Torpedo를 사용하여 첫 번째 프로젝트 만들기: 단계별 가이드
Golang에서 애플리케이션을 구축할 때 Hexagonal Architecture의 원칙을 준수하면 깔끔하고 모듈식이며 유지 관리가 가능한 코드를 보장할 수 있습니다. Torpedo를 사용하면 이 아키텍처를 쉽게 구현하는 동시에 개발 프로세스 속도를 높일 수 있습니다. 이 가이드에서는 설치부터 엔터티 생성 및 사용 사례까지 Torpedo를 사용하여 첫 번째 프로젝트를 만드는 방법을 안내합니다.
이 게시물은 문서화된 빠른 시작 가이드를 요약한 것입니다
1. 어뢰 시작하기
프로젝트 만들기에 앞서 시스템에 Go가 설치되어 있는지 확인하세요. 그런 다음 설치 가이드의 지침에 따라 Torpedo를 설치하세요
이 CLI 도구는 프로젝트 생성, 엔터티 생성 및 사용 사례 스캐폴딩을 처리합니다. 설치가 완료되면 첫 번째 프로젝트를 생성할 수 있습니다.
2. 첫 번째 프로젝트 설정
Torpedo로 만든 첫 번째 애플리케이션으로 구걸해 보세요!. Booking Fly라는 비행 예약 앱을 구축할 예정입니다.
Torpedo가 설치되어 있으면 실행만큼 간단하게 새 프로젝트를 만들 수 있습니다.
mkdir booking-fly && cd booking-fly
torpedo init
이 명령은 엔터티와 사용 사례를 정의해야 하는 .torpedo 폴더를 생성합니다. 이 폴더에 대한 자세한 내용은 .torpedo dir struct
에서 확인할 수 있습니다.3. 첫 번째 엔터티 정의
다음으로 도메인 엔터티를 정의해야 합니다. 엔터티는 사용자, 제품, 주문 등을 나타내는 애플리케이션 비즈니스 로직의 핵심 개체입니다.
첫 번째 엔터티를 정의하려면 .torpedo/entities 디렉터리 아래에 YAML 파일을 생성하세요. 예를 들어 간단한 User 엔터티를 만들어 보겠습니다.
.torpedo/entities/user.yaml
version: torpedo.darksub.io/v1.0 kind: entity spec: name: "user" plural: "users" description: "The frequent flyer user" doc: | The user entity represents a system user but also a frequent flyer. This entity is only for the example purpose. schema: reserved: id: type: ulid fields: - name: name type: string description: "The user full name" - name: email type: string description: "The user contact email" - name: password # it is not recommended to save passwords, this is an example only type: string encrypted: true description: "The user system password" - name: plan type: string description: "The user membership plan" validate: list: values: - GOLD - SILVER - BRONZE - name: miles type: integer description: "The accumulated flyer miles" relationships: - name: trips type: $rel ref: ".torpedo/entities/trip.yaml" cardinality: hasMany load: type: nested metadata: maxItems: 100 adapters: input: - type: http output: - type: memory
추가로 Trip 엔터티가 필요하므로 Trip 엔터티를 생성해 보겠습니다.
.torpedo/entities/trip.yaml
version: torpedo.darksub.io/v1.0 kind: entity spec: name: trip plural: trips description: "The user fly trip reservations" doc: | The trip entity handles all data related with the frequent flyer trip schema: reserved: id: type: ulid fields: - name: departure type: string description: "The trip departure airport" - name: arrival type: string description: "The trip arrival airport" - name: miles type: integer description: "The trip miles" - name: from type: date description: "The trip from date" - name: to type: date description: "The trip to date" adapters: input: - type: http output: - type: memory
Torpedo는 저장소 인터페이스 및 필요한 데이터베이스 처리 코드를 포함하여 해당 CRUD 작업과 함께 사용자 및 여행 엔터티에 대한 Go 코드를 생성합니다.
4. 사용 사례 만들기
엔티티가 준비되면 사용 사례를 사용하여 애플리케이션의 워크플로와 상호 작용하는 방법을 정의할 차례입니다. 사용 사례에는 기업에 적용되는 비즈니스 규칙과 프로세스가 요약되어 있습니다.
사용 사례를 정의하려면 .torpedo/use_cases 디렉터리 아래에 YAML 파일을 생성하세요. 다음은 항공편 예약에 대한 간단한 사용 사례의 예입니다.
.torpedo/use_cases/booking_fly.yaml
mkdir booking-fly && cd booking-fly
이 정의는 Torpedo에게 여행 및 사용자가 지정된 항공 예약을 처리하기 위한 사용자 정의 로직을 추가하기 위한 기본 코드를 생성하도록 지시합니다.
Torpedo는 엔터티와의 상호 작용을 포함하여 전체 사용 사례를 기반으로 합니다.
다음 단계(#5)를 완료한 후 사용 사례에서 생성된 스켈레톤 사용 사례 내에서 논리를 코딩하는 방법에 대해 알아보려면 빠른 시작 가이드를 읽어보세요
5. 모두 함께 배선
엔터티와 사용 사례를 정의하고 나면 Torpedo는 이러한 구성 요소 간의 연결이 육각형 아키텍처 원칙을 따르는지 확인합니다. 사용 사례는 서비스 인터페이스를 통해 엔터티와 상호 작용하는 반면, 어댑터(예: 데이터베이스 또는 API)는 지속성 및 외부 통신을 처리합니다.
이제 모든 것을 하나로 묶어 앱 사양을 작성할 차례입니다!. 여기에는 앱이 설명되어 있으므로 애플리케이션 정의는 가장 중요한 파일입니다. 다음 예는 Booking Fly 앱을 정의하는 방법을 보여줍니다.
.torpedo/app.yaml
torpedo init
애플리케이션 코드(엔티티, 사용 사례 등)를 생성하려면 다음 명령을 실행하세요.
version: torpedo.darksub.io/v1.0 kind: entity spec: name: "user" plural: "users" description: "The frequent flyer user" doc: | The user entity represents a system user but also a frequent flyer. This entity is only for the example purpose. schema: reserved: id: type: ulid fields: - name: name type: string description: "The user full name" - name: email type: string description: "The user contact email" - name: password # it is not recommended to save passwords, this is an example only type: string encrypted: true description: "The user system password" - name: plan type: string description: "The user membership plan" validate: list: values: - GOLD - SILVER - BRONZE - name: miles type: integer description: "The accumulated flyer miles" relationships: - name: trips type: $rel ref: ".torpedo/entities/trip.yaml" cardinality: hasMany load: type: nested metadata: maxItems: 100 adapters: input: - type: http output: - type: memory
이 명령은 육각형 아키텍처를 기반으로 디렉터리 구조를 설정하는 프로젝트 스캐폴드를 생성합니다. 프로젝트에는 엔터티, 사용 사례 및 어댑터에 대한 핵심 폴더가 포함됩니다. 이를 통해 비즈니스 로직과 인프라가 처음부터 분리된 상태로 유지됩니다.
이제 더 많은 엔터티, 사용 사례, 맞춤 어댑터를 추가하여 프로젝트를 확장할 수 있습니다. Torpedo의 구조를 통해 코드를 깔끔하고 모듈식으로 유지할 수 있으므로 애플리케이션이 성장함에 따라 쉽게 확장할 수 있습니다.
생성된 사용 사례 코드를 사용하여 자신만의 로직을 코딩하는 방법도 살펴보세요.
6. 애플리케이션 실행
엔티티와 사용 사례를 설정하고 나면 애플리케이션을 실행할 준비가 된 것입니다. Torpedo에는 테스트 및 개발을 위해 실행할 수 있는 Gin Gonic 프로젝트를 기반으로 하는 경량 서버가 포함되어 있습니다. 간단히 사용하세요:
종속성을 업데이트하기 전에 go mod tidy를 실행하는 것을 잊지 마세요!
version: torpedo.darksub.io/v1.0 kind: entity spec: name: trip plural: trips description: "The user fly trip reservations" doc: | The trip entity handles all data related with the frequent flyer trip schema: reserved: id: type: ulid fields: - name: departure type: string description: "The trip departure airport" - name: arrival type: string description: "The trip arrival airport" - name: miles type: integer description: "The trip miles" - name: from type: date description: "The trip from date" - name: to type: date description: "The trip to date" adapters: input: - type: http output: - type: memory
이제 애플리케이션의 API와 상호 작용하여 정의한 CRUD 작업 및 사용 사례를 실행할 수 있습니다.
7. 다음은 무엇입니까?
Torpedo를 사용하면 Hexagonal Architecture를 통해 깔끔하고 구조화된 Go 코드를 쉽게 생성할 수 있습니다. 하지만 이것은 시작에 불과합니다! 더 복잡한 워크플로우를 추가하고, 외부 서비스를 통합하고, 필요에 맞게 프레임워크를 사용자 정의하여 Torpedo의 기능을 계속 탐색할 수 있습니다.
곧 Torpedo에 추가될 고급 기능을 기대해 주시고, 가능한 기능을 탐색하면서 의견을 자유롭게 공유해 주세요!
결론
Torpedo를 사용하여 첫 번째 프로젝트를 만드는 것은 간단하고 빠릅니다. YAML의 엔터티 스키마와 사용 사례 정의의 강력한 기능을 활용하면 깔끔한 아키텍처 원칙을 유지하면서 강력한 Golang 애플리케이션을 빠르게 스캐폴딩할 수 있습니다. 이제 본격적으로 구축을 시작할 시간입니다! 귀하의 생각과 Torpedo가 귀하의 향후 프로젝트에 어떻게 도움이 될 수 있는지 알려주세요.
위 내용은 Torpedo를 사용하여 첫 번째 프로젝트 만들기: 단계별 가이드의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










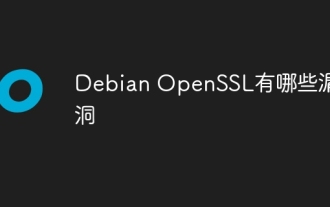
보안 통신에 널리 사용되는 오픈 소스 라이브러리로서 OpenSSL은 암호화 알고리즘, 키 및 인증서 관리 기능을 제공합니다. 그러나 역사적 버전에는 알려진 보안 취약점이 있으며 그 중 일부는 매우 유해합니다. 이 기사는 데비안 시스템의 OpenSSL에 대한 일반적인 취약점 및 응답 측정에 중점을 둘 것입니다. DebianopensSL 알려진 취약점 : OpenSSL은 다음과 같은 몇 가지 심각한 취약점을 경험했습니다. 심장 출혈 취약성 (CVE-2014-0160) :이 취약점은 OpenSSL 1.0.1 ~ 1.0.1F 및 1.0.2 ~ 1.0.2 베타 버전에 영향을 미칩니다. 공격자는이 취약점을 사용하여 암호화 키 등을 포함하여 서버에서 무단 읽기 민감한 정보를 사용할 수 있습니다.
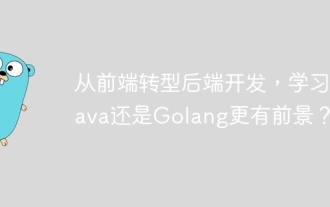
백엔드 학습 경로 : 프론트 엔드에서 백엔드 초보자로서 프론트 엔드에서 백엔드까지의 탐사 여행은 프론트 엔드 개발에서 변화하는 백엔드 초보자로서 이미 Nodejs의 기초를 가지고 있습니다.
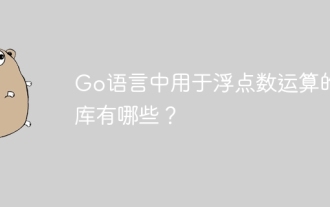
Go Language의 부동 소수점 번호 작동에 사용되는 라이브러리는 정확도를 보장하는 방법을 소개합니다.
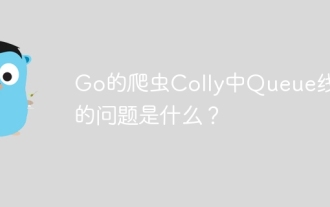
Go Crawler Colly의 대기열 스레딩 문제는 Colly Crawler 라이브러리를 GO 언어로 사용하는 문제를 탐구합니다. � ...
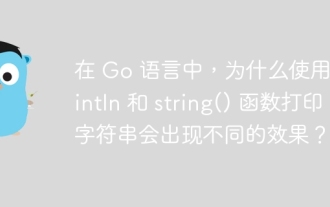
Go Language의 문자열 인쇄의 차이 : println 및 String () 함수 사용 효과의 차이가 진행 중입니다 ...
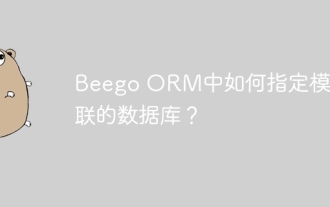
Beegoorm 프레임 워크에서 모델과 관련된 데이터베이스를 지정하는 방법은 무엇입니까? 많은 Beego 프로젝트에서는 여러 데이터베이스를 동시에 작동해야합니다. Beego를 사용할 때 ...
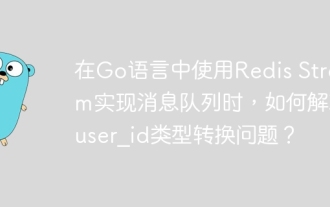
Go Language에서 메시지 대기열을 구현하기 위해 Redisstream을 사용하는 문제는 Go Language와 Redis를 사용하는 것입니다 ...
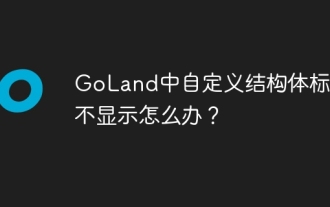
골란드의 사용자 정의 구조 레이블이 표시되지 않으면 어떻게해야합니까? Go Language 개발을 위해 Goland를 사용할 때 많은 개발자가 사용자 정의 구조 태그를 만날 것입니다 ...
