자산 심층 분석에 대한 액세스
JavaScript는 웹 개발에 광범위하게 사용되는 다재다능하고 강력한 프로그래밍 언어입니다. 주요 기능 중 하나는 속성과 메서드를 캡슐화할 수 있는 개체를 정의하는 기능입니다. 이러한 개체와 상호 작용하는 다양한 방법 중에서 접근자는 중요한 역할을 합니다.
자바스크립트에서 속성에 액세스하는 방법을 더 자세히 살펴보겠습니다.
JavaScript 개체를 사용한 당근 케이크 레시피
당근 케이크 레시피를 나타내는 JavaScript 개체를 만들어 보겠습니다. 해당 속성에 액세스하기 위해 점 표기법과 대괄호 표기법을 모두 사용합니다.
1단계: 당근 케이크 개체 정의
재료, 굽는 시간, 지침 등의 속성이 포함된 개체를 정의하겠습니다.
const carrotCake = { name: 'Carrot Cake', ingredients: { flour: '2 cups', sugar: '1 cup', carrots: '2 cups grated', eggs: '3 large', oil: '1 cup', bakingPowder: '2 tsp', cinnamon: '1 tsp', salt: '1/2 tsp' }, bakingTime: '45 minutes', instructions: [ 'Preheat the oven to 350°F (175°C).', 'In a bowl, mix flour, sugar, baking powder, cinnamon, and salt.', 'In another bowl, whisk eggs and oil together.', 'Combine the wet and dry ingredients, then fold in grated carrots.', 'Pour the batter into a greased cake pan.', 'Bake for 45 minutes or until a toothpick comes out clean.', 'Let cool before serving.' ] };
2단계: 점 표기법을 사용하여 속성에 액세스
점 표기법을 사용하여 carrotCake 개체의 속성에 액세스할 수 있습니다.
console.log(carrotCake.name); // Outputs: Carrot Cake console.log(carrotCake.bakingTime); // Outputs: 45 minutes console.log(carrotCake.ingredients.flour); // Outputs: 2 cups
3단계: 대괄호 표기법을 사용하여 속성에 액세스
대괄호 표기법을 사용할 수도 있습니다. 특히 공백이 있는 속성이나 동적 키를 사용할 때 유용합니다.
console.log(carrotCake['name']); // Outputs: Carrot Cake console.log(carrotCake['bakingTime']); // Outputs: 45 minutes console.log(carrotCake['ingredients']['sugar']); // Outputs: 1 cup
4단계: 재료 반복
for...in 루프를 사용하여 재료를 반복하여 모든 재료를 표시할 수 있습니다.
for (const ingredient in carrotCake.ingredients) { console.log(`${ingredient}: ${carrotCake.ingredients[ingredient]}`); }
다음과 같이 출력됩니다:
flour: 2 cups sugar: 1 cup carrots: 2 cups grated eggs: 3 large oil: 1 cup bakingPowder: 2 tsp cinnamon: 1 tsp salt: 1/2 tsp
JavaScript 개체 접근자란 무엇입니까?
접근자는 객체의 속성 값을 가져오거나 설정하는 메서드입니다. getter와 setter의 두 가지 형식이 있습니다.
Getters: 속성 값을 가져오는 메서드입니다.
Setter: 속성 값을 설정하는 메서드입니다.
이러한 접근자는 속성에 액세스하고 수정하는 방법을 제어하는 방법을 제공합니다. 이는 데이터 검증, 캡슐화 및 계산된 속성 제공에 유용할 수 있습니다.
Getter 및 Setter 정의
JavaScript에서는 객체 리터럴 내에서 또는 Object.defineProperty 메소드를 사용하여 getter 및 setter를 정의할 수 있습니다.
객체 리터럴 사용
다음은 객체 리터럴에서 getter 및 setter를 정의하는 방법에 대한 예입니다.
let person = { firstName: "Irena", lastName: "Doe", get fullName() { return `${this.firstName} ${this.lastName}`; // Returns full name }, set fullName(name) { let parts = name.split(' '); // Splits the name into parts this.firstName = parts[0]; // Sets first name this.lastName = parts[1]; // Sets last name } }; console.log(person.fullName); // Outputs: Irena Doe person.fullName = "Jane Smith"; // Updates first and last name console.log(person.firstName); // Outputs: Jane console.log(person.lastName); // Outputs: Smith
객체 정의: firstName 및 lastName 속성을 사용하여 person이라는 객체를 정의했습니다.
- Getter: fullName getter는 firstName과 lastName을 연결하여 액세스 시 전체 이름을 반환합니다.
- Setter: fullName setter는 제공된 전체 이름 문자열을 여러 부분으로 나누고 첫 번째 부분을 firstName에 할당하고 두 번째 부분을 lastName에 할당합니다. 용법: person.fullName을 기록하면 "Irena Doe"가 올바르게 출력됩니다. person.fullName을 "Jane Smith"로 설정하면 firstName 및 lastName 속성이 그에 따라 업데이트됩니다.
getter/setter와 점/괄호 표기법의 차이점을 설명하기 위해 당근 케이크 예제를 개선해 보겠습니다. 직접적인 속성 액세스와 getter 및 setter를 통한 속성 액세스가 모두 포함된 객체를 생성하겠습니다.
1단계: 당근 케이크 개체 정의
직접 속성과 특정 속성에 대한 getter/setter를 모두 사용하는 carrotCake 객체를 정의하겠습니다.
const carrotCake = { name: 'Carrot Cake', ingredients: { flour: '2 cups', sugar: '1 cup', carrots: '2 cups grated', eggs: '3 large', oil: '1 cup', bakingPowder: '2 tsp', cinnamon: '1 tsp', salt: '1/2 tsp' }, bakingTime: '45 minutes', instructions: [ 'Preheat the oven to 350°F (175°C).', 'In a bowl, mix flour, sugar, baking powder, cinnamon, and salt.', 'In another bowl, whisk eggs and oil together.', 'Combine the wet and dry ingredients, then fold in grated carrots.', 'Pour the batter into a greased cake pan.', 'Bake for 45 minutes or until a toothpick comes out clean.', 'Let cool before serving.' ] };
- 2단계: 점 및 괄호 표기법을 사용하여 속성에 액세스 점 표기법을 사용하여 속성에 직접 액세스하고 수정해 보겠습니다.
console.log(carrotCake.name); // Outputs: Carrot Cake console.log(carrotCake.bakingTime); // Outputs: 45 minutes console.log(carrotCake.ingredients.flour); // Outputs: 2 cups
- 3단계: Getter 및 Setter를 사용하여 속성에 액세스 이제 getter 및 setter를 사용하여 속성에 액세스하고 수정해 보겠습니다.
console.log(carrotCake['name']); // Outputs: Carrot Cake console.log(carrotCake['bakingTime']); // Outputs: 45 minutes console.log(carrotCake['ingredients']['sugar']); // Outputs: 1 cup
- 4단계: 재료 업데이트 재료를 업데이트하는 방법 사용:
for (const ingredient in carrotCake.ingredients) { console.log(`${ingredient}: ${carrotCake.ingredients[ingredient]}`); }
차이점을 요약해 보겠습니다
점/괄호 표기법:
- 속성을 직접 액세스하거나 수정합니다.
- 검증이나 논리가 적용되지 않습니다. 예를 들어 carrotCake._name = ''; 확인 없이 이름을 덮어씁니다. ###게터/세터:
- 속성에 액세스하고 수정하는 통제된 방법을 제공합니다.
- setter의 유효성 검사와 같은 사용자 정의 논리를 포함할 수 있습니다. 예: carrotCake.name = ''; 빈 이름을 설정하는 것을 방지합니다.
이 예에서는 JavaScript 개체에서 두 가지 접근 방식을 모두 사용할 수 있는 방법을 보여주고 논리를 캡슐화하고 데이터 무결성을 보장하기 위한 getter 및 setter의 이점을 강조합니다.
접근자 사용의 이점
캡슐화
접근자를 사용하면 더 깔끔한 인터페이스를 노출하면서 객체의 내부 표현을 숨길 수 있습니다. 이는 객체지향 프로그래밍에서 캡슐화의 기본 원칙입니다.검증
Setter는 속성을 업데이트하기 전에 데이터의 유효성을 검사하는 데 사용할 수 있습니다. 이렇게 하면 객체가 유효한 상태로 유지됩니다.
요약
이 예에서는 당근 케이크 레시피를 나타내는 간단한 JavaScript 개체를 만들었습니다. 점과 대괄호 표기법을 모두 사용하여 속성에 액세스하여 JavaScript에서 속성 접근자가 얼마나 다양한지 보여줍니다.
JavaScript 객체 접근자는 객체 속성과 상호 작용하는 방식을 향상시키는 강력한 기능입니다. getter 및 setter를 사용하면 캡슐화, 유효성 검사, 계산된 속성 및 읽기 전용 속성을 개체에 추가할 수 있습니다. 이러한 접근자를 이해하고 활용하면 더욱 강력하고 유지 관리가 가능하며 깔끔한 코드를 만들 수 있습니다. 계속해서 JavaScript를 탐색하고 익히면서 접근자를 개체에 통합하는 것은 의심할 여지 없이 프로그래밍 툴킷에서 귀중한 도구가 될 것입니다.
위 내용은 자산 심층 분석에 대한 액세스의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










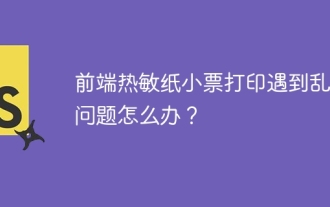
프론트 엔드 개발시 프론트 엔드 열지대 티켓 인쇄를위한 자주 묻는 질문과 솔루션, 티켓 인쇄는 일반적인 요구 사항입니다. 그러나 많은 개발자들이 구현하고 있습니다 ...
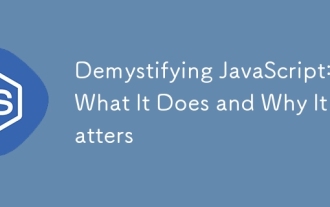
JavaScript는 현대 웹 개발의 초석이며 주요 기능에는 이벤트 중심 프로그래밍, 동적 컨텐츠 생성 및 비동기 프로그래밍이 포함됩니다. 1) 이벤트 중심 프로그래밍을 사용하면 사용자 작업에 따라 웹 페이지가 동적으로 변경 될 수 있습니다. 2) 동적 컨텐츠 생성을 사용하면 조건에 따라 페이지 컨텐츠를 조정할 수 있습니다. 3) 비동기 프로그래밍은 사용자 인터페이스가 차단되지 않도록합니다. JavaScript는 웹 상호 작용, 단일 페이지 응용 프로그램 및 서버 측 개발에 널리 사용되며 사용자 경험 및 크로스 플랫폼 개발의 유연성을 크게 향상시킵니다.
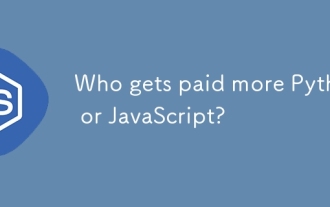
기술 및 산업 요구에 따라 Python 및 JavaScript 개발자에 대한 절대 급여는 없습니다. 1. 파이썬은 데이터 과학 및 기계 학습에서 더 많은 비용을 지불 할 수 있습니다. 2. JavaScript는 프론트 엔드 및 풀 스택 개발에 큰 수요가 있으며 급여도 상당합니다. 3. 영향 요인에는 경험, 지리적 위치, 회사 규모 및 특정 기술이 포함됩니다.
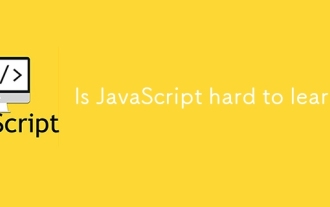
JavaScript를 배우는 것은 어렵지 않지만 어려운 일입니다. 1) 변수, 데이터 유형, 기능 등과 같은 기본 개념을 이해합니다. 2) 마스터 비동기 프로그래밍 및 이벤트 루프를 통해이를 구현하십시오. 3) DOM 운영을 사용하고 비동기 요청을 처리합니다. 4) 일반적인 실수를 피하고 디버깅 기술을 사용하십시오. 5) 성능을 최적화하고 모범 사례를 따르십시오.
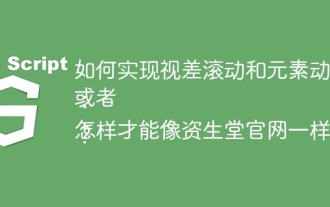
이 기사에서 시차 스크롤 및 요소 애니메이션 효과 실현에 대한 토론은 Shiseido 공식 웹 사이트 (https://www.shiseido.co.jp/sb/wonderland/)와 유사하게 달성하는 방법을 살펴볼 것입니다.
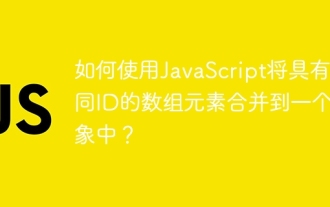
동일한 ID로 배열 요소를 JavaScript의 하나의 객체로 병합하는 방법은 무엇입니까? 데이터를 처리 할 때 종종 동일한 ID를 가질 필요가 있습니다 ...
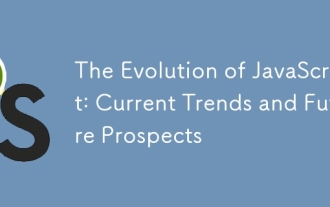
JavaScript의 최신 트렌드에는 Typescript의 Rise, 현대 프레임 워크 및 라이브러리의 인기 및 WebAssembly의 적용이 포함됩니다. 향후 전망은보다 강력한 유형 시스템, 서버 측 JavaScript 개발, 인공 지능 및 기계 학습의 확장, IoT 및 Edge 컴퓨팅의 잠재력을 포함합니다.
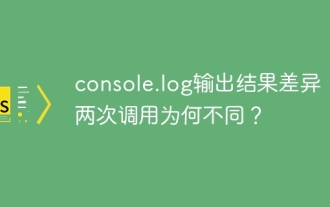
Console.log 출력의 차이의 근본 원인에 대한 심층적 인 논의. 이 기사에서는 Console.log 함수의 출력 결과의 차이점을 코드에서 분석하고 그에 따른 이유를 설명합니다. � ...
