jsDoc 전도
TLDR;
레거시 코드 기반으로 작업하세요. 우리 중 많은 사람들이 시간이 지나면서 피할 수 없습니다. Typescript 대신 jsDoc을 사용해 보도록 안내합니다. 그 놀라운 진실을 밝혀야 합니다!
먼저 정리하자: jsDoc 또는 TS는 개발자 시간을 의미합니다(나중에 검토 포함, 재사용, 모든 환경에서 해당 코드 보기: git 웹 페이지, 임의 편집기, 크롬, 파이어폭스 등). ..:devtool, vim, cat ... );
첫 번째 악장
편집기를 열고 jsDoc이 작동하는지 테스트하기 위한 적절한 설명을 작성하세요.
/** @typedef { 'JS' | 'JQuery' | 'TS' | 'jsDoc' } Phase; */ /** * On typing ' editor will popup string list. * * @type {Phase} */ const badCase = 'React'; // !!!! lint alert !!!! /** @type {Phase} */ const goodCase = 'JQuery';
jsDoc과 Typescript 비교
제 경험상 TS 코드를 대체할 수 있는 jsDoc이 있는데, 보너스로 이 둘 사이에 웜홀이 존재합니다.
jsDoc의 가장 큰 기능 imho: 이는 표준 JS 주석 시스템을 사용하므로 JS 코드를 손상시키지 마십시오. 이 코드는 JS가 실행 가능한 모든 곳에서 실행됩니다.
feature | jsDoc | Typescript |
---|---|---|
compiling freedom | Do not need compiling the code. | mandatory to compile |
dependency freedom | Can working without any dependency | at least TS is your dependency |
namespace freedom | Don't interfere Types and other imports names. You can use same name of component and component Type in React for example. | Typesript names are identical including as any other referenct |
rework freedom | Don't need to change existing code, just insert a comment. | at least some part in your code need to be turn to TS |
legacy freedom | Can be use even transform to Typescript the project is not option. Many legacy projects affected this situation. | need to push management to allow that modification |
Maximalism freedom | Don't need to use every where. Even you can use on a new commits, and after easy to search which is the new or reworked parts. | also enough to turn build system, and just part of code to TS |
future freedom | Later can easy trasnlate to TS. Under the hood this is use same technic, just different way. | need to work if decide to use JS instead TS |
mindset freedom | Because this is a comment your know well this is don't made any type check at runtime for you as TS also. | TS is noising your code |
jsDoc 편집자 경험
어떤 편집기에서든 jsDoc을 작성할 수 있지만 이해하는 경우는 거의 없습니다.
노드 모듈 경험
저는 npm 모듈인 jsdoc-duck도 만들었습니다. jsDoc 코딩 모듈입니다. 이는 TypeScript 없이는 jsDoc npm 모듈을 생성할 수 없다는 점을 강조했습니다. 아마도 vite 구축 매개변수를 파악하는 데 더 많은 시간을 투자한다면 올바른 솔루션을 찾을 수 있을 것입니다. 그러나 좋은 소식은 npm과 함께 해당 모듈을 사용하지 않고 대신 우리 코드를 빌려오는 것입니다. index.js를 한 위치에 복사하면 프로그램에 새 종속성을 삽입하는 데 사용되며 아무 일도 일어나지 않습니다. 모듈 소유자가 모듈을 다른 것으로 바꾸면.
jsDoc 사이의 웜홀 타이프스크립트
Typescript와 jsDoc이 서로 호환된다는 좋은 소식은 단지 jsDoc이 약간 다른 구문을 사용한다는 것입니다. 그러나 typescript에서 jsDoc 모듈 유형을 사용할 수 있으며 javascript/js jsDoc 프로그램에서도 typescript 모듈 유형을 사용할 수 있습니다. 따라서 최종 결정은 귀하의 손에 달려 있습니다.
노란 벽돌길을 따라가세요
VS 코드 예제는 jsDoc 코드에서 유형이 얼마나 잘 보이는지 보여줍니다. 제 생각에는 이것이 코드에 주는 노이즈가 놀라울 정도로 적습니다.
보너스
:: VS 코드 조각
/** @typedef { 'JS' | 'JQuery' | 'TS' | 'jsDoc' } Phase; */ /** * On typing ' editor will popup string list. * * @type {Phase} */ const badCase = 'React'; // !!!! lint alert !!!! /** @type {Phase} */ const goodCase = 'JQuery';
:: jsdoc-duck 코드
( 이 보기에서 구문 강조 표시는 유형을 이해하는 데 도움이 되지 않습니다). 하지만 이 짧은 프로그램은 jsDoc이 TS 고급 기능도 사용할 수 있는 좋은 예입니다.
"@type": { "prefix": ["ty"], "body": ["/** @type {<pre class="brush:php;toolbar:false">import { useMemo, useReducer } from "react"; /** * @template T - Payload Type * @typedef {T extends { type: infer U, payload?: infer P } ? { type: U, payload?: P } : never} ActionType */ /** @template AM - Actions Map @typedef {{ [K in AM['type']]: K }} Labels */ /** @template AM - Actions Map @typedef {{ [T in AM["type"]]: Extract<AM, { type: T }> extends { payload: infer P } ? (payload: P) => void : () => void }} Quack */ /** * @template ST - State * @template AM - Actions Map * @typedef {(state: ST, action: AM) => ST} Reducer */ /** * Factory function to create a typed action map. * @template AM - Actions Map * @param {Labels<AM>} labelsObject - The keys representing action labels. * @param {function} dispatch - The dispatch function for actions. * @return {Quack<AM>} The resulting typed action map. */ export const quackFactory = (labelsObject, dispatch) => Object .keys(labelsObject) .reduce( /** * @arg {Quack<AM>} acc * @arg {keyof Labels<AM>} type * @return {Quack<AM>} */ (acc, type) => ({ ...acc, [type]: (payload) => {dispatch({ type, payload });} }), {}); /** * A factory hook to create a state and a typed dispatch functions\ * @exports useDuck * @template AM - Actions Map * @template ST - State Typer * @param {(st: ST, action: AM) => ST} reducer - The reducer function to manage the state. * @param {ST} initialState - The initial state value. * @return {[ST, Quack<AM>]} The current state and a map of action dispatch functions. */ export const useDuck = (reducer, initialState, labels) => { const [state, dispatch] = useReducer(reducer, initialState); const quack = useMemo( () => quackFactory(labels, dispatch), [dispatch, labels] ); return ([state, quack]); };
코딩을 즐기세요. 대신 종속성을 추가하세요
위 내용은 jsDoc 전도의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
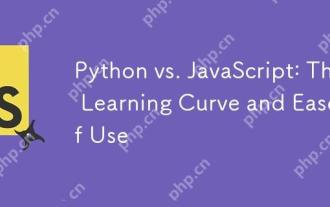
Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
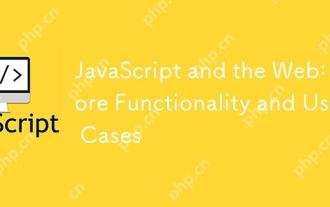
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.
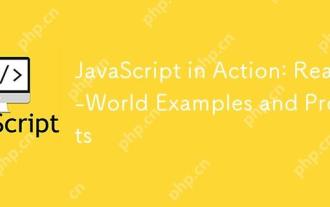
실제 세계에서 JavaScript의 응용 프로그램에는 프론트 엔드 및 백엔드 개발이 포함됩니다. 1) DOM 운영 및 이벤트 처리와 관련된 TODO 목록 응용 프로그램을 구축하여 프론트 엔드 애플리케이션을 표시합니다. 2) Node.js를 통해 RESTFULAPI를 구축하고 Express를 통해 백엔드 응용 프로그램을 시연하십시오.
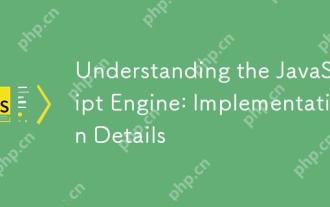
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.
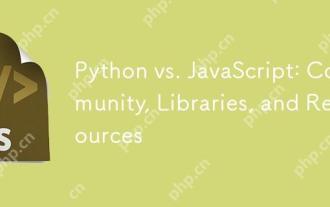
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
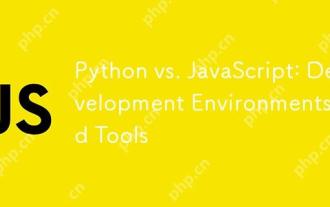
개발 환경에서 Python과 JavaScript의 선택이 모두 중요합니다. 1) Python의 개발 환경에는 Pycharm, Jupyternotebook 및 Anaconda가 포함되어 있으며 데이터 과학 및 빠른 프로토 타이핑에 적합합니다. 2) JavaScript의 개발 환경에는 Node.js, VScode 및 Webpack이 포함되어 있으며 프론트 엔드 및 백엔드 개발에 적합합니다. 프로젝트 요구에 따라 올바른 도구를 선택하면 개발 효율성과 프로젝트 성공률이 향상 될 수 있습니다.
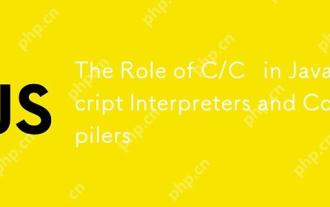
C와 C는 주로 통역사와 JIT 컴파일러를 구현하는 데 사용되는 JavaScript 엔진에서 중요한 역할을합니다. 1) C는 JavaScript 소스 코드를 구문 분석하고 추상 구문 트리를 생성하는 데 사용됩니다. 2) C는 바이트 코드 생성 및 실행을 담당합니다. 3) C는 JIT 컴파일러를 구현하고 런타임에 핫스팟 코드를 최적화하고 컴파일하며 JavaScript의 실행 효율을 크게 향상시킵니다.
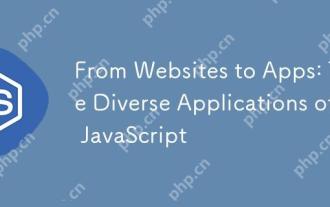
JavaScript는 웹 사이트, 모바일 응용 프로그램, 데스크탑 응용 프로그램 및 서버 측 프로그래밍에서 널리 사용됩니다. 1) 웹 사이트 개발에서 JavaScript는 HTML 및 CSS와 함께 DOM을 운영하여 동적 효과를 달성하고 jQuery 및 React와 같은 프레임 워크를 지원합니다. 2) 반응 및 이온 성을 통해 JavaScript는 크로스 플랫폼 모바일 애플리케이션을 개발하는 데 사용됩니다. 3) 전자 프레임 워크를 사용하면 JavaScript가 데스크탑 애플리케이션을 구축 할 수 있습니다. 4) node.js는 JavaScript가 서버 측에서 실행되도록하고 동시 요청이 높은 높은 요청을 지원합니다.
