코드를 기반으로 섹션에서 임의의 JSON 데이터를 정렬 해제하는 방법은 무엇입니까?
JSON에서 임의 데이터 언마샬링
특정 시나리오에서는 부분 또는 섹션에서 JSON 데이터를 언마샬링해야 할 수도 있습니다. 예를 들어, 데이터의 위쪽 절반은 아래쪽 절반에서 수행할 작업을 나타낼 수 있습니다(예: 위쪽 절반의 "코드"를 기반으로 특정 구조체로 역마샬링).
다음 샘플 구조를 고려하세요.
<code class="go">type Range struct { Start int End int } type User struct { ID int Pass int }</code>
예를 들어 JSON 데이터는 다음과 유사할 수 있습니다.
<code class="json">// Message with "Code" 4, signifying a Range structure { "Code": 4, "Payload": { "Start": 1, "End": 10 } } // Message with "Code" 3, signifying a User structure { "Code": 3, "Payload": { "ID": 1, "Pass": 1234 } }</code>
이러한 역정렬화를 달성하려면 다음과 같은 결정이 나올 때까지 JSON 데이터의 아래쪽 절반에 대한 구문 분석을 지연할 수 있습니다. 위쪽 절반에 있는 코드입니다. 다음 접근 방식을 고려하세요.
<code class="go">import ( "encoding/json" "fmt" ) type Message struct { Code int Payload json.RawMessage // Delay parsing until we know the code } func MyUnmarshall(m []byte) { var message Message var payload interface{} json.Unmarshal(m, &message) // Delay parsing until we know the color space switch message.Code { case 3: payload = new(User) case 4: payload = new(Range) } json.Unmarshal(message.Payload, payload) // Error checks omitted for readability fmt.Printf("\n%v%+v", message.Code, payload) // Perform actions on the data } func main() { json := []byte(`{"Code": 4, "Payload": {"Start": 1, "End": 10}}`) MyUnmarshall(json) json = []byte(`{"Code": 3, "Payload": {"ID": 1, "Pass": 1234}}`) MyUnmarshall(json) }</code>
이 접근 방식을 사용하면 지정된 코드에 따라 섹션의 임의 JSON 데이터를 효과적으로 역마샬링할 수 있습니다.
위 내용은 코드를 기반으로 섹션에서 임의의 JSON 데이터를 정렬 해제하는 방법은 무엇입니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
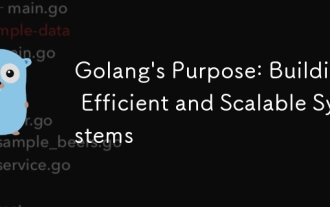
Go Language는 효율적이고 확장 가능한 시스템을 구축하는 데 잘 작동합니다. 장점은 다음과 같습니다. 1. 고성능 : 기계 코드로 컴파일, 빠른 달리기 속도; 2. 동시 프로그래밍 : 고어 라틴 및 채널을 통한 멀티 태스킹 단순화; 3. 단순성 : 간결한 구문, 학습 및 유지 보수 비용 절감; 4. 크로스 플랫폼 : 크로스 플랫폼 컴파일, 쉬운 배포를 지원합니다.
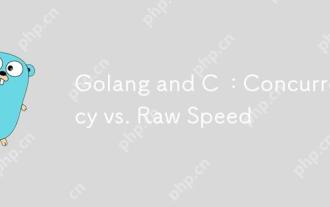
Golang은 동시성에서 C보다 낫고 C는 원시 속도에서 Golang보다 낫습니다. 1) Golang은 Goroutine 및 Channel을 통해 효율적인 동시성을 달성하며, 이는 많은 동시 작업을 처리하는 데 적합합니다. 2) C 컴파일러 최적화 및 표준 라이브러리를 통해 하드웨어에 가까운 고성능을 제공하며 극도의 최적화가 필요한 애플리케이션에 적합합니다.
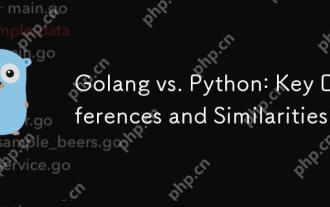
Golang과 Python은 각각 고유 한 장점이 있습니다. Golang은 고성능 및 동시 프로그래밍에 적합하지만 Python은 데이터 과학 및 웹 개발에 적합합니다. Golang은 동시성 모델과 효율적인 성능으로 유명하며 Python은 간결한 구문 및 풍부한 라이브러리 생태계로 유명합니다.
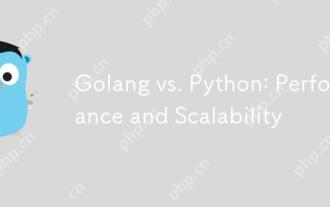
Golang은 성능과 확장 성 측면에서 Python보다 낫습니다. 1) Golang의 컴파일 유형 특성과 효율적인 동시성 모델은 높은 동시성 시나리오에서 잘 수행합니다. 2) 해석 된 언어로서 파이썬은 천천히 실행되지만 Cython과 같은 도구를 통해 성능을 최적화 할 수 있습니다.
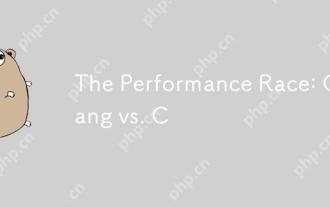
Golang과 C는 각각 공연 경쟁에서 고유 한 장점을 가지고 있습니다. 1) Golang은 높은 동시성과 빠른 발전에 적합하며 2) C는 더 높은 성능과 세밀한 제어를 제공합니다. 선택은 프로젝트 요구 사항 및 팀 기술 스택을 기반으로해야합니다.
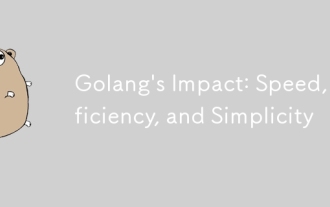
goimpactsdevelopmentpositively throughlyspeed, 효율성 및 단순성.
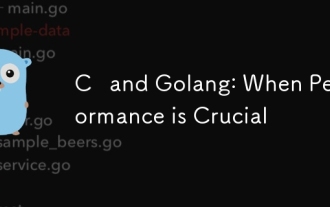
C는 하드웨어 리소스 및 고성능 최적화가 직접 제어되는 시나리오에 더 적합하지만 Golang은 빠른 개발 및 높은 동시성 처리가 필요한 시나리오에 더 적합합니다. 1.C의 장점은 게임 개발과 같은 고성능 요구에 적합한 하드웨어 특성 및 높은 최적화 기능에 가깝습니다. 2. Golang의 장점은 간결한 구문 및 자연 동시성 지원에 있으며, 이는 동시성 서비스 개발에 적합합니다.
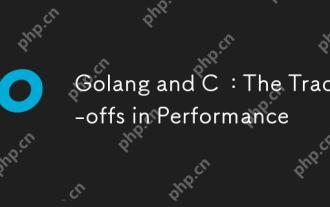
Golang과 C의 성능 차이는 주로 메모리 관리, 컴파일 최적화 및 런타임 효율에 반영됩니다. 1) Golang의 쓰레기 수집 메커니즘은 편리하지만 성능에 영향을 줄 수 있습니다. 2) C의 수동 메모리 관리 및 컴파일러 최적화는 재귀 컴퓨팅에서 더 효율적입니다.
