Python에서 MRZ(기계 판독 가능 영역) 인식을 구현하는 방법
機械読み取り可能ゾーン (MRZ) は、現代のパスポート、ビザ、ID カードに採用されている重要な機能です。これには、名前、性別、国コード、文書番号など、文書所有者に関する重要な情報が含まれています。 MRZ の認識は、国境管理、空港のセキュリティ、ホテルのチェックイン プロセスにおいて重要な役割を果たします。このチュートリアルでは、Dynamsoft Capture Vision SDK を利用して、Windows、Linux、および macOS に MRZ 認識を実装する方法を示します。プラットフォーム。このガイドでは、SDK の強力な機能を活用し、クロスプラットフォームの MRZ 検出をシームレスかつ効率的に行うための段階的なアプローチを提供します。
macOS での Python MRZ 認識デモ
前提条件
Dynamsoft Capture Vision トライアル ライセンス: Dynamsoft Capture Vision SDK の 30 日間のトライアル ライセンス キーを取得します。
-
Python パッケージ: 次のコマンドを使用して、必要な Python パッケージをインストールします。
pip install dynamsoft-capture-vision-bundle opencv-python
로그인 후 복사これらのパッケージは何のためにありますか?
- dynamsoft-capture-vision-bundle は、Python 用 Dynamsoft Capture Vision SDK です。
- opencv-python はカメラ フレームをキャプチャし、処理された画像結果を表示します。
Dynamsoft Python Capture Vision サンプルの開始
公式 MRZ スキャナーのサンプルは、Dynamsoft Capture Vision SDK を使用して簡単な Python ベースの MRZ リーダーを短時間で作成する方法を示しています。
ソース コードを見て、その機能を分析してみましょう:
import sys from dynamsoft_capture_vision_bundle import * import os class MRZResult: def __init__(self, item: ParsedResultItem): self.doc_type = item.get_code_type() self.raw_text=[] self.doc_id = None self.surname = None self.given_name = None self.nationality = None self.issuer = None self.gender = None self.date_of_birth = None self.date_of_expiry = None if self.doc_type == "MRTD_TD3_PASSPORT": if item.get_field_value("passportNumber") != None and item.get_field_validation_status("passportNumber") != EnumValidationStatus.VS_FAILED: self.doc_id = item.get_field_value("passportNumber") elif item.get_field_value("documentNumber") != None and item.get_field_validation_status("documentNumber") != EnumValidationStatus.VS_FAILED: self.doc_id = item.get_field_value("documentNumber") line = item.get_field_value("line1") if line is not None: if item.get_field_validation_status("line1") == EnumValidationStatus.VS_FAILED: line += ", Validation Failed" self.raw_text.append(line) line = item.get_field_value("line2") if line is not None: if item.get_field_validation_status("line2") == EnumValidationStatus.VS_FAILED: line += ", Validation Failed" self.raw_text.append(line) line = item.get_field_value("line3") if line is not None: if item.get_field_validation_status("line3") == EnumValidationStatus.VS_FAILED: line += ", Validation Failed" self.raw_text.append(line) if item.get_field_value("nationality") != None and item.get_field_validation_status("nationality") != EnumValidationStatus.VS_FAILED: self.nationality = item.get_field_value("nationality") if item.get_field_value("issuingState") != None and item.get_field_validation_status("issuingState") != EnumValidationStatus.VS_FAILED: self.issuer = item.get_field_value("issuingState") if item.get_field_value("dateOfBirth") != None and item.get_field_validation_status("dateOfBirth") != EnumValidationStatus.VS_FAILED: self.date_of_birth = item.get_field_value("dateOfBirth") if item.get_field_value("dateOfExpiry") != None and item.get_field_validation_status("dateOfExpiry") != EnumValidationStatus.VS_FAILED: self.date_of_expiry = item.get_field_value("dateOfExpiry") if item.get_field_value("sex") != None and item.get_field_validation_status("sex") != EnumValidationStatus.VS_FAILED: self.gender = item.get_field_value("sex") if item.get_field_value("primaryIdentifier") != None and item.get_field_validation_status("primaryIdentifier") != EnumValidationStatus.VS_FAILED: self.surname = item.get_field_value("primaryIdentifier") if item.get_field_value("secondaryIdentifier") != None and item.get_field_validation_status("secondaryIdentifier") != EnumValidationStatus.VS_FAILED: self.given_name = item.get_field_value("secondaryIdentifier") def to_string(self): msg = (f"Raw Text:\n") for index, line in enumerate(self.raw_text): msg += (f"\tLine {index + 1}: {line}\n") msg+=(f"Parsed Information:\n" f"\tDocumentType: {self.doc_type or ''}\n" f"\tDocumentID: {self.doc_id or ''}\n" f"\tSurname: {self.surname or ''}\n" f"\tGivenName: {self.given_name or ''}\n" f"\tNationality: {self.nationality or ''}\n" f"\tIssuingCountryorOrganization: {self.issuer or ''}\n" f"\tGender: {self.gender or ''}\n" f"\tDateofBirth(YYMMDD): {self.date_of_birth or ''}\n" f"\tExpirationDate(YYMMDD): {self.date_of_expiry or ''}\n") return msg def print_results(result: ParsedResult) -> None: tag = result.get_original_image_tag() if isinstance(tag, FileImageTag): print("File:", tag.get_file_path()) if result.get_error_code() != EnumErrorCode.EC_OK: print("Error:", result.get_error_string()) else: items = result.get_items() print("Parsed", len(items), "MRZ Zones.") for item in items: mrz_result = MRZResult(item) print(mrz_result.to_string()) if __name__ == '__main__': print("**********************************************************") print("Welcome to Dynamsoft Capture Vision - MRZ Sample") print("**********************************************************") error_code, error_message = LicenseManager.init_license("LICENSE-KEY") if error_code != EnumErrorCode.EC_OK and error_code != EnumErrorCode.EC_LICENSE_CACHE_USED: print("License initialization failed: ErrorCode:", error_code, ", ErrorString:", error_message) else: cvr_instance = CaptureVisionRouter() while (True): image_path = input( ">> Input your image full path:\n" ">> 'Enter' for sample image or 'Q'/'q' to quit\n" ).strip('\'"') if image_path.lower() == "q": sys.exit(0) if image_path == "": image_path = "../Images/passport-sample.jpg" if not os.path.exists(image_path): print("The image path does not exist.") continue result = cvr_instance.capture(image_path, "ReadPassportAndId") if result.get_error_code() != EnumErrorCode.EC_OK: print("Error:", result.get_error_code(), result.get_error_string()) else: parsed_result = result.get_parsed_result() if parsed_result is None or len(parsed_result.get_items()) == 0: print("No parsed results.") else: print_results(parsed_result) input("Press Enter to quit...")
説明
- LicenseManager.init_license メソッドは、有効なライセンス キーを使用して Dynamsoft Capture Vision SDK を初期化します。
- CaptureVisionRouter クラスは、画像処理タスクを管理し、さまざまな画像処理モジュールを調整します。そのキャプチャ メソッドは入力画像を処理し、結果を返します。
- ReadPassportAndId は、処理モードを指定する組み込みテンプレートです。 SDK は、MRZ 認識、文書端検出、バーコード検出などのさまざまな処理モードをサポートしています。
- get_parsed_result メソッドは、MRZ の認識結果を辞書として取得します。 MRZResult クラスは、関連する MRZ 情報を抽出してラップします。このクラスはさまざまなアプリケーション間で再利用できるため、utils.py ファイルに移動することをお勧めします。
次のセクションでは、OpenCV を使用して MRZ 認識結果を視覚化し、検出された MRZ ゾーンをパスポート画像上に表示します。
Visualizing Machine Readable Zone Location in a Passport Image
In the code above, result is an instance of the CapturedResult class. Calling its get_recognized_text_lines_result() method retrieves a list of TextLineResultItem objects. Each TextLineResultItem object contains the coordinates of the detected text line. Use the following code snippet to extract the coordinates and draw contours on the passport image:
cv_image = cv2.imread(image_path) line_result = result.get_recognized_text_lines_result() items = line_result.get_items() for item in items: location = item.get_location() x1 = location.points[0].x y1 = location.points[0].y x2 = location.points[1].x y2 = location.points[1].y x3 = location.points[2].x y3 = location.points[2].y x4 = location.points[3].x y4 = location.points[3].y del location cv2.drawContours( cv_image, [np.intp([(x1, y1), (x2, y2), (x3, y3), (x4, y4)])], 0, (0, 255, 0), 2) cv2.imshow( "Original Image with Detected MRZ Zone", cv_image) cv2.waitKey(0) cv2.destroyAllWindows()
Scanning and Recognizing MRZ in Real-time via Webcam
Scanning and recognizing MRZ in real-time via webcam requires capturing a continuous image stream. We can use the OpenCV library to capture frames from the webcam and process them with the Dynamsoft Capture Vision SDK. The following code snippet demonstrates how to implement real-time MRZ recognition using a webcam:
from dynamsoft_capture_vision_bundle import * import cv2 import numpy as np import queue from utils import * class FrameFetcher(ImageSourceAdapter): def has_next_image_to_fetch(self) -> bool: return True def add_frame(self, imageData): self.add_image_to_buffer(imageData) class MyCapturedResultReceiver(CapturedResultReceiver): def __init__(self, result_queue): super().__init__() self.result_queue = result_queue def on_captured_result_received(self, captured_result): self.result_queue.put(captured_result) if __name__ == '__main__': errorCode, errorMsg = LicenseManager.init_license( "LICENSE-KEY") if errorCode != EnumErrorCode.EC_OK and errorCode != EnumErrorCode.EC_LICENSE_CACHE_USED: print("License initialization failed: ErrorCode:", errorCode, ", ErrorString:", errorMsg) else: vc = cv2.VideoCapture(0) if not vc.isOpened(): print("Error: Camera is not opened!") exit(1) cvr = CaptureVisionRouter() fetcher = FrameFetcher() cvr.set_input(fetcher) # Create a thread-safe queue to store captured items result_queue = queue.Queue() receiver = MyCapturedResultReceiver(result_queue) cvr.add_result_receiver(receiver) errorCode, errorMsg = cvr.start_capturing("ReadPassportAndId") if errorCode != EnumErrorCode.EC_OK: print("error:", errorMsg) while True: ret, frame = vc.read() if not ret: print("Error: Cannot read frame!") break fetcher.add_frame(convertMat2ImageData(frame)) if not result_queue.empty(): captured_result = result_queue.get_nowait() items = captured_result.get_items() for item in items: if item.get_type() == EnumCapturedResultItemType.CRIT_TEXT_LINE: text = item.get_text() line_results = text.split('\n') location = item.get_location() x1 = location.points[0].x y1 = location.points[0].y x2 = location.points[1].x y2 = location.points[1].y x3 = location.points[2].x y3 = location.points[2].y x4 = location.points[3].x y4 = location.points[3].y cv2.drawContours( frame, [np.intp([(x1, y1), (x2, y2), (x3, y3), (x4, y4)])], 0, (0, 255, 0), 2) delta = y3 - y1 for line_result in line_results: cv2.putText( frame, line_result, (x1, y1), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 255), 1, cv2.LINE_AA) y1 += delta del location elif item.get_type() == EnumCapturedResultItemType.CRIT_PARSED_RESULT: mrz_result = MRZResult(item) print(mrz_result.to_string()) if cv2.waitKey(1) & 0xFF == ord('q'): break cv2.imshow('frame', frame) cvr.stop_capturing() vc.release() cv2.destroyAllWindows()
Explanation
- The FrameFetcher class implements the ImageSourceAdapter interface to feed frame data into the built-in buffer.
- The MyCapturedResultReceiver class implements the CapturedResultReceiver interface. The on_captured_result_received method runs on a native C++ worker thread, sending CapturedResult objects to the main thread where they are stored in a thread-safe queue for further use.
- A CapturedResult contains several CapturedResultItem objects. The CRIT_TEXT_LINE type represents recognized text lines, while the CRIT_PARSED_RESULT type represents parsed MRZ data.
Running the Real-time MRZ Recognition Demo on Windows
Source Code
https://github.com/yushulx/python-mrz-scanner-sdk/tree/main/examples/official
위 내용은 Python에서 MRZ(기계 판독 가능 영역) 인식을 구현하는 방법의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
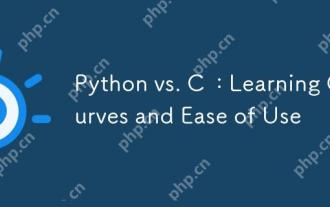
Python은 배우고 사용하기 쉽고 C는 더 강력하지만 복잡합니다. 1. Python Syntax는 간결하며 초보자에게 적합합니다. 동적 타이핑 및 자동 메모리 관리를 사용하면 사용하기 쉽지만 런타임 오류가 발생할 수 있습니다. 2.C는 고성능 응용 프로그램에 적합한 저수준 제어 및 고급 기능을 제공하지만 학습 임계 값이 높고 수동 메모리 및 유형 안전 관리가 필요합니다.
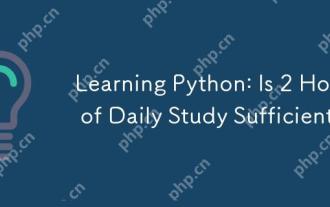
하루에 2 시간 동안 파이썬을 배우는 것으로 충분합니까? 목표와 학습 방법에 따라 다릅니다. 1) 명확한 학습 계획을 개발, 2) 적절한 학습 자원 및 방법을 선택하고 3) 실습 연습 및 검토 및 통합 연습 및 검토 및 통합,이 기간 동안 Python의 기본 지식과 고급 기능을 점차적으로 마스터 할 수 있습니다.
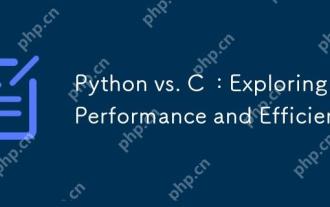
Python은 개발 효율에서 C보다 낫지 만 C는 실행 성능이 높습니다. 1. Python의 간결한 구문 및 풍부한 라이브러리는 개발 효율성을 향상시킵니다. 2.C의 컴파일 유형 특성 및 하드웨어 제어는 실행 성능을 향상시킵니다. 선택할 때는 프로젝트 요구에 따라 개발 속도 및 실행 효율성을 평가해야합니다.
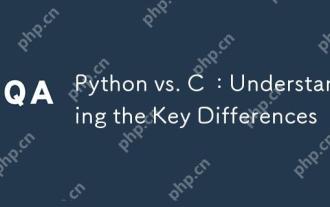
Python과 C는 각각 고유 한 장점이 있으며 선택은 프로젝트 요구 사항을 기반으로해야합니다. 1) Python은 간결한 구문 및 동적 타이핑으로 인해 빠른 개발 및 데이터 처리에 적합합니다. 2) C는 정적 타이핑 및 수동 메모리 관리로 인해 고성능 및 시스템 프로그래밍에 적합합니다.
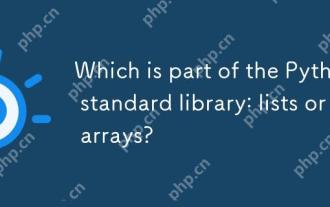
Pythonlistsarepartoftsandardlardlibrary, whileraysarenot.listsarebuilt-in, 다재다능하고, 수집 할 수있는 반면, arraysarreprovidedByTearRaymoduledlesscommonlyusedDuetolimitedFunctionality.
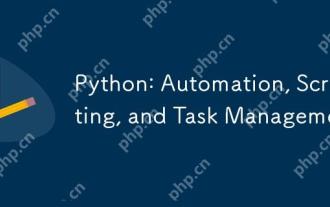
파이썬은 자동화, 스크립팅 및 작업 관리가 탁월합니다. 1) 자동화 : 파일 백업은 OS 및 Shutil과 같은 표준 라이브러리를 통해 실현됩니다. 2) 스크립트 쓰기 : PSUTIL 라이브러리를 사용하여 시스템 리소스를 모니터링합니다. 3) 작업 관리 : 일정 라이브러리를 사용하여 작업을 예약하십시오. Python의 사용 편의성과 풍부한 라이브러리 지원으로 인해 이러한 영역에서 선호하는 도구가됩니다.
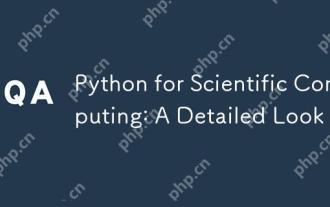
과학 컴퓨팅에서 Python의 응용 프로그램에는 데이터 분석, 머신 러닝, 수치 시뮬레이션 및 시각화가 포함됩니다. 1.numpy는 효율적인 다차원 배열 및 수학적 함수를 제공합니다. 2. Scipy는 Numpy 기능을 확장하고 최적화 및 선형 대수 도구를 제공합니다. 3. 팬더는 데이터 처리 및 분석에 사용됩니다. 4. matplotlib는 다양한 그래프와 시각적 결과를 생성하는 데 사용됩니다.
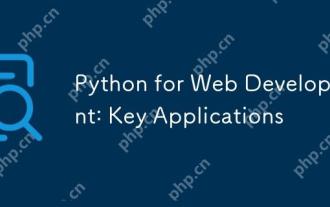
웹 개발에서 Python의 주요 응용 프로그램에는 Django 및 Flask 프레임 워크 사용, API 개발, 데이터 분석 및 시각화, 머신 러닝 및 AI 및 성능 최적화가 포함됩니다. 1. Django 및 Flask 프레임 워크 : Django는 복잡한 응용 분야의 빠른 개발에 적합하며 플라스크는 소형 또는 고도로 맞춤형 프로젝트에 적합합니다. 2. API 개발 : Flask 또는 DjangorestFramework를 사용하여 RESTFULAPI를 구축하십시오. 3. 데이터 분석 및 시각화 : Python을 사용하여 데이터를 처리하고 웹 인터페이스를 통해 표시합니다. 4. 머신 러닝 및 AI : 파이썬은 지능형 웹 애플리케이션을 구축하는 데 사용됩니다. 5. 성능 최적화 : 비동기 프로그래밍, 캐싱 및 코드를 통해 최적화
