React를 사용하여 멀티플레이어 체스 게임을 구축하는 방법 알아보기
안녕하세요. 환영합니다! ??
오늘 저는 SuperViz를 사용하여 멀티플레이어 체스 게임을 구축하는 방법을 안내하는 튜토리얼을 가져왔습니다. 멀티플레이어 게임에는 플레이어 간 실시간 동기화와 상호 작용이 필요하며 SuperViz 기능을 활용하는 이상적인 애플리케이션입니다.
이 튜토리얼에서는 두 플레이어가 실시간으로 서로 대결하여 서로의 움직임을 볼 수 있는 체스 게임을 만드는 방법을 보여줍니다.
react-chessboard 라이브러리를 사용하여 체스판을 설정하고, chess.js로 게임 상태를 관리하고, SuperViz로 플레이어 동작을 동기화하는 방법을 시연해 보겠습니다. 이 설정을 통해 여러 참가자가 체스 게임에 참여하고, 움직이고, 원활하고 대화형 체스 게임 환경을 경험할 수 있습니다. 시작해 보세요!
전제 조건
이 튜토리얼을 따르려면 SuperViz 계정과 개발자 토큰이 필요합니다. 이미 계정과 개발자 토큰이 있다면 다음 단계로 넘어갈 수 있습니다.
계정 만들기
계정을 만들려면 SuperViz 등록으로 이동하여 Google이나 이메일/비밀번호를 사용하여 계정을 만드세요. 이메일/비밀번호를 사용할 때 계정 확인을 위해 클릭해야 하는 확인 링크를 받게 된다는 점에 유의하는 것이 중요합니다.
개발자 토큰 검색
SDK를 사용하려면 개발자 토큰을 제공해야 합니다. 이 토큰은 SDK 요청을 계정과 연결하는 데 필수적입니다. 대시보드에서 개발 및 프로덕션 SuperViz 토큰을 모두 검색할 수 있습니다. 이 튜토리얼의 다음 단계에서 필요하므로 개발자 토큰을 복사하여 저장하세요.
1단계: React 애플리케이션 설정
시작하려면 SuperViz를 통합할 새 React 프로젝트를 설정해야 합니다.
1. 새로운 React 프로젝트 생성
먼저 Create React App with TypeScript를 사용하여 새로운 React 애플리케이션을 만듭니다.
npm create vite@latest chess-game -- --template react-ts cd chess-game
2. 필수 라이브러리 설치
다음으로 프로젝트에 필요한 라이브러리를 설치합니다.
npm install @superviz/sdk react-chessboard chess.js uuid
- @superviz/sdk: 동기화를 포함한 실시간 협업 기능을 통합하기 위한 SDK
- react-chessboard: React 애플리케이션에서 체스판을 렌더링하기 위한 라이브러리입니다.
- chess.js: 체스 게임 논리 및 규칙을 관리하기 위한 라이브러리입니다.
- uuid: 고유한 참가자 ID를 생성하는 데 유용한 고유 식별자 생성용 라이브러리입니다.
3. 순풍 구성
이 튜토리얼에서는 Tailwind CSS 프레임워크를 사용합니다. 먼저 tailwind 패키지를 설치하세요.
npm install -D tailwindcss postcss autoprefixer npx tailwindcss init -p
그런 다음 템플릿 경로를 구성해야 합니다. 프로젝트 루트에서 tailwind.config.js를 열고 다음 코드를 삽입하세요.
/** @type {import('tailwindcss').Config} */ export default { content: [ "./index.html", "./src/**/*.{js,ts,jsx,tsx}", ], theme: { extend: {}, }, plugins: [], }
그런 다음 tailwind 지시문을 전역 CSS 파일에 추가해야 합니다. (src/index.css)
@tailwind base; @tailwind components; @tailwind utilities;
4. 환경 변수 설정
프로젝트 루트에 .env 파일을 만들고 SuperViz 개발자 키를 추가하세요. 이 키는 SuperViz 서비스로 애플리케이션을 인증하는 데 사용됩니다.
VITE_SUPERVIZ_API_KEY=YOUR_SUPERVIZ_DEVELOPER_KEY
2단계: 메인 애플리케이션 구현
이 단계에서는 SuperViz를 초기화하고 실시간 체스 동작을 처리하는 기본 애플리케이션 로직을 구현하겠습니다.
1. 앱 구성 요소 구현
src/App.tsx를 열고 SuperViz를 사용하여 기본 애플리케이션 구성 요소를 설정하여 공동 작업 환경을 관리하세요.
import { v4 as generateId } from 'uuid'; import { useCallback, useEffect, useRef, useState } from "react"; import SuperVizRoom, { Realtime, RealtimeComponentEvent, RealtimeMessage, WhoIsOnline } from '@superviz/sdk'; import { Chessboard } from "react-chessboard"; import { Chess, Square } from 'chess.js';
설명:
- 가져오기: 상태 관리, SuperViz 초기화, 체스판 렌더링 및 고유 식별자 생성을 위해 React, SuperViz SDK, React-chessboard, chess.js 및 UUID에서 필요한 구성 요소를 가져옵니다.
2. 상수 정의
API 키, 룸 ID, 플레이어 ID에 대한 상수를 정의하세요.
const apiKey = import.meta.env.VITE_SUPERVIZ_API_KEY as string; const ROOM_ID = 'chess-game'; const PLAYER_ID = generateId();
설명:
- apiKey: 환경 변수에서 SuperViz API 키를 검색합니다.
- ROOM_ID: SuperViz 세션의 회의실 ID를 정의합니다.
- PLAYER_ID: uuid 라이브러리를 사용하여 고유한 플레이어 ID를 생성합니다.
3. 체스 메시지 유형 정의
체스 이동 메시지를 처리하기 위한 유형을 만듭니다.
type ChessMessageUpdate = RealtimeMessage & { data: { sourceSquare: Square; targetSquare: Square; }; };
설명:
- ChessMessageUpdate: 체스 이동에 대한 소스 및 대상 사각형을 포함하도록 RealtimeMessage를 확장합니다.
4. 앱 구성요소 생성
메인 App 구성 요소를 설정하고 상태 변수를 초기화합니다.
export default function App() { const [initialized, setInitialized] = useState(false); const [gameState, setGameState] = useState<Chess>(new Chess()); const [gameFen, setGameFen] = useState<string>(gameState.fen()); const channel = useRef<any | null>(null);
설명:
- initialized: A state variable to track whether the SuperViz environment has been set up.
- gameState: A state variable to manage the chess game state using the chess.js library.
- gameFen: A state variable to store the FEN (Forsyth-Edwards Notation) string representing the current game position.
- channel: A ref to store the real-time communication channel.
5. Initialize SuperViz and Real-Time Components
Create an initialize function to set up the SuperViz environment and configure real-time synchronization.
const initialize = useCallback(async () => { if (initialized) return; const superviz = await SuperVizRoom(apiKey, { roomId: ROOM_ID, participant: { id: PLAYER_ID, name: 'player-name', }, group: { id: 'chess-game', name: 'chess-game', } }); const realtime = new Realtime(); const whoIsOnline = new WhoIsOnline(); superviz.addComponent(realtime); superviz.addComponent(whoIsOnline); setInitialized(true); realtime.subscribe(RealtimeComponentEvent.REALTIME_STATE_CHANGED, () => { channel.current = realtime.connect('move-topic'); channel.current.subscribe('new-move', handleRealtimeMessage); }); }, [handleRealtimeMessage, initialized]);
Explanation:
- initialize: An asynchronous function that initializes the SuperViz room and checks if it's already initialized to prevent duplicate setups.
- SuperVizRoom: Configures the room, participant, and group details for the session.
- Realtime Subscription: Connects to the move-topic channel and listens for new moves, updating the local state accordingly.
6. Handle Chess Moves
Create a function to handle chess moves and update the game state.
const makeMove = useCallback((sourceSquare: Square, targetSquare: Square) => { try { const gameCopy = gameState; gameCopy.move({ from: sourceSquare, to: targetSquare, promotion: 'q' }); setGameState(gameCopy); setGameFen(gameCopy.fen()); return true; } catch (error) { console.log('Invalid Move', error); return false; } }, [gameState]);
Explanation:
- makeMove: Attempts to make a move on the chessboard, updating the game state and FEN string if the move is valid.
- Promotion: Automatically promotes a pawn to a queen if it reaches the last rank.
7. Handle Piece Drop
Create a function to handle piece drop events on the chessboard.
const onPieceDrop = (sourceSquare: Square, targetSquare: Square) => { const result = makeMove(sourceSquare, targetSquare); if (result) { channel.current.publish('new-move', { sourceSquare, targetSquare, }); } return result; };
Explanation:
- onPieceDrop: Handles the logic for when a piece is dropped on a new square, making the move and publishing it to the SuperViz channel if valid.
8. Handle Real-Time Messages
Create a function to handle incoming real-time messages for moves made by other players.
const handleRealtimeMessage = useCallback((message: ChessMessageUpdate) => { if (message.participantId === PLAYER_ID) return; const { sourceSquare, targetSquare } = message.data; makeMove(sourceSquare, targetSquare); }, [makeMove]);
Explanation:
- handleRealtimeMessage: Listens for incoming move messages and updates the game state if the move was made by another participant.
9. Use Effect Hook for Initialization
Use the useEffect hook to trigger the initialize function on component mount.
useEffect(() => { initialize(); }, [initialize]);
Explanation:
- useEffect: Calls the initialize function once when the component mounts, setting up the SuperViz environment and real-time synchronization.
10. Render the Application
Return the JSX structure for rendering the application, including the chessboard and collaboration features.
return ( <div className='w-full h-full bg-gray-200 flex items-center justify-center flex-col'> <header className='w-full p-5 bg-purple-400 flex items-center justify-between'> <h1 className='text-white text-2xl font-bold'>SuperViz Chess Game</h1> </header> <main className='w-full h-full flex items-center justify-center'> <div className='w-[500px] h-[500px] shadow-sm border-2 border-gray-300 rounded-md'> <Chessboard position={gameFen} onPieceDrop={onPieceDrop} /> <div className='w-[500px] h-[50px] bg-gray-300 flex items-center justify-center'> <p className='text-gray-800 text-2xl font-bold'>Turn: {gameState.turn() === 'b' ? 'Black' : 'White'}</p> </div> </div> </main> </div> );
Explanation:
- Header: Displays the title of the application.
- Chessboard: Renders the chessboard using the Chessboard component, with gameFen as the position and onPieceDrop as the event handler for piece drops.
- Turn Indicator: Displays the current player's turn (Black or White).
Step 3: Understanding the Project Structure
Here's a quick overview of how the project structure supports a multiplayer chess game:
- App.tsx
- Initializes the SuperViz environment.
- Sets up participant information and room details.
- Handles real-time synchronization for chess moves.
- Chessboard
- Displays the chessboard and manages piece movements.
- Integrates real-time communication to synchronize moves between players.
- Chess Logic
- Uses chess.js to manage game rules and validate moves.
- Updates the game state and FEN string to reflect the current board position.
Step 4: Running the Application
1. Start the React Application
To run your application, use the following command in your project directory:
npm run dev
This command will start the development server and open your application in the default web browser. You can interact with the chessboard and see moves in real-time as other participants join the session.
2. Testez l'application
- Mouvements d'échecs en temps réel : Ouvrez l'application dans plusieurs fenêtres ou onglets du navigateur pour simuler plusieurs participants et vérifiez que les mouvements effectués par un joueur sont reflétés en temps réel pour les autres.
- Interaction collaborative : Testez la réactivité de l'application en effectuant des mouvements et en observant comment l'état du jeu se met à jour pour tous les participants.
Résumé
Dans ce tutoriel, nous avons construit un jeu d'échecs multijoueur en utilisant SuperViz pour la synchronisation en temps réel. Nous avons configuré une application React pour gérer les mouvements d'échecs, permettant à plusieurs joueurs de collaborer de manière transparente sur un échiquier partagé. Cette configuration peut être étendue et personnalisée pour s'adapter à divers scénarios où une interaction de jeu est requise.
N'hésitez pas à explorer le code complet et d'autres exemples dans le référentiel GitHub pour plus de détails.
위 내용은 React를 사용하여 멀티플레이어 체스 게임을 구축하는 방법 알아보기의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
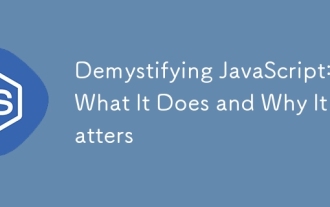
JavaScript는 현대 웹 개발의 초석이며 주요 기능에는 이벤트 중심 프로그래밍, 동적 컨텐츠 생성 및 비동기 프로그래밍이 포함됩니다. 1) 이벤트 중심 프로그래밍을 사용하면 사용자 작업에 따라 웹 페이지가 동적으로 변경 될 수 있습니다. 2) 동적 컨텐츠 생성을 사용하면 조건에 따라 페이지 컨텐츠를 조정할 수 있습니다. 3) 비동기 프로그래밍은 사용자 인터페이스가 차단되지 않도록합니다. JavaScript는 웹 상호 작용, 단일 페이지 응용 프로그램 및 서버 측 개발에 널리 사용되며 사용자 경험 및 크로스 플랫폼 개발의 유연성을 크게 향상시킵니다.
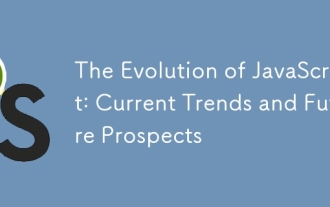
JavaScript의 최신 트렌드에는 Typescript의 Rise, 현대 프레임 워크 및 라이브러리의 인기 및 WebAssembly의 적용이 포함됩니다. 향후 전망은보다 강력한 유형 시스템, 서버 측 JavaScript 개발, 인공 지능 및 기계 학습의 확장, IoT 및 Edge 컴퓨팅의 잠재력을 포함합니다.
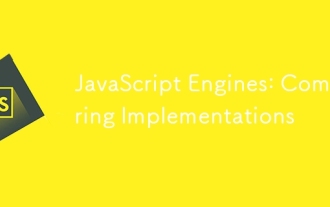
각각의 엔진의 구현 원리 및 최적화 전략이 다르기 때문에 JavaScript 엔진은 JavaScript 코드를 구문 분석하고 실행할 때 다른 영향을 미칩니다. 1. 어휘 분석 : 소스 코드를 어휘 단위로 변환합니다. 2. 문법 분석 : 추상 구문 트리를 생성합니다. 3. 최적화 및 컴파일 : JIT 컴파일러를 통해 기계 코드를 생성합니다. 4. 실행 : 기계 코드를 실행하십시오. V8 엔진은 즉각적인 컴파일 및 숨겨진 클래스를 통해 최적화하여 Spidermonkey는 유형 추론 시스템을 사용하여 동일한 코드에서 성능이 다른 성능을 제공합니다.
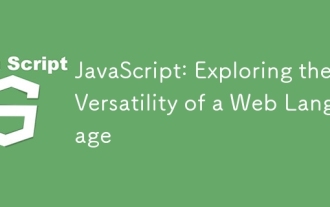
JavaScript는 현대 웹 개발의 핵심 언어이며 다양성과 유연성에 널리 사용됩니다. 1) 프론트 엔드 개발 : DOM 운영 및 최신 프레임 워크 (예 : React, Vue.js, Angular)를 통해 동적 웹 페이지 및 단일 페이지 응용 프로그램을 구축합니다. 2) 서버 측 개발 : Node.js는 비 차단 I/O 모델을 사용하여 높은 동시성 및 실시간 응용 프로그램을 처리합니다. 3) 모바일 및 데스크탑 애플리케이션 개발 : 크로스 플랫폼 개발은 개발 효율을 향상시키기 위해 반응 및 전자를 통해 실현됩니다.
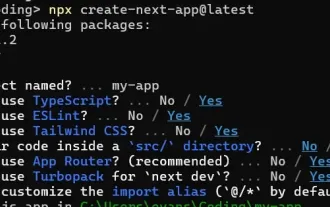
이 기사에서는 Contrim에 의해 확보 된 백엔드와의 프론트 엔드 통합을 보여 주며 Next.js를 사용하여 기능적인 Edtech SaaS 응용 프로그램을 구축합니다. Frontend는 UI 가시성을 제어하기 위해 사용자 권한을 가져오고 API가 역할 기반을 준수하도록합니다.
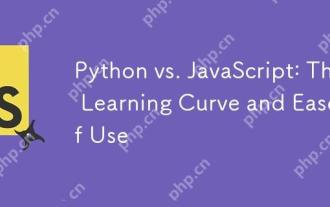
Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
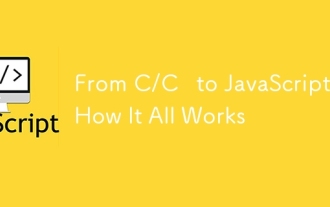
C/C에서 JavaScript로 전환하려면 동적 타이핑, 쓰레기 수집 및 비동기 프로그래밍으로 적응해야합니다. 1) C/C는 수동 메모리 관리가 필요한 정적으로 입력 한 언어이며 JavaScript는 동적으로 입력하고 쓰레기 수집이 자동으로 처리됩니다. 2) C/C를 기계 코드로 컴파일 해야하는 반면 JavaScript는 해석 된 언어입니다. 3) JavaScript는 폐쇄, 프로토 타입 체인 및 약속과 같은 개념을 소개하여 유연성과 비동기 프로그래밍 기능을 향상시킵니다.
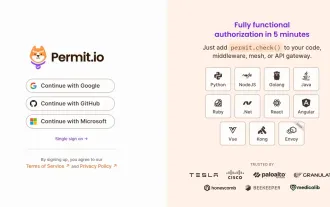
일상적인 기술 도구를 사용하여 기능적 다중 테넌트 SaaS 응용 프로그램 (Edtech 앱)을 구축했으며 동일한 작업을 수행 할 수 있습니다. 먼저, 다중 테넌트 SaaS 응용 프로그램은 무엇입니까? 멀티 테넌트 SAAS 응용 프로그램은 노래에서 여러 고객에게 서비스를 제공 할 수 있습니다.
