불필요한 React 구성 요소 다시 렌더링을 방지하는 방법
효율적이고 성능이 뛰어난 애플리케이션을 구축하려면 React Native가 구성 요소를 렌더링하는 방법을 이해하는 것이 필수적입니다. 컴포넌트의 상태나 props가 변경되면 React는 해당 변경 사항을 반영하기 위해 사용자 인터페이스(UI)를 자동으로 업데이트합니다. 결과적으로 React는 구성 요소의 render 메서드를 다시 호출하여 업데이트된 UI 표현을 생성합니다.
이 기사에서는 세 가지 React Hooks와 React에서 불필요한 렌더링을 방지하는 방법을 살펴보겠습니다
- 메모 사용
- 콜백 사용
- Ref 사용
이러한 도구를 사용하면 불필요한 재렌더링을 방지하고 성능을 개선하며 값을 효율적으로 저장하여 코드를 최적화할 수 있습니다.
이 기사를 마치면 이러한 편리한 React 후크를 사용하여 React 애플리케이션을 더 빠르고 반응적으로 만드는 방법을 더 잘 이해할 수 있습니다.
React의 useMemo 사용하기
React에서는 useMemo를 사용하여 불필요한 재렌더링을 방지하고 성능을 최적화할 수 있습니다.
useMemo 후크가 React 구성요소에서 불필요한 재렌더링을 어떻게 방지할 수 있는지 살펴보겠습니다.
useMemo는 함수의 결과를 기억하고 종속성을 추적함으로써 필요한 경우에만 프로세스가 다시 계산되도록 합니다.
다음 예를 고려해보세요.
import { useMemo, useState } from 'react'; function Page() { const [count, setCount] = useState(0); const [items] = useState(generateItems(300)); const selectedItem = useMemo(() => items.find((item) => item.id === count), [ count, items, ]); function generateItems(count) { const items = []; for (let i = 0; i < count; i++) { items.push({ id: i, isSelected: i === count - 1, }); } return items; } return ( <div className="tutorial"> <h1>Count: {count}</h1> <h1>Selected Item: {selectedItem?.id}</h1> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); } export default Page;
위 코드는 useMemo를 사용하여 selectedItem 계산을 최적화하는 Page라는 React 구성 요소입니다.
설명은 다음과 같습니다.
- 구성요소는 useState 후크를 사용하여 상태 변수 개수를 유지합니다.
- 항목 상태는 generateItems 함수의 결과와 함께 useState 후크를 사용하여 초기화됩니다.
- selectedItem은 items.find 작업의 결과를 기억하는 useMemo를 사용하여 계산됩니다. 개수나 항목이 변경된 경우에만 다시 계산됩니다.
- generateItems 함수는 주어진 개수를 기준으로 항목 배열을 생성합니다.
- 구성요소는 현재 count 값, selectedItem ID 및 개수를 증가시키는 버튼을 렌더링합니다.
useMemo를 사용하면 items.find 작업의 결과를 메모하여 성능을 최적화합니다. selectedItem의 계산은 종속성(개수 또는 항목)이 변경될 때만 수행되어 후속 렌더링 시 불필요한 재계산을 방지합니다.
메모이제이션은 렌더링 프로세스에 추가 오버헤드를 발생시키므로 계산 집약적인 작업에 선택적으로 사용해야 합니다.
React의 useCallback 사용하기
React의 useCallback 후크를 사용하면 함수를 메모하여 각 구성 요소 렌더링 중에 해당 함수가 다시 생성되는 것을 방지할 수 있습니다. useCallback을 활용합니다. 부품은 한 번만 생성되며 해당 종속성이 변경되지 않는 한 후속 렌더링에서 재사용됩니다.
다음 예를 고려해보세요.
import React, { useState, useCallback, memo } from 'react'; const allColors = ['red', 'green', 'blue', 'yellow', 'orange']; const shuffle = (array) => { const shuffledArray = [...array]; for (let i = shuffledArray.length - 1; i > 0; i--) { const j = Math.floor(Math.random() * (i + 1)); [shuffledArray[i], shuffledArray[j]] = [shuffledArray[j], shuffledArray[i]]; } return shuffledArray; }; const Filter = memo(({ onChange }) => { console.log('Filter rendered!'); return ( <input type='text' placeholder='Filter colors...' onChange={(e) => onChange(e.target.value)} /> ); }); function Page() { const [colors, setColors] = useState(allColors); console.log(colors[0]) const handleFilter = useCallback((text) => { const filteredColors = allColors.filter((color) => color.includes(text.toLowerCase()) ); setColors(filteredColors); }, [colors]); return ( <div className='tutorial'> <div className='align-center mb-2 flex'> <button onClick={() => setColors(shuffle(allColors))}> Shuffle </button> <Filter onChange={handleFilter} /> </div> <ul> {colors.map((color) => ( <li key={color}>{color}</li> ))} </ul> </div> ); } export default Page;
위 코드는 React 구성 요소의 간단한 색상 필터링 및 셔플링 기능을 보여줍니다. 단계별로 살펴보겠습니다.
- 초기 색상 배열은 allColors로 정의됩니다.
- shuffle 함수는 배열을 가져와 해당 요소를 무작위로 섞습니다. Fisher-Yates 알고리즘을 사용하여 셔플링을 수행합니다.
- Filter 컴포넌트는 입력 요소를 렌더링하는 메모화된 기능 컴포넌트입니다. onChange prop을 받아 입력 값이 변경되면 콜백 함수를 트리거합니다.
- 페이지 구성 요소는 색상 필터링 및 셔플링 기능을 렌더링하는 주요 구성 요소입니다.
- 상태 변수 colors는 useState 후크를 사용하여 초기화되며 초기 값은 allColors로 설정됩니다. 필터링된 색상 목록을 나타냅니다.
- handleFilter 함수는 useCallback 후크를 사용하여 생성됩니다. 텍스트 매개변수를 사용하고 제공된 텍스트를 기반으로 allColors 배열을 필터링합니다. 필터링된 색상은 useState 후크의 setColors 함수를 사용하여 설정됩니다. 종속성 배열 [colors]는 색상 상태가 변경되는 경우에만 handlerFilter 함수가 다시 생성되도록 보장하여 불필요한 재렌더링을 방지하여 성능을 최적화합니다.
- 페이지 구성 요소 내부에는 색상을 섞는 버튼이 있습니다. 버튼을 클릭하면 섞인 allColors 배열과 함께 setColors 함수가 호출됩니다.
- Filter 구성요소는 onChange prop이 handlerFilter 함수로 설정된 상태로 렌더링됩니다.
- 마지막으로 색상 배열이 매핑되어 색상 항목 목록을 으로 렌더링합니다.
- 요소.
useCallback 후크는 handlerFilter 함수를 기억하는 데 사용됩니다. 즉, 함수는 한 번만 생성되고 종속성(이 경우 색상 상태)이 동일하게 유지되는 경우 후속 렌더링에서 재사용됩니다.
This optimization prevents unnecessary re-renders of child components that receive the handleFilter function as a prop, such as the Filter component.
It ensures that the Filter component is not re-rendered if the colors state hasn't changed, improving performance.
Using React's useRef
Another approach to enhance performance in React applications and avoid unnecessary re-renders is using the useRef hook. Using useRef, we can store a mutable value that persists across renders, effectively preventing unnecessary re-renders.
This technique allows us to maintain a reference to a value without triggering component updates when that value changes. By leveraging the mutability of the reference, we can optimize performance in specific scenarios.
Consider the following example:
import React, { useRef, useState } from 'react'; function App() { const [name, setName] = useState(''); const inputRef = useRef(null); function handleClick() { inputRef.current.focus(); } return ( <div> <input type="text" value={name} onChange={(e) => setName(e.target.value)} ref={inputRef} /> <button onClick={handleClick}>Focus</button> </div> ); }
The example above has a simple input field and a button. The useRef hook creates a ref called inputRef. As soon as the button is clicked, the handleClick function is called, which focuses on the input element by accessing the current property of the inputRef ref object. As such, it prevents unnecessary rerendering of the component when the input value changes.
To ensure optimal use of useRef, reserve it solely for mutable values that do not impact the component's rendering. If a mutable value influences the component's rendering, it should be stored within its state instead.
Conclusion
Throughout this tutorial, we explored the concept of React re-rendering and its potential impact on the performance of our applications. We delved into the optimization techniques that can help mitigate unnecessary re-renders. React offers a variety of hooks that enable us to enhance the performance of our applications. We can effectively store values and functions between renders by leveraging these hooks, significantly boosting React application performance.
위 내용은 불필요한 React 구성 요소 다시 렌더링을 방지하는 방법의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
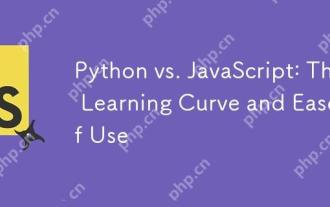
Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
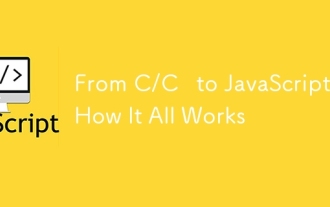
C/C에서 JavaScript로 전환하려면 동적 타이핑, 쓰레기 수집 및 비동기 프로그래밍으로 적응해야합니다. 1) C/C는 수동 메모리 관리가 필요한 정적으로 입력 한 언어이며 JavaScript는 동적으로 입력하고 쓰레기 수집이 자동으로 처리됩니다. 2) C/C를 기계 코드로 컴파일 해야하는 반면 JavaScript는 해석 된 언어입니다. 3) JavaScript는 폐쇄, 프로토 타입 체인 및 약속과 같은 개념을 소개하여 유연성과 비동기 프로그래밍 기능을 향상시킵니다.
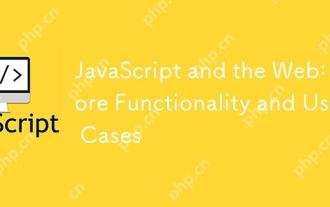
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.
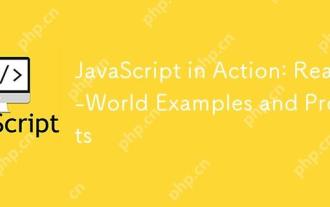
실제 세계에서 JavaScript의 응용 프로그램에는 프론트 엔드 및 백엔드 개발이 포함됩니다. 1) DOM 운영 및 이벤트 처리와 관련된 TODO 목록 응용 프로그램을 구축하여 프론트 엔드 애플리케이션을 표시합니다. 2) Node.js를 통해 RESTFULAPI를 구축하고 Express를 통해 백엔드 응용 프로그램을 시연하십시오.
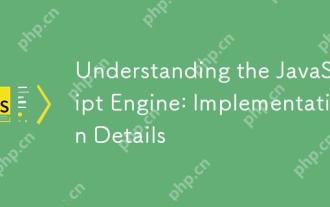
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.
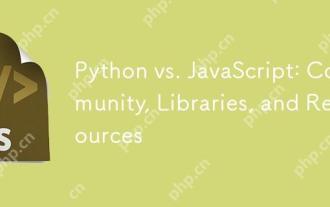
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
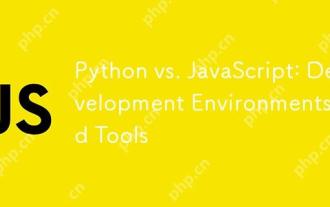
개발 환경에서 Python과 JavaScript의 선택이 모두 중요합니다. 1) Python의 개발 환경에는 Pycharm, Jupyternotebook 및 Anaconda가 포함되어 있으며 데이터 과학 및 빠른 프로토 타이핑에 적합합니다. 2) JavaScript의 개발 환경에는 Node.js, VScode 및 Webpack이 포함되어 있으며 프론트 엔드 및 백엔드 개발에 적합합니다. 프로젝트 요구에 따라 올바른 도구를 선택하면 개발 효율성과 프로젝트 성공률이 향상 될 수 있습니다.
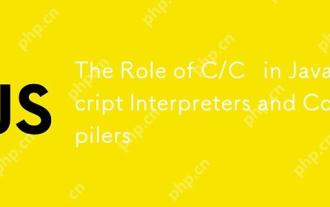
C와 C는 주로 통역사와 JIT 컴파일러를 구현하는 데 사용되는 JavaScript 엔진에서 중요한 역할을합니다. 1) C는 JavaScript 소스 코드를 구문 분석하고 추상 구문 트리를 생성하는 데 사용됩니다. 2) C는 바이트 코드 생성 및 실행을 담당합니다. 3) C는 JIT 컴파일러를 구현하고 런타임에 핫스팟 코드를 최적화하고 컴파일하며 JavaScript의 실행 효율을 크게 향상시킵니다.
