PyTorch에서는 isclose이고 같음
커피 한잔 사주세요😄
*메모:
- 내 게시물에서는 eq() 및 ne()에 대해 설명합니다.
- 내 게시물에서는 gt() 및 lt()에 대해 설명합니다.
- 내 게시물에는 ge() 및 le()에 대한 설명이 나와 있습니다.
- 내 게시물에는 torch.nan과 torch.inf가 설명되어 있습니다.
isclose()는 첫 번째 0D 이상의 D 텐서의 0개 이상의 요소가 두 번째 0D 이상의 D 텐서의 0개 이상의 요소와 요소별로 같거나 거의 같은지 확인하여 0D 이상의 요소를 얻을 수 있습니다. 아래와 같이 0개 이상의 요소로 구성된 D 텐서:
*메모:
- isclose()는 토치나 텐서와 함께 사용할 수 있습니다.
- 토치 또는 텐서(필수 유형: int, float, complex 또는 bool의 텐서)를 사용하는 첫 번째 인수(입력).
- torch의 두 번째 인수 또는 텐서의 첫 번째 인수는 other(필수 유형: int, float, complex 또는 bool의 텐서)입니다.
- torch의 세 번째 인수 또는 tensor의 두 번째 인수는 rtol(Optional-Default:1e-05-Type:float)입니다.
- torch의 네 번째 인수 또는 텐서의 세 번째 인수는 atol(Optional-Default:1e-08-Type:float)입니다.
- torch의 5번째 인수 또는 텐서의 4번째 인수는 equal_nan(Optional-Default:False-Type:bool)입니다.
*메모:
- True이면 nan과 nan은 True를 반환합니다.
- 기본적으로 nan과 nan은 False를 반환합니다.
- 공식은 |입력 - 기타| <= rtol x |기타| 아톨.
import torch tensor1 = torch.tensor([1.00001001, 1.00000996, 1.00000995, torch.nan]) tensor2 = torch.tensor([1., 1., 1., torch.nan]) torch.isclose(input=tensor1, other=tensor2) torch.isclose(input=tensor1, other=tensor2, rtol=1e-05, atol=1e-08, equal_nan=False) # 0.00001 # 0.00000001 tensor1.isclose(other=tensor2) torch.isclose(input=tensor2, other=tensor1) # tensor([False, False, True, False]) torch.isclose(input=tensor1, other=tensor2, equal_nan=True) # tensor([False, False, True, True]) tensor1 = torch.tensor([[1.00001001, 1.00000996], [1.00000995, torch.nan]]) tensor2 = torch.tensor([[1., 1.], [1., torch.nan]]) torch.isclose(input=tensor1, other=tensor2) torch.isclose(input=tensor2, other=tensor1) # tensor([[False, False], # [True, False]]) tensor1 = torch.tensor([[[1.00001001], [1.00000996]], [[1.00000995], [torch.nan]]]) tensor2 = torch.tensor([[[1.], [1.]], [[1.], [torch.nan]]]) torch.isclose(input=tensor1, other=tensor2) torch.isclose(input=tensor2, other=tensor1) # tensor([[[False], [False]], # [[True], [False]]]) tensor1 = torch.tensor([[1.00001001, 1.00000996], [1.00000995, torch.nan]]) tensor2 = torch.tensor([1., 1.]) torch.isclose(input=tensor1, other=tensor2) torch.isclose(input=tensor2, other=tensor1) # tensor([[False, False], # [True, False]]) tensor1 = torch.tensor([[1.00001001, 1.00000996], [1.00000995, torch.nan]]) tensor2 = torch.tensor(1.) torch.isclose(input=tensor1, other=tensor2) torch.isclose(input=tensor2, other=tensor1) # tensor([[False, False], # [True, False]]) tensor1 = torch.tensor([0, 1, 2]) tensor2 = torch.tensor(1) torch.isclose(input=tensor1, other=tensor2) # tensor([False, True, False]) tensor1 = torch.tensor([0.+0.j, 1.+0.j, 2.+0.j]) tensor2 = torch.tensor(1.+0.j) torch.isclose(input=tensor1, other=tensor2) # tensor([False, True, False]) tensor1 = torch.tensor([False, True, False]) tensor2 = torch.tensor(True) torch.isclose(input=tensor1, other=tensor2) # tensor([False, True, False])
equal()은 0D 이상의 D 텐서 중 두 개가 동일한 크기와 요소를 가지고 있는지 확인하여 아래와 같이 부울 값의 스칼라를 가져올 수 있습니다.
*메모:
- equal()은 토치나 텐서와 함께 사용할 수 있습니다.
- 토치 또는 텐서(필수 유형: int, float, complex 또는 bool의 텐서)를 사용하는 첫 번째 인수(입력).
- torch의 두 번째 인수 또는 텐서의 첫 번째 인수는 other(필수 유형: int, float, complex 또는 bool의 텐서)입니다.
import torch tensor1 = torch.tensor([5, 9, 3]) tensor2 = torch.tensor([5, 9, 3]) torch.equal(input=tensor1, other=tensor2) tensor1.equal(other=tensor2) torch.equal(input=tensor2, other=tensor1) # True tensor1 = torch.tensor([5, 9, 3]) tensor2 = torch.tensor([7, 9, 3]) torch.equal(input=tensor1, other=tensor2) torch.equal(input=tensor2, other=tensor1) # False tensor1 = torch.tensor([5, 9, 3]) tensor2 = torch.tensor([[5, 9, 3]]) torch.equal(input=tensor1, other=tensor2) torch.equal(input=tensor2, other=tensor1) # False tensor1 = torch.tensor([5., 9., 3.]) tensor2 = torch.tensor([5.+0.j, 9.+0.j, 3.+0.j]) torch.equal(input=tensor1, other=tensor2) torch.equal(input=tensor2, other=tensor1) # True tensor1 = torch.tensor([1.+0.j, 0.+0.j, 1.+0.j]) tensor2 = torch.tensor([True, False, True]) torch.equal(input=tensor1, other=tensor2) torch.equal(input=tensor2, other=tensor1) # True tensor1 = torch.tensor([], dtype=torch.int64) tensor2 = torch.tensor([], dtype=torch.float32) torch.equal(input=tensor1, other=tensor2) torch.equal(input=tensor2, other=tensor1) # True
위 내용은 PyTorch에서는 isclose이고 같음의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
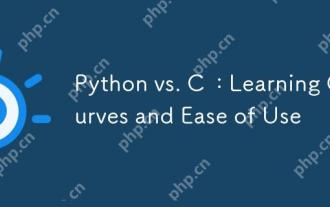
Python은 배우고 사용하기 쉽고 C는 더 강력하지만 복잡합니다. 1. Python Syntax는 간결하며 초보자에게 적합합니다. 동적 타이핑 및 자동 메모리 관리를 사용하면 사용하기 쉽지만 런타임 오류가 발생할 수 있습니다. 2.C는 고성능 응용 프로그램에 적합한 저수준 제어 및 고급 기능을 제공하지만 학습 임계 값이 높고 수동 메모리 및 유형 안전 관리가 필요합니다.
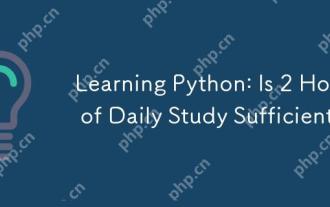
하루에 2 시간 동안 파이썬을 배우는 것으로 충분합니까? 목표와 학습 방법에 따라 다릅니다. 1) 명확한 학습 계획을 개발, 2) 적절한 학습 자원 및 방법을 선택하고 3) 실습 연습 및 검토 및 통합 연습 및 검토 및 통합,이 기간 동안 Python의 기본 지식과 고급 기능을 점차적으로 마스터 할 수 있습니다.
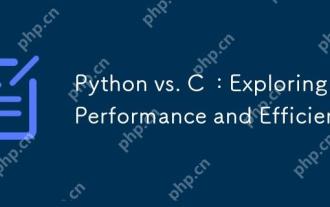
Python은 개발 효율에서 C보다 낫지 만 C는 실행 성능이 높습니다. 1. Python의 간결한 구문 및 풍부한 라이브러리는 개발 효율성을 향상시킵니다. 2.C의 컴파일 유형 특성 및 하드웨어 제어는 실행 성능을 향상시킵니다. 선택할 때는 프로젝트 요구에 따라 개발 속도 및 실행 효율성을 평가해야합니다.
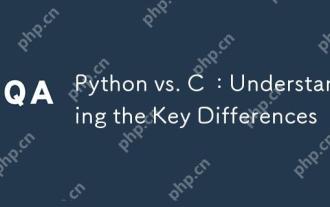
Python과 C는 각각 고유 한 장점이 있으며 선택은 프로젝트 요구 사항을 기반으로해야합니다. 1) Python은 간결한 구문 및 동적 타이핑으로 인해 빠른 개발 및 데이터 처리에 적합합니다. 2) C는 정적 타이핑 및 수동 메모리 관리로 인해 고성능 및 시스템 프로그래밍에 적합합니다.
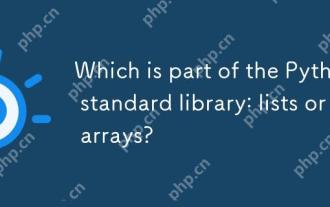
Pythonlistsarepartoftsandardlardlibrary, whileraysarenot.listsarebuilt-in, 다재다능하고, 수집 할 수있는 반면, arraysarreprovidedByTearRaymoduledlesscommonlyusedDuetolimitedFunctionality.
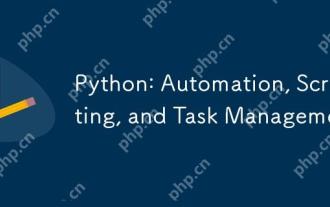
파이썬은 자동화, 스크립팅 및 작업 관리가 탁월합니다. 1) 자동화 : 파일 백업은 OS 및 Shutil과 같은 표준 라이브러리를 통해 실현됩니다. 2) 스크립트 쓰기 : PSUTIL 라이브러리를 사용하여 시스템 리소스를 모니터링합니다. 3) 작업 관리 : 일정 라이브러리를 사용하여 작업을 예약하십시오. Python의 사용 편의성과 풍부한 라이브러리 지원으로 인해 이러한 영역에서 선호하는 도구가됩니다.
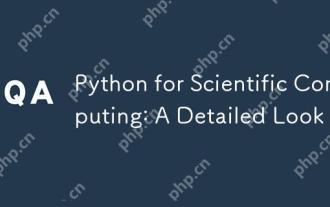
과학 컴퓨팅에서 Python의 응용 프로그램에는 데이터 분석, 머신 러닝, 수치 시뮬레이션 및 시각화가 포함됩니다. 1.numpy는 효율적인 다차원 배열 및 수학적 함수를 제공합니다. 2. Scipy는 Numpy 기능을 확장하고 최적화 및 선형 대수 도구를 제공합니다. 3. 팬더는 데이터 처리 및 분석에 사용됩니다. 4. matplotlib는 다양한 그래프와 시각적 결과를 생성하는 데 사용됩니다.
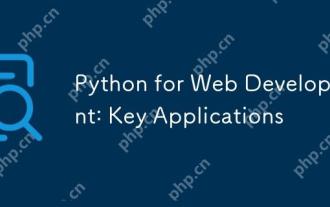
웹 개발에서 Python의 주요 응용 프로그램에는 Django 및 Flask 프레임 워크 사용, API 개발, 데이터 분석 및 시각화, 머신 러닝 및 AI 및 성능 최적화가 포함됩니다. 1. Django 및 Flask 프레임 워크 : Django는 복잡한 응용 분야의 빠른 개발에 적합하며 플라스크는 소형 또는 고도로 맞춤형 프로젝트에 적합합니다. 2. API 개발 : Flask 또는 DjangorestFramework를 사용하여 RESTFULAPI를 구축하십시오. 3. 데이터 분석 및 시각화 : Python을 사용하여 데이터를 처리하고 웹 인터페이스를 통해 표시합니다. 4. 머신 러닝 및 AI : 파이썬은 지능형 웹 애플리케이션을 구축하는 데 사용됩니다. 5. 성능 최적화 : 비동기 프로그래밍, 캐싱 및 코드를 통해 최적화
