Python에서 보안 암호화를 보장하는 방법은 무엇입니까?
대칭 키를 사용한 보안 암호화
Python에서 보안 암호화를 위해 권장되는 접근 방식은 암호화 라이브러리의 Fernet 레시피를 사용하는 것입니다. 무결성 확인을 위해 HMAC와 AES CBC 암호화를 사용하여 변조 및 무단 복호화로부터 데이터를 효과적으로 보호합니다.
Fernet 암호화 및 복호화
<code class="python">from cryptography.fernet import Fernet # Generate a secret key for encryption key = Fernet.generate_key() # Encode a message (plaintext) encoded_message = Fernet(key).encrypt(b"John Doe") # Decode the encrypted message (ciphertext) decoded_message = Fernet(key).decrypt(encoded_message) print(decoded_message.decode()) # Output: John Doe</code>
비밀번호 파생 Fernet 키
보안을 위해 무작위로 생성된 키를 사용하는 것이 좋지만 필요한 경우 비밀번호에서 키를 파생할 수도 있습니다.
<code class="python">from cryptography.fernet import Fernet from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC from cryptography.hazmat.backends import default_backend from cryptography.hazmat.primitives import hashes def derive_key(password): kdf = PBKDF2HMAC( algorithm=hashes.SHA256(), length=32, salt=secrets.token_bytes(16), iterations=100_000, backend=default_backend() ) return b64e(kdf.derive(password.encode())) # Generate a password using a key derivation function key = derive_key(password) # Encrypt and decrypt using the password-derived Fernet key encoded_message = Fernet(key).encrypt(b"John Doe") decoded_message = Fernet(key).decrypt(encoded_message) print(decoded_message.decode()) # Output: John Doe</code>
데이터 숨기기
민감하지 않은 데이터의 경우 base64 사용을 고려하세요. 암호화 대신 인코딩:
<code class="python">from base64 import urlsafe_b64encode as b64e # Encode data encoded_data = b64e(b"Hello world!") # Decode data decoded_data = b64d(encoded_data) print(decoded_data) # Output: b'Hello world!'</code>
데이터 서명
HMAC를 사용하여 무결성을 보장하기 위해 데이터 서명:
<code class="python">import hmac import hashlib # Sign data using a secret key key = secrets.token_bytes(32) signature = hmac.new(key, b"Data to sign", hashlib.sha256).digest() # Verify the signature def verify(data, signature, key): expected = hmac.new(key, data, hashlib.sha256).digest() return hmac.compare_digest(expected, signature) # Verify the signature using the same key print(verify(b"Data to sign", signature, key)) # Output: True</code>
기타: 안전하지 않은 체계의 올바른 구현
AES CFB:
<code class="python">import secrets from base64 import urlsafe_b64encode as b64e, urlsafe_b64decode as b64d from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes from cryptography.hazmat.backends import default_backend backend = default_backend() def aes_cfb_encrypt(message, key): algorithm = algorithms.AES(key) iv = secrets.token_bytes(algorithm.block_size // 8) cipher = Cipher(algorithm, modes.CFB(iv), backend=backend) encryptor = cipher.encryptor() return b64e(iv + encryptor.update(message) + encryptor.finalize()) def aes_cfb_decrypt(ciphertext, key): iv_ciphertext = b64d(ciphertext) algorithm = algorithms.AES(key) size = algorithm.block_size // 8 iv, encrypted = iv_ciphertext[:size], iv_ciphertext[size:] cipher = Cipher(algorithm, modes.CFB(iv), backend=backend) decryptor = cipher.decryptor() return decryptor.update(encrypted) + decryptor.finalize()</code>
AES ECB:
<code class="python">from base64 import urlsafe_b64encode as b64e, urlsafe_b64decode as b64d from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes from cryptography.hazmat.primitives import padding from cryptography.hazmat.backends import default_backend backend = default_backend() def aes_ecb_encrypt(message, key): cipher = Cipher(algorithms.AES(key), modes.ECB(), backend=backend) encryptor = cipher.encryptor() padder = padding.PKCS7(cipher.algorithm.block_size).padder() padded_message = padder.update(message.encode()) + padder.finalize() return b64e(encryptor.update(padded_message) + encryptor.finalize()) def aes_ecb_decrypt(ciphertext, key): cipher = Cipher(algorithms.AES(key), modes.ECB(), backend=backend) decryptor = cipher.decryptor() unpadder = padding.PKCS7(cipher.algorithm.block_size).unpadder() padded_message = decryptor.update(b64d(ciphertext)) + decryptor.finalize() return unpadder.update(padded_message) + unpadder.finalize()</code>
참고: AES ECB는 그렇지 않습니다. 안전한 암호화를 위해 권장됩니다.
위 내용은 Python에서 보안 암호화를 보장하는 방법은 무엇입니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










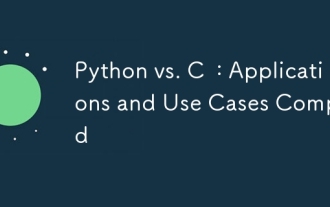
Python은 데이터 과학, 웹 개발 및 자동화 작업에 적합한 반면 C는 시스템 프로그래밍, 게임 개발 및 임베디드 시스템에 적합합니다. Python은 단순성과 강력한 생태계로 유명하며 C는 고성능 및 기본 제어 기능으로 유명합니다.
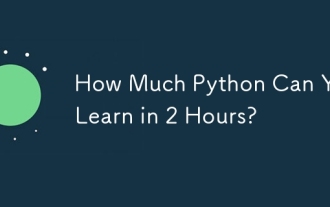
2 시간 이내에 파이썬의 기본 사항을 배울 수 있습니다. 1. 변수 및 데이터 유형을 배우십시오. 이를 통해 간단한 파이썬 프로그램 작성을 시작하는 데 도움이됩니다.
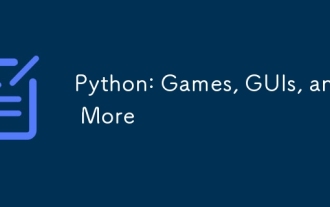
Python은 게임 및 GUI 개발에서 탁월합니다. 1) 게임 개발은 Pygame을 사용하여 드로잉, 오디오 및 기타 기능을 제공하며 2D 게임을 만드는 데 적합합니다. 2) GUI 개발은 Tkinter 또는 PYQT를 선택할 수 있습니다. Tkinter는 간단하고 사용하기 쉽고 PYQT는 풍부한 기능을 가지고 있으며 전문 개발에 적합합니다.
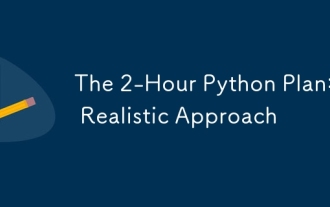
2 시간 이내에 Python의 기본 프로그래밍 개념과 기술을 배울 수 있습니다. 1. 변수 및 데이터 유형을 배우기, 2. 마스터 제어 흐름 (조건부 명세서 및 루프), 3. 기능의 정의 및 사용을 이해하십시오. 4. 간단한 예제 및 코드 스 니펫을 통해 Python 프로그래밍을 신속하게 시작하십시오.
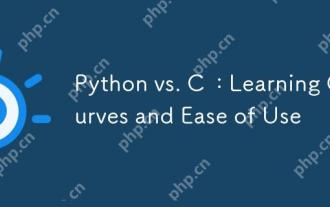
Python은 배우고 사용하기 쉽고 C는 더 강력하지만 복잡합니다. 1. Python Syntax는 간결하며 초보자에게 적합합니다. 동적 타이핑 및 자동 메모리 관리를 사용하면 사용하기 쉽지만 런타임 오류가 발생할 수 있습니다. 2.C는 고성능 응용 프로그램에 적합한 저수준 제어 및 고급 기능을 제공하지만 학습 임계 값이 높고 수동 메모리 및 유형 안전 관리가 필요합니다.
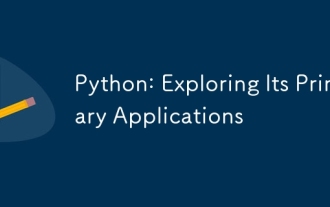
Python은 웹 개발, 데이터 과학, 기계 학습, 자동화 및 스크립팅 분야에서 널리 사용됩니다. 1) 웹 개발에서 Django 및 Flask 프레임 워크는 개발 프로세스를 단순화합니다. 2) 데이터 과학 및 기계 학습 분야에서 Numpy, Pandas, Scikit-Learn 및 Tensorflow 라이브러리는 강력한 지원을 제공합니다. 3) 자동화 및 스크립팅 측면에서 Python은 자동화 된 테스트 및 시스템 관리와 같은 작업에 적합합니다.
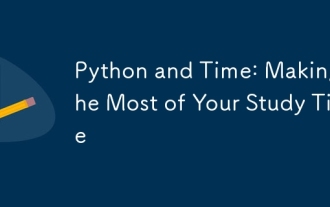
제한된 시간에 Python 학습 효율을 극대화하려면 Python의 DateTime, Time 및 Schedule 모듈을 사용할 수 있습니다. 1. DateTime 모듈은 학습 시간을 기록하고 계획하는 데 사용됩니다. 2. 시간 모듈은 학습과 휴식 시간을 설정하는 데 도움이됩니다. 3. 일정 모듈은 주간 학습 작업을 자동으로 배열합니다.

Python은 초보자부터 고급 개발자에 이르기까지 모든 요구에 적합한 단순성과 힘에 호의적입니다. 다목적 성은 다음과 같이 반영됩니다. 1) 배우고 사용하기 쉽고 간단한 구문; 2) Numpy, Pandas 등과 같은 풍부한 라이브러리 및 프레임 워크; 3) 다양한 운영 체제에서 실행할 수있는 크로스 플랫폼 지원; 4) 작업 효율성을 향상시키기위한 스크립팅 및 자동화 작업에 적합합니다.
