걸쳐 적용되므로 Go 구문의 구체적인 내용보다는 개념을 이해하는 데 집중하세요.
1. 프로그래밍 표준 수용
더 나은 프로그래머가 되기 위한 첫 번째 단계 중 하나는 프로그래밍 표준을 준수하는 것입니다. 표준은 명명 규칙, 코드 구성 및 파일 구조와 같은 측면에 대한 지침을 제공합니다. 이러한 규칙을 따르면 코드가 일관되고 읽기 쉬워지는데, 이는 공동작업과 장기적인 유지 관리에 매우 중요합니다.
각 프로그래밍 언어에는 일반적으로 고유한 규칙이 있습니다. Go의 경우 공식 Go 스타일 가이드에 설명되어 있습니다. 팀의 규칙이든 언어별 지침이든 상황에 맞는 표준을 배우고 수용하는 것이 중요합니다.
다음 표준이 어떻게 코드 가독성을 향상시킬 수 있는지 예를 살펴보겠습니다.
// Before: Inconsistent styling func dosomething(x int,y string)string{ if x>10{ return y+"is big" }else{ return y+"is small" }}
이 코드에는 몇 가지 문제가 있습니다.
- 일관되지 않은 간격과 들여쓰기로 인해 코드 구조를 이해하기 어렵습니다.
- 함수 이름이 Go의 camelCase 규칙을 따르지 않습니다.
- 불필요한 else 절은 인지적 복잡성을 더합니다.
이제 Go 표준을 따르도록 이 코드를 리팩토링해 보겠습니다.
// After: Following Go standards func doSomething(x int, y string) string { if x > 10 { return y + " is big" } return y + " is small" }
이 개선된 버전에서는:
- 적절한 들여쓰기는 코드의 구조를 명확하게 보여줍니다.
- 함수 이름은 Go의 명명 규칙을 따릅니다.
- else 절이 제거되어 논리가 단순화되었습니다.
이러한 변화는 단지 미적인 측면에만 국한되지 않습니다. 코드 가독성과 유지 관리성이 크게 향상됩니다. 팀으로 작업할 때 이러한 표준을 일관되게 적용하면 모든 사람이 코드베이스를 더 쉽게 이해하고 사용할 수 있습니다.
팀에 확립된 표준이 없더라도 프로그래밍 커뮤니티에서 널리 통용되는 규칙을 솔선적으로 따를 수 있습니다. 시간이 지남에 따라 이 방법을 사용하면 프로젝트 전체에서 더 읽기 쉽고 유지 관리하기 쉬운 코드가 만들어질 것입니다.
2. 디자인 원칙을 따르세요
프로그래밍 디자인 원칙은 더 나은 코드를 작성하는 데 도움이 되는 지침입니다. 이러한 원칙은 코드 아키텍처뿐만 아니라 시스템 설계, 심지어 개발 프로세스의 일부 측면에도 적용될 수 있습니다.
많은 디자인 원칙이 있으며 일부는 특정 상황에 더 관련이 있습니다. 다른 것들은 KISS(Keep It Simple, Stupid) 또는 YAGNI(You Ain't Gonna Need It)와 같이 좀 더 일반적입니다.
이러한 일반 원칙 중에서 SOLID 원칙이 가장 영향력이 큽니다. 코드를 개선할 수 있는 방법에 중점을 두고 각 원칙을 살펴보겠습니다.
단일 책임 원칙(SRP)
이 원칙은 잘 정의된 단일 목적으로 구성 요소(함수, 클래스 또는 모듈)를 설계하도록 권장합니다. 구성 요소에 여러 가지 책임이 있으면 이해, 테스트 및 유지 관리가 더 어려워집니다.
SRP를 준수하기 위해 함수를 리팩토링하는 예를 살펴보겠습니다.
// Before: A function doing too much func processOrder(order Order) error { // Validate order if order.Total <= 0 { return errors.New("invalid order total") } // Save to database db.Save(order) // Send confirmation email sendEmail(order.CustomerEmail, "Order Confirmation", orderDetails(order)) // Update inventory for _, item := range order.Items { updateInventory(item.ID, item.Quantity) } return nil }
이 기능은 주문 확인, 데이터베이스에 저장, 이메일 보내기, 재고 업데이트 등 서로 관련되지 않은 여러 작업을 수행합니다. 각각 하나의 책임을 맡은 별도의 기능으로 나누어 보겠습니다.
// After: Breaking it down into single responsibilities func processOrder(order Order) error { if err := validateOrder(order); err != nil { return err } if err := saveOrder(order); err != nil { return err } if err := sendOrderConfirmation(order); err != nil { return err } return updateInventoryForOrder(order) }
이제 다음 기능을 각각 구현해 보겠습니다.
func validateOrder(order Order) error { if order.Total <= 0 { return errors.New("invalid order total") } return nil } func saveOrder(order Order) error { return db.Save(order) } func sendOrderConfirmation(order Order) error { return sendEmail(order.CustomerEmail, "Order Confirmation", orderDetails(order)) } func updateInventoryForOrder(order Order) error { for _, item := range order.Items { if err := updateInventory(item.ID, item.Quantity); err != nil { return err } } return nil }
이 리팩터링 버전에서는:
- 각 기능에는 하나의 명확한 책임이 있습니다.
- 코드가 모듈화되어 테스트하기가 더 쉽습니다.
- 개별 구성요소는 다른 구성요소에 영향을 주지 않고 재사용하거나 수정할 수 있습니다.
저를 믿으십시오. 미래의 당신은 이 정도의 조직에 감사할 것입니다.
개방-폐쇄 원칙(OCP)
개방-폐쇄 원칙은 소프트웨어 엔터티가 확장에는 개방되고 수정에는 폐쇄되어야 한다고 조언합니다. 즉, 기존 코드를 변경하지 않고도 새로운 기능을 추가할 수 있어야 합니다.
Liskov Substitution Principle (LSP)
LSP states that objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program. This ensures that inheritance hierarchies are well-designed and maintainable.
Interface Segregation Principle (ISP)
ISP suggests that no code should be forced to depend on methods it does not use. In practice, this often means creating smaller, more focused interfaces.This modularity makes your code easier to manage and test.
Here's an example demonstrating ISP:
// Before: A large interface that many structs only partially implement type Worker interface { DoWork() TakeBreak() GetPaid() FileTicket() }
This large interface forces implementers to define methods they might not need. Let's break it down into smaller, more focused interfaces:
// After: Smaller, more focused interfaces type Worker interface { DoWork() } type BreakTaker interface { TakeBreak() } type Payable interface { GetPaid() } type TicketFiler interface { FileTicket() }
Now, structs can implement only the interfaces they need:
type Developer struct{} func (d Developer) DoWork() { fmt.Println("Writing code") } func (d Developer) TakeBreak() { fmt.Println("Browsing Reddit") } func (d Developer) FileTicket() { fmt.Println("Creating a Jira ticket") } type Contractor struct{} func (c Contractor) DoWork() { fmt.Println("Completing assigned task") } func (c Contractor) GetPaid() { fmt.Println("Invoicing for work done") }
This modularity makes your code easier to manage and test. In Go, interfaces are implicitly implemented, which makes this principle particularly easy to apply.
Dependency Inversion Principle (DIP)
DIP promotes the use of abstractions rather than concrete implementations. By depending on interfaces or abstract classes, you decouple your code, making it more flexible and easier to maintain. This also facilitates easier testing by allowing mock implementations.
Applying the SOLID (see what I did there?) principles leads to decoupled, modular code that is easier to maintain, scale, reuse, and test. I’ve found that these principles, while sometimes challenging to apply at first, have consistently led to more robust and flexible codebases.
While these principles are valuable, remember that they are guidelines, not strict rules.There will always be exceptions to the rules, but it’s important to remember that following these principles is a continuous process and not a one-time event. It will take time and effort to develop good habits, but the rewards are well worth it.
3. Utilize Design Patterns
Design patterns provide reusable solutions to common programming problems. They are not rigid implementations but rather templates that can be adapted to fit specific needs. Many design patterns are related to SOLID principles, often aiming to uphold one or more of these principles in their design.
Design patterns are typically categorized into three types:
Creational Patterns
These patterns deal with object creation mechanisms. An example is the Factory Method pattern, which creates objects based on a set of criteria while abstracting the instantiation logic.
Let's look at a simple Factory Method example in Go:
type PaymentMethod interface { Pay(amount float64) string } type CashPayment struct{} func (c CashPayment) Pay(amount float64) string { return fmt.Sprintf("Paid %.2f using cash", amount) } type CreditCardPayment struct{} func (cc CreditCardPayment) Pay(amount float64) string { return fmt.Sprintf("Paid %.2f using credit card", amount) }
Here, we define a PaymentMethod interface and two concrete implementations. Now, let's create a factory function:
func GetPaymentMethod(method string) (PaymentMethod, error) { switch method { case "cash": return CashPayment{}, nil case "credit": return CreditCardPayment{}, nil default: return nil, fmt.Errorf("Payment method %s not supported", method) } }
This factory function creates the appropriate payment method based on the input string. Here's how you might use it:
method, err := GetPaymentMethod("cash") if err != nil { fmt.Println(err) return } fmt.Println(method.Pay(42.42))
This pattern allows for easy extension of payment methods without modifying existing code.
Structural Patterns
Structural patterns deal with object composition, promoting better interaction between classes. The Adapter pattern, for example, allows incompatible interfaces to work together.
Behavioral Patterns
Behavioral patterns focus on communication between objects. The Observer pattern is a common behavioral pattern that facilitates a publish-subscribe model, enabling objects to react to events.
It's important to note that there are many more design patterns, and some are more relevant in specific contexts. For example, game development might heavily use the Object Pool pattern, while it's less common in web development.
Design patterns help solve recurring problems and create a universal vocabulary among developers. However, don't feel pressured to learn all patterns at once. Instead, familiarize yourself with the concepts, and when facing a new problem, consider reviewing relevant patterns that might offer a solution. Over time, you'll naturally incorporate these patterns into your design process.
4. Practice Good Naming Conventions
Clear naming conventions are crucial for writing readable and maintainable code. This practice is closely related to programming standards and deserves special attention.
Use Descriptive Names
Choose names that clearly describe the purpose of the variable, function, or class. Avoid unnecessary encodings or cryptic abbreviations.
Consider this poorly named function:
// Bad func calc(a, b int) int { return a + b }
Now, let's improve it with more descriptive names:
// Good func calculateSum(firstNumber, secondNumber int) int { return firstNumber + secondNumber }
However, be cautious not to go to the extreme with overly long names:
// Too verbose func calculateSumOfTwoIntegersAndReturnTheResult(firstInteger, secondInteger int) int { return firstInteger + secondInteger }
Balance Clarity and Conciseness
Aim for names that are clear but not overly verbose. Good naming practices make your code self-documenting, reducing the need for excessive comments.
Prefer Good Names to Comments
Often, the need for comments arises from poorly named elements in your code. If you find yourself writing a comment to explain what a piece of code does, consider whether you could rename variables or functions to make the code self-explanatory.
Replace Magic Values with Named Constants
Using named constants instead of hard-coded values clarifies their meaning and helps keep your code consistent.
// Bad if user.Age >= 18 { // Allow access } // Good const LegalAge = 18 if user.Age >= LegalAge { // Allow access }
By following these naming conventions, you'll create code that's easier to read, understand, and maintain.
5. Prioritize Testing
Testing is an essential practice for ensuring that your code behaves as expected. While there are many established opinions on testing methodologies, it's okay to develop an approach that works best for you and your team.
Unit Testing
Unit tests focus on individual modules in isolation. They provide quick feedback on whether specific parts of your code are functioning correctly.
Here's a simple example of a unit test in Go:
func TestCalculateSum(t *testing.T) { result := calculateSum(3, 4) expected := 7 if result != expected { t.Errorf("calculateSum(3, 4) = %d; want %d", result, expected) } }
Integration Testing
Integration tests examine how different modules work together. They help identify issues that might arise from interactions between various parts of the code.
End-to-End Testing
End-to-end tests simulate user interactions with the entire application. They validate that the system works as a whole, providing a user-centric view of functionality.
Remember, well-tested code is not only more reliable but also easier to refactor and maintain over time. The SOLID principles we discussed earlier can make testing easier by encouraging modular, decoupled code that is simpler to isolate and validate.
6. Take Your Time to Plan and Execute
While it might be tempting to rush through projects, especially when deadlines are tight, thoughtful planning is crucial for long-term success. Rushing can lead to technical debt and future maintenance challenges.
Take the time to carefully consider architectural decisions and plan your approach. Building a solid foundation early on will save time and effort in the long run. However, be cautious not to over-plan—find a balance that works for your project's needs.
Different projects may require varying levels of planning. A small prototype might need minimal upfront design, while a large, complex system could benefit from more extensive planning. The key is to be flexible and adjust your approach based on the project's requirements and constraints.
Conclusion
By following these principles and practices, you can become a better programmer. Focus on writing code that is clear, maintainable, and thoughtfully structured. Remember the words of Martin Fowler: "Any fool can write code that a computer can understand. Good programmers write code that humans can understand."
Becoming a better programmer is a continuous process. Don't be discouraged if you don't get everything right immediately. Keep learning, keep practicing, and most importantly, keep coding. With time and dedication, you'll see significant improvements in your skills and the quality of your work.
Here are some resources you might find helpful for further learning:
- For general programming principles: Programming Principles
- For understanding the importance of basics: The Basics
- For a deep dive into software design: The Philosophy of Software Design and its discussion
- For practical programming advice: The Pragmatic Programmer
- For improving existing code: Refactoring
Now, armed with these principles, start applying them to your projects. Consider creating a small web API that goes beyond simple CRUD operations, or develop a tool that solves a problem you face in your daily work. Remember, the best way to learn is by doing. Happy coding!
위 내용은 개발 원칙의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
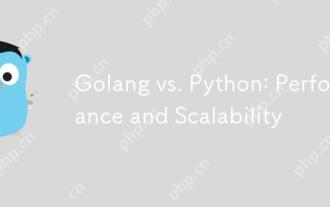
Golang은 성능과 확장 성 측면에서 Python보다 낫습니다. 1) Golang의 컴파일 유형 특성과 효율적인 동시성 모델은 높은 동시성 시나리오에서 잘 수행합니다. 2) 해석 된 언어로서 파이썬은 천천히 실행되지만 Cython과 같은 도구를 통해 성능을 최적화 할 수 있습니다.
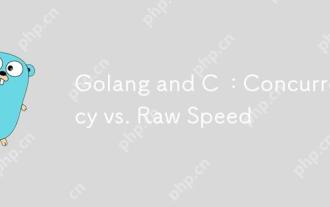
Golang은 동시성에서 C보다 낫고 C는 원시 속도에서 Golang보다 낫습니다. 1) Golang은 Goroutine 및 Channel을 통해 효율적인 동시성을 달성하며, 이는 많은 동시 작업을 처리하는 데 적합합니다. 2) C 컴파일러 최적화 및 표준 라이브러리를 통해 하드웨어에 가까운 고성능을 제공하며 극도의 최적화가 필요한 애플리케이션에 적합합니다.
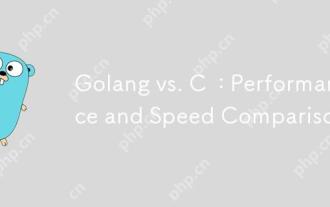
Golang은 빠른 개발 및 동시 시나리오에 적합하며 C는 극도의 성능 및 저수준 제어가 필요한 시나리오에 적합합니다. 1) Golang은 쓰레기 수집 및 동시성 메커니즘을 통해 성능을 향상시키고, 고전성 웹 서비스 개발에 적합합니다. 2) C는 수동 메모리 관리 및 컴파일러 최적화를 통해 궁극적 인 성능을 달성하며 임베디드 시스템 개발에 적합합니다.
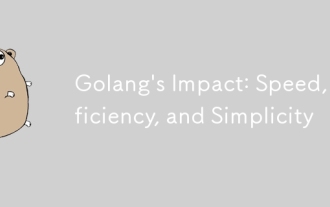
goimpactsdevelopmentpositively throughlyspeed, 효율성 및 단순성.
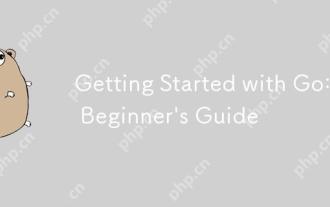
goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity, 효율성, 및 콘크리 론 피처
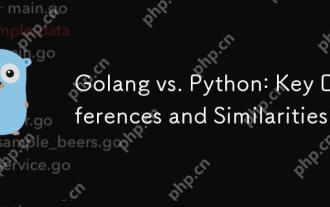
Golang과 Python은 각각 고유 한 장점이 있습니다. Golang은 고성능 및 동시 프로그래밍에 적합하지만 Python은 데이터 과학 및 웹 개발에 적합합니다. Golang은 동시성 모델과 효율적인 성능으로 유명하며 Python은 간결한 구문 및 풍부한 라이브러리 생태계로 유명합니다.
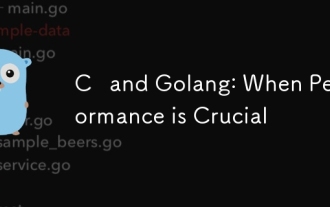
C는 하드웨어 리소스 및 고성능 최적화가 직접 제어되는 시나리오에 더 적합하지만 Golang은 빠른 개발 및 높은 동시성 처리가 필요한 시나리오에 더 적합합니다. 1.C의 장점은 게임 개발과 같은 고성능 요구에 적합한 하드웨어 특성 및 높은 최적화 기능에 가깝습니다. 2. Golang의 장점은 간결한 구문 및 자연 동시성 지원에 있으며, 이는 동시성 서비스 개발에 적합합니다.
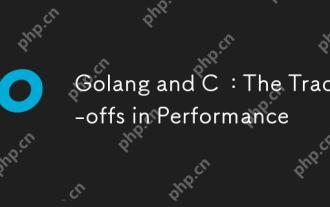
Golang과 C의 성능 차이는 주로 메모리 관리, 컴파일 최적화 및 런타임 효율에 반영됩니다. 1) Golang의 쓰레기 수집 메커니즘은 편리하지만 성능에 영향을 줄 수 있습니다. 2) C의 수동 메모리 관리 및 컴파일러 최적화는 재귀 컴퓨팅에서 더 효율적입니다.
