Symfony 속성을 사용하여 DTO를 검증하는 쉬운 방법
소개
DTO는 비즈니스 로직을 포함하지 않고 데이터 속성을 캡슐화하는 간단한 개체입니다. 여러 소스의 데이터를 단일 개체로 집계하는 데 자주 사용되므로 관리 및 전송이 더 쉬워집니다. DTO를 사용하면 개발자는 특히 분산 시스템이나 API에서 메소드 호출 수를 줄이고 성능을 향상시키며 데이터 처리를 단순화할 수 있습니다.
예를 들어 DTO를 사용하여 HTTP 요청을 통해 수신된 데이터를 매핑할 수 있습니다. 해당 DTO는 수신된 페이로드 값을 해당 속성에 보유하고 애플리케이션 내에서 이를 사용할 수 있습니다. 예를 들어 DTO에 보관된 데이터에서 데이터베이스에 유지할 준비가 된 교리 엔터티 개체를 생성합니다. DTO 데이터는 이미 검증되었으므로 데이터베이스가 유지되는 동안 오류가 발생할 가능성을 줄일 수 있습니다.
MapQueryString 및 MapRequestPayload 속성
MapQueryString 및 MapRequestPayload 속성을 사용하면 수신된 쿼리 문자열과 페이로드 매개변수를 각각 매핑할 수 있습니다. 두 가지 모두의 예를 살펴보겠습니다.
MapQueryString 예
쿼리 문자열 내에서 다음 매개변수를 수신할 수 있는 Symfony 경로가 있다고 가정해 보겠습니다.
- from: 필수 from 날짜
- to: 필수 데이트
- age: 선택적 연령
위 매개변수를 기반으로 다음 dto에 매핑하려고 합니다.
readonly class QueryInputDto { public function __construct( #[Assert\Datetime(message: 'From must be a valid datetime')] #[Assert\NotBlank(message: 'From date cannot be empty')] public string $from, #[Assert\Datetime(message: 'To must be a valid datetime')] #[Assert\NotBlank(message: 'To date cannot be empty')] public string $to, public ?int $age = null ){} }
이를 매핑하려면 다음과 같은 방법으로 MapQueryString 속성만 사용해야 합니다.
#[Route('/my-get-route', name: 'my_route_name', methods: ['GET'])] public function myAction(#[MapQueryString] QueryInputDTO $queryInputDto) { // your code }
보시다시피, Symfony는 $queryInputDto 인수가 #[MapQueryString] 속성으로 플래그 지정되었음을 감지하면 수신된 쿼리 문자열 매개변수를 해당 인수에 자동으로 매핑합니다. QueryInputDTO 클래스.
MapRequestPayload 예시
이 경우 JSON 요청 페이로드 내에서 새 사용자를 등록하는 데 필요한 데이터를 수신하는 Symfony 경로가 있다고 가정해 보겠습니다. 해당 매개변수는 다음과 같습니다.
- 이름: 필수
- 이메일: 필수
- 생년월일(dob): 필수
위 매개변수를 기반으로 다음 dto에 매핑하려고 합니다.
readonly class PayloadInputDto { public function __construct( #[Assert\NotBlank(message: 'Name cannot be empty')] public string $name, #[Assert\NotBlank(message: 'Email cannot be empty')] #[Assert\Email(message: 'Email must be a valid email')] public string $email, #[Assert\NotBlank(message: 'From date cannot be empty')] #[Assert\Date(message: 'Dob must be a valid date')] public ?string $dob = null ){} }
매핑하려면 다음과 같이 MapRequestPayload 속성만 사용해야 합니다.
#[Route('/my-post-route', name: 'my_post_route', methods: ['POST'])] public function myAction(#[MapRequestPayload] PayloadInputDTO $payloadInputDto) { // your code }
이전 섹션에서 살펴본 것처럼 Symfony는 $payloadInputDto 인수가 #[MapRequestPayload] 속성으로 플래그 지정되었음을 감지하면 수신된 페이로드 매개변수를 해당 인수에 자동으로 매핑합니다. PayloadInputDTO 클래스
의 인스턴스MapRequestPayload는 JSON 페이로드와 양식 URL로 인코딩된 페이로드 모두에서 작동합니다.
DTO 유효성 검사 오류 처리
매핑 프로세스 중에 유효성 검사가 실패하는 경우(예: 필수 이메일이 전송되지 않은 경우) Symfony는 422 처리할 수 없는 콘텐츠 예외를 발생시킵니다. 이러한 종류의 예외를 포착하고 클라이언트에 json과 같은 유효성 검사 오류를 반환하려면 이벤트 구독자를 생성하고 KernelException 이벤트를 계속 수신 대기하면 됩니다.
class KernelSubscriber implements EventSubscriberInterface { public static function getSubscribedEvents(): array { return [ KernelEvents::EXCEPTION => 'onException' ]; } public function onException(ExceptionEvent $event): void { $exception = $event->getThrowable(); if($exception instanceof UnprocessableEntityHttpException) { $previous = $exception->getPrevious(); if($previous instanceof ValidationFailedException) { $errors = []; foreach($previous->getViolations() as $violation) { $errors[] = [ 'path' => $violation->getPropertyPath(), 'error' => $violation->getMessage() ]; } $event->setResponse(new JsonResponse($errors)); } } } }
onException 메서드가 트리거된 후 이벤트 예외가 UnprocessableEntityHttpException의 인스턴스인지 확인합니다. 그렇다면 이전 예외가 ValidationFailedException 클래스의 인스턴스인지 확인하면서 처리할 수 없는 오류가 실패한 유효성 검사에서 발생한 것인지도 확인합니다. 그렇다면 모든 위반 오류를 배열에 저장하고(속성 경로만 키로, 위반 메시지만 오류로) 이러한 오류로부터 JSON 응답을 생성하고 이벤트에 새 응답을 설정합니다.
다음 이미지는 이메일이 전송되지 않아 실패한 요청에 대한 JSON 응답을 보여줍니다.
@baseUrl = http://127.0.0.1:8000 POST {{baseUrl}}/my-post-route Content-Type: application/json { "name" : "Peter Parker", "email" : "", "dob" : "1990-06-28" } ------------------------------------------------------------- HTTP/1.1 422 Unprocessable Entity Cache-Control: no-cache, private Content-Type: application/json Date: Fri, 20 Sep 2024 16:44:20 GMT Host: 127.0.0.1:8000 X-Powered-By: PHP/8.2.23 X-Robots-Tag: noindex Transfer-Encoding: chunked [ { "path": "email", "error": "Email cannot be empty" } ]
위 이미지 요청은 http 파일을 사용하여 생성되었습니다.
사용자 정의 리졸버 만들기
쿼리 문자열 매개변수를 "f"라는 배열로 수신하는 경로가 있다고 가정해 보겠습니다. 다음과 같습니다:
/my-get-route?f[from]=2024-08-20 16:24:08&f[to]=&f[age]=14
요청에서 해당 배열을 확인한 다음 데이터의 유효성을 검사하는 사용자 지정 확인자를 만들 수 있습니다. 코딩해 보겠습니다.
use Symfony\Component\EventDispatcher\EventSubscriberInterface; use Symfony\Component\HttpFoundation\Request; use Symfony\Component\HttpKernel\Attribute\MapQueryString; use Symfony\Component\HttpKernel\Controller\ValueResolverInterface; use Symfony\Component\HttpKernel\ControllerMetadata\ArgumentMetadata; use Symfony\Component\HttpKernel\Event\ControllerArgumentsEvent; use Symfony\Component\HttpKernel\Exception\UnprocessableEntityHttpException; use Symfony\Component\HttpKernel\KernelEvents; use Symfony\Component\Serializer\SerializerInterface; use Symfony\Component\Validator\Exception\ValidationFailedException; use Symfony\Component\Validator\Validator\ValidatorInterface; class CustomQsValueResolver implements ValueResolverInterface, EventSubscriberInterface { public function __construct( private readonly ValidatorInterface $validator, private readonly SerializerInterface $serializer ){} public static function getSubscribedEvents(): array { return [ KernelEvents::CONTROLLER_ARGUMENTS => 'onKernelControllerArguments', ]; } public function resolve(Request $request, ArgumentMetadata $argument): iterable { $attribute = $argument->getAttributesOfType(MapQueryString::class, ArgumentMetadata::IS_INSTANCEOF)[0] ?? null; if (!$attribute) { return []; } if ($argument->isVariadic()) { throw new \LogicException(sprintf('Mapping variadic argument "$%s" is not supported.', $argument->getName())); } $attribute->metadata = $argument; return [$attribute]; } public function onKernelControllerArguments(ControllerArgumentsEvent $event): void { $arguments = $event->getArguments(); $request = $event->getRequest(); foreach ($arguments as $i => $argument) { if($argument instanceof MapQueryString ) { $qs = $request->get('f', []); if(count($qs) > 0) { $object = $this->serializer->denormalize($qs, $argument->metadata->getType()); $violations = $this->validator->validate($object); if($violations->count() > 0) { $validationFailedException = new ValidationFailedException(null, $violations); throw new UnprocessableEntityHttpException('Unale to process received data', $validationFailedException); } $arguments[$i] = $object; } } } $event->setArguments($arguments); } }
The CustomQsValueResolver implements the ValueResolverInterface and the EventSubscriberInterface, allowing it to resolve arguments for controller actions and listen to specific events in the Symfony event system. In this case, the resolver listens to the Kernel CONTROLLER_ARGUMENTS event.
Let's analyze it step by step:
The constructor
The constructor takes two dependencies: The Validator service for validating objects and a the Serializer service for denormalizing data into objects.
The getSubscribedEvents method
The getSubscribedEvents method returns an array mapping the KernelEvents::CONTROLLER_ARGUMENTS symfony event to the onKernelControllerArguments method. This means that when the CONTROLLER_ARGUMENTS event is triggered (always a controller is reached), the onKernelControllerArguments method will be called.
The resolve method
The resolve method is responsible for resolving the value of an argument based on the request and its metadata.
- It checks if the argument has the MapQueryString attribute. If not, it returns an empty array.
- If the argument is variadic, that is, it can accept a variable number of arguments, it throws a LogicException, indicating that mapping variadic arguments is not supported.
- If the attribute is found, it sets the metadata property of the attribute and returns it as a php iterable.
The onKernelControllerArguments method
The onKernelControllerArguments method is called when the CONTROLLER_ARGUMENTS event is triggered.
- It retrieves the current arguments and the request from the event.
- It iterates over the arguments, checking for arguments flagged as MapQueryString
- If found, it retrieves the query string parameters holded by the "f" array using $request->get('f', []).
- If there are parameters, it denormalizes them into an object of the type specified in the argument's metadata (The Dto class).
- It then validates the object using the validator. If there are validation violations, it throws an UnprocessableEntityHttpException which wraps a ValidationFailedException with the validation errors.
- If validation passes, it replaces the original argument with the newly created object.
Using the resolver in the controller
To instruct the MapQueryString attribute to use our recently created resolver instead of the default one, we must specify it with the attribute resolver value. Let's see how to do it:
#[Route('/my-get-route', name: 'my_route_name', methods: ['GET'])] public function myAction(#[MapQueryString(resolver: CustomQsValueResolver::class)] QueryInputDTO $queryInputDto) { // your code }
Conclusion
In this article, we have analized how symfony makes our lives easier by making common application tasks very simple, such as receiving and validating data from an API. To do that, it offers us the MapQueryString and MapRequestPayload attributes. In addition, it also offers us the possibility of creating our custom mapping resolvers for cases that require specific needs.
If you like my content and enjoy reading it and you are interested in learning more about PHP, you can read my ebook about how to create an operation-oriented API using PHP and the Symfony Framework. You can find it here: Building an Operation-Oriented Api using PHP and the Symfony Framework: A step-by-step guide
위 내용은 Symfony 속성을 사용하여 DTO를 검증하는 쉬운 방법의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










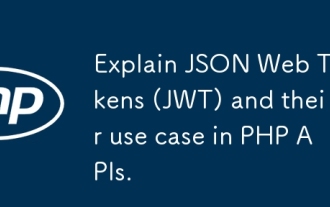
JWT는 주로 신분증 인증 및 정보 교환을 위해 당사자간에 정보를 안전하게 전송하는 데 사용되는 JSON을 기반으로 한 개방형 표준입니다. 1. JWT는 헤더, 페이로드 및 서명의 세 부분으로 구성됩니다. 2. JWT의 작업 원칙에는 세 가지 단계가 포함됩니다. JWT 생성, JWT 확인 및 Parsing Payload. 3. PHP에서 인증에 JWT를 사용하면 JWT를 생성하고 확인할 수 있으며 사용자 역할 및 권한 정보가 고급 사용에 포함될 수 있습니다. 4. 일반적인 오류에는 서명 검증 실패, 토큰 만료 및 대형 페이로드가 포함됩니다. 디버깅 기술에는 디버깅 도구 및 로깅 사용이 포함됩니다. 5. 성능 최적화 및 모범 사례에는 적절한 시그니처 알고리즘 사용, 타당성 기간 설정 합리적,

PHP8.1의 열거 기능은 명명 된 상수를 정의하여 코드의 명확성과 유형 안전성을 향상시킵니다. 1) 열거는 정수, 문자열 또는 객체 일 수 있으며 코드 가독성 및 유형 안전성을 향상시킬 수 있습니다. 2) 열거는 클래스를 기반으로하며 Traversal 및 Reflection과 같은 객체 지향적 특징을 지원합니다. 3) 열거는 유형 안전을 보장하기 위해 비교 및 할당에 사용될 수 있습니다. 4) 열거는 복잡한 논리를 구현하는 방법을 추가하는 것을 지원합니다. 5) 엄격한 유형 확인 및 오류 처리는 일반적인 오류를 피할 수 있습니다. 6) 열거는 마법의 가치를 줄이고 유지 관리를 향상 시키지만 성능 최적화에주의를 기울입니다.
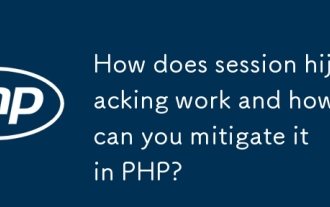
세션 납치는 다음 단계를 통해 달성 할 수 있습니다. 1. 세션 ID를 얻으십시오. 2. 세션 ID 사용, 3. 세션을 활성 상태로 유지하십시오. PHP에서 세션 납치를 방지하는 방법에는 다음이 포함됩니다. 1. 세션 _regenerate_id () 함수를 사용하여 세션 ID를 재생산합니다. 2. 데이터베이스를 통해 세션 데이터를 저장하십시오.
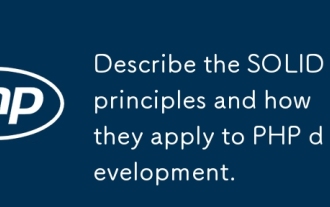
PHP 개발에서 견고한 원칙의 적용에는 다음이 포함됩니다. 1. 단일 책임 원칙 (SRP) : 각 클래스는 하나의 기능 만 담당합니다. 2. Open and Close Principle (OCP) : 변경은 수정보다는 확장을 통해 달성됩니다. 3. Lisch의 대체 원칙 (LSP) : 서브 클래스는 프로그램 정확도에 영향을 미치지 않고 기본 클래스를 대체 할 수 있습니다. 4. 인터페이스 격리 원리 (ISP) : 의존성 및 사용되지 않은 방법을 피하기 위해 세밀한 인터페이스를 사용하십시오. 5. 의존성 반전 원리 (DIP) : 높고 낮은 수준의 모듈은 추상화에 의존하며 종속성 주입을 통해 구현됩니다.
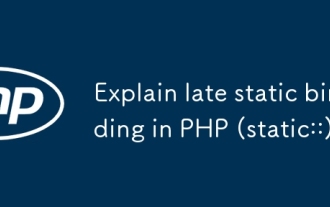
정적 바인딩 (정적 : :)는 PHP에서 늦은 정적 바인딩 (LSB)을 구현하여 클래스를 정의하는 대신 정적 컨텍스트에서 호출 클래스를 참조 할 수 있습니다. 1) 구문 분석 프로세스는 런타임에 수행됩니다. 2) 상속 관계에서 통화 클래스를 찾아보십시오. 3) 성능 오버 헤드를 가져올 수 있습니다.
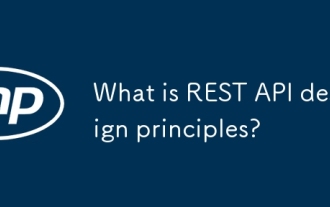
RESTAPI 설계 원칙에는 자원 정의, URI 설계, HTTP 방법 사용, 상태 코드 사용, 버전 제어 및 증오가 포함됩니다. 1. 자원은 명사로 표현되어야하며 계층 구조로 유지해야합니다. 2. HTTP 방법은 Get이 자원을 얻는 데 사용되는 것과 같은 의미론을 준수해야합니다. 3. 404와 같이 상태 코드는 올바르게 사용해야합니다. 자원이 존재하지 않음을 의미합니다. 4. 버전 제어는 URI 또는 헤더를 통해 구현할 수 있습니다. 5. 증오는 응답으로 링크를 통한 클라이언트 작업을 부팅합니다.
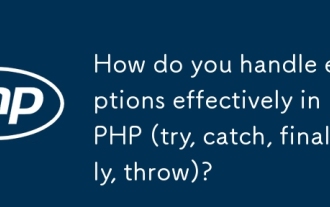
PHP에서는 시도, 캐치, 마지막으로 키워드를 통해 예외 처리가 이루어집니다. 1) 시도 블록은 예외를 던질 수있는 코드를 둘러싸고 있습니다. 2) 캐치 블록은 예외를 처리합니다. 3) 마지막으로 블록은 코드가 항상 실행되도록합니다. 4) 던지기는 수동으로 예외를 제외하는 데 사용됩니다. 이러한 메커니즘은 코드의 견고성과 유지 관리를 향상시키는 데 도움이됩니다.
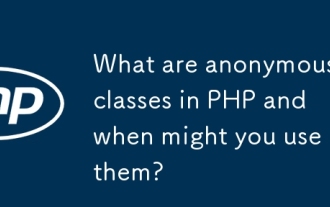
PHP에서 익명 클래스의 주요 기능은 일회성 객체를 만드는 것입니다. 1. 익명 클래스를 사용하면 이름이없는 클래스가 코드에 직접 정의 될 수 있으며, 이는 임시 요구 사항에 적합합니다. 2. 클래스를 상속하거나 인터페이스를 구현하여 유연성을 높일 수 있습니다. 3. 사용할 때 성능 및 코드 가독성에주의를 기울이고 동일한 익명 클래스를 반복적으로 정의하지 마십시오.
