TypeScript의 데코레이터 이해: 첫 번째 원칙 접근 방식
TypeScript의 데코레이터는 클래스, 메서드, 속성 및 매개변수의 동작을 수정하기 위한 강력한 메커니즘을 제공합니다. 현대적인 편리함처럼 보일 수도 있지만 데코레이터는 객체 지향 프로그래밍에서 발견되는 잘 확립된 데코레이터 패턴에 뿌리를 두고 있습니다. 데코레이터를 사용하면 로깅, 유효성 검사, 액세스 제어 등의 일반적인 기능을 추상화하여 개발자가 더 깔끔하고 유지 관리하기 쉬운 코드를 작성할 수 있습니다.
이 기사에서는 데코레이터를 첫 번째 원칙부터 살펴보고, 핵심 기능을 분석하고, 처음부터 구현해 보겠습니다. 그 과정에서 일상적인 TypeScript 개발에서 데코레이터의 유용성을 보여주는 몇 가지 실제 애플리케이션을 살펴보겠습니다.
데코레이터란 무엇입니까?
TypeScript에서 데코레이터는 단순히 클래스, 메서드, 속성 또는 매개변수에 연결할 수 있는 함수입니다. 이 함수는 디자인 타임에 실행되므로 코드가 실행되기 전에 코드의 동작이나 구조를 변경할 수 있습니다. 데코레이터를 사용하면 메타 프로그래밍이 가능하므로 원래 논리를 수정하지 않고도 추가 기능을 추가할 수 있습니다.
메서드가 호출될 때 기록하는 메소드 데코레이터의 간단한 예부터 시작해 보겠습니다.
function log(target: any, propertyKey: string, descriptor: PropertyDescriptor) { const originalMethod = descriptor.value; descriptor.value = function (...args: any[]) { console.log(`Calling ${propertyKey} with arguments: ${args}`); return originalMethod.apply(this, args); }; return descriptor; } class Example { @log greet(name: string) { return `Hello, ${name}`; } } const example = new Example(); example.greet('John');
여기서 로그 데코레이터는 Greeting 메소드를 래핑하여 실행하기 전에 해당 호출과 매개변수를 기록합니다. 이 패턴은 핵심 로직에서 로깅과 같은 교차 문제를 분리하는 데 유용합니다.
데코레이터의 작동 방식
TypeScript의 데코레이터는 꾸미고 있는 항목과 관련된 메타데이터를 가져오는 함수입니다. 이 메타데이터(예: 클래스 프로토타입, 메서드 이름, 속성 설명자)를 기반으로 데코레이터는 동작을 수정하거나 데코레이트된 객체를 교체할 수도 있습니다.
데코레이터의 유형
데코레이터는 각각 다른 목적을 가진 다양한 대상에 적용될 수 있습니다.
- 클래스 데코레이터 : 클래스의 생성자를 받는 함수입니다.
function classDecorator(constructor: Function) { // Modify or extend the class constructor or prototype }
- 메소드 데코레이터 : 대상 객체, 메소드 이름, 메소드 설명자를 전달받는 함수입니다.
function methodDecorator(target: any, propertyKey: string, descriptor: PropertyDescriptor) { // Modify the method's descriptor }
- Property Decorators : 대상 객체와 속성 이름을 전달받는 함수입니다.
function propertyDecorator(target: any, propertyKey: string) { // Modify the behavior of the property }
- 매개변수 데코레이터 : 매개변수의 대상, 메소드 이름, 인덱스를 전달받는 함수입니다.
function parameterDecorator(target: any, propertyKey: string, parameterIndex: number) { // Modify or inspect the method's parameter }
데코레이터에 인수 전달
데코레이터의 가장 강력한 기능 중 하나는 인수를 받아 동작을 사용자 정의할 수 있는 능력입니다. 예를 들어 인수에 따라 조건부로 메서드 호출을 기록하는 메서드 데코레이터를 만들어 보겠습니다.
function logConditionally(shouldLog: boolean) { return function (target: any, propertyKey: string, descriptor: PropertyDescriptor) { const originalMethod = descriptor.value; descriptor.value = function (...args: any[]) { if (shouldLog) { console.log(`Calling ${propertyKey} with arguments: ${args}`); } return originalMethod.apply(this, args); }; return descriptor; }; } class Example { @logConditionally(true) greet(name: string) { return `Hello, ${name}`; } } const example = new Example(); example.greet('TypeScript Developer');
logConditionally 데코레이터에 true를 전달하여 메소드가 실행을 기록하도록 합니다. false를 전달하면 로깅을 건너뜁니다. 이러한 유연성은 데코레이터를 다재다능하고 재사용 가능하게 만드는 핵심입니다.
데코레이터의 실제 응용
데코레이터는 많은 라이브러리와 프레임워크에서 실용적으로 사용되었습니다. 다음은 데코레이터가 복잡한 기능을 간소화하는 방법을 보여주는 몇 가지 주목할만한 예입니다.
- 클래스 유효성 검사기의 유효성 검사: 데이터 기반 애플리케이션에서는 유효성 검사가 중요합니다. 클래스 유효성 검사기 패키지는 데코레이터를 사용하여 TypeScript 클래스의 필드 유효성 검사 프로세스를 단순화합니다.
import { IsEmail, IsNotEmpty } from 'class-validator'; class User { @IsNotEmpty() name: string; @IsEmail() email: string; }
이 예에서 @IsEmail 및 @IsNotEmpty 데코레이터는 이메일 필드가 유효한 이메일 주소이고 이름 필드가 비어 있지 않은지 확인합니다. 이러한 데코레이터는 수동 유효성 검사 논리가 필요하지 않아 시간을 절약합니다.
- TypeORM을 사용한 객체 관계형 매핑: 데코레이터는 TypeScript 클래스를 데이터베이스 테이블에 매핑하기 위해 TypeORM과 같은 ORM 프레임워크에서 널리 사용됩니다. 이 매핑은 데코레이터를 사용하여 선언적으로 수행됩니다.
import { Entity, Column, PrimaryGeneratedColumn } from 'typeorm'; @Entity() class User { @PrimaryGeneratedColumn() id: number; @Column() name: string; @Column() email: string; }
여기서 @Entity, @Column 및 @PrimaryGeneratedColumn은 User 테이블의 구조를 정의합니다. 이러한 데코레이터는 SQL 테이블 생성의 복잡성을 추상화하여 코드를 더 읽기 쉽고 유지 관리하기 쉽게 만듭니다.
- Angular 종속성 주입: Angular에서 데코레이터는 서비스와 구성 요소를 관리하는 데 중추적인 역할을 합니다. @Injectable 데코레이터는 클래스를 다른 구성 요소나 서비스에 주입할 수 있는 서비스로 표시합니다.
@Injectable({ providedIn: 'root', }) class UserService { constructor(private http: HttpClient) {} }
이 경우 @Injectable 데코레이터는 UserService가 전역적으로 제공되어야 함을 Angular의 종속성 주입 시스템에 신호로 보냅니다. 이를 통해 애플리케이션 전반에 걸쳐 서비스를 원활하게 통합할 수 있습니다.
자신만의 데코레이터 구현: 분석
데코레이터의 핵심은 기능일 뿐입니다. 데코레이터를 처음부터 만드는 과정을 자세히 살펴보겠습니다.
Class Decorator
A class decorator receives the constructor of the class and can be used to modify the class prototype or even replace the constructor.
function AddTimestamp(constructor: Function) { constructor.prototype.timestamp = new Date(); } @AddTimestamp class MyClass { id: number; constructor(id: number) { this.id = id; } } const instance = new MyClass(1); console.log(instance.timestamp); // Outputs the current timestamp
Method Decorator
A method decorator modifies the method descriptor, allowing you to alter the behavior of the method itself.
function logExecutionTime(target: any, propertyKey: string, descriptor: PropertyDescriptor) { const originalMethod = descriptor.value; descriptor.value = function (...args: any[]) { const start = performance.now(); const result = originalMethod.apply(this, args); const end = performance.now(); console.log(`${propertyKey} executed in ${end - start}ms`); return result; }; return descriptor; } class Service { @logExecutionTime execute() { // Simulate work for (let i = 0; i < 1e6; i++) {} } } const service = new Service(); service.execute(); // Logs the execution time
Property Decorator
A property decorator allows you to intercept property access and modification, which can be useful for tracking changes.
function trackChanges(target: any, propertyKey: string) { let value = target[propertyKey]; const getter = () => value; const setter = (newValue: any) => { console.log(`${propertyKey} changed from ${value} to ${newValue}`); value = newValue; }; Object.defineProperty(target, propertyKey, { get: getter, set: setter, }); } class Product { @trackChanges price: number; constructor(price: number) { this.price = price; } } const product = new Product(100); product.price = 200; // Logs the change
Conclusion
Decorators in TypeScript allow you to abstract and reuse functionality in a clean, declarative manner. Whether you're working with validation, ORMs, or dependency injection, decorators help reduce boilerplate and keep your code modular and maintainable. Understanding how they work from first principles makes it easier to leverage their full potential and craft custom solutions tailored to your application.
By taking a deeper look at the structure and real-world applications of decorators, you've now seen how they can simplify complex coding tasks and streamline code across various domains.
위 내용은 TypeScript의 데코레이터 이해: 첫 번째 원칙 접근 방식의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
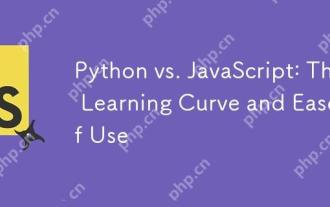
Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
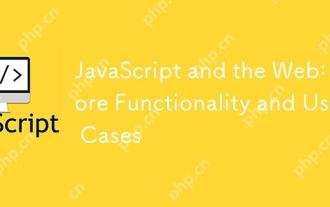
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.
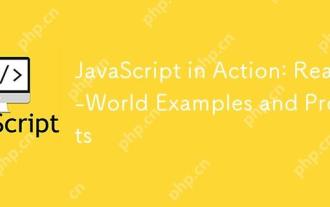
실제 세계에서 JavaScript의 응용 프로그램에는 프론트 엔드 및 백엔드 개발이 포함됩니다. 1) DOM 운영 및 이벤트 처리와 관련된 TODO 목록 응용 프로그램을 구축하여 프론트 엔드 애플리케이션을 표시합니다. 2) Node.js를 통해 RESTFULAPI를 구축하고 Express를 통해 백엔드 응용 프로그램을 시연하십시오.
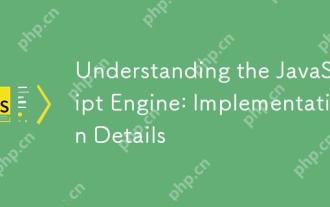
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.
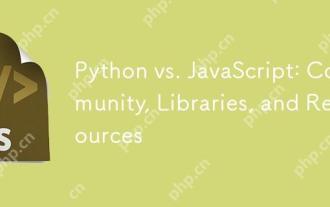
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
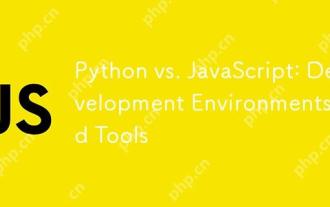
개발 환경에서 Python과 JavaScript의 선택이 모두 중요합니다. 1) Python의 개발 환경에는 Pycharm, Jupyternotebook 및 Anaconda가 포함되어 있으며 데이터 과학 및 빠른 프로토 타이핑에 적합합니다. 2) JavaScript의 개발 환경에는 Node.js, VScode 및 Webpack이 포함되어 있으며 프론트 엔드 및 백엔드 개발에 적합합니다. 프로젝트 요구에 따라 올바른 도구를 선택하면 개발 효율성과 프로젝트 성공률이 향상 될 수 있습니다.
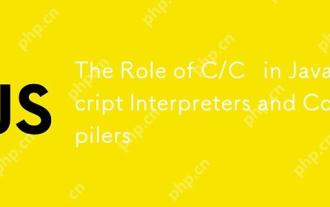
C와 C는 주로 통역사와 JIT 컴파일러를 구현하는 데 사용되는 JavaScript 엔진에서 중요한 역할을합니다. 1) C는 JavaScript 소스 코드를 구문 분석하고 추상 구문 트리를 생성하는 데 사용됩니다. 2) C는 바이트 코드 생성 및 실행을 담당합니다. 3) C는 JIT 컴파일러를 구현하고 런타임에 핫스팟 코드를 최적화하고 컴파일하며 JavaScript의 실행 효율을 크게 향상시킵니다.
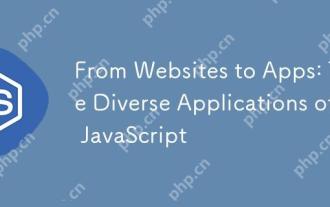
JavaScript는 웹 사이트, 모바일 응용 프로그램, 데스크탑 응용 프로그램 및 서버 측 프로그래밍에서 널리 사용됩니다. 1) 웹 사이트 개발에서 JavaScript는 HTML 및 CSS와 함께 DOM을 운영하여 동적 효과를 달성하고 jQuery 및 React와 같은 프레임 워크를 지원합니다. 2) 반응 및 이온 성을 통해 JavaScript는 크로스 플랫폼 모바일 애플리케이션을 개발하는 데 사용됩니다. 3) 전자 프레임 워크를 사용하면 JavaScript가 데스크탑 애플리케이션을 구축 할 수 있습니다. 4) node.js는 JavaScript가 서버 측에서 실행되도록하고 동시 요청이 높은 높은 요청을 지원합니다.
