Go를 사용하여 JSON에서 Snake-Case를 CamelCase 키로 변환하는 방법은 무엇입니까?
JSON에서 Snake-Case를 CamelCase 키로 변환
Go에서는 JSON 문서의 키를 snake_case에서 camelCase로 변환하는 것이 어려울 수 있습니다. 특히 JSON이 중첩되어 있고 임의로 큰 유형 또는 인터페이스{} 유형이 포함될 수 있는 경우.
방법 1: 태그와 함께 다른 구조체 사용
간단한 변환의 경우 다음을 수행할 수 있습니다. 다양한 태그로 다양한 구조체를 정의하는 Go의 기능을 활용하세요. JSON을 snake_case 태그를 사용하여 소스 구조체로 역정렬화한 다음 이를 camelCase 태그를 사용하여 대상 구조체로 간단하게 변환합니다.
<code class="go">import ( "encoding/json" ) // Source struct with snake_case tags type ESModel struct { AB string `json:"a_b"` } // Target struct with camelCase tags type APIModel struct { AB string `json:"aB"` } func ConvertKeys(json []byte) []byte { var x ESModel json.Unmarshal(b, &x) b, _ = json.MarshalIndent(APIModel(x), "", " ") return b }</code>
방법 2: 맵 키를 재귀적으로 변환
더 복잡한 JSON 문서의 경우 이를 맵으로 역마샬링해 볼 수 있습니다. 성공하면 맵 내의 모든 키와 값에 키 변환 함수를 반복적으로 적용합니다.
<code class="go">import ( "bytes" "encoding/json" "fmt" "strings" ) func ConvertKeys(j json.RawMessage) json.RawMessage { m := make(map[string]json.RawMessage) if err := json.Unmarshal([]byte(j), &m); err != nil { // Not a JSON object return j } for k, v := range m { fixed := fixKey(k) delete(m, k) m[fixed] = convertKeys(v) } b, err := json.Marshal(m) if err != nil { return j } return json.RawMessage(b) } func fixKey(key string) string { return strings.ToUpper(key) }</code>
위 내용은 Go를 사용하여 JSON에서 Snake-Case를 CamelCase 키로 변환하는 방법은 무엇입니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
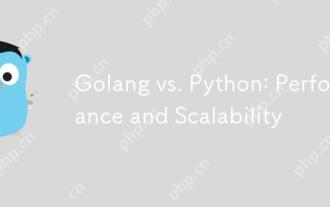
Golang은 성능과 확장 성 측면에서 Python보다 낫습니다. 1) Golang의 컴파일 유형 특성과 효율적인 동시성 모델은 높은 동시성 시나리오에서 잘 수행합니다. 2) 해석 된 언어로서 파이썬은 천천히 실행되지만 Cython과 같은 도구를 통해 성능을 최적화 할 수 있습니다.
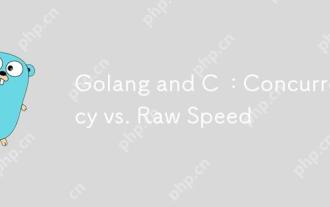
Golang은 동시성에서 C보다 낫고 C는 원시 속도에서 Golang보다 낫습니다. 1) Golang은 Goroutine 및 Channel을 통해 효율적인 동시성을 달성하며, 이는 많은 동시 작업을 처리하는 데 적합합니다. 2) C 컴파일러 최적화 및 표준 라이브러리를 통해 하드웨어에 가까운 고성능을 제공하며 극도의 최적화가 필요한 애플리케이션에 적합합니다.
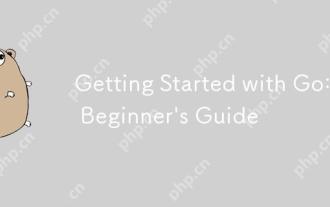
goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity, 효율성, 및 콘크리 론 피처
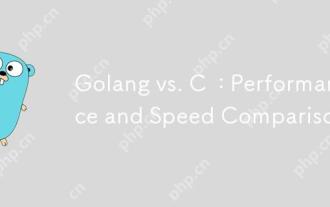
Golang은 빠른 개발 및 동시 시나리오에 적합하며 C는 극도의 성능 및 저수준 제어가 필요한 시나리오에 적합합니다. 1) Golang은 쓰레기 수집 및 동시성 메커니즘을 통해 성능을 향상시키고, 고전성 웹 서비스 개발에 적합합니다. 2) C는 수동 메모리 관리 및 컴파일러 최적화를 통해 궁극적 인 성능을 달성하며 임베디드 시스템 개발에 적합합니다.
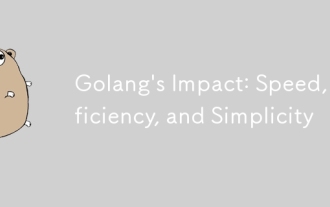
goimpactsdevelopmentpositively throughlyspeed, 효율성 및 단순성.
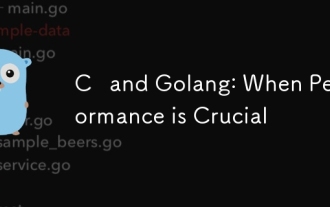
C는 하드웨어 리소스 및 고성능 최적화가 직접 제어되는 시나리오에 더 적합하지만 Golang은 빠른 개발 및 높은 동시성 처리가 필요한 시나리오에 더 적합합니다. 1.C의 장점은 게임 개발과 같은 고성능 요구에 적합한 하드웨어 특성 및 높은 최적화 기능에 가깝습니다. 2. Golang의 장점은 간결한 구문 및 자연 동시성 지원에 있으며, 이는 동시성 서비스 개발에 적합합니다.
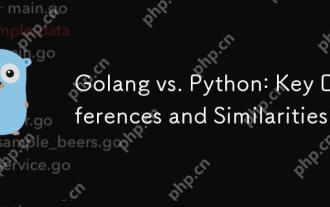
Golang과 Python은 각각 고유 한 장점이 있습니다. Golang은 고성능 및 동시 프로그래밍에 적합하지만 Python은 데이터 과학 및 웹 개발에 적합합니다. Golang은 동시성 모델과 효율적인 성능으로 유명하며 Python은 간결한 구문 및 풍부한 라이브러리 생태계로 유명합니다.
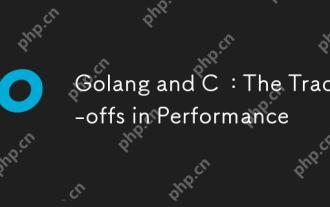
Golang과 C의 성능 차이는 주로 메모리 관리, 컴파일 최적화 및 런타임 효율에 반영됩니다. 1) Golang의 쓰레기 수집 메커니즘은 편리하지만 성능에 영향을 줄 수 있습니다. 2) C의 수동 메모리 관리 및 컴파일러 최적화는 재귀 컴퓨팅에서 더 효율적입니다.
