PHP에서 가장 가까운 5의 배수로 반올림하는 방법은 무엇입니까?
PHP에서 가장 가까운 5의 배수로 반올림
PHP에서 숫자로 작업할 때 가장 가까운 특정 값으로 반올림해야 하는 경우가 많습니다. 일반적인 시나리오 중 하나는 가장 가까운 5의 배수로 반올림하는 것입니다.
문제 설명
정수를 입력으로 사용하고 가장 가까운 5의 배수를 반환하는 PHP 함수를 찾습니다. 예를 들어 52로 호출하면 55를 반환해야 합니다.
내장된 round() 함수는 기본적으로 이 기능을 제공하지 않습니다. 음수 정밀도와 함께 사용하면 가장 가까운 10의 거듭제곱으로 반올림됩니다.
해결책
원하는 반올림 동작을 달성하려면 사용자 정의 함수를 생성할 수 있습니다.
<code class="php">function roundUpToNearestMultiple($number, $multiplier = 5) { // Check if the number is already a multiple of the multiplier if ($number % $multiplier == 0) { return $number; } // Calculate the nearest multiple of the multiplier greater than the number $nextMultiple = ceil($number / $multiplier) * $multiplier; // Round the number up to the next multiple return $nextMultiple; }</code>
사용 예
<code class="php">echo roundUpToNearestMultiple(52); // Outputs 55 echo roundUpToNearestMultiple(55); // Outputs 55 echo roundUpToNearestMultiple(47); // Outputs 50</code>
기타 반올림 전략
가장 가까운 배수로 반올림하는 것 외에도 다양한 반올림 전략이 필요한 시나리오가 발생할 수 있습니다. 다음은 몇 가지 변형입니다.
1. 현재 숫자를 제외하고 다음 배수로 반올림
<code class="php">function roundUpToNextMultiple($number, $multiplier = 5) { return roundUpToNearestMultiple($number + 1, $multiplier); }</code>
2. 현재 숫자를 포함하여 가장 가까운 배수로 반올림
<code class="php">function roundToNearestMultipleInclusive($number, $multiplier = 5) { if ($number % $multiplier == 0) { return $number; } $lowerMultiple = floor($number / $multiplier) * $multiplier; $upperMultiple = ceil($number / $multiplier) * $multiplier; return round($number - $lowerMultiple) > round($upperMultiple - $number) ? $lowerMultiple : $upperMultiple; }</code>
3. 정수로 반올림한 후 가장 가까운 배수로 올림
<code class="php">function roundUpToIntegerAndNearestMultiple($number, $multiplier = 5) { $roundedNumber = ceil($number); if ($roundedNumber % $multiplier == 0) { return $roundedNumber; } return roundUpToNearestMultiple($roundedNumber, $multiplier); }</code>
위 내용은 PHP에서 가장 가까운 5의 배수로 반올림하는 방법은 무엇입니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
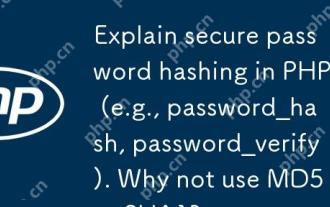
PHP에서 Password_hash 및 Password_Verify 기능을 사용하여 보안 비밀번호 해싱을 구현해야하며 MD5 또는 SHA1을 사용해서는 안됩니다. 1) Password_hash는 보안을 향상시키기 위해 소금 값이 포함 된 해시를 생성합니다. 2) Password_verify 암호를 확인하고 해시 값을 비교하여 보안을 보장합니다. 3) MD5 및 SHA1은 취약하고 소금 값이 부족하며 현대 암호 보안에는 적합하지 않습니다.
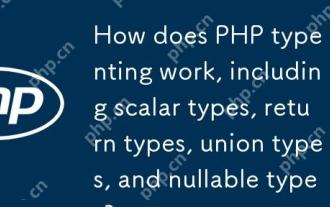
PHP 유형은 코드 품질과 가독성을 향상시키기위한 프롬프트입니다. 1) 스칼라 유형 팁 : PHP7.0이므로 int, float 등과 같은 기능 매개 변수에 기본 데이터 유형을 지정할 수 있습니다. 2) 반환 유형 프롬프트 : 기능 반환 값 유형의 일관성을 확인하십시오. 3) Union 유형 프롬프트 : PHP8.0이므로 기능 매개 변수 또는 반환 값에 여러 유형을 지정할 수 있습니다. 4) Nullable 유형 프롬프트 : NULL 값을 포함하고 널 값을 반환 할 수있는 기능을 포함 할 수 있습니다.
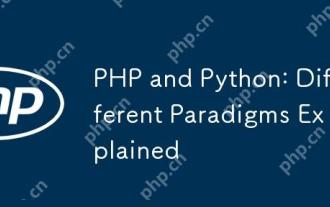
PHP는 주로 절차 적 프로그래밍이지만 객체 지향 프로그래밍 (OOP)도 지원합니다. Python은 OOP, 기능 및 절차 프로그래밍을 포함한 다양한 패러다임을 지원합니다. PHP는 웹 개발에 적합하며 Python은 데이터 분석 및 기계 학습과 같은 다양한 응용 프로그램에 적합합니다.
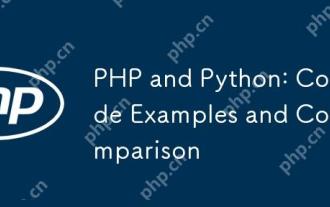
PHP와 Python은 고유 한 장점과 단점이 있으며 선택은 프로젝트 요구와 개인 선호도에 달려 있습니다. 1.PHP는 대규모 웹 애플리케이션의 빠른 개발 및 유지 보수에 적합합니다. 2. Python은 데이터 과학 및 기계 학습 분야를 지배합니다.
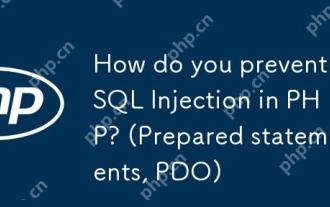
PHP에서 전처리 문과 PDO를 사용하면 SQL 주입 공격을 효과적으로 방지 할 수 있습니다. 1) PDO를 사용하여 데이터베이스에 연결하고 오류 모드를 설정하십시오. 2) 준비 방법을 통해 전처리 명세서를 작성하고 자리 표시자를 사용하여 데이터를 전달하고 방법을 실행하십시오. 3) 쿼리 결과를 처리하고 코드의 보안 및 성능을 보장합니다.
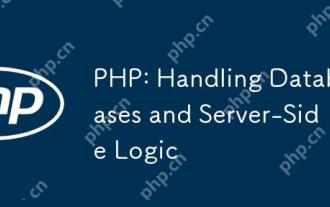
PHP는 MySQLI 및 PDO 확장 기능을 사용하여 데이터베이스 작업 및 서버 측 로직 프로세싱에서 상호 작용하고 세션 관리와 같은 기능을 통해 서버 측로 로직을 처리합니다. 1) MySQLI 또는 PDO를 사용하여 데이터베이스에 연결하고 SQL 쿼리를 실행하십시오. 2) 세션 관리 및 기타 기능을 통해 HTTP 요청 및 사용자 상태를 처리합니다. 3) 트랜잭션을 사용하여 데이터베이스 작업의 원자력을 보장하십시오. 4) SQL 주입 방지, 디버깅을 위해 예외 처리 및 폐쇄 연결을 사용하십시오. 5) 인덱싱 및 캐시를 통해 성능을 최적화하고, 읽을 수있는 코드를 작성하고, 오류 처리를 수행하십시오.
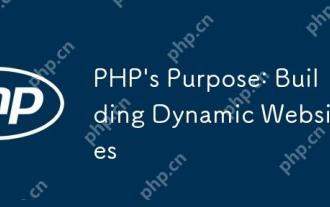
PHP는 동적 웹 사이트를 구축하는 데 사용되며 해당 핵심 기능에는 다음이 포함됩니다. 1. 데이터베이스와 연결하여 동적 컨텐츠를 생성하고 웹 페이지를 실시간으로 생성합니다. 2. 사용자 상호 작용 및 양식 제출을 처리하고 입력을 확인하고 작업에 응답합니다. 3. 개인화 된 경험을 제공하기 위해 세션 및 사용자 인증을 관리합니다. 4. 성능을 최적화하고 모범 사례를 따라 웹 사이트 효율성 및 보안을 개선하십시오.
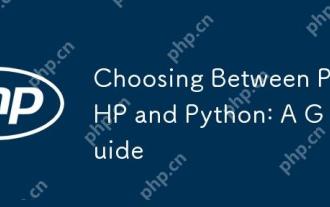
PHP는 웹 개발 및 빠른 프로토 타이핑에 적합하며 Python은 데이터 과학 및 기계 학습에 적합합니다. 1.PHP는 간단한 구문과 함께 동적 웹 개발에 사용되며 빠른 개발에 적합합니다. 2. Python은 간결한 구문을 가지고 있으며 여러 분야에 적합하며 강력한 라이브러리 생태계가 있습니다.
