두 개의 포인터와 슬라이딩 윈도우 패턴
두 포인터 및 슬라이딩 윈도우 패턴
패턴 1: 상수 창(예: 창 = 4 또는 일부 정수 값)
예를 들어 (-ve 및 +ve) 정수 배열이 주어지면 크기가 k인 연속 창에 대한 최대 합계를 찾습니다.
패턴 2:(가변 창 크기)
- 접근 방식:
- 무차별 대입: 가능한 모든 하위 배열을 생성하고 최대 길이 하위 배열을 선택합니다. sum <=k($O(n^2)$의 시간 복잡도를 갖습니다.
- 베팅/최적: 두 개의 포인터와 슬라이딩 윈도우를 사용하여 시간 복잡도를 O(n)으로 줄입니다.
패턴 3:
이 문제는 확장할 때(오른쪽++), 축소할 때(왼쪽++) 어려워지기 때문에 해결하기가 매우 어렵습니다.
이 문제는 패턴 2
를 사용하여 해결할 수 있습니다.
합계가 k인 부분 문자열 수를 찾는 것과 같은 문제를 해결하는 데 사용됩니다.
-
다음과 같이 분류할 수 있습니다
- sum
- sum
패턴 4: 가장 짧은/최소 창 <조건>
패턴 2에 대한 다양한 접근 방식:
예: sum<=k
인 가장 큰 하위 배열
public class Sample{ public static void main(String args[]){ n = 10; int arr[] = new int[n]; //Brute force approach for finding the longest subarray with sum <=k //tc : O(n^2) int maxlen=0; for(int i =0;i<arr.length;i++){ int sum =0; for(int j = i+1;j<arr.length;j++){ sum+=arr[j]; if(sum<=k){ maxLen = Integer.max(maxLen, j-i+1); } else if(sum > k) break; /// optimization if the sum is greater than the k, what is the point in going forward? } } <p><strong>두 개의 포인터와 슬라이딩 창을 사용하는 더 나은 접근 방법</strong><br> </p> <pre class="brush:php;toolbar:false"> //O(n+n) in the worst case r will move from 0 to n and in the worst case left will move from 0 0 n as well so 2n int left = 0; int right =0; int sum = 0; int maxLen = 0; while(right<arr.length){ sum+=arr[right]; while(sum > k){ sum = sum-arr[left]; left++; } if(sum <=k){ maxLen = Integer.max(maxLen, right-left+1); //if asked to print max subarray length,we print maxLen else asked for printing the subarray keep track of // left and right in a different variable } right++; }
최적의 접근 방식:
하위 배열을 찾으면 길이를 maxLen에 저장하지만 arr[right]를 추가하는 동안 합계가 k보다 커지면 현재 sum = sum-arr[left]를 수행하고 left++를 수행하여 왼쪽으로 축소됩니다.
현재 최대 길이가 maxLen이라는 것을 알고 있습니다. 왼쪽 인덱스를 계속 축소하면 조건(<=k)을 충족하는 다른 하위 배열을 얻을 수 있지만 길이가 현재 maxLen보다 작을 수 있으므로 다음까지 maxLen을 업데이트하지 않습니다. 조건을 만족하고 len >를 갖는 또 다른 하위 배열을 찾습니다. maxLen이면 maxLen만 업데이트됩니다.
최적의 접근 방식은 하위 배열이 (<=k)int left = 0 조건을 만족하지 않는 경우 하위 배열 길이가 maxLen보다 큰 한 왼쪽만 축소하는 것입니다.
int right =0; int sum = 0; int maxLen = 0; while(rightk){// this will ensure that the left is incremented one by one (not till the sum<=k because this might reduce the length i.e right-left+1 which will not be taken into consideration) sum = sum-arr[left]; left++; } if(sum <=k){ maxLen = Integer.max(maxLen, right-left+1); //if asked to print max subarray length,we print maxLen else asked for printing the subarray keep track of // left and right in a different variable } right++; } } } 위 내용은 두 개의 포인터와 슬라이딩 윈도우 패턴의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
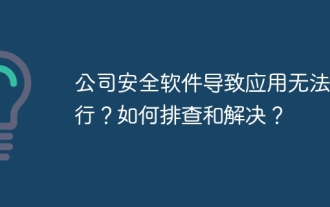
일부 애플리케이션이 제대로 작동하지 않는 회사의 보안 소프트웨어에 대한 문제 해결 및 솔루션. 많은 회사들이 내부 네트워크 보안을 보장하기 위해 보안 소프트웨어를 배포 할 것입니다. ...
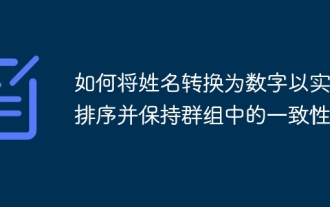
많은 응용 프로그램 시나리오에서 정렬을 구현하기 위해 이름으로 이름을 변환하는 솔루션, 사용자는 그룹으로, 특히 하나로 분류해야 할 수도 있습니다.
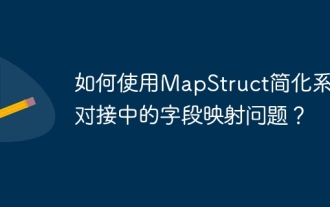
시스템 도킹의 필드 매핑 처리 시스템 도킹을 수행 할 때 어려운 문제가 발생합니다. 시스템의 인터페이스 필드를 효과적으로 매핑하는 방법 ...
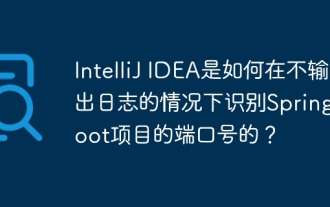
IntellijideAultimate 버전을 사용하여 봄을 시작하십시오 ...
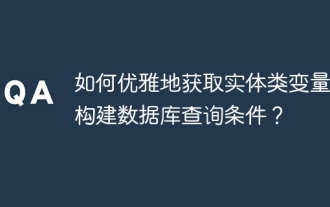
데이터베이스 작업에 MyBatis-Plus 또는 기타 ORM 프레임 워크를 사용하는 경우 엔티티 클래스의 속성 이름을 기반으로 쿼리 조건을 구성해야합니다. 매번 수동으로 ...
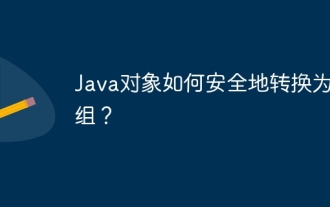
Java 객체 및 배열의 변환 : 캐스트 유형 변환의 위험과 올바른 방법에 대한 심층적 인 논의 많은 Java 초보자가 객체를 배열로 변환 할 것입니다 ...
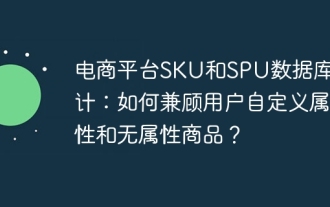
전자 상거래 플랫폼에서 SKU 및 SPU 테이블의 디자인에 대한 자세한 설명이 기사는 전자 상거래 플랫폼에서 SKU 및 SPU의 데이터베이스 설계 문제, 특히 사용자 정의 판매를 처리하는 방법에 대해 논의 할 것입니다 ...
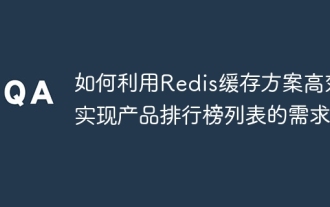
Redis 캐싱 솔루션은 제품 순위 목록의 요구 사항을 어떻게 인식합니까? 개발 과정에서 우리는 종종 a ... 표시와 같은 순위의 요구 사항을 처리해야합니다.
