C의 문자열에서 선행 및 후행 공백을 자르는 방법은 무엇입니까?
문자열에서 선행 및 후행 공백 제거
C에서 문자열 객체의 선행 및 후행 공백을 제거하는 것은 일반적인 작업입니다. 문자열 클래스에는 이를 수행하는 기본 메소드가 없지만 문자열 조작 기술의 조합을 통해 달성할 수 있습니다.
선행 및 후행 공백을 제거하려면 find_first_not_of 및 find_last_not_of 함수를 사용하여 첫 번째와 마지막을 식별할 수 있습니다. 문자열의 공백이 아닌 문자. 이러한 위치가 결정되면 substr 함수를 사용하여 앞뒤 공백 없이 부분 문자열을 추출할 수 있습니다.
#include <string> std::string trim(const std::string& str) { const auto strBegin = str.find_first_not_of(" "); if (strBegin == std::string::npos) { return ""; } const auto strEnd = str.find_last_not_of(" "); const auto strRange = strEnd - strBegin + 1; return str.substr(strBegin, strRange); }
추가 공백을 줄이기 위해 서식 확장
문자열에서 단어 사이의 추가 공백을 제거하려면 보다 포괄적인 접근 방식이 필요합니다. 이는 find_first_of, find_last_not_of 및 substr 함수를 반복적으로 사용하여 공백의 하위 범위를 자리 표시자 문자 또는 문자열로 바꾸면 달성할 수 있습니다.
std::string reduce(const std::string& str, const std::string& fill = " ") { auto result = trim(str); auto beginSpace = result.find_first_of(" "); while (beginSpace != std::string::npos) { const auto endSpace = result.find_first_not_of(" ", beginSpace); const auto range = endSpace - beginSpace; result.replace(beginSpace, range, fill); const auto newStart = beginSpace + fill.length(); beginSpace = result.find_first_of(" ", newStart); } return result; }
사용 예
다음 코드 조각은 이러한 사용 방법을 보여줍니다. 함수:
const std::string foo = " too much\t \tspace\t\t\t "; const std::string bar = "one\ntwo"; std::cout << "[" << trim(foo) << "]" << std::endl; std::cout << "[" << reduce(foo) << "]" << std::endl; std::cout << "[" << reduce(foo, "-") << "]" << std::endl; std::cout << "[" << trim(bar) << "]" << std::endl;
이 코드는 다음과 같은 출력을 생성합니다.
[too much space] [too much space] [too-much-space] [one two]
위 내용은 C의 문자열에서 선행 및 후행 공백을 자르는 방법은 무엇입니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
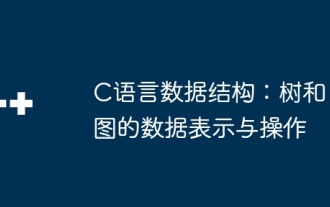
C 언어 데이터 구조 : 트리 및 그래프의 데이터 표현은 노드로 구성된 계층 적 데이터 구조입니다. 각 노드에는 데이터 요소와 하위 노드에 대한 포인터가 포함되어 있습니다. 이진 트리는 특별한 유형의 트리입니다. 각 노드에는 최대 두 개의 자식 노드가 있습니다. 데이터는 structtreenode {intdata; structtreenode*왼쪽; structReenode*오른쪽;}을 나타냅니다. 작업은 트리 트래버스 트리 (사전 조정, 인 순서 및 나중에 순서) 검색 트리 삽입 노드 삭제 노드 그래프는 요소가 정점 인 데이터 구조 모음이며 이웃을 나타내는 오른쪽 또는 무의미한 데이터로 모서리를 통해 연결할 수 있습니다.
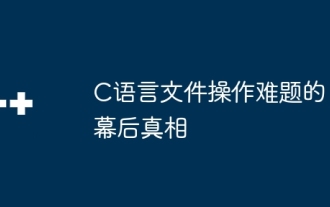
파일 작동 문제에 대한 진실 : 파일 개방이 실패 : 불충분 한 권한, 잘못된 경로 및 파일이 점유 된 파일. 데이터 쓰기 실패 : 버퍼가 가득 차고 파일을 쓸 수 없으며 디스크 공간이 불충분합니다. 기타 FAQ : 파일이 느리게 이동, 잘못된 텍스트 파일 인코딩 및 이진 파일 읽기 오류.
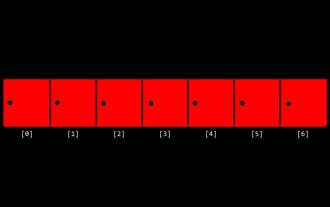
알고리즘은 문제를 해결하기위한 일련의 지침이며 실행 속도 및 메모리 사용량은 다양합니다. 프로그래밍에서 많은 알고리즘은 데이터 검색 및 정렬을 기반으로합니다. 이 기사에서는 여러 데이터 검색 및 정렬 알고리즘을 소개합니다. 선형 검색은 배열 [20,500,10,5,100,1,50]이 있으며 숫자 50을 찾아야한다고 가정합니다. 선형 검색 알고리즘은 대상 값이 발견되거나 전체 배열이 통과 될 때까지 배열의 각 요소를 하나씩 점검합니다. 알고리즘 플로우 차트는 다음과 같습니다. 선형 검색의 의사 코드는 다음과 같습니다. 각 요소를 확인하십시오. 대상 값이 발견되는 경우 : true return false clanue 구현 : #includeintmain (void) {i 포함
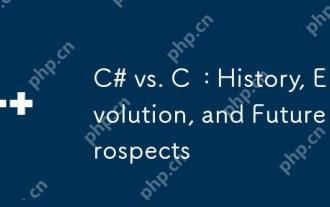
C#과 C의 역사와 진화는 독특하며 미래의 전망도 다릅니다. 1.C는 1983 년 Bjarnestroustrup에 의해 발명되어 객체 지향 프로그래밍을 C 언어에 소개했습니다. Evolution 프로세스에는 자동 키워드 소개 및 Lambda Expressions 소개 C 11, C 20 도입 개념 및 코 루틴과 같은 여러 표준화가 포함되며 향후 성능 및 시스템 수준 프로그래밍에 중점을 둘 것입니다. 2.C#은 2000 년 Microsoft에 의해 출시되었으며 C와 Java의 장점을 결합하여 진화는 단순성과 생산성에 중점을 둡니다. 예를 들어, C#2.0은 제네릭과 C#5.0 도입 된 비동기 프로그래밍을 소개했으며, 이는 향후 개발자의 생산성 및 클라우드 컴퓨팅에 중점을 둘 것입니다.
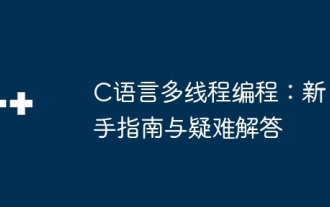
C 언어 멀티 스레딩 프로그래밍 안내서 : 스레드 생성 : pthread_create () 함수를 사용하여 스레드 ID, 속성 및 스레드 함수를 지정합니다. 스레드 동기화 : 뮤텍스, 세마포어 및 조건부 변수를 통한 데이터 경쟁 방지. 실제 사례 : 멀티 스레딩을 사용하여 Fibonacci 번호를 계산하고 여러 스레드에 작업을 할당하고 결과를 동기화하십시오. 문제 해결 : 프로그램 충돌, 스레드 정지 응답 및 성능 병목 현상과 같은 문제를 해결합니다.
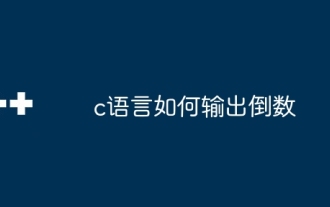
C에서 카운트 다운을 출력하는 방법? 답변 : 루프 명령문을 사용하십시오. 단계 : 1. 변수 n을 정의하고 카운트 다운 번호를 출력에 저장합니다. 2. n이 1보다 작을 때까지 n을 지속적으로 인쇄하려면 while 루프를 사용하십시오. 3. 루프 본체에서 n의 값을 인쇄하십시오. 4. 루프가 끝나면 n을 1 씩 빼기 위해 다음 작은 상호 상호를 출력합니다.
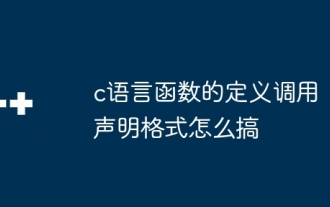
C 언어 기능에는 정의, 호출 및 선언이 포함됩니다. 함수 정의 함수 이름, 매개 변수 및 반환 유형, 기능 본체 구현 함수를 지정합니다. 함수 호출 기능 실행 및 매개 변수를 제공합니다. 함수 선언은 기능 유형의 컴파일러에 알려줍니다. 값 패스는 매개 변수 패스에 사용되며, 반환 유형에주의를 기울이고, 일관된 코드 스타일을 유지하며, 기능의 오류를 처리합니다. 이 지식을 마스터하면 우아하고 강력한 C 코드를 작성하는 데 도움이 될 수 있습니다.
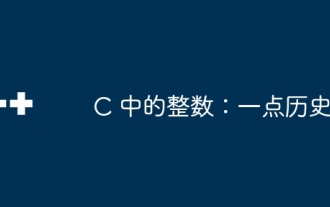
정수는 프로그래밍에서 가장 기본적인 데이터 유형이며 프로그래밍의 초석으로 간주 될 수 있습니다. 프로그래머의 임무는 이러한 숫자의 의미를 부여하는 것입니다. 소프트웨어가 아무리 복잡하더라도, 프로세서는 정수 만 이해하기 때문에 궁극적으로 정수 작업으로 이어집니다. 음수를 나타내기 위해, 우리는 2의 보완을 도입했다. 소수점 숫자를 나타내려면 과학적 표기법을 만들었으므로 부동 소수점 숫자가 있습니다. 그러나 최종 분석에서는 모든 것이 여전히 0과 1에서 분리 할 수 없습니다. C의 정수의 간단한 기록은 거의 기본 유형입니다. 컴파일러가 경고를 발행 할 수 있지만, 많은 경우에는 다음과 같은 코드를 작성할 수 있습니다. 메인 (void) {return0;} 기술적 관점에서 다음 코드와 동일합니다. intmain (void) {return0;}.
