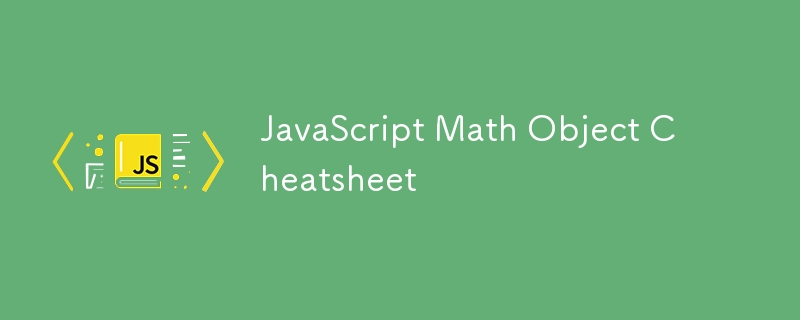
JavaScript의 Math 객체는 수학 작업을 수행하기 위한 속성 및 메서드 집합을 제공합니다. Math 개체에 대한 포괄적인 치트 시트는 다음과 같습니다.
속성
Math 객체에는 상수 세트가 있습니다.
Property |
Description |
Value (Approx.) |
Math.E |
Euler's number |
2.718 |
Math.LN2 |
Natural logarithm of 2 |
0.693 |
Math.LN10 |
Natural logarithm of 10 |
2.302 |
Math.LOG2E |
Base 2 logarithm of Math.E
|
1.442 |
Math.LOG10E |
Base 10 logarithm of Math.E
|
0.434 |
Math.PI |
Ratio of a circle's circumference to its diameter |
3.14159 |
Math.SQRT1_2 |
Square root of 1/2 |
0.707 |
Math.SQRT2 |
Square root of 2 |
1.414 |
방법
1. 반올림 방법
Method |
Description |
Example |
Math.round(x) |
Rounds to the nearest integer |
Math.round(4.5) → 5
|
Math.floor(x) |
Rounds down to the nearest integer |
Math.floor(4.7) → 4
|
Math.ceil(x) |
Rounds up to the nearest integer |
Math.ceil(4.1) → 5
|
Math.trunc(x) |
Removes the decimal part (truncates) |
Math.trunc(4.9) → 4
|
2. 난수 생성
Method |
Description |
Example |
Math.random() |
Generates a random number between 0 and 1 (exclusive) |
Math.random() → 0.53
|
Custom Random Int Generator |
Random integer between min and max
|
Math.floor(Math.random() * (max - min 1)) min |
3. 산술 방법
Method |
Description |
Example |
Math.abs(x) |
Absolute value |
Math.abs(-7) → 7
|
Math.pow(x, y) |
Raises x to the power of y
|
Math.pow(2, 3) → 8
|
Math.sqrt(x) |
Square root of x
|
Math.sqrt(16) → 4
|
Math.cbrt(x) |
Cube root of x
|
Math.cbrt(27) → 3
|
Math.hypot(...values) |
Square root of the sum of squares of arguments |
Math.hypot(3, 4) → 5
|
4. 지수 및 로그 방법
Method |
Description |
Example |
Math.exp(x) |
e^x |
Math.exp(1) → 2.718
|
Math.log(x) |
Natural logarithm (ln(x)) |
Math.log(10) → 2.302
|
Math.log2(x) |
Base 2 logarithm of x
|
Math.log2(8) → 3
|
Math.log10(x) |
Base 10 logarithm of x
|
Math.log10(100) → 2
|
5. 삼각법
Method |
Description |
Example |
Math.sin(x) |
Sine of x (x in radians) |
Math.sin(Math.PI / 2) → 1
|
Math.cos(x) |
Cosine of x (x in radians) |
Math.cos(0) → 1
|
Math.tan(x) |
Tangent of x (x in radians) |
Math.tan(Math.PI / 4) → 1
|
Math.asin(x) |
Arcsine of x (returns radians) |
Math.asin(1) → 1.57
|
Math.acos(x) |
Arccosine of x
|
Math.acos(1) → 0
|
Math.atan(x) |
Arctangent of x
|
Math.atan(1) → 0.785
|
Math.atan2(y, x) |
Arctangent of y / x
|
Math.atan2(1, 1) → 0.785
|
6. 최소, 최대 및 클램핑
Method |
Description |
Example |
Math.max(...values) |
Returns the largest value |
Math.max(5, 10, 15) → 15
|
Math.min(...values) |
Returns the smallest value |
Math.min(5, 10, 15) → 5
|
Custom Clamping |
Restrict a value to a range |
Math.min(Math.max(x, min), max) |
7. 다른 방법
Method |
Description |
Example |
Math.sign(x) |
Returns 1, -1, or 0 based on sign of x
|
Math.sign(-10) → -1
|
Math.fround(x) |
Nearest 32-bit floating-point number |
Math.fround(5.5) → 5.5
|
Math.clz32(x) |
Counts leading zero bits in 32-bit binary |
Math.clz32(1) → 31
|
예
1에서 100 사이의 임의의 정수
const randomInt = Math.floor(Math.random() * 100) + 1;
console.log(randomInt);
로그인 후 복사
원 면적 계산
const radius = 5;
const area = Math.PI * Math.pow(radius, 2);
console.log(area); // 78.54
로그인 후 복사
로그인 후 복사
각도를 라디안으로 변환
const degrees = 90;
const radians = degrees * (Math.PI / 180);
console.log(radians); // 1.57
로그인 후 복사
배열에서 가장 큰 숫자 찾기
const nums = [5, 3, 9, 1];
console.log(Math.max(...nums)); // 9
로그인 후 복사
수학 객체의 확장된 사용 사례
Math 객체에는 실용적인 응용 프로그램이 많이 있습니다. 다음은 이를 효과적으로 사용하는 방법을 보여주는 일반적인 시나리오 및 예시 목록입니다.
1. 무작위화
범위 내에서 임의의 정수 생성
function getRandomInt(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
console.log(getRandomInt(1, 10)); // Random number between 1 and 10
로그인 후 복사
배열 섞기
function shuffleArray(arr) {
return arr.sort(() => Math.random() - 0.5);
}
console.log(shuffleArray([1, 2, 3, 4, 5])); // Shuffled array
로그인 후 복사
주사위 굴리기 시뮬레이션
function rollDice() {
return Math.floor(Math.random() * 6) + 1; // Random number between 1 and 6
}
console.log(rollDice());
로그인 후 복사
2. 기하학과 모양
원의 면적 계산
const radius = 5;
const area = Math.PI * Math.pow(radius, 2);
console.log(area); // 78.54
로그인 후 복사
로그인 후 복사
삼각형의 빗변 계산
const a = 3, b = 4;
const hypotenuse = Math.hypot(a, b);
console.log(hypotenuse); // 5
로그인 후 복사
각도를 라디안으로 변환
function degreesToRadians(degrees) {
return degrees * (Math.PI / 180);
}
console.log(degreesToRadians(90)); // 1.57
로그인 후 복사
3. 금융과 비즈니스
복리 공식
function compoundInterest(principal, rate, time, n) {
return principal * Math.pow((1 + rate / n), n * time);
}
console.log(compoundInterest(1000, 0.05, 10, 12)); // 47.01
로그인 후 복사
통화 값 반올림
const amount = 19.56789;
const rounded = Math.round(amount * 100) / 100; // Round to 2 decimal places
console.log(rounded); // 19.57
로그인 후 복사
할인 계산
function calculateDiscount(price, discount) {
return Math.floor(price * (1 - discount / 100));
}
console.log(calculateDiscount(200, 15)); // 0
로그인 후 복사
4. 게임과 애니메이션
동전 던지기 시뮬레이션
function coinToss() {
return Math.random() < 0.5 ? 'Heads' : 'Tails';
}
console.log(coinToss());
로그인 후 복사
부드러운 애니메이션을 위한 이징 기능
function easeOutQuad(t) {
return t * (2 - t); // Simple easing function
}
console.log(easeOutQuad(0.5)); // 0.75
로그인 후 복사
2D 그리드의 무작위 생성 좌표
function randomCoordinates(gridSize) {
const x = Math.floor(Math.random() * gridSize);
const y = Math.floor(Math.random() * gridSize);
return { x, y };
}
console.log(randomCoordinates(10)); // e.g., {x: 7, y: 2}
로그인 후 복사
5. 데이터 분석
배열에서 최대값과 최소값 찾기
const scores = [85, 90, 78, 92, 88];
console.log(Math.max(...scores)); // 92
console.log(Math.min(...scores)); // 78
로그인 후 복사
데이터 정규화
function normalize(value, min, max) {
return (value - min) / (max - min);
}
console.log(normalize(75, 0, 100)); // 0.75
로그인 후 복사
6. 물리학과 공학
자유 낙하 후 속도 계산
const gravity = 9.8; // m/s^2
const time = 3; // seconds
const velocity = gravity * time;
console.log(velocity); // 29.4 m/s
로그인 후 복사
진자의 기간
function pendulumPeriod(length) {
return 2 * Math.PI * Math.sqrt(length / 9.8);
}
console.log(pendulumPeriod(1)); // 2.006 seconds
로그인 후 복사
7. 숫자조작
범위 내에서 숫자 고정
function clamp(value, min, max) {
return Math.min(Math.max(value, min), max);
}
console.log(clamp(15, 10, 20)); // 15
console.log(clamp(5, 10, 20)); // 10
로그인 후 복사
음수를 양수로 변환
console.log(Math.abs(-42)); // 42
로그인 후 복사
숫자의 정수 부분 찾기
console.log(Math.trunc(4.9)); // 4
console.log(Math.trunc(-4.9)); // -4
로그인 후 복사
8. 문제해결
숫자가 2의 거듭제곱인지 확인
function isPowerOfTwo(n) {
return Math.log2(n) % 1 === 0;
}
console.log(isPowerOfTwo(8)); // true
console.log(isPowerOfTwo(10)); // false
로그인 후 복사
피보나치 수 생성
function fibonacci(n) {
const phi = (1 + Math.sqrt(5)) / 2;
return Math.round((Math.pow(phi, n) - Math.pow(-phi, -n)) / Math.sqrt(5));
}
console.log(fibonacci(10)); // 55
로그인 후 복사
9. 기타
임의 색상(RGB) 생성
function getRandomColor() {
const r = Math.floor(Math.random() * 256);
const g = Math.floor(Math.random() * 256);
const b = Math.floor(Math.random() * 256);
return `rgb(${r}, ${g}, ${b})`;
}
console.log(getRandomColor()); // e.g., rgb(123, 45, 67)
로그인 후 복사
생년월일로 나이 계산
function calculateAge(birthYear) {
const currentYear = new Date().getFullYear();
return currentYear - birthYear;
}
console.log(calculateAge(1990)); // e.g., 34
로그인 후 복사
위 내용은 JavaScript 수학 객체 치트시트의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!