JavaScript 날짜 객체 치트시트
JavaScript의 날짜 개체는 날짜와 시간 작업에 사용됩니다. 날짜 및 시간 값을 생성, 조작 및 형식 지정하는 방법을 제공합니다.
날짜 만들기
다음과 같은 여러 가지 방법으로 날짜 개체를 만들 수 있습니다.
- 현재 날짜 및 시간:
const now = new Date(); console.log(now); // Current date and time
- 구체적인 날짜:
const specificDate = new Date(2024, 10, 21); // Year, Month (0-based), Day console.log(specificDate); // Thu Nov 21 2024
- 문자열에서:
const fromString = new Date("2024-11-21T10:00:00"); console.log(fromString); // Thu Nov 21 2024 10:00:00 GMT
- 타임스탬프에서(Unix 시대 이후의 밀리초):
const fromTimestamp = new Date(1732231200000); console.log(fromTimestamp); // Thu Nov 21 2024 10:00:00 GMT
일반적인 방법
날짜 및 시간 가져오기
Method | Description | Example |
---|---|---|
getFullYear() | Returns the year | date.getFullYear() -> 2024 |
getMonth() | Returns the month (0-11) | date.getMonth() -> 10 (November) |
getDate() | Returns the day of the month (1-31) | date.getDate() -> 21 |
getDay() | Returns the weekday (0-6, Sun=0) | date.getDay() -> 4 (Thursday) |
getHours() | Returns the hour (0-23) | date.getHours() -> 10 |
getMinutes() | Returns the minutes (0-59) | date.getMinutes() -> 0 |
getSeconds() | Returns the seconds (0-59) | date.getSeconds() -> 0 |
getTime() | Returns timestamp in milliseconds | date.getTime() -> 1732231200000 |
설명
Method | Description | Example |
---|---|---|
setFullYear(year) | Sets the year | date.setFullYear(2025) |
setMonth(month) | Sets the month (0-11) | date.setMonth(0) -> January |
setDate(day) | Sets the day of the month | date.setDate(1) -> First day of the month |
setHours(hour) | Sets the hour (0-23) | date.setHours(12) |
setMinutes(minutes) | Sets the minutes (0-59) | date.setMinutes(30) |
setSeconds(seconds) | Sets the seconds (0-59) | date.setSeconds(45) |
날짜 형식 지정
Method | Description | Example |
---|---|---|
toDateString() | Returns date as a human-readable string | date.toDateString() -> "Thu Nov 21 2024" |
toISOString() | Returns date in ISO format | date.toISOString() -> "2024-11-21T10:00:00.000Z" |
toLocaleDateString() | Returns date in localized format | date.toLocaleDateString() -> "11/21/2024" |
toLocaleTimeString() | Returns time in localized format | date.toLocaleTimeString() -> "10:00:00 AM" |
설명
- 일반적인 사용 사례
const now = new Date(); console.log(now); // Current date and time
- 두 날짜 사이의 날짜 계산
- :
const specificDate = new Date(2024, 10, 21); // Year, Month (0-based), Day console.log(specificDate); // Thu Nov 21 2024
- 카운트다운 타이머
- :
const fromString = new Date("2024-11-21T10:00:00"); console.log(fromString); // Thu Nov 21 2024 10:00:00 GMT
- 현재 날짜 형식
- :
const fromTimestamp = new Date(1732231200000); console.log(fromTimestamp); // Thu Nov 21 2024 10:00:00 GMT
- 요일 찾기
- :
const startDate = new Date("2024-11-01"); const endDate = new Date("2024-11-21"); const diffInTime = endDate - startDate; // Difference in milliseconds const diffInDays = diffInTime / (1000 * 60 * 60 * 24); // Convert to days console.log(diffInDays); // 20
- 윤년 확인
- :
const targetDate = new Date("2024-12-31T23:59:59"); setInterval(() => { const now = new Date(); const timeLeft = targetDate - now; // Milliseconds left const days = Math.floor(timeLeft / (1000 * 60 * 60 * 24)); const hours = Math.floor((timeLeft / (1000 * 60 * 60)) % 24); const minutes = Math.floor((timeLeft / (1000 * 60)) % 60); const seconds = Math.floor((timeLeft / 1000) % 60); console.log(`${days}d ${hours}h ${minutes}m ${seconds}s`); }, 1000);
일수 더하기/빼기
:
- 전문가의 팁
const now = new Date(); const formatted = `${now.getFullYear()}-${now.getMonth() + 1}-${now.getDate()}`; console.log(formatted); // "2024-11-21"
- Date.now()
- 를 사용하세요.
지역 간 날짜 작업 시 시간대
에 주의하세요. 고급 처리를 위해 Moment.js - 또는
Day.js와 같은 라이브러리를 사용하세요.
0부터 시작됩니다
위 내용은 JavaScript 날짜 객체 치트시트의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
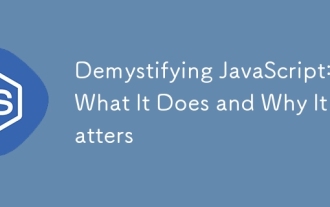
JavaScript는 현대 웹 개발의 초석이며 주요 기능에는 이벤트 중심 프로그래밍, 동적 컨텐츠 생성 및 비동기 프로그래밍이 포함됩니다. 1) 이벤트 중심 프로그래밍을 사용하면 사용자 작업에 따라 웹 페이지가 동적으로 변경 될 수 있습니다. 2) 동적 컨텐츠 생성을 사용하면 조건에 따라 페이지 컨텐츠를 조정할 수 있습니다. 3) 비동기 프로그래밍은 사용자 인터페이스가 차단되지 않도록합니다. JavaScript는 웹 상호 작용, 단일 페이지 응용 프로그램 및 서버 측 개발에 널리 사용되며 사용자 경험 및 크로스 플랫폼 개발의 유연성을 크게 향상시킵니다.
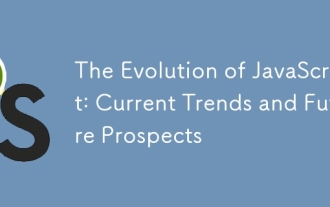
JavaScript의 최신 트렌드에는 Typescript의 Rise, 현대 프레임 워크 및 라이브러리의 인기 및 WebAssembly의 적용이 포함됩니다. 향후 전망은보다 강력한 유형 시스템, 서버 측 JavaScript 개발, 인공 지능 및 기계 학습의 확장, IoT 및 Edge 컴퓨팅의 잠재력을 포함합니다.
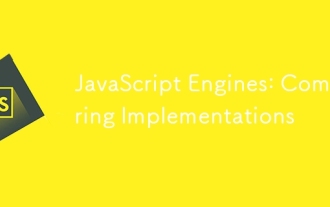
각각의 엔진의 구현 원리 및 최적화 전략이 다르기 때문에 JavaScript 엔진은 JavaScript 코드를 구문 분석하고 실행할 때 다른 영향을 미칩니다. 1. 어휘 분석 : 소스 코드를 어휘 단위로 변환합니다. 2. 문법 분석 : 추상 구문 트리를 생성합니다. 3. 최적화 및 컴파일 : JIT 컴파일러를 통해 기계 코드를 생성합니다. 4. 실행 : 기계 코드를 실행하십시오. V8 엔진은 즉각적인 컴파일 및 숨겨진 클래스를 통해 최적화하여 Spidermonkey는 유형 추론 시스템을 사용하여 동일한 코드에서 성능이 다른 성능을 제공합니다.
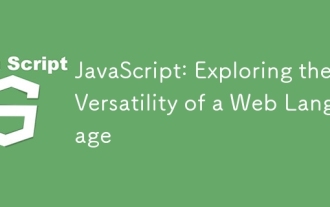
JavaScript는 현대 웹 개발의 핵심 언어이며 다양성과 유연성에 널리 사용됩니다. 1) 프론트 엔드 개발 : DOM 운영 및 최신 프레임 워크 (예 : React, Vue.js, Angular)를 통해 동적 웹 페이지 및 단일 페이지 응용 프로그램을 구축합니다. 2) 서버 측 개발 : Node.js는 비 차단 I/O 모델을 사용하여 높은 동시성 및 실시간 응용 프로그램을 처리합니다. 3) 모바일 및 데스크탑 애플리케이션 개발 : 크로스 플랫폼 개발은 개발 효율을 향상시키기 위해 반응 및 전자를 통해 실현됩니다.
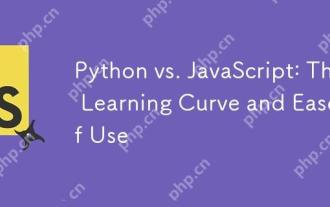
Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
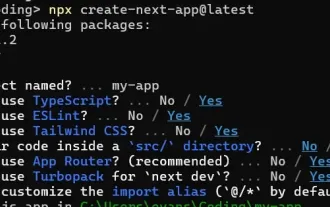
이 기사에서는 Contrim에 의해 확보 된 백엔드와의 프론트 엔드 통합을 보여 주며 Next.js를 사용하여 기능적인 Edtech SaaS 응용 프로그램을 구축합니다. Frontend는 UI 가시성을 제어하기 위해 사용자 권한을 가져오고 API가 역할 기반을 준수하도록합니다.
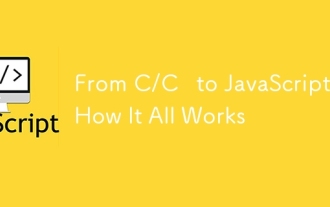
C/C에서 JavaScript로 전환하려면 동적 타이핑, 쓰레기 수집 및 비동기 프로그래밍으로 적응해야합니다. 1) C/C는 수동 메모리 관리가 필요한 정적으로 입력 한 언어이며 JavaScript는 동적으로 입력하고 쓰레기 수집이 자동으로 처리됩니다. 2) C/C를 기계 코드로 컴파일 해야하는 반면 JavaScript는 해석 된 언어입니다. 3) JavaScript는 폐쇄, 프로토 타입 체인 및 약속과 같은 개념을 소개하여 유연성과 비동기 프로그래밍 기능을 향상시킵니다.
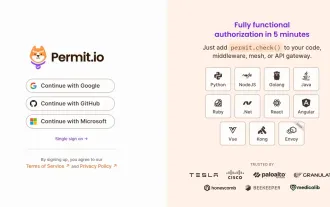
일상적인 기술 도구를 사용하여 기능적 다중 테넌트 SaaS 응용 프로그램 (Edtech 앱)을 구축했으며 동일한 작업을 수행 할 수 있습니다. 먼저, 다중 테넌트 SaaS 응용 프로그램은 무엇입니까? 멀티 테넌트 SAAS 응용 프로그램은 노래에서 여러 고객에게 서비스를 제공 할 수 있습니다.
