std::forward가 ID 템플릿을 사용하여 템플릿 인수 공제를 비활성화하는 이유는 무엇입니까?
올바른 전달을 보장하기 위해 std::forward를 사용하여 템플릿 인수 추론 비활성화
VS2010에서 std::forward 정의를 고려하세요.
<code class="cpp">template<class _Ty> inline _Ty&& forward(typename identity<_Ty>::type& _Arg) { // forward _Arg, given explicitly specified type parameter return ((_Ty&&)_Arg); }</code>
ID 템플릿의 목적은 템플릿을 비활성화하는 것입니다. 인수 공제. 이 시나리오에서 이것이 중요한 이유는 무엇입니까?
템플릿 인수 추론은 잘못된 유형 추론으로 이어질 수 있습니다. X 유형의 객체에 대한 rvalue 참조가 매개변수 유형 T&를 사용하여 템플릿 함수에 전달되면 템플릿 인수 추론은 T를 X로 추론하여 매개변수 유형 X&가 됩니다. 그러나 완벽한 전달을 위해 매개변수에는 이름이 있으므로 lvalue입니다. 따라서 std::forward에서 템플릿 인수 추론을 사용하면 추론된 매개변수 유형이 lvalue 참조 또는 const lvalue 참조가 됩니다.
<code class="cpp">template<typename T> T&& forward_with_deduction(T&& obj) { return static_cast<T&&>(obj); }</code>
다음 예를 고려하세요.
<code class="cpp">void test(int&){} void test(const int&){} void test(int&&){} template<typename T> void perfect_forwarder(T&& obj) { test(forward_with_deduction(obj)); } int main() { int x; const int& y(x); int&& z = std::move(x); test(forward_with_deduction(7)); // 7 is an int&&, correctly calls test(int&&) test(forward_with_deduction(z)); // z is treated as an int&, calls test(int&) // All the below call test(int&) or test(const int&) because in perfect_forwarder 'obj' is treated as // an int& or const int& (because it is named) so T in forward_with_deduction is deduced as int& // or const int&. The T&& in static_cast<T&&>(obj) then collapses to int& or const int& - which is not what // we want in the bottom two cases. perfect_forwarder(x); perfect_forwarder(y); perfect_forwarder(std::move(x)); perfect_forwarder(std::move(y)); }</code>
이 예에서는 Perfect_forwarder의 매개변수가 이름 때문에 lvalue 또는 const lvalue 참조로 처리되기 때문에 완벽한 전달이 실패합니다. 이로 인해 Forward_with_deduction에서 잘못된 유형 추론이 발생하여 원하지 않는 static_cast 의미 체계가 발생합니다.
std::forward의 ID 템플릿을 사용하여 템플릿 인수 추론을 비활성화하면 std::forward가 항상 rvalue 참조를 반환합니다. lvalue와 rvalue의 완벽한 전달을 수정하세요.
위 내용은 std::forward가 ID 템플릿을 사용하여 템플릿 인수 공제를 비활성화하는 이유는 무엇입니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
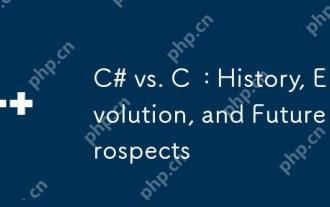
C#과 C의 역사와 진화는 독특하며 미래의 전망도 다릅니다. 1.C는 1983 년 Bjarnestroustrup에 의해 발명되어 객체 지향 프로그래밍을 C 언어에 소개했습니다. Evolution 프로세스에는 자동 키워드 소개 및 Lambda Expressions 소개 C 11, C 20 도입 개념 및 코 루틴과 같은 여러 표준화가 포함되며 향후 성능 및 시스템 수준 프로그래밍에 중점을 둘 것입니다. 2.C#은 2000 년 Microsoft에 의해 출시되었으며 C와 Java의 장점을 결합하여 진화는 단순성과 생산성에 중점을 둡니다. 예를 들어, C#2.0은 제네릭과 C#5.0 도입 된 비동기 프로그래밍을 소개했으며, 이는 향후 개발자의 생산성 및 클라우드 컴퓨팅에 중점을 둘 것입니다.
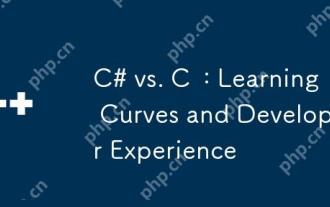
C# 및 C 및 개발자 경험의 학습 곡선에는 상당한 차이가 있습니다. 1) C#의 학습 곡선은 비교적 평평하며 빠른 개발 및 기업 수준의 응용 프로그램에 적합합니다. 2) C의 학습 곡선은 가파르고 고성능 및 저수준 제어 시나리오에 적합합니다.
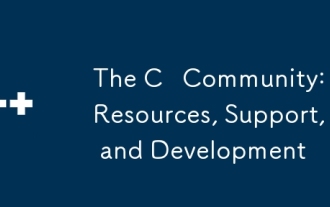
C 학습자와 개발자는 StackoverFlow, Reddit의 R/CPP 커뮤니티, Coursera 및 EDX 코스, GitHub의 오픈 소스 프로젝트, 전문 컨설팅 서비스 및 CPPCon에서 리소스와 지원을받을 수 있습니다. 1. StackoverFlow는 기술적 인 질문에 대한 답변을 제공합니다. 2. Reddit의 R/CPP 커뮤니티는 최신 뉴스를 공유합니다. 3. Coursera와 Edx는 공식적인 C 과정을 제공합니다. 4. LLVM 및 부스트 기술 향상과 같은 GitHub의 오픈 소스 프로젝트; 5. JetBrains 및 Perforce와 같은 전문 컨설팅 서비스는 기술 지원을 제공합니다. 6. CPPCON 및 기타 회의는 경력을 돕습니다
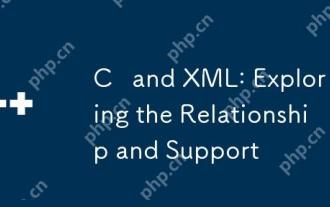
C는 XML과 타사 라이브러리 (예 : TinyXML, Pugixml, Xerces-C)와 상호 작용합니다. 1) 라이브러리를 사용하여 XML 파일을 구문 분석하고 C- 처리 가능한 데이터 구조로 변환하십시오. 2) XML을 생성 할 때 C 데이터 구조를 XML 형식으로 변환하십시오. 3) 실제 애플리케이션에서 XML은 종종 구성 파일 및 데이터 교환에 사용되어 개발 효율성을 향상시킵니다.
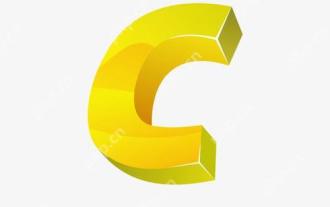
C에서 정적 분석의 적용에는 주로 메모리 관리 문제 발견, 코드 로직 오류 확인 및 코드 보안 개선이 포함됩니다. 1) 정적 분석은 메모리 누출, 이중 릴리스 및 초기화되지 않은 포인터와 같은 문제를 식별 할 수 있습니다. 2) 사용하지 않은 변수, 데드 코드 및 논리적 모순을 감지 할 수 있습니다. 3) Coverity와 같은 정적 분석 도구는 버퍼 오버플로, 정수 오버플로 및 안전하지 않은 API 호출을 감지하여 코드 보안을 개선 할 수 있습니다.
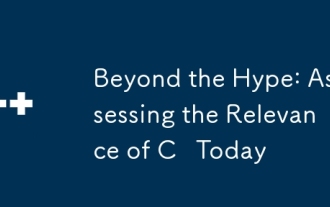
C는 여전히 현대 프로그래밍과 관련이 있습니다. 1) 고성능 및 직접 하드웨어 작동 기능은 게임 개발, 임베디드 시스템 및 고성능 컴퓨팅 분야에서 첫 번째 선택이됩니다. 2) 스마트 포인터 및 템플릿 프로그래밍과 같은 풍부한 프로그래밍 패러다임 및 현대적인 기능은 유연성과 효율성을 향상시킵니다. 학습 곡선은 가파르지만 강력한 기능은 오늘날의 프로그래밍 생태계에서 여전히 중요합니다.
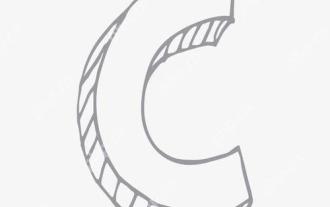
C에서 Chrono 라이브러리를 사용하면 시간과 시간 간격을보다 정확하게 제어 할 수 있습니다. 이 도서관의 매력을 탐구합시다. C의 크로노 라이브러리는 표준 라이브러리의 일부로 시간과 시간 간격을 다루는 현대적인 방법을 제공합니다. 시간과 C 시간으로 고통받는 프로그래머에게는 Chrono가 의심 할 여지없이 혜택입니다. 코드의 가독성과 유지 가능성을 향상시킬뿐만 아니라 더 높은 정확도와 유연성을 제공합니다. 기본부터 시작합시다. Chrono 라이브러리에는 주로 다음 주요 구성 요소가 포함됩니다. std :: Chrono :: System_Clock : 현재 시간을 얻는 데 사용되는 시스템 클럭을 나타냅니다. STD :: 크론
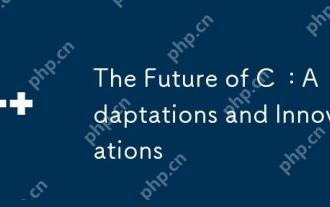
C의 미래는 병렬 컴퓨팅, 보안, 모듈화 및 AI/기계 학습에 중점을 둘 것입니다. 1) 병렬 컴퓨팅은 코 루틴과 같은 기능을 통해 향상 될 것입니다. 2)보다 엄격한 유형 검사 및 메모리 관리 메커니즘을 통해 보안이 향상 될 것입니다. 3) 변조는 코드 구성 및 편집을 단순화합니다. 4) AI 및 머신 러닝은 C가 수치 컴퓨팅 및 GPU 프로그래밍 지원과 같은 새로운 요구에 적응하도록 촉구합니다.
