Golang의 메모리 사용량 최적화: 힙에 변수가 할당되는 시기
Golang으로 애플리케이션을 개발할 때 직면하는 일반적인 과제 중 하나는 메모리 관리입니다. Golang은 스택과 힙이라는 두 가지 주요 메모리 저장 위치를 사용합니다. 변수가 힙과 스택에 할당되는 시점을 이해하는 것은 우리가 구축하는 애플리케이션의 성능을 최적화하는 데 중요합니다. 이번 글에서는 변수가 힙에 할당되는 주요 조건을 살펴보고 Go 컴파일러가 메모리 할당을 결정하는 데 사용하는 이스케이프 분석의 개념을 소개하겠습니다.
TL;DR
Golang에서는 힙이나 스택에 변수를 할당할 수 있습니다. 힙 할당은 변수가 함수 범위나 더 큰 개체보다 오래 지속되어야 할 때 발생합니다. Go는 이스케이프 분석을 사용하여 변수를 힙에 할당해야 하는지 여부를 결정합니다.
힙 할당은 다음 시나리오에서 발생합니다.
- 변수는 함수나 범위를 "이스케이프"합니다.
- 변수는 전역 변수와 같이 수명 주기가 긴 위치에 저장됩니다.
- 변수는 함수 외부에서 사용되는 구조체에 배치됩니다.
- 큰 스택을 사용하지 않기 위해 큰 객체는 힙에 할당됩니다.
- 로컬 변수에 대한 참조를 저장하는 클로저는 힙 할당을 트리거합니다.
- 변수가 인터페이스에 캐스팅되면 힙 할당이 자주 발생합니다.
메모리는 가비지 컬렉터(GC)에 의해 관리되기 때문에 힙 할당 속도가 느리므로 사용량을 최소화하는 것이 중요합니다.
스택과 힙이란 무엇입니까?
본론에 들어가기에 앞서 먼저 스택과 힙의 차이점을 알아보겠습니다.
- 스택: 스택 메모리는 함수나 고루틴의 지역 변수를 저장하는 데 사용됩니다. 스택은 가장 최근의 데이터가 가장 먼저 제거되는 LIFO(후입선출) 방식으로 작동합니다. 스택에 할당된 변수는 함수가 실행되는 동안에만 유지되며 함수가 범위를 벗어나면 자동으로 제거됩니다. 스택의 할당 및 할당 해제는 매우 빠르지만 스택 크기가 제한됩니다.
- 힙: 힙 메모리는 함수 수명 주기 이후에도 지속되어야 하는 객체나 변수를 저장하는 데 사용됩니다. 스택과 달리 힙은 LIFO 패턴을 따르지 않으며 사용되지 않은 메모리를 주기적으로 정리하는 가비지 수집기(GC)에 의해 관리됩니다. 힙은 장기 저장에 더 유연하지만 힙 메모리에 액세스하는 속도가 느리고 GC를 통한 추가 관리가 필요합니다.
탈출 분석이란 무엇입니까?
이스케이프 분석은 변수를 스택에 할당할 수 있는지 아니면 힙으로 이동해야 하는지 결정하기 위해 Go 컴파일러에서 수행하는 프로세스입니다. 변수가 함수나 범위를 "이스케이프"하면 힙에 할당됩니다. 반대로 변수가 함수 범위 내에 남아 있으면 스택에 저장할 수 있습니다.
변수는 언제 힙에 할당되나요?
여러 조건으로 인해 변수가 힙에 할당됩니다. 각 상황에 대해 논의해 보겠습니다.
1. 변수가 함수나 범위에서 "이스케이프"되는 경우
함수 내에서 변수가 선언되었지만 해당 참조가 함수를 이스케이프할 때 힙 할당이 발생합니다. 예를 들어 함수에서 지역 변수에 대한 포인터를 반환하면 해당 변수가 힙에 할당됩니다.
예:
func newInt() *int { x := 42 return &x // "x" is allocated on the heap because a pointer is returned }
이 예에서 변수 x는 newInt() 함수가 완료된 후에도 활성 상태로 유지되어야 하므로 Go는 힙에 x를 할당합니다.
2. 변수가 더 오래 지속되는 위치에 저장되는 경우
변수가 선언된 범위보다 수명주기가 긴 위치에 변수가 저장되면 힙에 할당됩니다. 전형적인 예는 지역 변수에 대한 참조가 전역 변수나 더 오래 지속되는 구조체에 저장되는 경우입니다. 예:
var global *int func setGlobal() { x := 100 global = &x // "x" is allocated on the heap because it's stored in a global variable }
여기서 변수 x는 setGlobal() 함수 이후에도 살아남아야 하므로 힙에 할당해야 합니다. 마찬가지로 지역 변수가 생성된 함수 외부에서 사용되는 구조체에 배치되면 해당 변수가 힙에 할당됩니다. 예:
type Node struct { value *int } func createNode() *Node { x := 50 return &Node{value: &x} // "x" must be on the heap because it's stored in Node }
이 예에서는 x가 Node에 저장되고 함수에서 반환되므로 x는 함수보다 오래 지속되어야 하므로 힙에 할당됩니다.
3. 대형 물체의 경우
때로는 객체가 "이스케이프"되지 않더라도 대형 배열이나 슬라이스와 같은 대형 객체에 힙 할당이 필요합니다. 이는 너무 많은 스택 공간을 사용하지 않기 위해 수행됩니다. 예:
func largeSlice() []int { return make([]int, 1000000) // Heap allocation due to large size }
Golang은 크기가 스택에 비해 너무 크기 때문에 이 큰 조각을 저장하기 위해 힙을 사용합니다.
4. Closures that Store References to Local Variables
Closures in Golang often lead to heap allocation if the closure holds a reference to a local variable in the function where the closure is defined. For example:
func createClosure() func() int { x := 10 return func() int { return x } // "x" must be on the heap because it's used by the closure }
Since the closure func() int holds a reference to x, x must be allocated on the heap to ensure it remains alive after the createClosure() function finishes.
5. Interfaces and Dynamic Dispatch
When variables are cast to an interface, Go may need to store the dynamic type of the variable on the heap. This happens because information about the variable's type needs to be stored alongside its value. For example:
func asInterface() interface{} { x := 42 return x // Heap allocation because the variable is cast to interface{} }
In this case, Go will allocate x on the heap to ensure the dynamic type information is available.
Other Factors That Cause Heap Allocation
In addition to the conditions mentioned above, there are several other factors that may cause variables to be allocated on the heap:
1. Goroutines
Variables used within goroutines are often allocated on the heap because the lifecycle of a goroutine can extend beyond the function in which it was created.
2. Variables Managed by the Garbage Collector (GC)
If Go detects that a variable needs to be managed by the Garbage Collector (GC) (for example, because it's used across goroutines or has complex references), that variable may be allocated on the heap.
Conclusion
Understanding when and why a variable is allocated on the heap is crucial for optimizing the performance of Go applications. Escape analysis plays a key role in determining whether a variable can be allocated on the stack or must be allocated on the heap. While the heap provides flexibility for storing objects that need a longer lifespan, excessive heap usage can increase the workload of the Garbage Collector and slow down application performance. By following these guidelines, you can manage memory more efficiently and ensure your application runs with optimal performance.
If there’s anything you think I’ve missed or if you have additional experience and tips related to memory management in Go, feel free to share them in the comments below. Further discussion can help all of us better understand this topic and continue developing more efficient coding practices.
위 내용은 Golang의 메모리 사용량 최적화: 힙에 변수가 할당되는 시기의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
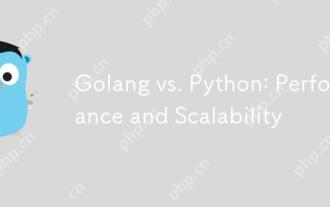
Golang은 성능과 확장 성 측면에서 Python보다 낫습니다. 1) Golang의 컴파일 유형 특성과 효율적인 동시성 모델은 높은 동시성 시나리오에서 잘 수행합니다. 2) 해석 된 언어로서 파이썬은 천천히 실행되지만 Cython과 같은 도구를 통해 성능을 최적화 할 수 있습니다.
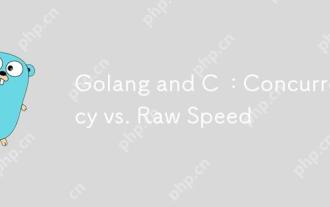
Golang은 동시성에서 C보다 낫고 C는 원시 속도에서 Golang보다 낫습니다. 1) Golang은 Goroutine 및 Channel을 통해 효율적인 동시성을 달성하며, 이는 많은 동시 작업을 처리하는 데 적합합니다. 2) C 컴파일러 최적화 및 표준 라이브러리를 통해 하드웨어에 가까운 고성능을 제공하며 극도의 최적화가 필요한 애플리케이션에 적합합니다.
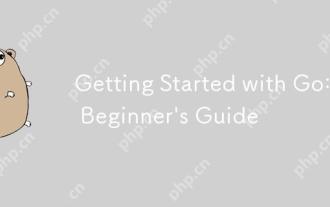
goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity, 효율성, 및 콘크리 론 피처
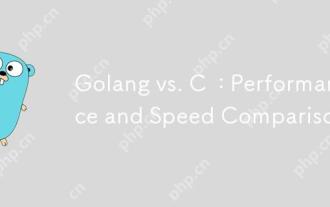
Golang은 빠른 개발 및 동시 시나리오에 적합하며 C는 극도의 성능 및 저수준 제어가 필요한 시나리오에 적합합니다. 1) Golang은 쓰레기 수집 및 동시성 메커니즘을 통해 성능을 향상시키고, 고전성 웹 서비스 개발에 적합합니다. 2) C는 수동 메모리 관리 및 컴파일러 최적화를 통해 궁극적 인 성능을 달성하며 임베디드 시스템 개발에 적합합니다.
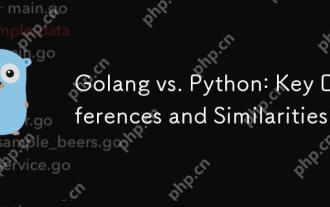
Golang과 Python은 각각 고유 한 장점이 있습니다. Golang은 고성능 및 동시 프로그래밍에 적합하지만 Python은 데이터 과학 및 웹 개발에 적합합니다. Golang은 동시성 모델과 효율적인 성능으로 유명하며 Python은 간결한 구문 및 풍부한 라이브러리 생태계로 유명합니다.
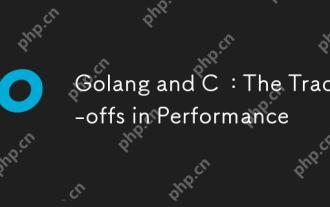
Golang과 C의 성능 차이는 주로 메모리 관리, 컴파일 최적화 및 런타임 효율에 반영됩니다. 1) Golang의 쓰레기 수집 메커니즘은 편리하지만 성능에 영향을 줄 수 있습니다. 2) C의 수동 메모리 관리 및 컴파일러 최적화는 재귀 컴퓨팅에서 더 효율적입니다.
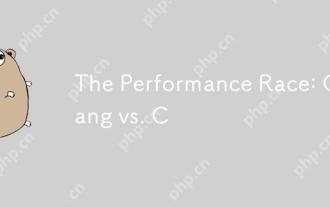
Golang과 C는 각각 공연 경쟁에서 고유 한 장점을 가지고 있습니다. 1) Golang은 높은 동시성과 빠른 발전에 적합하며 2) C는 더 높은 성능과 세밀한 제어를 제공합니다. 선택은 프로젝트 요구 사항 및 팀 기술 스택을 기반으로해야합니다.
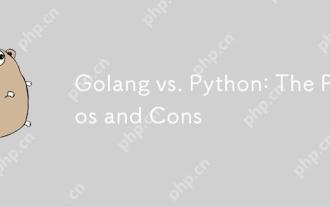
golangisidealforbuildingscalablesystemsdueToitsefficiencyandconcurrency
