배열 프로토타입 - JavaScript 과제
이 게시물의 모든 코드는 repo Github에서 확인하실 수 있습니다.
어레이 프로토타입 관련 과제
Array.prototype.at()
/** * @param {number} index * @return {any | undefiend} */ Array.prototype.myAt = function (index) { const len = this.length; if (index < -len || index >= len) { return; } return this[(index + len) % len]; }; // Usage example console.log([1, 2, 3, 4].myAt(2)); // => 3 console.log([1, 2, 3, 4].myAt(-1)); // => 4 console.log([1, 2, 3, 4].myAt(5)); // => undefined
Array.prototype.concat()
/** * @template T * @param {...(T | Array<T>)} itemes * @return {Array<T>} */ Array.prototype.myConcat = function (...items) { const newArray = [...this]; for (const item of items) { if (Array.isArray(item)) { newArray.push(...item); } else { newArray.push(item); } } return newArray; }; // Usage example console.log([1, 2, 3].myConcat([])); // => [1, 2, 3]; console.log([1, 2, 3].myConcat([4, 5, 6, [2]])); // => [1, 2, 3, 4, 5, 6, [2]];
Array.prototype.every()
/** * @template T * @param { (value: T, index: number, array: Array<T>) => boolean } callbackFn * @param {any} [thisArg] * @return {boolean} */ Array.prototype.myEvery = function (callbackFn, thisArg) { const len = this.length; let flag = true; for (let i = 0; i < len; i += 1) { if (Object.hasOwn(this, i) && !callbackFn.call(thisArg, this[i], i, this)) { flag = false; break; } } return flag; }; // Usage example console.log([1, 2, 3].myEvery((item) => item > 2)); // => false console.log([1, 2, 3].myEvery((item) => item > 0)); // => true
배열.프로토타입.필터()
/** * @template T, U * @param { (value: T, index: number, array: Array<T>) => boolean } callbackFn * @param { any } [thisArg] * @return {Array<T>} */ Array.prototype.myFilter = function (callbackFn, thisArg) { const newArray = []; for (let i = 0; i < this.length; i += 1) { if (Object.hasOwn(this, i) && callbackFn.call(thisArg, this[i], i, this)) { newArray.push(this[i]); } } return newArray; }; // Usage example console.log([1, 2, 3, 4].myFilter((value) => value % 2 == 0)); // => [2, 4] console.log([1, 2, 3, 4].myFilter((value) => value < 3)); // => [1, 2]
배열.프로토타입.플랫()
/** * @param { Array } arr * @param { number } depth * @returns { Array } */ function flatten(arr, depth = 1) { const newArray = []; for (let i = 0; i < arr.length; i += 1) { if (Array.isArray(arr[i]) && depth !== 0) { newArray.push(...flatten(arr[i], depth - 1)); } else { newArray.push(arr[i]); } } return newArray; } // Usage example const words = ["spray", "elite", "exuberant", "destruction", "present"]; const result = words.filter((word) => word.length > 6); console.log(result); // => ["exuberant", "destruction", "present"]
Array.prototype.forEach()
/** * @template T, U * @param { (value: T, index: number, array: Array<T>) => U } callbackFn * @param {any} [thisArg] * @return {Array<U>} */ Array.prototype.myForEach = function (callbackFn, thisArg) { if (this == null) { throw new TypeError("this is null or not defined"); } if (typeof callbackFn !== "function") { throw new TypeError(callbackFn + " is not a function"); } const O = Object(this); // Zero-fill Right Shift to ensure that the result if always non-negative. const len = O.length >>> 0; for (let i = 0; i < len; i += 1) { if (Object.hasOwn(O, i)) { callbackFn.call(thisArg, O[i], i, O); } } }; // Usage example console.log( [1, 2, 3].myForEach((el) => el * el), null ); // => [1, 4, 9];
Array.prototype.indexOf()
/** * @param {any} searchElement * @param {number} fromIndex * @return {number} */ Array.prototype.myIndexOf = function (searchElement, fromIndex = 0) { const len = this.length; if (fromIndex < 0) { fromIndex = Math.max(0, fromIndex + this.length); } for (let i = fromIndex; i < len; i += 1) { if (this[i] === searchElement) { return i; } } return -1; } // Usage example console.log([1, 2, 3, 4, 5].myIndexOf(3)); // => 2 console.log([1, 2, 3, 4, 5].myIndexOf(6)); // => -1 console.log([1, 2, 3, 4, 5].myIndexOf(1)); // => 0 console.log(['a', 'b', 'c'].myIndexOf('b')); // => 1 console.log([NaN].myIndexOf(NaN)); // => -1 (since NaN !== NaN)
배열.프로토타입.라스트()
/** * @return {null|boolean|number|string|Array|Object} */ Array.prototype.myLast = function () { return this.length ? this.at(-1) : -1; }; // Usage example console.log([].myLast()); // => -1; console.log([1].myLast()); // => 1 console.log([1, 2].myLast()); // => 2
Array.prototype.map()
/** * @template T, U * @param { (value: T, index: number, array: Array<T>) => U } callbackFn * @param {any} [thisArg] * @return {Array<U>} */ Array.prototype.myMap = function (callbackFn, thisArg) { const len = this.length; const newArray = Array.from({ length: len }); for (let i = 0; i < len; i += 1) { if (Object.hasOwn(this, i)) { newArray[i] = callbackFn.call(thisArg, this[i], i, this); } } return newArray; }; // Usage example console.log([1, 2, 3, 4].myMap((i) => i)); // => [1, 2, 3, 4] console.log([1, 2, 3, 4].myMap((i) => i * i)); // => [1, 4, 9, 16])
배열.프로토타입.리듀스()
/** * @template T, U * @param { (previousValue: U, currentValue: T, currentIndex: number, array: Array<T>) => U } callbackFn * @param {U} [initialValue] * @return {U} */ Array.prototype.myReduce = function (callbackFn, initialValue) { const hasInitialValue = initialValue !== undefined; const len = this.length; if (!hasInitialValue && !len) { throw new Error("Reduce of empty array with no initial value"); } let accumulator = hasInitialValue ? initialValue : this[0]; let startingIndex = hasInitialValue ? 0 : 1; for (let i = startingIndex; i < len; i += 1) { if (Object.hasOwn(this, i)) { accumulator = callbackFn(accumulator, this[i], i, this); } } return accumulator; }; // Usage example const numbers = [1, 2, 3, 4, 5]; const sum = numbers.myReduce((acc, num) => acc + num, 0); console.log(sum); // => 15 const products = numbers.myReduce((acc, num) => acc * num, 1);
Array.prototype.some()
/** * @template T * @param { (value: T, index: number, array: Array<T>) => boolean } callbackFn * @param {any} [thisArg] * @return {boolean} */ Array.prototype.mySome = function (callbackFn, thisArg) { const len = this.length; let flag = false; for (let i = 0; i < len; i += 1) { if (Object.hasOwn(this, i) && callbackFn.call(thisArg, this[i], i, this)) { flag = true; break; } } return flag; }; // Usage example console.log([1, 2, 3].mySome((item) => item > 2)); // => true console.log([1, 2, 3].mySome((item) => item < 0)); // => false
Array.prototype.square()
/** * @return {Array<number>} */ Array.prototype.mySquare = function () { const len = this.length; const newArray = Array.from({ length: len }); for (let i = 0; i < len; i += 1) { newArray[i] = this[i] * this[i]; } return newArray; }; // Usage example console.log([1, 2, 3].mySquare()); // => [1, 4, 9]; console.log([].mySquare()); // => [];
참조
- 그레이트프론트엔드
- Array.prototype.at() - MDN
- Array.prototype.concat() - MDN
- Array.prototype.every() - MDN
- Array.prototype.filter() - MDN
- Array.prototype.Flat() - MDN
- Array.prototype.forEach() - MDN
- Array.prototype.indexOf() - MDN
- Array.prototype.map() - MDN
- Array.prototype.reduce() - MDN
- Array.prototype.some() - MDN
- 2635. 배열의 각 요소에 변환 적용 - LeetCode
- 2634. 배열의 요소 필터링 - LeetCode
- 2626. 배열 축소 변환 - LeetCode
- 2619. 배열 프로토타입 마지막 - LeetCode
- 2625. 깊게 중첩된 배열 평면화 - LeetCode
- 3. Array.prototype.Flat() 구현 - BFE.dev
- 151. Array.prototype.map() 구현 - BFE.dev
- 146. Array.prototype.reduce() 구현 - BFE.dev
위 내용은 배열 프로토타입 - JavaScript 과제의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










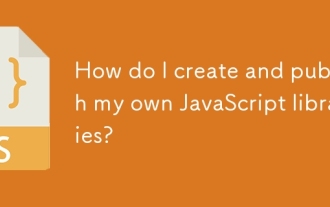
기사는 JavaScript 라이브러리 작성, 게시 및 유지 관리, 계획, 개발, 테스트, 문서 및 홍보 전략에 중점을 둡니다.
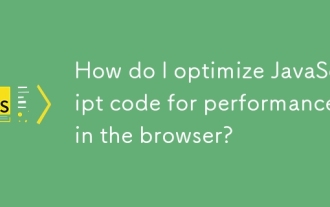
이 기사는 브라우저에서 JavaScript 성능을 최적화하기위한 전략에 대해 설명하고 실행 시간을 줄이고 페이지로드 속도에 미치는 영향을 최소화하는 데 중점을 둡니다.
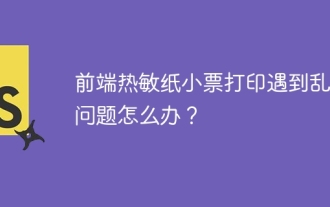
프론트 엔드 개발시 프론트 엔드 열지대 티켓 인쇄를위한 자주 묻는 질문과 솔루션, 티켓 인쇄는 일반적인 요구 사항입니다. 그러나 많은 개발자들이 구현하고 있습니다 ...
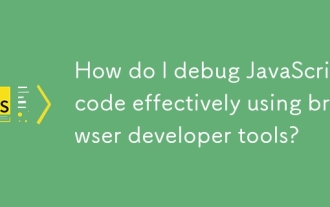
이 기사는 브라우저 개발자 도구를 사용하여 효과적인 JavaScript 디버깅, 중단 점 설정, 콘솔 사용 및 성능 분석에 중점을 둡니다.
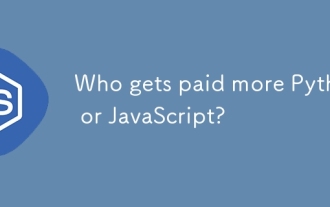
기술 및 산업 요구에 따라 Python 및 JavaScript 개발자에 대한 절대 급여는 없습니다. 1. 파이썬은 데이터 과학 및 기계 학습에서 더 많은 비용을 지불 할 수 있습니다. 2. JavaScript는 프론트 엔드 및 풀 스택 개발에 큰 수요가 있으며 급여도 상당합니다. 3. 영향 요인에는 경험, 지리적 위치, 회사 규모 및 특정 기술이 포함됩니다.
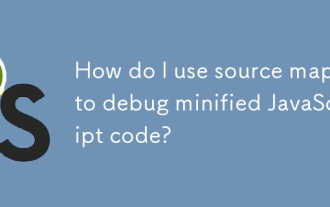
이 기사는 소스 맵을 사용하여 원래 코드에 다시 매핑하여 미니어링 된 JavaScript를 디버그하는 방법을 설명합니다. 소스 맵 활성화, 브레이크 포인트 설정 및 Chrome Devtools 및 Webpack과 같은 도구 사용에 대해 설명합니다.
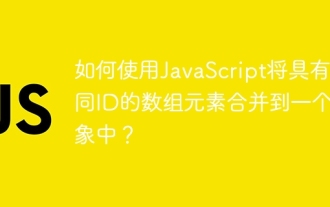
동일한 ID로 배열 요소를 JavaScript의 하나의 객체로 병합하는 방법은 무엇입니까? 데이터를 처리 할 때 종종 동일한 ID를 가질 필요가 있습니다 ...
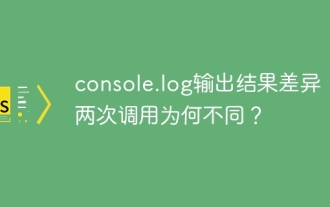
Console.log 출력의 차이의 근본 원인에 대한 심층적 인 논의. 이 기사에서는 Console.log 함수의 출력 결과의 차이점을 코드에서 분석하고 그에 따른 이유를 설명합니다. � ...
