React Toastify 시작하기: 알림 강화
소개
최신 웹 애플리케이션에서는 원활하고 매력적인 경험을 유지하기 위해 사용자에게 실시간 피드백을 제공하는 것이 중요합니다. 알림은 사용자의 작업 흐름을 방해하지 않으면서 성공적인 작업, 오류 또는 경고와 같은 중요한 이벤트를 전달하는 데 중추적인 역할을 합니다. 이것이 React Toastify가 작동하는 곳입니다. React 애플리케이션에 사용자 정의 가능한 토스트 알림을 추가하는 프로세스를 단순화하는 인기 있는 라이브러리입니다. 사용자의 여정을 방해할 수 있는 기존 경고 상자와 달리 토스트 알림은 미묘하고 우아한 방식으로 나타나 사용자를 현재 상황에서 벗어나지 않으면서 중요한 정보가 전달되도록 합니다.
Toastify를 사용하면 개발자는 보기에 좋고 매우 유연한 알림을 쉽게 구현할 수 있어 위치, 스타일, 타이밍을 사용자 정의할 수 있으며 설정과 사용도 쉽습니다. 따라서 효과적인 피드백 메커니즘을 통해 사용자 경험을 향상시키려는 개발자에게 없어서는 안 될 도구입니다.
React Toastify를 사용하는 이유는 무엇입니까?
토스트 알림은 웹 애플리케이션의 여러 일반적인 시나리오에서 필수적입니다. 예를 들어, 사용자가 양식을 제출한 후 작업이 완료되었음을 확인하는 성공 메시지를 표시하거나 문제가 발생한 경우 오류 메시지를 표시할 수 있습니다. 마찬가지로 API 호출을 처리할 때 토스트 알림을 통해 사용자에게 성공적인 데이터 검색이나 오류 등의 결과를 알릴 수 있습니다.
React-Toastify는 이러한 알림을 원활하고 효율적으로 처리합니다. 기본 브라우저 경고 및 기타 라이브러리와 차별화되는 몇 가지 주요 이점은 다음과 같습니다.
- 간편한 통합: 설정이 간단하여 알림 표시를 시작하는 데 최소한의 구성만 필요합니다. 직관적인 API를 통해 초보자도 쉽게 접근할 수 있어 개발자가 복잡한 설정 없이 빠르게 토스트 알림을 추가할 수 있습니다.
- 맞춤형 디자인 및 배치: Toastify의 뛰어난 기능 중 하나는 알림의 모양과 동작을 사용자 정의하는 기능입니다. 스타일을 쉽게 수정하고 화면의 어느 위치에나 배치할 수 있으며(예: 오른쪽 위, 왼쪽 아래) 맞춤 전환도 만들 수 있습니다. 이러한 유연성은 애플리케이션 전체에서 일관된 UI/UX를 유지하는 데 도움이 됩니다.
자동 및 수동 해제 모두 지원: Toastify를 사용하면 알림이 표시되는 기간을 제어할 수 있습니다. 지정된 시간이 지나면 자동으로 사라지도록 선택하거나 사용자가 수동으로 알림을 닫도록 허용하여 상황에 따라 더 나은 사용자 경험을 제공할 수 있습니다.
기본 브라우저 경고와의 비교: 기본 브라우저 경고는 방해가 되며 해제될 때까지 사용자 상호작용을 차단합니다. 반면에 Toastify는 화면 모서리에 표시되어 사용자가 페이지와 계속 상호 작용할 수 있도록 방해하지 않고 우아한 알림 메시지를 제공합니다. 또한 브라우저 경고로는 불가능한 다양한 토스트 유형(성공, 오류, 정보) 및 더욱 풍부한 스타일과 같은 고급 기능을 지원합니다.
React-Toastify를 React 애플리케이션에 통합하면 강력하고 사용자 정의 가능한 알림 관리 방법을 얻을 수 있으므로 원활하고 현대적인 사용자 경험을 유지하면서 사용자에게 더 쉽게 피드백을 제공할 수 있습니다.
설치 및 설정
React-Toastify를 시작하는 것은 간단하며 몇 단계만 거치면 됩니다. React 프로젝트에 설치하고 설정하는 방법은 다음과 같습니다.
1단계: React Toastify 설치
먼저 프로젝트에 React-Toastify 패키지를 추가해야 합니다. 터미널에서 다음 명령을 사용하세요:
npm install react-toastify
2단계: 프로젝트에서 React Toastify 가져오기 및 사용
패키지가 설치되면 React Toastify와 핵심 구성 요소를 React 프로젝트로 가져와야 합니다. 최소한 화면에 토스트 알림을 렌더링하는 ToastContainer를 가져와야 합니다.
설정 방법은 다음과 같습니다.
- ToastContainer를 가져와 구성 요소에 토스트합니다.
- ToastContainer가 구성 요소의 JSX에 포함되어 있는지 확인하세요.
- 토스트 기능을 사용하여 토스트 알림을 실행하세요.
예:
import React from 'react'; import { ToastContainer, toast } from 'react-toastify'; import 'react-toastify/dist/ReactToastify.css'; const App = () => { const notify = () => toast("This is a toast notification!"); return ( <div> <h1>React Toastify Example</h1> <button onClick={notify}>Show Notification</button> <ToastContainer /> </div> ); }; export default App;
3단계: 토스트 스타일 추가
알림에 기본 스타일을 적용하려면 React Toastify CSS 파일을 가져오는 것을 잊지 마세요.
import 'react-toastify/dist/ReactToastify.css';
Now, when you click the button, a toast notification will appear on the screen. The ToastContainer can be positioned anywhere in your app, and the toasts will automatically appear within it. You can further customize the appearance and behavior of the toast, which we will explore in the following sections.
Basic Usage of React Toastify
Once you have React Toastify set up, you can easily trigger various types of notifications based on user actions. Let's explore how to use it to display different toast notifications for success, error, info, and warning messages.
Example 1: Triggering a Success Notification
A common use case for a success notification is after a form submission. You can trigger it using the following code:
toast.success("Form submitted successfully!");
This will display a success message styled with a green icon, indicating a positive action.
Example 2: Error Notification
You can also display an error message when something goes wrong, such as a failed API call or form validation error:
toast.error("Something went wrong. Please try again!");
This shows a red-colored toast indicating an issue that requires the user's attention.
Example 3: Info Notification
Info notifications are useful when you want to inform the user about a status or update without implying success or failure. For example:
toast.info("New updates are available!");
Example 4: Warning Notification
If you need to alert the user to potential issues or important warnings, you can use the warning notification:
toast.warn("Your session is about to expire!");
This shows an orange toast, typically used for warnings or cautions.
import React from 'react'; import { ToastContainer, toast } from 'react-toastify'; import 'react-toastify/dist/ReactToastify.css'; const App = () => { const showToasts = () => { toast.success("Form submitted successfully!"); toast.error("Something went wrong. Please try again!"); toast.info("New updates are available!"); toast.warn("Your session is about to expire!"); }; return (); }; export default App;React Toastify Notifications
With this code, clicking the button will trigger all types of notifications, allowing you to see how each one looks and behaves in your application.
Customizing Toast Notifications
One of the great features of React Toastify is its flexibility in customizing toast notifications to fit the look and feel of your application. You can easily adjust the position, duration, and even styling to suit your needs. Let’s walk through some of these customizations.
Customizing Position
React Toastify allows you to position toast notifications in various locations on the screen. By default, toasts are displayed in the top-right corner, but you can customize their position using the position property of the ToastContainer or while triggering individual toasts.
Available positions:
- top-right (default)
- top-center
- top-left
- bottom-right
- bottom-center
- bottom-left
Here’s an example of how to change the position of toasts globally via the ToastContainer:
<ToastContainer position="bottom-left" />
If you want to customize the position of individual toasts, you can pass the position option like this:
toast.success("Operation successful!", { position: "top-center" });
This will display the success notification at the top-center of the screen.
Adjusting the Auto-Dismiss Timer
toast.info("This will disappear in 3 seconds!", { autoClose: 3000 });
If you want the toast to stay on screen until the user manually dismisses it, set autoClose to false:
toast.warn("This requires manual dismissal.", { autoClose: false });
Customizing Toast Styling
React Toastify provides the flexibility to style your toasts either through CSS classes or inline styles. You can pass a custom CSS class to the className or bodyClassName options to style the overall toast or its content.
Here’s an example of using a custom CSS class to style a toast:
toast("Custom styled toast!", { className: "custom-toast", bodyClassName: "custom-toast-body", autoClose: 5000 });
In your CSS file, you can define the styling:
.custom-toast { background-color: #333; color: #fff; } .custom-toast-body { font-size: 18px; }
This gives you complete control over how your notifications appear, allowing you to match the toast design with your application’s overall theme.
Advanced Features of React Toastify
React Toastify offers useful features to enhance the functionality of your toast notifications. Here's how you can leverage progress bars, custom icons, transitions, and event listeners.
Progress Bars in Toast Notifications
By default, React Toastify includes a progress bar that indicates how long the toast will stay visible. To disable the progress bar:
toast.info("No progress bar", { hideProgressBar: true });
Custom Icons and Transitions
You can replace default icons with custom icons for a more personalized look:
toast("Custom Icon", { icon: "?" });
To apply custom transitions like Bounce:
toast("Bounce Animation", { transition: Bounce });
Adding Event Listeners to Toasts
React Toastify allows you to add event listeners to handle custom actions, such as on click:
toast.info("Click me!", { onClick: () => alert("Toast clicked!") });
You can also trigger actions when a toast is closed:
toast.success("Success!", { onClose: () => console.log("Toast closed") });
Best Practices for Using React Toastify
To ensure that toast notifications enhance rather than hinder the user experience, it's important to follow best practices. Here are some guidelines to consider:
-
알림을 아껴서 사용하세요
알림은 도움이 될 수 있지만 과도하게 사용하면 사용자를 좌절시키거나 주의가 산만해질 수 있습니다. 성공적인 작업(예: 양식 제출) 확인 또는 주의가 필요한 오류 메시지 표시와 같은 중요한 업데이트에 대한 토스트 알림을 예약하세요.
-
올바른 알림 유형을 선택하세요
적절한 토스트 유형(성공, 오류, 정보, 경고)을 사용하여 올바른 톤을 전달하세요. 예를 들어 성공 메시지는 완료된 작업에 사용해야 하고 경고는 잠재적인 문제에 대해 예약해야 합니다.
-
사용자 작업 차단 방지
토스트 메시지는 방해가 되지 않으므로 중요한 사용자 상호작용을 차단해서는 안 됩니다. 사용자가 작업을 계속하는 것을 방해하지 않는 방식으로 알림을 표시합니다.
-
상황에 따라 타이밍을 맞춤설정하세요
토스트에 대한 적절한 자동 종료 시간을 설정합니다. 오류 메시지는 더 오래 유지되어야 하지만 성공 알림은 빠르게 사라질 수 있습니다. 중요한 문제의 경우 사용자가 알림을 수동으로 닫을 수 있도록 하는 것이 좋습니다.
결론
React-Toastify는 React 애플리케이션에서 알림을 원활하고 효율적으로 구현하여 사용자에게 실시간 피드백을 제공하기 위한 유연한 솔루션을 제공합니다. 사용자 정의 가능한 디자인, 위치 지정 옵션, 진행률 표시줄 및 이벤트 리스너와 같은 고급 기능 지원을 통해 알림 프로세스를 단순화하는 동시에 사용자 경험을 효과적으로 제어할 수 있습니다.
모범 사례를 따르고 토스트 알림을 현명하게 사용하면 사용자에게 부담을 주지 않으면서 상호 작용을 향상할 수 있습니다. 더 자세한 정보와 고급 사용 사례를 보려면 공식 React Toastify 문서를 확인하세요.
위 내용은 React Toastify 시작하기: 알림 강화의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
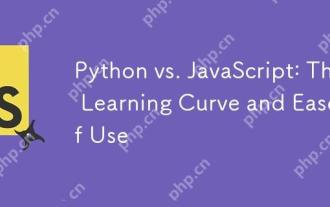
Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
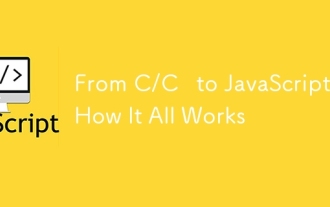
C/C에서 JavaScript로 전환하려면 동적 타이핑, 쓰레기 수집 및 비동기 프로그래밍으로 적응해야합니다. 1) C/C는 수동 메모리 관리가 필요한 정적으로 입력 한 언어이며 JavaScript는 동적으로 입력하고 쓰레기 수집이 자동으로 처리됩니다. 2) C/C를 기계 코드로 컴파일 해야하는 반면 JavaScript는 해석 된 언어입니다. 3) JavaScript는 폐쇄, 프로토 타입 체인 및 약속과 같은 개념을 소개하여 유연성과 비동기 프로그래밍 기능을 향상시킵니다.
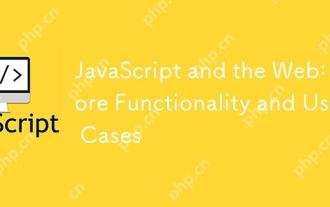
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.
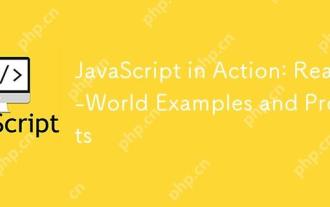
실제 세계에서 JavaScript의 응용 프로그램에는 프론트 엔드 및 백엔드 개발이 포함됩니다. 1) DOM 운영 및 이벤트 처리와 관련된 TODO 목록 응용 프로그램을 구축하여 프론트 엔드 애플리케이션을 표시합니다. 2) Node.js를 통해 RESTFULAPI를 구축하고 Express를 통해 백엔드 응용 프로그램을 시연하십시오.
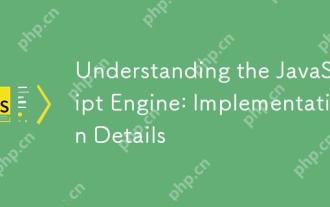
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.
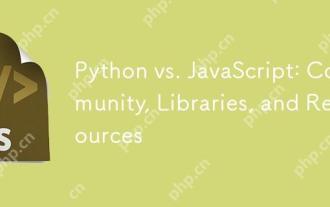
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
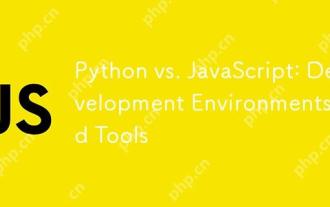
개발 환경에서 Python과 JavaScript의 선택이 모두 중요합니다. 1) Python의 개발 환경에는 Pycharm, Jupyternotebook 및 Anaconda가 포함되어 있으며 데이터 과학 및 빠른 프로토 타이핑에 적합합니다. 2) JavaScript의 개발 환경에는 Node.js, VScode 및 Webpack이 포함되어 있으며 프론트 엔드 및 백엔드 개발에 적합합니다. 프로젝트 요구에 따라 올바른 도구를 선택하면 개발 효율성과 프로젝트 성공률이 향상 될 수 있습니다.
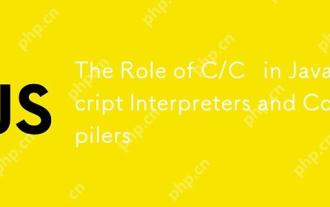
C와 C는 주로 통역사와 JIT 컴파일러를 구현하는 데 사용되는 JavaScript 엔진에서 중요한 역할을합니다. 1) C는 JavaScript 소스 코드를 구문 분석하고 추상 구문 트리를 생성하는 데 사용됩니다. 2) C는 바이트 코드 생성 및 실행을 담당합니다. 3) C는 JIT 컴파일러를 구현하고 런타임에 핫스팟 코드를 최적화하고 컴파일하며 JavaScript의 실행 효율을 크게 향상시킵니다.
