C#의 TextReader
C#의 TextReader는 텍스트 파일에서 텍스트나 일련의 문자를 읽는 데 사용됩니다. TextReader 클래스는 System.IO 네임스페이스 아래에 있습니다. 스트림과 문자열에서 각각 문자를 읽는 데 사용되는 StreamReader 및 StringReader의 추상 기본 클래스입니다. TextReader는 추상 클래스이기 때문에 객체를 생성할 수 없습니다. TextReader는 기본적으로 스레드로부터 안전하지 않습니다. TextReader 클래스를 파생하는 클래스는 유용한 TextReader 인스턴스를 만들기 위해 Peek() 및 Read() 메서드를 최소한으로 구현해야 합니다.
구문:
TextReader 생성 구문은 다음과 같습니다.
TextReader text_reader = File.OpenText(file_path);
위 명령문은 'file_path'에 지정된 위치에 있는 파일을 엽니다. 그런 다음 text_reader의 도움으로 TextReader 클래스의 메서드를 사용하여 파일의 내용을 읽을 수 있습니다.
아래와 같이 'using' 블록을 사용하여 TextReader를 만들 수도 있습니다.
using(TextReader text_reader = File.OpenText(file_path)) { //user code }
'using' 블록 작업의 장점은 객체 작업이 완료되고 객체가 더 이상 필요하지 않은 후 내부에 지정된 객체가 획득한 메모리를 해제한다는 것입니다.
C#에서 TextReader는 어떻게 작동하나요?
TextReader를 사용하려면 코드에 System.IO 네임스페이스를 가져와야 합니다. TextReader는 추상 클래스이므로 'new' 키워드를 사용하여 인스턴스를 직접 생성할 수는 없지만 아래와 같이 File 클래스의 OpenText() 메서드를 사용하여 동일한 결과를 얻을 수 있습니다.
TextReader text_reader = File.OpenText(file_path);
OpenText() 메서드는 파일 위치를 입력으로 가져온 다음 읽기 위해 동일한 위치에 있는 기존 UTF-8 인코딩 텍스트 파일을 엽니다.
File.OpenText() 메서드는 TextReader의 파생 클래스인 StreamReader 클래스의 개체를 반환하므로 코드에서 TextReader 클래스의 유용한 인스턴스를 만드는 데 도움이 됩니다. 이 인스턴스는 TextReader 클래스의 메서드를 호출하여 파일의 내용을 읽는 데 사용할 수 있습니다. TextReader 클래스는 추상 클래스 MarshalByRefObject에서 파생됩니다. 상속 계층 구조는 다음과 같습니다.
객체 → MarshalByRefObject → TextReader
StreamReader와 StringReader라는 두 가지 파생 클래스를 사용하여 TextReader로 작업할 수 있습니다.
- StreamReader: 특정 인코딩의 바이트 스트림에서 문자를 읽는 데 사용됩니다.
- StringReader: 문자열에서 텍스트를 읽는 데 사용됩니다.
다음 표에서 TextReader의 몇 가지 중요한 메소드를 찾아보세요.
Method | Description |
Close() | It is used to close the TextReader and to release any system resources associated with it. |
Dispose() | It is used to release all the resources used by an object of TextReader. |
Peek() | It is used to read the next character without changing the state of the reader and it returns the next available character without actually reading it from the reader. |
Read() | It is used to read the next character from the text reader and it also advances the character position by one character. |
ReadLine() | It is used to read a line of characters from the text reader and it also returns the data as a string. |
ReadToEnd() | It is used to read all characters from the current position to the end of the text reader and it returns them as one string. |
Examples of TextReader in C#
We can pass a text file name in a TextReader constructor to create an object. Following are the different examples of TextReader in C#.
Example #1
Reading a line of a file using the ReadLine() method of TextReader.
Code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.IO; namespace ConsoleApp3 { public class Program { public static void Main() { string file = @"E:\Content\TextReader.txt"; try { if (File.Exists(file)) { // opening the text file and reading a line using (TextReader textReader = File.OpenText(file)) { Console.WriteLine(textReader.ReadLine()); } } else { Console.WriteLine("File does not exist!"); } Console.ReadKey(); } catch (Exception ex) { Console.WriteLine(ex.Message); } } } }
Output:
Example #2
Reading five characters from a file using the ReadBlock() method of TextReader.
Code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.IO; namespace ConsoleApp3 { public class Program { public static void Main() { string file = @"E:\Content\TextReader.txt"; try { if (File.Exists(file)) { //Opening the text file and reading 5 characters using (TextReader textReader = File.OpenText(file)) { char[] ch = new char[5]; textReader.ReadBlock(ch, 0, 5); Console.WriteLine(ch); } } else { Console.WriteLine("File does not exist!"); } Console.ReadKey(); } catch (Exception ex) { Console.WriteLine(ex.Message); } } } }
Output:
Example #3
Reading the whole content of a text file using the ReadToEnd() method of TextReader.
Code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.IO; namespace ConsoleApp3 { public class Program { public static void Main() { string file = @"E:\Content\TextReader.txt"; string content = String.Empty; try { if (File.Exists(file)) { //Opening a text file and reading the whole content using (TextReader tr = File.OpenText(file)) { content = tr.ReadToEnd(); Console.WriteLine(content); } } else { Console.WriteLine("File does not exist!"); } Console.ReadKey(); } catch (Exception ex) { Console.WriteLine(ex.Message); } } } }
Output:
Example #4
Reading the content of a text file using TextReader and writing it to another file.
Code:
using System; using System.Text; using System.Threading.Tasks; using System.IO; namespace ConsoleApp3 { public class Program { public static void Main() { string fileToRead = @"E:\Content\TextReader.txt"; string fileToWrite = @"E:\Content\TextReaderAndWriter.txt"; StringBuilder content = new StringBuilder(); string str = String.Empty; try { //checking if the file exists to read if (File.Exists(fileToRead)) { //Opening a text file and reading the whole content using (TextReader textReader = File.OpenText(fileToRead)) { while ((str = textReader.ReadLine()) != null) { content.Append("\n" + str); } } } else { Console.WriteLine("File does not exist!"); } //checking if the file to write content already exists if (File.Exists(fileToWrite)) { File.Delete(fileToWrite); } //creating file if it does not exist using (TextWriter textWriter = File.CreateText(fileToWrite)) { textWriter.WriteLine(content); } Console.ReadKey(); } catch (Exception ex) { Console.WriteLine(ex.Message); } } } }
Output:
Conclusion
- TextReader is an abstract class that is used to read text or sequential series of characters from a text file.
- StreamReader and StringReader are two derived classes of TextReader with the help of which we can implement the methods of TextReader to read content from the text files.
위 내용은 C#의 TextReader의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
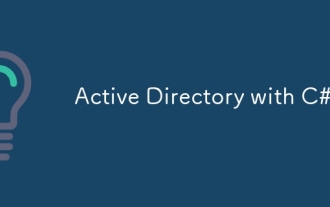
C#을 사용한 Active Directory 가이드. 여기에서는 소개와 구문 및 예제와 함께 C#에서 Active Directory가 작동하는 방식에 대해 설명합니다.
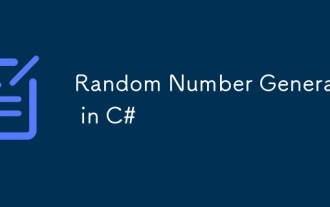
C#의 난수 생성기 가이드입니다. 여기서는 난수 생성기의 작동 방식, 의사 난수 및 보안 숫자의 개념에 대해 설명합니다.
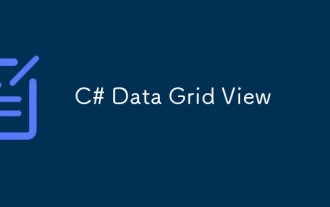
C# 데이터 그리드 뷰 가이드. 여기서는 SQL 데이터베이스 또는 Excel 파일에서 데이터 그리드 보기를 로드하고 내보내는 방법에 대한 예를 설명합니다.
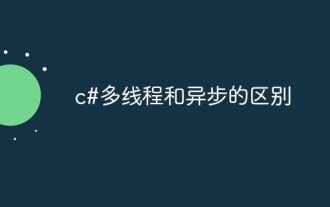
멀티 스레딩과 비동기식의 차이점은 멀티 스레딩이 동시에 여러 스레드를 실행하는 반면, 현재 스레드를 차단하지 않고 비동기식으로 작업을 수행한다는 것입니다. 멀티 스레딩은 컴퓨팅 집약적 인 작업에 사용되며 비동기식은 사용자 상호 작용에 사용됩니다. 멀티 스레딩의 장점은 컴퓨팅 성능을 향상시키는 것이지만 비동기의 장점은 UI 스레드를 차단하지 않는 것입니다. 멀티 스레딩 또는 비동기식을 선택하는 것은 작업의 특성에 따라 다릅니다. 계산 집약적 작업은 멀티 스레딩을 사용하고 외부 리소스와 상호 작용하고 UI 응답 성을 비동기식으로 유지 해야하는 작업을 사용합니다.

XML 형식을 수정하는 방법에는 여러 가지가 있습니다. Notepad와 같은 텍스트 편집기로 수동으로 편집; XMLBeautifier와 같은 온라인 또는 데스크탑 XML 서식 도구와 자동 포맷; XSLT와 같은 XML 변환 도구를 사용하여 변환 규칙을 정의합니다. 또는 Python과 같은 프로그래밍 언어를 사용하여 구문 분석하고 작동합니다. 원본 파일을 수정하고 백업 할 때주의하십시오.
