React와 Firebase를 사용하여 실시간 멀티플레이어 게임 구축: Gladiator Taunt Wars
소개
이 심층 가이드에서는 Gladiator Taunt Wars의 자세한 예를 들어 Firebase와 React를 사용하여 실시간 멀티플레이어 게임을 구축하는 방법을 살펴보겠습니다. 이 게임 모드에서 플레이어는 전략적 도발 결투에 참여하며, 차례대로 도발을 선택하고 대응하여 상대방의 체력(HP)을 줄입니다. 이 문서에서는 Firebase 설정, 매치메이킹, 게임 상태 관리, 애니메이션, 실시간 업데이트 및 ELO 기반 리더보드 통합을 포함하여 이러한 게임 구축의 모든 측면을 다룹니다. 마지막에는 반응성이 뛰어나고 매력적인 실시간 멀티플레이어 경험을 구현하는 방법을 확실하게 이해하게 될 것입니다.
Firebase 설정 및 프로젝트 초기화
Firebase 설정
실시간 데이터 처리 및 플레이어 확인을 위해 Firestore 및 인증으로 Firebase를 초기화합니다. 이는 경기 데이터, 플레이어 정보 및 실시간 순위표 업데이트를 저장하고 관리하기 위한 백본을 제공합니다. 일치 데이터에 대한 액세스를 제한하여 인증된 플레이어만 관련 정보를 보고 업데이트할 수 있도록 Firestore 규칙을 설정하세요.
React 프로젝트 구조
매치메이킹 시스템, 게임 보드, 리더보드, 채팅 등 각 게임 요소를 나타내는 재사용 가능한 구성 요소로 React 프로젝트를 구성하세요. 명확하고 유지 관리 가능한 아키텍처를 위해 구성 요소를 계층적으로 구성합니다.
게임의 주요 구성 요소
- 메인 메뉴 및 매치메이킹 MainMenu 구성 요소는 플레이어에게 옵션을 제공하여 매치메이킹에 참여하고, 통계를 보고, 순위표에 액세스할 수 있도록 합니다. 매치메이킹 구성요소는 일관성을 위해 Firestore 트랜잭션을 활용하여 플레이어를 실시간으로 페어링합니다.
중매 논리
startSearching 함수는 Firestore의 대기열에 플레이어를 추가하여 매치메이킹 프로세스를 시작합니다. 상대가 발견되면 두 플레이어의 ID를 저장하고 게임 매개변수를 초기화하는 새로운 경기 문서가 생성됩니다.
const startSearching = async () => { const user = auth.currentUser; if (user && db) { try { const matchmakingRef = collection(db, 'tauntWars_matchmaking'); const userDocRef = doc(matchmakingRef, user.uid); await runTransaction(db, async (transaction) => { const userDoc = await transaction.get(userDocRef); if (!userDoc.exists()) { transaction.set(userDocRef, { userId: user.uid, status: 'waiting', timestamp: serverTimestamp() }); } else { transaction.update(userDocRef, { status: 'waiting', timestamp: serverTimestamp() }); } const q = query(matchmakingRef, where('status', '==', 'waiting')); const waitingPlayers = await getDocs(q); if (waitingPlayers.size > 1) { // Pairing logic } }); } catch (error) { setIsSearching(false); } } };
이 기능은 Firestore 트랜잭션을 사용하여 플레이어가 매치메이킹 시스템을 방해할 수 있는 이중 매치를 방지합니다. Firebase의 serverTimestamp 기능은 여러 시간대에 걸쳐 일관된 타임스탬프를 보장하는 데 유용합니다.
- GameBoard 구성 요소의 실시간 게임 상태 게임 상태 변경 듣기 GameBoard 구성 요소는 tauntWars_matches 컬렉션의 변경 사항을 수신합니다. 플레이어가 도발을 선택하거나 응답하면 변경 사항이 즉시 Firestore에 반영되어 두 플레이어 모두에 대해 다시 렌더링이 트리거됩니다.
const startSearching = async () => { const user = auth.currentUser; if (user && db) { try { const matchmakingRef = collection(db, 'tauntWars_matchmaking'); const userDocRef = doc(matchmakingRef, user.uid); await runTransaction(db, async (transaction) => { const userDoc = await transaction.get(userDocRef); if (!userDoc.exists()) { transaction.set(userDocRef, { userId: user.uid, status: 'waiting', timestamp: serverTimestamp() }); } else { transaction.update(userDocRef, { status: 'waiting', timestamp: serverTimestamp() }); } const q = query(matchmakingRef, where('status', '==', 'waiting')); const waitingPlayers = await getDocs(q); if (waitingPlayers.size > 1) { // Pairing logic } }); } catch (error) { setIsSearching(false); } } };
게임 단계 처리
플레이어는 차례를 번갈아 가며 각각 도발이나 반응을 선택합니다. currentTurn 속성은 게임이 어떤 작업 단계에 있는지 나타냅니다. 각 작업은 Firestore에서 업데이트되어 두 클라이언트에서 실시간 동기화를 트리거합니다. 예를 들어, 도발을 선택한 플레이어는 currentTurn을 "응답"으로 전환하여 상대에게 응답을 선택하도록 알립니다.
- 플레이어 액션 및 도발 선택 ActionSelection 구성 요소 이 구성요소는 사용 가능한 도발을 표시하고 선택 프로세스를 처리합니다. 플레이어는 Firestore에 저장되고 다음 단계를 트리거하는 도발이나 응답을 선택합니다.
useEffect(() => { const matchRef = doc(db, 'tauntWars_matches', matchId); const unsubscribe = onSnapshot(matchRef, (docSnapshot) => { if (docSnapshot.exists()) { setMatchData(docSnapshot.data()); if (docSnapshot.data().currentTurn === 'response') { setResponses(getAvailableResponses(docSnapshot.data().selectedTaunt)); } } }); return () => unsubscribe(); }, [matchId]);
타이머 구성요소는 각 턴의 지속 시간을 제한합니다. 이 타임아웃 기능은 안정적인 게임 흐름을 유지하고, 시간 내에 행동하지 못한 플레이어에게 페널티를 주어 HP를 감소시킵니다.
const handleTauntSelection = async (taunt) => { const otherPlayer = currentPlayer === matchData.player1 ? matchData.player2 : matchData.player1; await updateDoc(doc(db, 'tauntWars_matches', matchId), { currentTurn: 'response', turn: otherPlayer, selectedTaunt: taunt.id, }); };
- Konva를 사용한 건강 및 공격용 애니메이션 CanvasComponent: React-konva를 사용하여 상태 변화 및 공격에 애니메이션을 적용합니다. 체력 막대는 도발에 따라 입거나 받는 피해를 시각적으로 나타내므로 참여도가 높아집니다.
const Timer = ({ isPlayerTurn, onTimeUp }) => { const [timeLeft, setTimeLeft] = useState(30); useEffect(() => { if (isPlayerTurn) { const interval = setInterval(() => { setTimeLeft(prev => { if (prev <= 1) { clearInterval(interval); onTimeUp(); return 0; } return prev - 1; }); }, 1000); return () => clearInterval(interval); } }, [isPlayerTurn, onTimeUp]); };
이런 방식으로 공격을 시뮬레이션함으로써 각 도발이나 대응의 위력과 결과를 시각적으로 표시하여 더욱 몰입도 높은 경험을 선사합니다.
- ChatBox를 통한 실시간 채팅 ChatBox 구성 요소는 도발 및 응답 메시지를 표시하는 실시간 채팅입니다. 이 채팅 인터페이스는 플레이어에게 피드백과 맥락을 제공하여 대화형 경험을 선사합니다.
const animateAttack = useCallback((attacker, defender) => { const targetX = attacker === 'player1' ? player1Pos.x + 50 : player2Pos.x - 50; const attackerRef = attacker === 'player1' ? player1Ref : player2Ref; attackerRef.current.to({ x: targetX, duration: 0.2, onFinish: () => attackerRef.current.to({ x: player1Pos.x, duration: 0.2 }) }); });
각 메시지는 사용자 ID를 기준으로 조건부로 렌더링되어 독특한 스타일로 주고받은 메시지를 구분합니다.
- ELO 순위가 포함된 리더보드 EloLeaderboard 구성 요소는 ELO 등급을 기준으로 플레이어를 정렬합니다. 각 경기는 실시간으로 가져와 표시되는 Firestore의 플레이어 등급을 업데이트합니다.
const ChatBox = ({ matchId }) => { const [messages, setMessages] = useState([]); useEffect(() => { const chatRef = collection(db, 'tauntWars_matches', matchId, 'chat'); const unsubscribe = onSnapshot(chatRef, (snapshot) => { setMessages(snapshot.docs.map((doc) => doc.data())); }); return () => unsubscribe(); }, [matchId]); };
리더보드는 ELO를 기준으로 플레이어 순위를 매기므로 플레이어가 경쟁 동기를 부여하고 진행 상황을 추적할 수 있습니다.
기술적 과제와 모범 사례
Firestore 트랜잭션과의 일관성
트랜잭션을 사용하면 특히 매치메이킹 및 점수 업데이트 중에 Firestore에 대한 동시 읽기/쓰기가 데이터 무결성을 유지하도록 보장합니다.
실시간 청취자 최적화
메모리 누수를 방지하려면 unsubscribe()를 사용하여 리스너 정리를 사용합니다. 또한 쿼리를 제한하면 Firestore 읽기 횟수를 줄여 비용과 성능을 최적화하는 데 도움이 될 수 있습니다.
캔버스를 활용한 반응형 디자인
CanvasComponent는 뷰포트에 따라 크기를 조정하여 게임이 여러 장치에서 반응하도록 만듭니다. React-konva 라이브러리를 사용하면 대화형 요소를 강력하게 렌더링하여 플레이어에게 애니메이션을 통해 시각적 피드백을 제공할 수 있습니다.
가장자리 케이스 처리
플레이어가 게임 도중에 연결을 끊는 시나리오를 생각해 보세요. 이를 위해 일치 데이터가 업데이트되고 중단된 일치 인스턴스가 닫히도록 하는 정리 기능을 구현하십시오.
마무리
Firebase와 React를 사용하면 실시간 사용자 작업에 적응하는 빠르게 진행되는 멀티플레이어 환경을 만들 수 있습니다. Gladiator Taunt Wars의 예는 실시간 업데이트, 보안 거래 및 동적 애니메이션을 통합하여 매력적이고 시각적으로 매력적인 게임을 제작하는 방법을 보여줍니다.
결론
Gladiators Battle을 위한 Gladiator Taunt Wars를 구축하는 것은 React, Firebase 및 몰입형 게임 메커니즘을 결합하여 웹 기반 게임에서 로마 경기장 전투의 강렬함을 포착하는 보람 있는 여정이었습니다. Firebase의 실시간 Firestore 데이터베이스, 보안 인증, 강력한 호스팅 기능을 활용하여 플레이어가 전략적 전투에서 대결할 수 있는 원활한 커뮤니티 중심 환경을 만들 수 있었습니다. 지속적인 배포를 위해 GitHub Actions를 통합하면 개발이 간소화되어 게임 플레이와 사용자 상호 작용을 향상하는 데 집중할 수 있습니다.
Gladiator Taunt Wars를 계속 확장하면서 AI 기반 상대와 향상된 매치 전략을 포함한 새로운 기능의 잠재력에 대해 기대하고 있습니다. 역사적 전략과 현대 기술의 결합은 플레이어가 검투사 세계에 참여할 수 있는 역동적인 방법을 제공합니다.
이 시리즈의 향후 기사에서는 실시간 데이터 흐름 최적화, 복잡한 게임 상태 관리, AI 활용을 통한 플레이어 참여 향상 등 Firebase를 사용하여 대화형 웹 애플리케이션을 만드는 기술적인 세부정보를 자세히 알아볼 것입니다. 반응성이 뛰어난 실시간 멀티플레이어 환경을 만들기 위해 프런트엔드와 백엔드 서비스를 연결하는 모범 사례를 살펴보겠습니다.
자신만의 대화형 게임을 개발 중이거나 Gladiators Battle의 기술에 대해 궁금하다면 이 시리즈는 Firebase를 사용하여 최신 웹 애플리케이션을 구축하는 데 대한 귀중한 통찰력을 제공합니다. 고대 역사와 최첨단 기술을 지속적으로 결합하여 오늘날의 디지털 세계에 맞는 검투사 전투의 즐거움을 재해석하는 과정에 참여하세요.
? 더 알아보기:
검투사 전투 탐험: https://gladiatorsbattle.com에서 로마 세계로 뛰어들어 전략과 전투를 경험하세요
GitHub를 확인하세요: https://github.com/HanGPIErr/Gladiators-Battle-Documentation에서 코드베이스와 기여를 확인하세요.
LinkedIn에서 연결: 내 프로젝트에 대한 업데이트를 보려면 LinkedIn에서 팔로우하세요 https://www.linkedin.com/in/pierre-romain-lopez/
내 X 계정도 있습니다: https://x.com/GladiatorsBT
위 내용은 React와 Firebase를 사용하여 실시간 멀티플레이어 게임 구축: Gladiator Taunt Wars의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
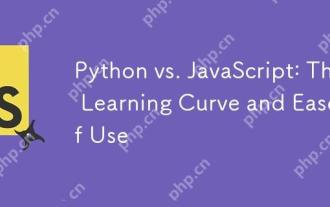
Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
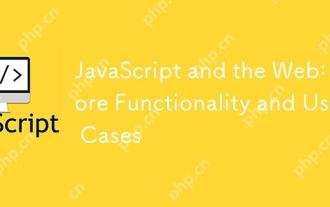
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.
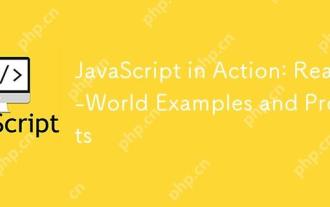
실제 세계에서 JavaScript의 응용 프로그램에는 프론트 엔드 및 백엔드 개발이 포함됩니다. 1) DOM 운영 및 이벤트 처리와 관련된 TODO 목록 응용 프로그램을 구축하여 프론트 엔드 애플리케이션을 표시합니다. 2) Node.js를 통해 RESTFULAPI를 구축하고 Express를 통해 백엔드 응용 프로그램을 시연하십시오.
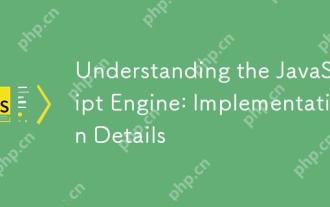
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.
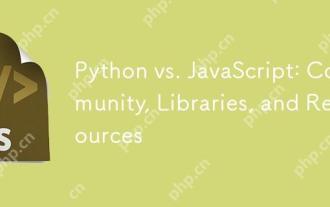
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
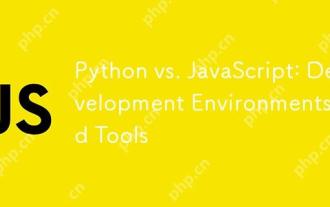
개발 환경에서 Python과 JavaScript의 선택이 모두 중요합니다. 1) Python의 개발 환경에는 Pycharm, Jupyternotebook 및 Anaconda가 포함되어 있으며 데이터 과학 및 빠른 프로토 타이핑에 적합합니다. 2) JavaScript의 개발 환경에는 Node.js, VScode 및 Webpack이 포함되어 있으며 프론트 엔드 및 백엔드 개발에 적합합니다. 프로젝트 요구에 따라 올바른 도구를 선택하면 개발 효율성과 프로젝트 성공률이 향상 될 수 있습니다.
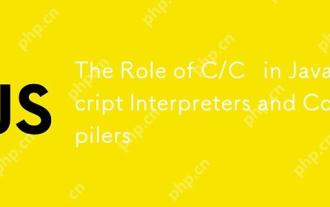
C와 C는 주로 통역사와 JIT 컴파일러를 구현하는 데 사용되는 JavaScript 엔진에서 중요한 역할을합니다. 1) C는 JavaScript 소스 코드를 구문 분석하고 추상 구문 트리를 생성하는 데 사용됩니다. 2) C는 바이트 코드 생성 및 실행을 담당합니다. 3) C는 JIT 컴파일러를 구현하고 런타임에 핫스팟 코드를 최적화하고 컴파일하며 JavaScript의 실행 효율을 크게 향상시킵니다.

Python은 데이터 과학 및 자동화에 더 적합한 반면 JavaScript는 프론트 엔드 및 풀 스택 개발에 더 적합합니다. 1. Python은 데이터 처리 및 모델링을 위해 Numpy 및 Pandas와 같은 라이브러리를 사용하여 데이터 과학 및 기계 학습에서 잘 수행됩니다. 2. 파이썬은 간결하고 자동화 및 스크립팅이 효율적입니다. 3. JavaScript는 프론트 엔드 개발에 없어서는 안될 것이며 동적 웹 페이지 및 단일 페이지 응용 프로그램을 구축하는 데 사용됩니다. 4. JavaScript는 Node.js를 통해 백엔드 개발에 역할을하며 전체 스택 개발을 지원합니다.
