Go를 사용하여 MongoDB에서 여러 속성 값을 기반으로 항목을 검색하는 방법은 무엇입니까?
Go에서 MongoDB의 여러 속성 값을 확인하여 항목 목록 검색
MongoDB에서는 다음을 사용하여 여러 속성 값을 기반으로 항목을 검색할 수 있습니다. 집계 파이프라인. mgo.v2 패키지를 사용한 Go 구현은 다음과 같습니다.
<code class="go">import ( "context" "fmt" "gopkg.in/mgo.v2" "gopkg.in/mgo.v2/bson" ) func RetrieveItemListByMultipleAttributeValues(databaseName, collectionName string, venueIDs []string) (map[string]int, error) { ctx := context.Background() // Create a new MongoDB connection. session, err := mgo.Dial("mongodb://host:port") if err != nil { return nil, fmt.Errorf("Error dialing MongoDB: %w", err) } defer session.Close() // Select the database and collection. collection := session.DB(databaseName).C(collectionName) // Define the aggregation pipeline. pipeline := []bson.M{ {"$match": bson.M{"venueList.id": bson.M{"$in": venueIDs}}}, {"$unwind": "$venueList"}, {"$match": bson.M{"venueList.id": bson.M{"$in": venueIDs}}}, {"$unwind": "$venueList.sum"}, {"$group": bson.M{ "_id": "$venueList.sum.name", "count": bson.M{"$sum": "$venueList.sum.value"}, }}, {"$group": bson.M{ "_id": nil, "counts": bson.M{ "$push": bson.M{ "name": "$_id", "count": "$count", }, }, }}, } // Run the aggregation pipeline. resultIterator, err := collection.Pipe(pipeline).Iter() if err != nil { return nil, fmt.Errorf("Error running aggregation pipeline: %w", err) } // Create a map to store the aggregated results. result := make(map[string]int) // Decode each result and add it to the map. for resultIterator.Next(&result) { for _, count := range result["counts"].([]interface{}) { result[count.(map[string]interface{})["name"].(string)] = count.(map[string]interface{})["count"].(int) } } // Close the result iterator. resultIterator.Close() // Return the result map. return result, nil }</code>
위 내용은 Go를 사용하여 MongoDB에서 여러 속성 값을 기반으로 항목을 검색하는 방법은 무엇입니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
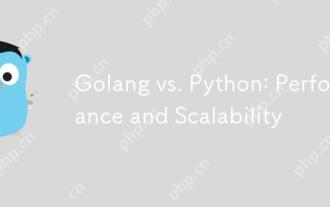
Golang은 성능과 확장 성 측면에서 Python보다 낫습니다. 1) Golang의 컴파일 유형 특성과 효율적인 동시성 모델은 높은 동시성 시나리오에서 잘 수행합니다. 2) 해석 된 언어로서 파이썬은 천천히 실행되지만 Cython과 같은 도구를 통해 성능을 최적화 할 수 있습니다.
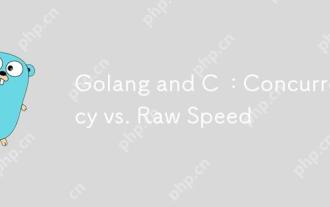
Golang은 동시성에서 C보다 낫고 C는 원시 속도에서 Golang보다 낫습니다. 1) Golang은 Goroutine 및 Channel을 통해 효율적인 동시성을 달성하며, 이는 많은 동시 작업을 처리하는 데 적합합니다. 2) C 컴파일러 최적화 및 표준 라이브러리를 통해 하드웨어에 가까운 고성능을 제공하며 극도의 최적화가 필요한 애플리케이션에 적합합니다.
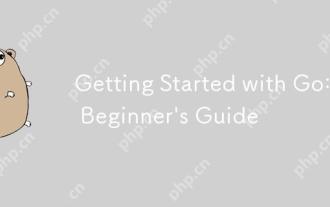
goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity, 효율성, 및 콘크리 론 피처
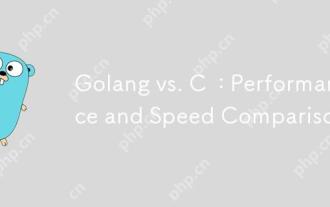
Golang은 빠른 개발 및 동시 시나리오에 적합하며 C는 극도의 성능 및 저수준 제어가 필요한 시나리오에 적합합니다. 1) Golang은 쓰레기 수집 및 동시성 메커니즘을 통해 성능을 향상시키고, 고전성 웹 서비스 개발에 적합합니다. 2) C는 수동 메모리 관리 및 컴파일러 최적화를 통해 궁극적 인 성능을 달성하며 임베디드 시스템 개발에 적합합니다.
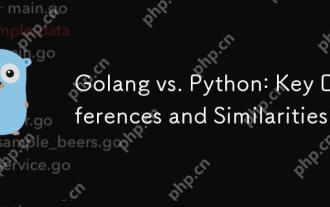
Golang과 Python은 각각 고유 한 장점이 있습니다. Golang은 고성능 및 동시 프로그래밍에 적합하지만 Python은 데이터 과학 및 웹 개발에 적합합니다. Golang은 동시성 모델과 효율적인 성능으로 유명하며 Python은 간결한 구문 및 풍부한 라이브러리 생태계로 유명합니다.
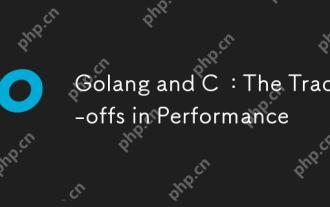
Golang과 C의 성능 차이는 주로 메모리 관리, 컴파일 최적화 및 런타임 효율에 반영됩니다. 1) Golang의 쓰레기 수집 메커니즘은 편리하지만 성능에 영향을 줄 수 있습니다. 2) C의 수동 메모리 관리 및 컴파일러 최적화는 재귀 컴퓨팅에서 더 효율적입니다.
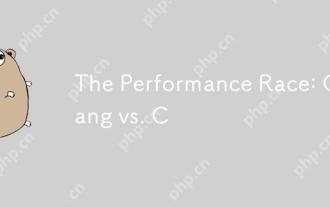
Golang과 C는 각각 공연 경쟁에서 고유 한 장점을 가지고 있습니다. 1) Golang은 높은 동시성과 빠른 발전에 적합하며 2) C는 더 높은 성능과 세밀한 제어를 제공합니다. 선택은 프로젝트 요구 사항 및 팀 기술 스택을 기반으로해야합니다.
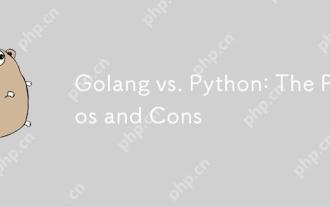
golangisidealforbuildingscalablesystemsdueToitsefficiencyandconcurrency
