C#의 패턴
패턴은 반복되는 장식적인 디자인입니다. C#으로 패턴을 작성하는 간단한 코드가 있습니다. 별 패턴, 문자 패턴, 숫자 패턴과 같은 다양한 유형의 패턴을 인쇄하는 코드를 작성할 수 있습니다. 다음은 별, 문자, 숫자 값의 패턴을 인쇄하는 다양한 예입니다. 이러한 예제는 for 루프 내부의 루프인 루프 또는 중첩 루프로 구성됩니다. 패턴은 순서대로 또는 논리적인 방식으로 디자인하는 방법입니다. 삼각형, 피라미드, 다이아몬드 및 기타 대칭을 인쇄할 수 있습니다.
C#의 상위 3가지 패턴
C#의 상위 3가지 패턴 유형은 다음과 같습니다.
1. 별무늬
별 패턴을 인쇄하는 예시는 다음과 같습니다.
예시 #1
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace StarPattern { class Program { static void Main(string[] args) { int x, y, z; for (x =6; x >= 1; x--) { for (y = 1; y < x; y++) { Console.Write(" "); } for (z = 6; z >= x; z--) { Console.Write("*"); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예시 #2
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace StarPattern { class Program { static void Main(string[] args) { int x, y; for (x = 1; x <= 6; x++) { for (y = 1; y <= x; y++) { Console.Write("*"); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예시 #3
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace StarPattern { class Program { static void Main(string[] args) { int x, y; for (x = 5; x >= 1; x--) { for (y = 1; y <= x; y++) { Console.Write("*"); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예시 #4
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace StarPattern { class Program { static void Main(string[] args) { int x, y, z; for (x = 5; x >= 1; x--) { for (y = 5; y > x; y--) { Console.Write(" "); } for (z = 1; z <=x; z++) { Console.Write("*"); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예시 #5
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace StarPattern { class Program { static void Main(string[] args) { int x, y, z; for (x= 1; x <= 5; x++) { for (y = x; y < 5; y++) { Console.Write(" "); } for (z = 1; z < (x * 2); z++) { Console.Write("*"); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예시 #6
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace StarPattern { class Program { static void Main(string[] args) { int x, y, z; for (x = 5; x >= 1; x--) { for (y = 5; y > x; y--) { Console.Write(" "); } for (z = 1; z < (x * 2); z++) { Console.Write("*"); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예 #7
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace StarPattern { class Program { static void Main(string[] args) { int x, y; for (x = 1; x <= 5; x++) { for (y = x; y < 5; y++) { Console.Write(" "); } for (y = 1; y <= (2 * x - 1); y++) { if (x == 5 || y == 1 || y == (2 * x - 1)) { Console.Write("*"); } else { Console.Write(" "); } } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예 #8
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace CharacterPattern { class Program { static void Main(string[] args) { int x, y; for (x = 1; x <= 5; x++) { for (y = 1; y <= 5; y++) { Console.Write("*"); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예 #9
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace CharacterPattern { class Program { static void Main(string[] args) { int x, y; for (x = 1; x <= 5; x++) { for (y = 1; y <= x; y++) { if (y == 1 || y== x || x == 5) { Console.Write("*"); } else { Console.Write(" "); } } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
2. 숫자 패턴
다음은 숫자 패턴을 인쇄하는 예시입니다.
예시 #1
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace NumberPattern { class Program { static void Main(string[] args) { int x, y; for (x = 1; x <= 5; x++) { for (y = 1; y <= x; y++) { Console.Write(y); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예시 #2
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace NumberPattern { class Program { static void Main(string[] args) { int x, y; for (x = 5; x >= 1; x--) { for (y = 1; y <= x; y++) { Console.Write(y); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예시 #3
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace NumberPattern { class Program { static void Main(string[] args) { int x, y; for (x = 5; x >= 1; x--) { for (y = x; y <= 5; y++) { Console.Write(y); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예시 #4
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace NumberPattern { class Program { static void Main(string[] args) { int x, y; for (x = 1; x <= 5; x++) { for (y = x; y <= 5; y++) { Console.Write(y); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예시 #5
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace NumberPattern { class Program { static void Main(string[] args) { int x, y; for (x = 1; x <= 5; x++) { for (y = 1; y <= x; y++) { Console.Write(x); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예시 #6
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace NumberPattern { class Program { static void Main(string[] args) { int x, y; for (x = 5; x >= 1; x--) { for (y = 5; y >= x; y--) { Console.Write(x); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예 #7
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace NumberPattern { class Program { static void Main(string[] args) { int x, y; for (x = 5; x >= 1; x--) { for (y = 1; y <= x; y++) { Console.Write(x); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예 #8
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace NumberPattern { class Program { static void Main(string[] args) { int x, y; for (x = 1; x <= 5; x++) { for (y = 5; y >= x; y--) { Console.Write(x); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예 #9
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace NumberPattern { class Program { static void Main(string[] args) { int x, y; for (x = 6; x >= 1; x--) { for (y = x; y >= 1; y--) { Console.Write(y); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예 #10
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace NumberPattern { class Program { static void Main(string[] args) { int x, y; for (x = 1; x <= 5; x++) { for (y = 6; y >= x; y--) { Console.Write(y); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예시 #11
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace NumberPattern { class Program { static void Main(string[] args) { int x, y; for (x = 7; x >= 1; x -= 2) { for (y = 1; y <= x; y++) { Console.Write(y); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
3. 문자패턴
다음은 문자 패턴 인쇄 예시입니다.
예시 #1
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace CharacterPattern { class Program { static void Main(string[] args) { int x, y; int z = 5; for (x = 1; x <= z; x++) { for (y = 1; y <= x; y++) { Console.Write((char)(x + 64)); } Console.WriteLine(""); } Console.ReadLine(); } } }
출력:
예시 #2
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace CharacterPattern { class Program { static void Main(string[] args) { int x, y; int z = 5; for (x = 1; x <= z; x++) { for (y = x; y <= z; y++) { Console.Write((char)(x + 64)); } Console.WriteLine(""); } Console.ReadLine(); } } }
출력:
예시 #3
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace CharacterPattern { class Program { static void Main(string[] args) { int x, y; int z = 5; for (x = 1; x <= z; x++) { for (y = 1; y <= x; y++) { Console.Write((char)(z - x + 1 + 64)); } Console.WriteLine(""); } Console.ReadLine(); } } }
출력:
예시 #4
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace CharacterPattern { class Program { static void Main(string[] args) { int x, y; int z = 5; for (x = 1; x <= z; x++) { for (y = x; y<= z; y++) { Console.Write((char)(y + 64)); } Console.WriteLine(); } Console.ReadLine(); } } }
출력:
예시 #5
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace CharacterPattern { class Program { static void Main(string[] args) { int x, y, z; int k = 5; for (x = 1; x <= k; x++) { for (y = 1; y <= k - x; y++) { Console.Write(" "); } for (z = 1; z <= x; z++) { Console.Write((char)(x + 64)); } Console.WriteLine(); } Console.ReadLine(); } } }
Output:
Example #6
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace CharacterPattern { class Program { static void Main(string[] args) { int x, y; int a = 5; for (x = 1; x <= a; x++) { for (y = x; y >= 1; y--) { Console.Write((char)(y + 64)); } Console.WriteLine(""); } Console.ReadLine(); } } }
Output:
Example #7
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace CharacterPattern { class Program { static void Main(string[] args) { int x, y; int a = 5; for (x = a; x >= 1; x--) { for (y = a; y >= x; y--) { Console.Write((char)(y + 64)); } Console.WriteLine(""); } Console.ReadLine(); } } }
Output:
Example #8
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace CharacterPattern { class Program { static void Main(string[] args) { int x, y; int a = 5; for (x = 1; x <= a; x++) { for (y = a; y >= x; y--) { Console.Write((char)(y + 64)); } Console.WriteLine(""); } Console.ReadLine(); } } }
Output:
Example #9
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace CharacterPattern { class Program { static void Main(string[] args) { int x, y; int z = 5; for (x = z; x >= 1; x--) { for (y = x; y >= 1; y--) { Console.Write((char)(y + 64)); } Console.WriteLine(""); } Console.ReadLine(); } } }
Output:
Example #10
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace CharacterPattern { class Program { static void Main(string[] args) { int x, y; int z = 6; for (x = 1; x <= z; x++) { for (y = 1; y<= z - x; y++) { Console.Write(" "); } for (y = 1; y <= x; y++) { Console.Write((char)(y + 64)); } for (y = x - 1; y >= 1; y--) { Console.Write((char)(y + 64)); } Console.WriteLine(); } Console.ReadLine(); } } }
Output:
Conclusion
So above are some examples of various types of patterns. We can print any type of pattern with some changes in the loops.
위 내용은 C#의 패턴의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










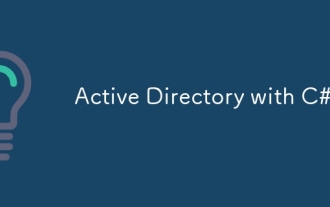
C#을 사용한 Active Directory 가이드. 여기에서는 소개와 구문 및 예제와 함께 C#에서 Active Directory가 작동하는 방식에 대해 설명합니다.
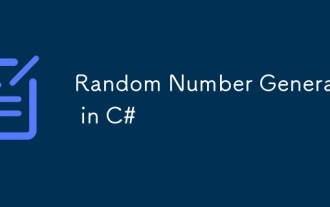
C#의 난수 생성기 가이드입니다. 여기서는 난수 생성기의 작동 방식, 의사 난수 및 보안 숫자의 개념에 대해 설명합니다.
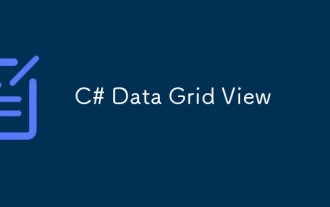
C# 데이터 그리드 뷰 가이드. 여기서는 SQL 데이터베이스 또는 Excel 파일에서 데이터 그리드 보기를 로드하고 내보내는 방법에 대한 예를 설명합니다.
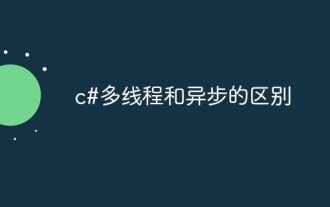
멀티 스레딩과 비동기식의 차이점은 멀티 스레딩이 동시에 여러 스레드를 실행하는 반면, 현재 스레드를 차단하지 않고 비동기식으로 작업을 수행한다는 것입니다. 멀티 스레딩은 컴퓨팅 집약적 인 작업에 사용되며 비동기식은 사용자 상호 작용에 사용됩니다. 멀티 스레딩의 장점은 컴퓨팅 성능을 향상시키는 것이지만 비동기의 장점은 UI 스레드를 차단하지 않는 것입니다. 멀티 스레딩 또는 비동기식을 선택하는 것은 작업의 특성에 따라 다릅니다. 계산 집약적 작업은 멀티 스레딩을 사용하고 외부 리소스와 상호 작용하고 UI 응답 성을 비동기식으로 유지 해야하는 작업을 사용합니다.
